Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Stylus / RawStylusInput.cs / 1 / RawStylusInput.cs
using System; using System.Collections; using System.Windows.Media; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input.StylusPlugIns { ///////////////////////////////////////////////////////////////////////// ////// [TBS] /// public class RawStylusInput { ///////////////////////////////////////////////////////////////////// ////// [TBS] /// /// [TBS] /// [TBS] /// [TBS] internal RawStylusInput( RawStylusInputReport report, GeneralTransform tabletToElementTransform, StylusPlugInCollection targetPlugInCollection) { if (report == null) { throw new ArgumentNullException("report"); } if (tabletToElementTransform.Inverse == null) { throw new ArgumentException(SR.Get(SRID.Stylus_MatrixNotInvertable), "tabletToElementTransform"); } if (targetPlugInCollection == null) { throw new ArgumentNullException("targetPlugInCollection"); } // We should always see this GeneralTransform is frozen since we access this from multiple threads. System.Diagnostics.Debug.Assert(tabletToElementTransform.IsFrozen); _report = report; _tabletToElementTransform = tabletToElementTransform; _targetPlugInCollection = targetPlugInCollection; } ////// /// public int StylusDeviceId { get { return _report.StylusDeviceId; } } ////// /// public int TabletDeviceId { get { return _report.TabletDeviceId; } } ////// /// public int Timestamp { get { return _report.Timestamp; } } ////// Returns a copy of the StylusPoints /// public StylusPointCollection GetStylusPoints() { return GetStylusPoints(Transform.Identity); } ////// Internal method called by StylusDevice to prevent two copies /// ////// Critical: This code accesses InputReport.InputSource /// TreatAsSafe: The method only gets a transform from PresentationSource and returns StylusPointCollection. /// Those operations are considered safe. /// This method is called by: /// RawStylusInput.GetStylusPoints /// StylusDevice.UpdateEventStylusPoints(RawStylusInputReport, Boolean) /// [SecurityCritical, SecurityTreatAsSafe] internal StylusPointCollection GetStylusPoints(GeneralTransform transform) { if (_stylusPoints == null) { // GeneralTransformGroup group = new GeneralTransformGroup(); if ( StylusDeviceId == 0) { // Only do this for the Mouse group.Children.Add(new MatrixTransform(_report.InputSource.CompositionTarget.TransformFromDevice)); } group.Children.Add(_tabletToElementTransform); group.Children.Add(transform); return new StylusPointCollection(_report.StylusPointDescription, _report.GetRawPacketData(), group, Matrix.Identity); } else { return _stylusPoints.Clone(transform, _stylusPoints.Description); } } ////// Replaces the StylusPoints. /// ////// Callers must have Unmanaged code permission to call this API. /// /// stylusPoints ////// Callers must have Unmanaged code permission to call this API. /// public void SetStylusPoints(StylusPointCollection stylusPoints) { // To modify the points we require Unmanaged code permission. SecurityHelper.DemandUnmanagedCode(); if (null == stylusPoints) { throw new ArgumentNullException("stylusPoints"); } if (!StylusPointDescription.AreCompatible( stylusPoints.Description, _report.StylusPointDescription)) { throw new ArgumentException(SR.Get(SRID.IncompatibleStylusPointDescriptions), "stylusPoints"); } if (stylusPoints.Count == 0) { throw new ArgumentException(SR.Get(SRID.Stylus_StylusPointsCantBeEmpty), "stylusPoints"); } _stylusPoints = stylusPoints.Clone(); } ////// Returns the RawStylusInputCustomDataList used to notify plugins before /// PreviewStylus event has been processed by application. /// public void NotifyWhenProcessed(object callbackData) { if (_currentNotifyPlugIn == null) { throw new InvalidOperationException(SR.Get(SRID.Stylus_CanOnlyCallForDownMoveOrUp)); } if (_customData == null) { _customData = new RawStylusInputCustomDataList(); } _customData.Add(new RawStylusInputCustomData(_currentNotifyPlugIn, callbackData)); } ////// True if a StylusPlugIn has modifiedthe StylusPoints. /// internal bool StylusPointsModified { get { return _stylusPoints != null; } } ////// Target StylusPlugInCollection that real time pen input sent to. /// internal StylusPlugInCollection Target { get { return _targetPlugInCollection; } } ////// Real RawStylusInputReport that this