Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / ProjectionCamera.cs / 1 / ProjectionCamera.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { abstract partial class ProjectionCamera : Camera { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new ProjectionCamera Clone() { return (ProjectionCamera)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new ProjectionCamera CloneCurrentValue() { return (ProjectionCamera)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void NearPlaneDistancePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(NearPlaneDistanceProperty); } private static void FarPlaneDistancePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(FarPlaneDistanceProperty); } private static void PositionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(PositionProperty); } private static void LookDirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(LookDirectionProperty); } private static void UpDirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(UpDirectionProperty); } #region Public Properties ////// NearPlaneDistance - double. Default value is (double)0.125. /// public double NearPlaneDistance { get { return (double) GetValue(NearPlaneDistanceProperty); } set { SetValueInternal(NearPlaneDistanceProperty, value); } } ////// FarPlaneDistance - double. Default value is (double)Double.PositiveInfinity. /// public double FarPlaneDistance { get { return (double) GetValue(FarPlaneDistanceProperty); } set { SetValueInternal(FarPlaneDistanceProperty, value); } } ////// Position - Point3D. Default value is new Point3D(). /// public Point3D Position { get { return (Point3D) GetValue(PositionProperty); } set { SetValueInternal(PositionProperty, value); } } ////// LookDirection - Vector3D. Default value is new Vector3D(0,0,-1). /// public Vector3D LookDirection { get { return (Vector3D) GetValue(LookDirectionProperty); } set { SetValueInternal(LookDirectionProperty, value); } } ////// UpDirection - Vector3D. Default value is new Vector3D(0,1,0). /// public Vector3D UpDirection { get { return (Vector3D) GetValue(UpDirectionProperty); } set { SetValueInternal(UpDirectionProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the ProjectionCamera.NearPlaneDistance property. /// public static readonly DependencyProperty NearPlaneDistanceProperty; ////// The DependencyProperty for the ProjectionCamera.FarPlaneDistance property. /// public static readonly DependencyProperty FarPlaneDistanceProperty; ////// The DependencyProperty for the ProjectionCamera.Position property. /// public static readonly DependencyProperty PositionProperty; ////// The DependencyProperty for the ProjectionCamera.LookDirection property. /// public static readonly DependencyProperty LookDirectionProperty; ////// The DependencyProperty for the ProjectionCamera.UpDirection property. /// public static readonly DependencyProperty UpDirectionProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_NearPlaneDistance = (double)0.125; internal const double c_FarPlaneDistance = (double)Double.PositiveInfinity; internal static Point3D s_Position = new Point3D(); internal static Vector3D s_LookDirection = new Vector3D(0,0,-1); internal static Vector3D s_UpDirection = new Vector3D(0,1,0); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static ProjectionCamera() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(ProjectionCamera); NearPlaneDistanceProperty = RegisterProperty("NearPlaneDistance", typeof(double), typeofThis, (double)0.125, new PropertyChangedCallback(NearPlaneDistancePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); FarPlaneDistanceProperty = RegisterProperty("FarPlaneDistance", typeof(double), typeofThis, (double)Double.PositiveInfinity, new PropertyChangedCallback(FarPlaneDistancePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); PositionProperty = RegisterProperty("Position", typeof(Point3D), typeofThis, new Point3D(), new PropertyChangedCallback(PositionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); LookDirectionProperty = RegisterProperty("LookDirection", typeof(Vector3D), typeofThis, new Vector3D(0,0,-1), new PropertyChangedCallback(LookDirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); UpDirectionProperty = RegisterProperty("UpDirection", typeof(Vector3D), typeofThis, new Vector3D(0,1,0), new PropertyChangedCallback(UpDirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { abstract partial class ProjectionCamera : Camera { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new ProjectionCamera Clone() { return (ProjectionCamera)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new ProjectionCamera CloneCurrentValue() { return (ProjectionCamera)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void NearPlaneDistancePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(NearPlaneDistanceProperty); } private static void FarPlaneDistancePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(FarPlaneDistanceProperty); } private static void PositionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(PositionProperty); } private static void LookDirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(LookDirectionProperty); } private static void UpDirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProjectionCamera target = ((ProjectionCamera) d); target.PropertyChanged(UpDirectionProperty); } #region Public Properties ////// NearPlaneDistance - double. Default value is (double)0.125. /// public double NearPlaneDistance { get { return (double) GetValue(NearPlaneDistanceProperty); } set { SetValueInternal(NearPlaneDistanceProperty, value); } } ////// FarPlaneDistance - double. Default value is (double)Double.PositiveInfinity. /// public double FarPlaneDistance { get { return (double) GetValue(FarPlaneDistanceProperty); } set { SetValueInternal(FarPlaneDistanceProperty, value); } } ////// Position - Point3D. Default value is new Point3D(). /// public Point3D Position { get { return (Point3D) GetValue(PositionProperty); } set { SetValueInternal(PositionProperty, value); } } ////// LookDirection - Vector3D. Default value is new Vector3D(0,0,-1). /// public Vector3D LookDirection { get { return (Vector3D) GetValue(LookDirectionProperty); } set { SetValueInternal(LookDirectionProperty, value); } } ////// UpDirection - Vector3D. Default value is new Vector3D(0,1,0). /// public Vector3D UpDirection { get { return (Vector3D) GetValue(UpDirectionProperty); } set { SetValueInternal(UpDirectionProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the ProjectionCamera.NearPlaneDistance property. /// public static readonly DependencyProperty NearPlaneDistanceProperty; ////// The DependencyProperty for the ProjectionCamera.FarPlaneDistance property. /// public static readonly DependencyProperty FarPlaneDistanceProperty; ////// The DependencyProperty for the ProjectionCamera.Position property. /// public static readonly DependencyProperty PositionProperty; ////// The DependencyProperty for the ProjectionCamera.LookDirection property. /// public static readonly DependencyProperty LookDirectionProperty; ////// The DependencyProperty for the ProjectionCamera.UpDirection property. /// public static readonly DependencyProperty UpDirectionProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_NearPlaneDistance = (double)0.125; internal const double c_FarPlaneDistance = (double)Double.PositiveInfinity; internal static Point3D s_Position = new Point3D(); internal static Vector3D s_LookDirection = new Vector3D(0,0,-1); internal static Vector3D s_UpDirection = new Vector3D(0,1,0); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static ProjectionCamera() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(ProjectionCamera); NearPlaneDistanceProperty = RegisterProperty("NearPlaneDistance", typeof(double), typeofThis, (double)0.125, new PropertyChangedCallback(NearPlaneDistancePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); FarPlaneDistanceProperty = RegisterProperty("FarPlaneDistance", typeof(double), typeofThis, (double)Double.PositiveInfinity, new PropertyChangedCallback(FarPlaneDistancePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); PositionProperty = RegisterProperty("Position", typeof(Point3D), typeofThis, new Point3D(), new PropertyChangedCallback(PositionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); LookDirectionProperty = RegisterProperty("LookDirection", typeof(Vector3D), typeofThis, new Vector3D(0,0,-1), new PropertyChangedCallback(LookDirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); UpDirectionProperty = RegisterProperty("UpDirection", typeof(Vector3D), typeofThis, new Vector3D(0,1,0), new PropertyChangedCallback(UpDirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
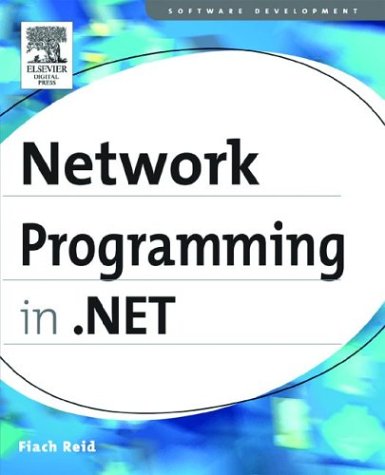
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChannelDemuxer.cs
- WsatAdminException.cs
- XmlSchemaDatatype.cs
- DbMetaDataColumnNames.cs
- Frame.cs
- DelegateBodyWriter.cs
- DataGridViewTextBoxEditingControl.cs
- SystemResources.cs
- AutoGeneratedField.cs
- MatrixAnimationUsingPath.cs
- HandleCollector.cs
- ExtensibleClassFactory.cs
- TextHidden.cs
- _PooledStream.cs
- DialogDivider.cs
- DynamicValidator.cs
- DocumentEventArgs.cs
- WebPartConnectionsEventArgs.cs
- IntSecurity.cs
- SqlRecordBuffer.cs
- WebPartCloseVerb.cs
- ComponentGlyph.cs
- SocketInformation.cs
- ProbeMatchesCD1.cs
- SubMenuStyle.cs
- StateChangeEvent.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- EventItfInfo.cs
- SQLChars.cs
- DelegateBodyWriter.cs
- Int64Animation.cs
- ObjectDataSourceDesigner.cs
- RealizationContext.cs
- SettingsPropertyWrongTypeException.cs
- TargetException.cs
- SqlDataSourceConfigureFilterForm.cs
- EventsTab.cs
- PropertyPathWorker.cs
- JumpItem.cs
- LogicalExpressionEditor.cs
- NamespaceMapping.cs
- URLIdentityPermission.cs
- SystemWebSectionGroup.cs
- MiniConstructorInfo.cs
- Cursor.cs
- CharUnicodeInfo.cs
- PolyBezierSegmentFigureLogic.cs
- SqlTypesSchemaImporter.cs
- TableCellCollection.cs
- IDispatchConstantAttribute.cs
- ProjectionAnalyzer.cs
- Activity.cs
- GradientPanel.cs
- CopyNamespacesAction.cs
- ContentValidator.cs
- SettingsPropertyCollection.cs
- VarRemapper.cs
- PolicyException.cs
- SecurityPolicySection.cs
- RSAProtectedConfigurationProvider.cs
- EdmRelationshipRoleAttribute.cs
- TypeUtils.cs
- CLRBindingWorker.cs
- ListViewCancelEventArgs.cs
- XmlDataSource.cs
- ChannelPool.cs
- ColumnResult.cs
- UnicodeEncoding.cs
- ScopedMessagePartSpecification.cs
- OdbcDataAdapter.cs
- FontDialog.cs
- DesignObjectWrapper.cs
- LabelEditEvent.cs
- ShaderEffect.cs
- DynamicPropertyHolder.cs
- MessageQuerySet.cs
- Command.cs
- SystemInfo.cs
- ThemeableAttribute.cs
- CodeDirectoryCompiler.cs
- Simplifier.cs
- InternalResources.cs
- GAC.cs
- FieldTemplateFactory.cs
- WebServiceHost.cs
- Rule.cs
- SubclassTypeValidatorAttribute.cs
- TemplateComponentConnector.cs
- PersianCalendar.cs
- DataGridViewToolTip.cs
- ExtenderProviderService.cs
- DataSourceHelper.cs
- ProjectionPathSegment.cs
- TransactionsSectionGroup.cs
- ResourceManagerWrapper.cs
- TextOnlyOutput.cs
- InvalidEnumArgumentException.cs
- WarningException.cs
- _TimerThread.cs
- hresults.cs