Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / MS / Internal / IO / Packaging / CompoundFile / CompoundFileStorageReference.cs / 1 / CompoundFileStorageReference.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of the CompoundFileStorageReference class. // // History: // 03/31/2003: BruceMac: Created. // 05/01/2003: BruceMac: Split off from CompoundFileReference class. // 05/20/2003: RogerCh: Ported to WCP tree. // 08/11/2003: LGolding: Fix Bug 864168 (some of BruceMac's bug fixes were lost // in port to WCP tree). // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security.Permissions; using System.Text; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging.CompoundFile { ////// Logical reference to a container storage /// ////// Use this class to represent a logical reference to a container storage, /// internal class CompoundFileStorageReference : CompoundFileReference, IComparable { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Full path from the container root to this storage /// public override string FullName { get { return _fullName; } } //------------------------------------------------------ // // public methods // //----------------------------------------------------- ////// Make a new Storage Reference /// /// whack-delimited name ///pass null or String.Empty to create a reference to the root storage public CompoundFileStorageReference(string fullName) { SetFullName(fullName); } #region Operators ///Compare for equality /// the CompoundFileReference to compare to public override bool Equals(object o) { if (o == null) return false; // Standard behavior. // support subclassing - our subclasses can call us and do any additive work themselves if (o.GetType() != GetType()) return false; // Note that because of the GetType() checking above, the casting must be valid. CompoundFileStorageReference r = (CompoundFileStorageReference)o; return (String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()) == 0); } ///Returns an integer suitable for including this object in a hash table public override int GetHashCode() { return _fullName.GetHashCode(); } #endregion #region IComparable ////// Compares two CompoundFileReferences /// /// CompoundFileReference to compare to this one ///Supports the IComparable interface ///less than zero if this instance is less than the given reference, zero if they are equal /// and greater than zero if this instance is greater than the given reference int IComparable.CompareTo(object o) { if (o == null) return 1; // Standard behavior. // different type? if (o.GetType() != GetType()) throw new ArgumentException( SR.Get(SRID.CanNotCompareDiffTypes)); // Note that because of the GetType() checking above, the casting must be valid. CompoundFileStorageReference r = (CompoundFileStorageReference)o; return String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ ////// Assign the fullName /// /// name ///cache a duplicate copy of the storage name to save having to do this for /// every call to get_Name ///if leading or trailing path delimiter private void SetFullName(string fullName) { if (fullName == null || fullName.Length == 0) { _fullName = String.Empty; } else { // fail on leading path separator to match functionality across the board // Although we need to do ToUpperInvariant before we do string comparison, in this case // it is not necessary since PathSeparatorAsString is a path symbol if (fullName.StartsWith(ContainerUtilities.PathSeparatorAsString, StringComparison.Ordinal)) throw new ArgumentException( SR.Get(SRID.DelimiterLeading), "fullName"); _fullName = fullName; // ensure that the string is a legal whack-path string[] strings = ContainerUtilities.ConvertBackSlashPathToStringArrayPath(_fullName); if (strings.Length == 0) throw new ArgumentException ( SR.Get(SRID.CompoundFilePathNullEmpty), "fullName"); } } //----------------------------------------------------- // // Private members // //------------------------------------------------------ // this can never be null - use String.Empty private String _fullName; // whack-path } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of the CompoundFileStorageReference class. // // History: // 03/31/2003: BruceMac: Created. // 05/01/2003: BruceMac: Split off from CompoundFileReference class. // 05/20/2003: RogerCh: Ported to WCP tree. // 08/11/2003: LGolding: Fix Bug 864168 (some of BruceMac's bug fixes were lost // in port to WCP tree). // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security.Permissions; using System.Text; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging.CompoundFile { ////// Logical reference to a container storage /// ////// Use this class to represent a logical reference to a container storage, /// internal class CompoundFileStorageReference : CompoundFileReference, IComparable { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Full path from the container root to this storage /// public override string FullName { get { return _fullName; } } //------------------------------------------------------ // // public methods // //----------------------------------------------------- ////// Make a new Storage Reference /// /// whack-delimited name ///pass null or String.Empty to create a