Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / TextLine.cs / 1 / TextLine.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextLine.cs // // Contents: Text line API // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 2-25-2003 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using MS.Internal.TextFormatting; using MS.Internal.PresentationCore; namespace System.Windows.Media.TextFormatting { ////// Once a line is complete, FormatLine returns a TextLine object to the client. /// The text layout client may then use the TextLines measurement and drawing methods. /// public abstract class TextLine : ITextMetrics, IDisposable { ////// Clean up text line internal resource /// public abstract void Dispose(); ////// Client to draw the line /// /// drawing context /// drawing origin /// indicate the inversion of the drawing surface public abstract void Draw( DrawingContext drawingContext, Point origin, InvertAxes inversion ); ////// Client to collapse the line and get a collapsed line that fits for display /// /// a list of collapsing properties public abstract TextLine Collapse( params TextCollapsingProperties[] collapsingPropertiesList ); ////// Client to get a collection of collapsed characer ranges after a line has been collapsed /// public abstract IListGetTextCollapsedRanges(); /// /// Client to get the character hit corresponding to the specified /// distance from the beginning of the line. /// /// distance in text flow direction from the beginning of the line ///character hit public abstract CharacterHit GetCharacterHitFromDistance( double distance ); ////// Client to get the distance from the beginning of the line from the specified /// character hit. /// /// character hit of the character to query the distance. ///distance in text flow direction from the beginning of the line. public abstract double GetDistanceFromCharacterHit( CharacterHit characterHit ); ////// Client to get the next character hit for caret navigation /// /// the current character hit ///the next character hit public abstract CharacterHit GetNextCaretCharacterHit( CharacterHit characterHit ); ////// Client to get the previous character hit for caret navigation /// /// the current character hit ///the previous character hit public abstract CharacterHit GetPreviousCaretCharacterHit( CharacterHit characterHit ); ////// Client to get the previous character hit after backspacing /// /// the current character hit ///the character hit after backspacing public abstract CharacterHit GetBackspaceCaretCharacterHit( CharacterHit characterHit ); ////// Determine whether the input character hit is a valid caret stop. /// /// the current character hit /// the starting cp index of the line ////// It is used by Framework to iterate through each codepoint in the line to /// see if the code point is a caret stop. In general, the leading edge of /// codepoint is a valid caret stop if moving forward and then backward will /// return back to it, vice versa for the trailing edge of a codepoint. /// [FriendAccessAllowed] internal bool IsAtCaretCharacterHit(CharacterHit characterHit, int cpFirst) { // TrailingLength is used as a flag to indicate whether the character // hit is on the leading or trailing edge of the character. if (characterHit.TrailingLength == 0) { CharacterHit nextHit = GetNextCaretCharacterHit(characterHit); if (nextHit == characterHit) { // At this point we only know that no caret stop is available // after the input, we caliberate the input to the end of the line. nextHit = new CharacterHit(cpFirst + Length - 1, 1); } CharacterHit previousHit = GetPreviousCaretCharacterHit(nextHit); return previousHit == characterHit; } else { CharacterHit previousHit = GetPreviousCaretCharacterHit(characterHit); CharacterHit nextHit = GetNextCaretCharacterHit(previousHit); return nextHit == characterHit; } } ////// Client to get an array of bounding rectangles of a range of characters within a text line. /// /// index of first character of specified range /// number of characters of the specified range ///an array of bounding rectangles. public abstract IListGetTextBounds( int firstTextSourceCharacterIndex, int textLength ); /// /// Client to get a collection of TextRun span objects within a line /// public abstract IList> GetTextRunSpans(); /// /// Client to get IndexedGlyphRuns enumerable to enumerate each IndexedGlyphRun object /// in the line. Through IndexedGlyphRun client can obtain glyph information of /// a text source character. /// public abstract IEnumerableGetIndexedGlyphRuns(); /// /// Client to get a boolean value indicates whether content of the line overflows /// the specified paragraph width. /// public abstract bool HasOverflowed { get; } ////// Client to get a boolean value indicates whether a line has been collapsed /// public abstract bool HasCollapsed { get; } ////// Client to get a Boolean flag indicating whether the line is truncated in the /// middle of a word. This flag is set only when TextParagraphProperties.TextWrapping /// is set to TextWrapping.Wrap and a single word is longer than the formatting /// paragraph width. In such situation, TextFormatter truncates the line in the middle /// of the word to honor the desired behavior specified by TextWrapping.Wrap setting. /// public virtual bool IsTruncated { get { return false; } } ////// Client to acquire a state at the point where line is broken by line breaking process; /// can be null when the line ends by the ending of the paragraph. Client may pass this /// value back to TextFormatter as an input argument to TextFormatter.FormatLine