Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Security / RightsManagement / LocalizedNameDescriptionPair.cs / 1 / LocalizedNameDescriptionPair.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This class represents an immutable pair of Strings (Name, Description) // That are generally used to represent name and description of an unsigned publish license // (a.k.a. template). Unsigned Publish License has property called LocalizedNameDescriptionDictionary // which holds a map of a local Id to a Name Description pair, in order to support scenarios of // building locale specific template browsing applications. // // History: // 11/14/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Windows; using MS.Internal.Security.RightsManagement; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; // Allow use of presharp warning numbers [6506] and [6518] unknown to the compiler #pragma warning disable 1634, 1691 namespace System.Security.RightsManagement { ////// LocalizedNameDescriptionPair class represent an immutable (Name, Description) pair of strings. This is /// a basic building block for structures that need to express locale specific information about /// Unsigned Publish Licenses. /// ////// Critical: This class exposes access to methods that eventually do one or more of the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attrbiute automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unamanged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class LocalizedNameDescriptionPair { ////// Constructor for the read only LocalizedNameDescriptionPair class. It takes values for Name and Description as parameters. /// public LocalizedNameDescriptionPair(string name, string description) { SecurityHelper.DemandRightsManagementPermission(); if (name == null) { throw new ArgumentNullException("name"); } if (description == null) { throw new ArgumentNullException("description"); } _name = name; _description = description; } ////// Read only Name property. /// public string Name { get { SecurityHelper.DemandRightsManagementPermission(); return _name; } } ////// Read only Description property. /// public string Description { get { SecurityHelper.DemandRightsManagementPermission(); return _description; } } ////// Test for equality. /// public override bool Equals(object obj) { SecurityHelper.DemandRightsManagementPermission(); if ((obj == null) || (obj.GetType() != GetType())) { return false; } LocalizedNameDescriptionPair localizedNameDescr = obj as LocalizedNameDescriptionPair; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 return (String.CompareOrdinal(localizedNameDescr.Name, Name) == 0) && (String.CompareOrdinal(localizedNameDescr.Description, Description) == 0); #pragma warning restore 6506 } ////// Compute hash code. /// public override int GetHashCode() { SecurityHelper.DemandRightsManagementPermission(); return Name.GetHashCode() ^ Description.GetHashCode(); } private string _name; private string _description; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This class represents an immutable pair of Strings (Name, Description) // That are generally used to represent name and description of an unsigned publish license // (a.k.a. template). Unsigned Publish License has property called LocalizedNameDescriptionDictionary // which holds a map of a local Id to a Name Description pair, in order to support scenarios of // building locale specific template browsing applications. // // History: // 11/14/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Windows; using MS.Internal.Security.RightsManagement; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; // Allow use of presharp warning numbers [6506] and [6518] unknown to the compiler #pragma warning disable 1634, 1691 namespace System.Security.RightsManagement { ////// LocalizedNameDescriptionPair class represent an immutable (Name, Description) pair of strings. This is /// a basic building block for structures that need to express locale specific information about /// Unsigned Publish Licenses. /// ////// Critical: This class exposes access to methods that eventually do one or more of