Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Automation / TableProviderWrapper.cs / 1 / TableProviderWrapper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TableProviderWrapper: MarshalByRefObject, ITableProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableProviderWrapper( AutomationPeer peer, ITableProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface ITableProvider // //----------------------------------------------------- #region Interface ITableProvider public IRawElementProviderSimple GetItem(int row, int column) { return (IRawElementProviderSimple) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetItem ), new int [ ] { row, column } ); } public int RowCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowCount ), null ); } } public int ColumnCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnCount ), null ); } } public IRawElementProviderSimple [] GetRowHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowHeaders ), null ); } public IRawElementProviderSimple [] GetColumnHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnHeaders ), null ); } public RowOrColumnMajor RowOrColumnMajor { get { return (RowOrColumnMajor) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowOrColumnMajor ), null ); } } #endregion Interface ITableProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TableProviderWrapper( peer, (ITableProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object GetItem( object arg ) { int [ ] coords = (int [ ]) arg; return _iface.GetItem( coords[ 0 ], coords[ 1 ] ); } private object GetRowCount( object unused ) { return _iface.RowCount; } private object GetColumnCount( object unused ) { return _iface.ColumnCount; } private object GetRowHeaders( object unused ) { return _iface.GetRowHeaders(); } private object GetColumnHeaders( object unused ) { return _iface.GetColumnHeaders(); } private object GetRowOrColumnMajor( object unused ) { return _iface.RowOrColumnMajor; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITableProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TableProviderWrapper: MarshalByRefObject, ITableProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableProviderWrapper( AutomationPeer peer, ITableProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface ITableProvider // //----------------------------------------------------- #region Interface ITableProvider public IRawElementProviderSimple GetItem(int row, int column) { return (IRawElementProviderSimple) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetItem ), new int [ ] { row, column } ); } public int RowCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowCount ), null ); } } public int ColumnCount { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnCount ), null ); } } public IRawElementProviderSimple [] GetRowHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowHeaders ), null ); } public IRawElementProviderSimple [] GetColumnHeaders() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnHeaders ), null ); } public RowOrColumnMajor RowOrColumnMajor { get { return (RowOrColumnMajor) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowOrColumnMajor ), null ); } } #endregion Interface ITableProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TableProviderWrapper( peer, (ITableProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object GetItem( object arg ) { int [ ] coords = (int [ ]) arg; return _iface.GetItem( coords[ 0 ], coords[ 1 ] ); } private object GetRowCount( object unused ) { return _iface.RowCount; } private object GetColumnCount( object unused ) { return _iface.ColumnCount; } private object GetRowHeaders( object unused ) { return _iface.GetRowHeaders(); } private object GetColumnHeaders( object unused ) { return _iface.GetColumnHeaders(); } private object GetRowOrColumnMajor( object unused ) { return _iface.RowOrColumnMajor; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITableProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
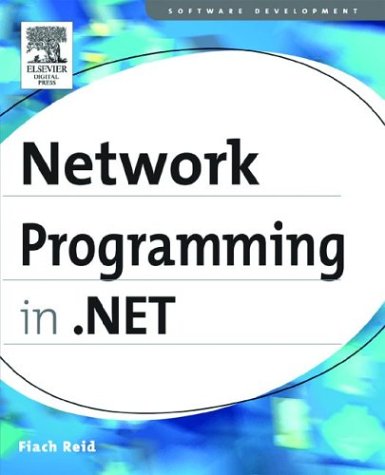
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectMemberMapping.cs
- LockedAssemblyCache.cs
- _AutoWebProxyScriptWrapper.cs
- InputMethodStateChangeEventArgs.cs
- ExpressionBuilderContext.cs
- EventLogInternal.cs
- RadioButtonPopupAdapter.cs
- RowParagraph.cs
- GroupQuery.cs
- EntityAdapter.cs
- XmlSchemaSubstitutionGroup.cs
- WeakRefEnumerator.cs
- Repeater.cs
- TextParaClient.cs
- SqlUtil.cs
- Parameter.cs
- InstanceView.cs
- DataControlCommands.cs
- FaultPropagationRecord.cs
- QueryIntervalOp.cs
- ObjectContextServiceProvider.cs
- Line.cs
- FullTextState.cs
- DataGridViewRowPostPaintEventArgs.cs
- HitTestDrawingContextWalker.cs
- Expressions.cs
- QueryPageSettingsEventArgs.cs
- PrintDialogException.cs
- ButtonChrome.cs
- RepeaterCommandEventArgs.cs
- CmsInterop.cs
- _UncName.cs
- EntityDesignPluralizationHandler.cs
- ProfilePropertySettings.cs
- StylusCaptureWithinProperty.cs
- EncodingTable.cs
- EntityViewGenerator.cs
- HttpServerUtilityWrapper.cs
- AddInServer.cs
- PixelShader.cs
- OdbcConnectionHandle.cs
- DispatcherBuilder.cs
- SoapEnumAttribute.cs
- TabPage.cs
- ExpressionEvaluator.cs
- WorkflowNamespace.cs
- Int16Converter.cs
- Underline.cs
- ReferentialConstraint.cs
- DetailsViewUpdateEventArgs.cs
- ZoomPercentageConverter.cs
- PasswordPropertyTextAttribute.cs
- Model3D.cs
- KeyValuePair.cs
- OpCodes.cs
- ExpressionQuoter.cs
- SecurityCriticalDataForSet.cs
- CreateUserWizard.cs
- MbpInfo.cs
- BuildProvider.cs
- StylusButtonCollection.cs
- ScrollEvent.cs
- ColorInterpolationModeValidation.cs
- ObjectListTitleAttribute.cs
- InvalidPrinterException.cs
- ICspAsymmetricAlgorithm.cs
- WebPartExportVerb.cs
- PanelDesigner.cs
- TextBlock.cs
- OperationContractGenerationContext.cs
- DefaultAsyncDataDispatcher.cs
- TextDecorationCollection.cs
- InvalidateEvent.cs
- ColumnWidthChangingEvent.cs
- EncodingTable.cs
- ContainsSearchOperator.cs
- sortedlist.cs
- SignalGate.cs
- EndpointDiscoveryMetadata.cs
- XsltFunctions.cs
- DataKey.cs
- ServiceReflector.cs
- EmptyQuery.cs
- MediaSystem.cs
- SetStoryboardSpeedRatio.cs
- BooleanFunctions.cs
- ManagementOperationWatcher.cs
- HostedTcpTransportManager.cs
- FixedBufferAttribute.cs
- ResourceContainer.cs
- WindowsListViewGroup.cs
- AtlasWeb.Designer.cs
- SafeBitVector32.cs
- ClearCollection.cs
- TemplateBindingExtension.cs
- Select.cs
- TypefaceMetricsCache.cs
- ListItemCollection.cs
- IUnknownConstantAttribute.cs
- FormsAuthenticationTicket.cs