when /// formatting the next line within the same paragraph. /// public abstract TextLineBreak GetTextLineBreak(); #region ITextMetrics ////// Client to get the number of text source positions of this line /// public abstract int Length { get; } ////// Client to get the number of whitespace characters at the end of the line. /// public abstract int TrailingWhitespaceLength { get; } ////// Client to get the number of characters following the last character /// of the line that may trigger reformatting of the current line. /// public abstract int DependentLength { get; } ////// Client to get the number of newline characters at line end /// public abstract int NewlineLength { get; } ////// Client to get distance from paragraph start to line start /// public abstract double Start { get; } ////// Client to get the total width of this line /// public abstract double Width { get; } ////// Client to get the total width of this line including width of whitespace characters at the end of the line. /// public abstract double WidthIncludingTrailingWhitespace { get; } ////// Client to get the height of the line /// public abstract double Height { get; } ////// Client to get the height of the text (or other content) in the line; this property may differ from the Height /// property if the client specified the line height /// public abstract double TextHeight { get; } ////// Client to get the height of the actual black of the line /// public abstract double Extent { get; } ////// Client to get the distance from top to baseline of this text line /// public abstract double Baseline { get; } ////// Client to get the distance from the top of the text (or other content) to the baseline of this text line; /// this property may differ from the Baseline property if the client specified the line height /// public abstract double TextBaseline { get; } ////// Client to get the distance from the before edge of line height /// to the baseline of marker of the line if any. /// public abstract double MarkerBaseline { get; } ////// Client to get the overall height of the list items marker of the line if any. /// public abstract double MarkerHeight { get; } ////// Client to get the distance covering all black preceding the leading edge of the line. /// public abstract double OverhangLeading { get; } ////// Client to get the distance covering all black following the trailing edge of the line. /// public abstract double OverhangTrailing { get; } ////// Client to get the distance from the after edge of line height to the after edge of the extent of the line. /// public abstract double OverhangAfter { get; } #endregion } ////// Indicate the inversion of axes of the drawing surface /// [Flags] public enum InvertAxes { ////// Drawing surface is not inverted in either axis /// None = 0, ////// Drawing surface is inverted in horizontal axis /// Horizontal = 1, ////// Drawing surface is inverted in vertical axis /// Vertical = 2, ////// Drawing surface is inverted in both axes /// Both = (Horizontal | Vertical), } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
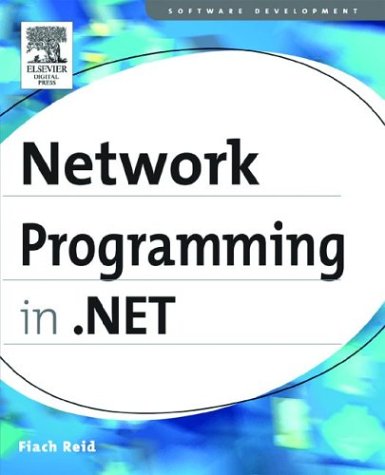
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutputCacheProfileCollection.cs
- LinkTarget.cs
- EnumValidator.cs
- ServiceDeploymentInfo.cs
- EditorPart.cs
- XomlCompiler.cs
- PropertyCollection.cs
- DTCTransactionManager.cs
- MarkupExtensionParser.cs
- HttpCacheParams.cs
- LineUtil.cs
- HtmlTernaryTree.cs
- PropertyEmitterBase.cs
- MenuBase.cs
- StringTraceRecord.cs
- CodeThrowExceptionStatement.cs
- WebPartManager.cs
- ISCIIEncoding.cs
- PlainXmlWriter.cs
- LocalizedNameDescriptionPair.cs
- WindowsToolbar.cs
- Vector.cs
- MethodBuilder.cs
- Win32.cs
- CaseInsensitiveComparer.cs
- Permission.cs
- XPathItem.cs
- KeyValueSerializer.cs
- ReliabilityContractAttribute.cs
- LineMetrics.cs
- WebPartConnectionsCancelEventArgs.cs
- ImplicitInputBrush.cs
- MenuItemBindingCollection.cs
- PanelDesigner.cs
- NameObjectCollectionBase.cs
- WebPartCollection.cs
- AdornerHitTestResult.cs
- DependencyPropertyDescriptor.cs
- HttpRuntime.cs
- PeerNameResolver.cs
- Attribute.cs
- NotifyIcon.cs
- InOutArgumentConverter.cs
- BreakSafeBase.cs
- DropDownList.cs
- HandlerMappingMemo.cs
- NameSpaceExtractor.cs
- URLMembershipCondition.cs
- DynamicValidatorEventArgs.cs
- BinHexEncoder.cs
- DataGridViewAdvancedBorderStyle.cs
- PagedDataSource.cs
- OdbcReferenceCollection.cs
- UpDownEvent.cs
- TableCellCollection.cs
- OptimizedTemplateContentHelper.cs
- Trace.cs
- DispatcherOperation.cs
- FilteredAttributeCollection.cs
- CharacterBuffer.cs
- ColorMap.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- DataBindingExpressionBuilder.cs
- ButtonAutomationPeer.cs
- SiteMapNodeCollection.cs
- AssemblyResourceLoader.cs
- XmlSerializationReader.cs
- XmlSchemaSimpleTypeList.cs
- DesignerSerializationOptionsAttribute.cs
- StorageMappingItemCollection.cs
- ProjectionCamera.cs
- __ConsoleStream.cs
- InternalBase.cs
- RawContentTypeMapper.cs
- StorageEntityContainerMapping.cs
- ImageListStreamer.cs
- XmlSchemaSequence.cs
- WebUtil.cs
- RegexGroupCollection.cs
- LogicalExpr.cs
- SelectionItemProviderWrapper.cs
- AuthorizationContext.cs
- GridItemPattern.cs
- SyndicationDeserializer.cs
- LinqDataSource.cs
- XmlStreamStore.cs
- Int32.cs
- PaintEvent.cs
- HttpCookieCollection.cs
- TableProviderWrapper.cs
- DbProviderFactory.cs
- WebPartDescriptionCollection.cs
- Clock.cs
- PKCS1MaskGenerationMethod.cs
- TableRow.cs
- PerfService.cs
- SqlLiftIndependentRowExpressions.cs
- XmlSerializerFaultFormatter.cs
- StringSource.cs
- TypeSystem.cs