reference to the root storage public CompoundFileStorageReference(string fullName) { SetFullName(fullName); } #region Operators ///Compare for equality /// the CompoundFileReference to compare to public override bool Equals(object o) { if (o == null) return false; // Standard behavior. // support subclassing - our subclasses can call us and do any additive work themselves if (o.GetType() != GetType()) return false; // Note that because of the GetType() checking above, the casting must be valid. CompoundFileStorageReference r = (CompoundFileStorageReference)o; return (String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()) == 0); } ///Returns an integer suitable for including this object in a hash table public override int GetHashCode() { return _fullName.GetHashCode(); } #endregion #region IComparable ////// Compares two CompoundFileReferences /// /// CompoundFileReference to compare to this one ///Supports the IComparable interface ///less than zero if this instance is less than the given reference, zero if they are equal /// and greater than zero if this instance is greater than the given reference int IComparable.CompareTo(object o) { if (o == null) return 1; // Standard behavior. // different type? if (o.GetType() != GetType()) throw new ArgumentException( SR.Get(SRID.CanNotCompareDiffTypes)); // Note that because of the GetType() checking above, the casting must be valid. CompoundFileStorageReference r = (CompoundFileStorageReference)o; return String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ ////// Assign the fullName /// /// name ///cache a duplicate copy of the storage name to save having to do this for /// every call to get_Name ///if leading or trailing path delimiter private void SetFullName(string fullName) { if (fullName == null || fullName.Length == 0) { _fullName = String.Empty; } else { // fail on leading path separator to match functionality across the board // Although we need to do ToUpperInvariant before we do string comparison, in this case // it is not necessary since PathSeparatorAsString is a path symbol if (fullName.StartsWith(ContainerUtilities.PathSeparatorAsString, StringComparison.Ordinal)) throw new ArgumentException( SR.Get(SRID.DelimiterLeading), "fullName"); _fullName = fullName; // ensure that the string is a legal whack-path string[] strings = ContainerUtilities.ConvertBackSlashPathToStringArrayPath(_fullName); if (strings.Length == 0) throw new ArgumentException ( SR.Get(SRID.CompoundFilePathNullEmpty), "fullName"); } } //----------------------------------------------------- // // Private members // //------------------------------------------------------ // this can never be null - use String.Empty private String _fullName; // whack-path } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
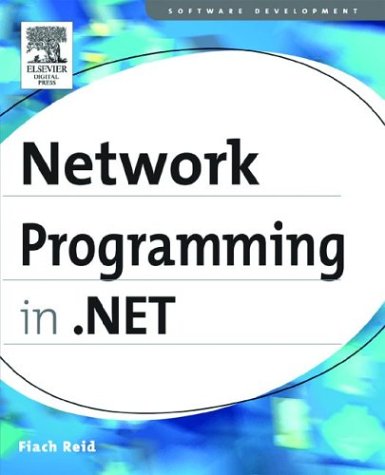
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataSourceEnumerator.cs
- SplitterPanel.cs
- Themes.cs
- WindowsUserNameCachingSecurityTokenAuthenticator.cs
- SafeCoTaskMem.cs
- DeclaredTypeElementCollection.cs
- CodeLinePragma.cs
- ContentType.cs
- ActivitySurrogateSelector.cs
- BufferedWebEventProvider.cs
- LockedAssemblyCache.cs
- WebScriptMetadataMessageEncodingBindingElement.cs
- PropertyReferenceSerializer.cs
- StrokeNodeOperations2.cs
- TagPrefixCollection.cs
- MaskInputRejectedEventArgs.cs
- CompiledQuery.cs
- DesignerOptionService.cs
- CharacterBuffer.cs
- WindowShowOrOpenTracker.cs
- SqlAliasesReferenced.cs
- ErrorRuntimeConfig.cs
- WebControlsSection.cs
- VariableModifiersHelper.cs
- BinarySerializer.cs
- JoinElimination.cs
- WeakEventManager.cs
- MDIClient.cs
- XamlTypeMapper.cs
- FragmentQueryProcessor.cs
- ToolboxItemCollection.cs
- Serializer.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- TraceHwndHost.cs
- CdpEqualityComparer.cs
- PropertyTab.cs
- MetaForeignKeyColumn.cs
- ConfigXmlText.cs
- SpellCheck.cs
- FixedSOMLineRanges.cs
- EpmSourcePathSegment.cs
- DbSource.cs
- SmtpMail.cs
- XmlWriter.cs
- XmlCodeExporter.cs
- TemplateBindingExtensionConverter.cs
- grammarelement.cs
- Dump.cs
- DataTemplateKey.cs
- KeysConverter.cs
- XmlKeywords.cs
- EditorPartDesigner.cs
- TextParentUndoUnit.cs
- FontInfo.cs
- StructuredType.cs
- KeyEvent.cs
- HttpResponse.cs
- WsdlContractConversionContext.cs
- SkinBuilder.cs
- ScaleTransform3D.cs
- SynchronizedDispatch.cs
- DES.cs
- SolidColorBrush.cs
- AssociationProvider.cs
- HeaderedItemsControl.cs
- FloatUtil.cs
- NativeActivityTransactionContext.cs
- DataGridViewCellCollection.cs
- ItemPager.cs
- TypefaceMetricsCache.cs
- FixedPageProcessor.cs
- UdpDiscoveryEndpointElement.cs
- MarginsConverter.cs
- GetCryptoTransformRequest.cs
- DataStorage.cs
- Int32Collection.cs
- LinqDataSourceEditData.cs
- LogReservationCollection.cs
- ConfigXmlText.cs
- OleDbFactory.cs
- BooleanAnimationBase.cs
- GridLengthConverter.cs
- DictionaryBase.cs
- CellTreeNode.cs
- CodeIdentifiers.cs
- PseudoWebRequest.cs
- FamilyCollection.cs
- MainMenu.cs
- SystemColors.cs
- HtmlInputImage.cs
- ClientBuildManagerCallback.cs
- SoapProtocolReflector.cs
- Schema.cs
- EncodingStreamWrapper.cs
- RoleGroup.cs
- CodePropertyReferenceExpression.cs
- PersonalizableAttribute.cs
- WindowsUpDown.cs
- EncodingNLS.cs
- ConfigurationLocation.cs