report is generated from. /// internal RawStylusInputReport Report { get { return _report; } } ////// Matrix that was used for rawstylusinput packets. /// internal GeneralTransform ElementTransform { get { return _tabletToElementTransform; } } ////// Retrieves the RawStylusInputCustomDataList associated with this input. /// internal RawStylusInputCustomDataList CustomDataList { get { if (_customData == null) { _customData = new RawStylusInputCustomDataList(); } return _customData; } } ////// StylusPlugIn that is adding a notify event. /// internal StylusPlugIn CurrentNotifyPlugIn { get { return _currentNotifyPlugIn; } set { _currentNotifyPlugIn = value; } } ///////////////////////////////////////////////////////////////////// RawStylusInputReport _report; GeneralTransform _tabletToElementTransform; StylusPlugInCollection _targetPlugInCollection; StylusPointCollection _stylusPoints; StylusPlugIn _currentNotifyPlugIn; RawStylusInputCustomDataList _customData; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Windows.Media; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input.StylusPlugIns { ///////////////////////////////////////////////////////////////////////// ////// [TBS] /// public class RawStylusInput { ///////////////////////////////////////////////////////////////////// ////// [TBS] /// /// [TBS] /// [TBS] /// [TBS] internal RawStylusInput( RawStylusInputReport report, GeneralTransform tabletToElementTransform, StylusPlugInCollection targetPlugInCollection) { if (report == null) { throw new ArgumentNullException("report"); } if (tabletToElementTransform.Inverse == null) { throw new ArgumentException(SR.Get(SRID.Stylus_MatrixNotInvertable), "tabletToElementTransform"); } if (targetPlugInCollection == null) { throw new ArgumentNullException("targetPlugInCollection"); } // We should always see this GeneralTransform is frozen since we access this from multiple threads. System.Diagnostics.Debug.Assert(tabletToElementTransform.IsFrozen); _report = report; _tabletToElementTransform = tabletToElementTransform; _targetPlugInCollection = targetPlugInCollection; } ////// /// public int StylusDeviceId { get { return _report.StylusDeviceId; } } ////// /// public int TabletDeviceId { get { return _report.TabletDeviceId; } } ////// /// public int Timestamp { get { return _report.Timestamp; } } ////// Returns a copy of the StylusPoints /// public StylusPointCollection GetStylusPoints() { return GetStylusPoints(Transform.Identity); } ////// Internal method called by StylusDevice to prevent two copies /// ////// Critical: This code accesses InputReport.InputSource /// TreatAsSafe: The method only gets a transform from PresentationSource and returns StylusPointCollection. /// Those operations are considered safe. /// This method is called by: /// RawStylusInput.GetStylusPoints /// StylusDevice.UpdateEventStylusPoints(RawStylusInputReport, Boolean) /// [SecurityCritical, SecurityTreatAsSafe] internal StylusPointCollection GetStylusPoints(GeneralTransform transform) { if (_stylusPoints == null) { // GeneralTransformGroup group = new GeneralTransformGroup(); if ( StylusDeviceId == 0) { // Only do this for the Mouse group.Children.Add(new MatrixTransform(_report.InputSource.CompositionTarget.TransformFromDevice)); } group.Children.Add(_tabletToElementTransform); group.Children.Add(transform); return new StylusPointCollection(_report.StylusPointDescription, _report.GetRawPacketData(), group, Matrix.Identity); } else { return _stylusPoints.Clone(transform, _stylusPoints.Description); } } ////// Replaces the StylusPoints. /// ////// Callers must have Unmanaged code permission to call this API. /// /// stylusPoints ////// Callers must have Unmanaged code permission to call this API. /// public void SetStylusPoints(StylusPointCollection stylusPoints) { // To modify the points we require Unmanaged code permission. SecurityHelper.DemandUnmanagedCode(); if (null == stylusPoints) { throw new ArgumentNullException("stylusPoints"); } if (!StylusPointDescription.AreCompatible( stylusPoints.Description, _report.StylusPointDescription)) { throw new ArgumentException(SR.Get(SRID.IncompatibleStylusPointDescriptions), "stylusPoints"); } if (stylusPoints.Count == 0) { throw new ArgumentException(SR.Get(SRID.Stylus_StylusPointsCantBeEmpty), "stylusPoints"); } _stylusPoints = stylusPoints.Clone(); } ////// Returns the RawStylusInputCustomDataList used to notify plugins before /// PreviewStylus event has been processed by application. /// public void NotifyWhenProcessed(object callbackData) { if (_currentNotifyPlugIn == null) { throw new InvalidOperationException(SR.Get(SRID.Stylus_CanOnlyCallForDownMoveOrUp)); } if (_customData == null) { _customData = new RawStylusInputCustomDataList(); } _customData.Add(new RawStylusInputCustomData(_currentNotifyPlugIn, callbackData)); } ////// True if a StylusPlugIn has modifiedthe StylusPoints. /// internal bool StylusPointsModified { get { return _stylusPoints != null; } } ////// Target StylusPlugInCollection that real time pen input sent to. /// internal StylusPlugInCollection Target { get { return _targetPlugInCollection; } } ////// Real RawStylusInputReport that this report is generated from. /// internal RawStylusInputReport Report { get { return _report; } } ////// Matrix that was used for rawstylusinput packets. /// internal GeneralTransform ElementTransform { get { return _tabletToElementTransform; } } ////// Retrieves the RawStylusInputCustomDataList associated with this input. /// internal RawStylusInputCustomDataList CustomDataList { get { if (_customData == null) { _customData = new RawStylusInputCustomDataList(); } return _customData; } } ////// StylusPlugIn that is adding a notify event. /// internal StylusPlugIn CurrentNotifyPlugIn { get { return _currentNotifyPlugIn; } set { _currentNotifyPlugIn = value; } } ///////////////////////////////////////////////////////////////////// RawStylusInputReport _report; GeneralTransform _tabletToElementTransform; StylusPlugInCollection _targetPlugInCollection; StylusPointCollection _stylusPoints; StylusPlugIn _currentNotifyPlugIn; RawStylusInputCustomDataList _customData; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
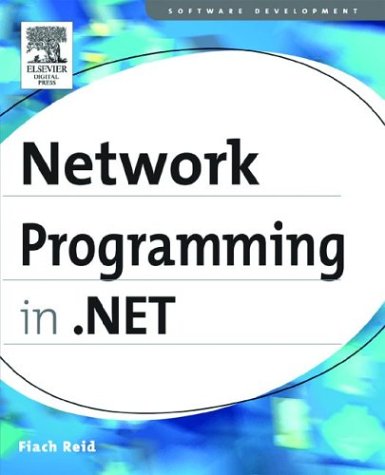
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapEffectDrawingContextState.cs
- OpenFileDialog.cs
- Triplet.cs
- CompoundFileStorageReference.cs
- PointKeyFrameCollection.cs
- SizeLimitedCache.cs
- MultiTrigger.cs
- StreamInfo.cs
- SQLGuid.cs
- BaseParser.cs
- WebPartDisplayMode.cs
- OleDbError.cs
- ConstNode.cs
- BindingsCollection.cs
- util.cs
- ElementMarkupObject.cs
- TimeSpanOrInfiniteValidator.cs
- BaseDataBoundControl.cs
- ProxyHwnd.cs
- XmlEncoding.cs
- DataBinding.cs
- ReaderWriterLock.cs
- selecteditemcollection.cs
- TextSpan.cs
- ServiceModelSectionGroup.cs
- MenuItemBindingCollection.cs
- WindowsNonControl.cs
- DictionaryTraceRecord.cs
- ListParagraph.cs
- WebHttpSecurityElement.cs
- ToolTipAutomationPeer.cs
- ModuleBuilderData.cs
- WebPartTransformer.cs
- CorruptingExceptionCommon.cs
- QueryReaderSettings.cs
- OrderPreservingMergeHelper.cs
- DesignerWebPartChrome.cs
- AddInProcess.cs
- BrowserTree.cs
- Set.cs
- HttpFileCollection.cs
- TextBox.cs
- HttpHandlersInstallComponent.cs
- Panel.cs
- Duration.cs
- LightweightEntityWrapper.cs
- SocketInformation.cs
- TextEditorParagraphs.cs
- CodeMemberEvent.cs
- Vector3dCollection.cs
- HttpBrowserCapabilitiesWrapper.cs
- EventWaitHandleSecurity.cs
- GridViewEditEventArgs.cs
- DataGridViewCellStyleConverter.cs
- XmlArrayItemAttributes.cs
- AttachmentCollection.cs
- Collection.cs
- OleDbException.cs
- PhonemeConverter.cs
- CatalogUtil.cs
- XmlDataSource.cs
- XmlLangPropertyAttribute.cs
- ReadOnlyDictionary.cs
- SqlConnectionPoolProviderInfo.cs
- Invariant.cs
- SplitterCancelEvent.cs
- FindCriteriaElement.cs
- DrawingAttributes.cs
- HandlerMappingMemo.cs
- SecurityAttributeGenerationHelper.cs
- SystemColorTracker.cs
- HtmlWindowCollection.cs
- FunctionImportMapping.cs
- ShaderRenderModeValidation.cs
- DayRenderEvent.cs
- _SslState.cs
- Model3DCollection.cs
- CodeRegionDirective.cs
- ConfigurationStrings.cs
- ListItem.cs
- FixedPageStructure.cs
- ToolStripGrip.cs
- DBCommandBuilder.cs
- CryptoHandle.cs
- FormsIdentity.cs
- Table.cs
- Site.cs
- ControlCachePolicy.cs
- BamlRecordHelper.cs
- TimeSpanOrInfiniteConverter.cs
- BitVector32.cs
- PagesSection.cs
- CacheChildrenQuery.cs
- IResourceProvider.cs
- ObjectAssociationEndMapping.cs
- WebDescriptionAttribute.cs
- WorkflowDefinitionContext.cs
- ContextStack.cs
- TreeNodeStyleCollectionEditor.cs
- X509CertificateCollection.cs