the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attrbiute automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unamanged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class LocalizedNameDescriptionPair { ////// Constructor for the read only LocalizedNameDescriptionPair class. It takes values for Name and Description as parameters. /// public LocalizedNameDescriptionPair(string name, string description) { SecurityHelper.DemandRightsManagementPermission(); if (name == null) { throw new ArgumentNullException("name"); } if (description == null) { throw new ArgumentNullException("description"); } _name = name; _description = description; } ////// Read only Name property. /// public string Name { get { SecurityHelper.DemandRightsManagementPermission(); return _name; } } ////// Read only Description property. /// public string Description { get { SecurityHelper.DemandRightsManagementPermission(); return _description; } } ////// Test for equality. /// public override bool Equals(object obj) { SecurityHelper.DemandRightsManagementPermission(); if ((obj == null) || (obj.GetType() != GetType())) { return false; } LocalizedNameDescriptionPair localizedNameDescr = obj as LocalizedNameDescriptionPair; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 return (String.CompareOrdinal(localizedNameDescr.Name, Name) == 0) && (String.CompareOrdinal(localizedNameDescr.Description, Description) == 0); #pragma warning restore 6506 } ////// Compute hash code. /// public override int GetHashCode() { SecurityHelper.DemandRightsManagementPermission(); return Name.GetHashCode() ^ Description.GetHashCode(); } private string _name; private string _description; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
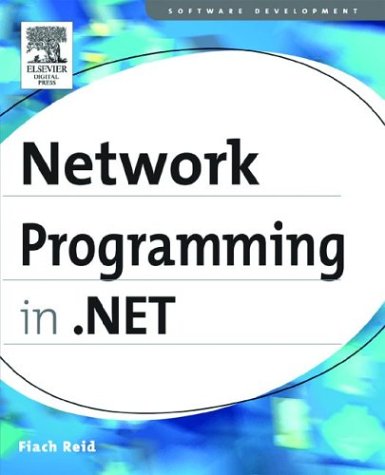
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeLocalMemHandle.cs
- XmlSchemaRedefine.cs
- XamlStream.cs
- NativeActivityMetadata.cs
- AsyncOperation.cs
- CollectionViewGroupRoot.cs
- TagNameToTypeMapper.cs
- StringResourceManager.cs
- BasicExpandProvider.cs
- TraceData.cs
- MaterialGroup.cs
- WhitespaceRuleLookup.cs
- AccessDataSource.cs
- GridLength.cs
- SpecialTypeDataContract.cs
- ComponentCommands.cs
- StreamHelper.cs
- CollectionView.cs
- SiteMapDataSource.cs
- WeakReferenceKey.cs
- LeftCellWrapper.cs
- cookie.cs
- loginstatus.cs
- SharedDp.cs
- ServicePointManager.cs
- LicenseProviderAttribute.cs
- PermissionSetTriple.cs
- Propagator.JoinPropagator.cs
- CompilerHelpers.cs
- BitmapScalingModeValidation.cs
- XPathEmptyIterator.cs
- TypedServiceChannelBuilder.cs
- ImageInfo.cs
- RowToFieldTransformer.cs
- RectangleF.cs
- MatrixKeyFrameCollection.cs
- EditorPartDesigner.cs
- Vector3DAnimation.cs
- ConstructorBuilder.cs
- SqlComparer.cs
- FrameworkTextComposition.cs
- RbTree.cs
- AssertFilter.cs
- GacUtil.cs
- WebPartMinimizeVerb.cs
- PixelShader.cs
- PointAnimationClockResource.cs
- ProcessHost.cs
- TextOutput.cs
- TextWriter.cs
- PresentationTraceSources.cs
- ProjectionPlan.cs
- WebScriptMetadataMessage.cs
- SecuritySessionSecurityTokenProvider.cs
- StandardTransformFactory.cs
- SqlParameter.cs
- XmlAnyAttributeAttribute.cs
- WebPartUtil.cs
- HierarchicalDataSourceControl.cs
- TitleStyle.cs
- ObjectKeyFrameCollection.cs
- SoapFault.cs
- DefaultValueAttribute.cs
- XmlEventCache.cs
- IDispatchConstantAttribute.cs
- RightNameExpirationInfoPair.cs
- AttachmentService.cs
- CodeArrayCreateExpression.cs
- XmlSchemas.cs
- Utils.cs
- WebPartConnectionsEventArgs.cs
- IIS7WorkerRequest.cs
- BitmapMetadataEnumerator.cs
- KeyPressEvent.cs
- EnumBuilder.cs
- TextSearch.cs
- TaiwanLunisolarCalendar.cs
- FileStream.cs
- DiffuseMaterial.cs
- SafeProcessHandle.cs
- FreeFormDesigner.cs
- DateTimeConverter.cs
- TimeSpanSecondsConverter.cs
- HighContrastHelper.cs
- ResourceDescriptionAttribute.cs
- AspNetSynchronizationContext.cs
- ChannelOptions.cs
- GridViewColumnHeaderAutomationPeer.cs
- ISFTagAndGuidCache.cs
- MultiSelector.cs
- ObjectDataProvider.cs
- ButtonBaseDesigner.cs
- CacheMemory.cs
- HandleRef.cs
- ExpressionBuilder.cs
- SimpleMailWebEventProvider.cs
- TextElementAutomationPeer.cs
- ErrorStyle.cs
- TrackingProfileSerializer.cs
- ResourceReferenceKeyNotFoundException.cs