Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / CodeDOM / CodeTypeDeclaration.cs / 1305376 / CodeTypeDeclaration.cs
//------------------------------------------------------------------------------ //// // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using Microsoft.Win32; using System.Collections; using System.Reflection; using System.Runtime.Serialization; using System.Runtime.InteropServices; ///[....] // Copyright (c) Microsoft Corporation. All rights reserved. ///// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeTypeDeclaration : CodeTypeMember { private TypeAttributes attributes = Reflection.TypeAttributes.Public | Reflection.TypeAttributes.Class; private CodeTypeReferenceCollection baseTypes = new CodeTypeReferenceCollection(); private CodeTypeMemberCollection members = new CodeTypeMemberCollection(); private bool isEnum; private bool isStruct; private int populated = 0x0; private const int BaseTypesCollection = 0x1; private const int MembersCollection = 0x2; // Need to be made optionally serializable [OptionalField] private CodeTypeParameterCollection typeParameters; [OptionalField] private bool isPartial = false; ////// Represents a /// class or nested class. /// ////// public event EventHandler PopulateBaseTypes; ////// An event that will be fired the first time the BaseTypes Collection is accessed. /// ////// public event EventHandler PopulateMembers; ////// An event that will be fired the first time the Members Collection is accessed. /// ////// public CodeTypeDeclaration() { } ////// Initializes a new instance of ///. /// /// public CodeTypeDeclaration(string name) { Name = name; } ////// Initializes a new instance of ///with the specified name. /// /// public TypeAttributes TypeAttributes { get { return attributes; } set { attributes = value; } } ////// Gets or sets the attributes of the class. /// ////// public CodeTypeReferenceCollection BaseTypes { get { if (0 == (populated & BaseTypesCollection)) { populated |= BaseTypesCollection; if (PopulateBaseTypes != null) PopulateBaseTypes(this, EventArgs.Empty); } return baseTypes; } } ////// Gets or sets /// the base types of the class. /// ////// public bool IsClass { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Class && !isEnum && !isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Class; isStruct = false; isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is a class. /// ////// public bool IsStruct { get { return isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = true; isEnum = false; } else { isStruct = false; } } } ////// Gets or sets a value /// indicating whether the class is a struct. /// ////// public bool IsEnum { get { return isEnum; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = false; isEnum = true; } else { isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is an enumeration. /// ////// public bool IsInterface { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Interface; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Interface; isStruct = false; isEnum = false; } else { attributes &= ~TypeAttributes.Interface; } } } public bool IsPartial { get { return isPartial; } set { isPartial = value; } } ////// Gets or sets a value /// indicating whether the class is an interface. /// ////// public CodeTypeMemberCollection Members { get { if (0 == (populated & MembersCollection)) { populated |= MembersCollection; if (PopulateMembers != null) PopulateMembers(this, EventArgs.Empty); } return members; } } [System.Runtime.InteropServices.ComVisible(false)] public CodeTypeParameterCollection TypeParameters { get { if( typeParameters == null) { typeParameters = new CodeTypeParameterCollection(); } return typeParameters; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Gets or sets the class member collection members. /// ///// // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using Microsoft.Win32; using System.Collections; using System.Reflection; using System.Runtime.Serialization; using System.Runtime.InteropServices; ///[....] // Copyright (c) Microsoft Corporation. All rights reserved. ///// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeTypeDeclaration : CodeTypeMember { private TypeAttributes attributes = Reflection.TypeAttributes.Public | Reflection.TypeAttributes.Class; private CodeTypeReferenceCollection baseTypes = new CodeTypeReferenceCollection(); private CodeTypeMemberCollection members = new CodeTypeMemberCollection(); private bool isEnum; private bool isStruct; private int populated = 0x0; private const int BaseTypesCollection = 0x1; private const int MembersCollection = 0x2; // Need to be made optionally serializable [OptionalField] private CodeTypeParameterCollection typeParameters; [OptionalField] private bool isPartial = false; ////// Represents a /// class or nested class. /// ////// public event EventHandler PopulateBaseTypes; ////// An event that will be fired the first time the BaseTypes Collection is accessed. /// ////// public event EventHandler PopulateMembers; ////// An event that will be fired the first time the Members Collection is accessed. /// ////// public CodeTypeDeclaration() { } ////// Initializes a new instance of ///. /// /// public CodeTypeDeclaration(string name) { Name = name; } ////// Initializes a new instance of ///with the specified name. /// /// public TypeAttributes TypeAttributes { get { return attributes; } set { attributes = value; } } ////// Gets or sets the attributes of the class. /// ////// public CodeTypeReferenceCollection BaseTypes { get { if (0 == (populated & BaseTypesCollection)) { populated |= BaseTypesCollection; if (PopulateBaseTypes != null) PopulateBaseTypes(this, EventArgs.Empty); } return baseTypes; } } ////// Gets or sets /// the base types of the class. /// ////// public bool IsClass { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Class && !isEnum && !isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Class; isStruct = false; isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is a class. /// ////// public bool IsStruct { get { return isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = true; isEnum = false; } else { isStruct = false; } } } ////// Gets or sets a value /// indicating whether the class is a struct. /// ////// public bool IsEnum { get { return isEnum; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = false; isEnum = true; } else { isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is an enumeration. /// ////// public bool IsInterface { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Interface; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Interface; isStruct = false; isEnum = false; } else { attributes &= ~TypeAttributes.Interface; } } } public bool IsPartial { get { return isPartial; } set { isPartial = value; } } ////// Gets or sets a value /// indicating whether the class is an interface. /// ////// public CodeTypeMemberCollection Members { get { if (0 == (populated & MembersCollection)) { populated |= MembersCollection; if (PopulateMembers != null) PopulateMembers(this, EventArgs.Empty); } return members; } } [System.Runtime.InteropServices.ComVisible(false)] public CodeTypeParameterCollection TypeParameters { get { if( typeParameters == null) { typeParameters = new CodeTypeParameterCollection(); } return typeParameters; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Gets or sets the class member collection members. /// ///
Link Menu
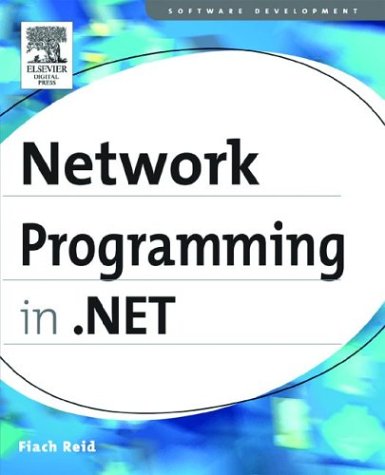
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ElementNotEnabledException.cs
- RawStylusInputCustomData.cs
- COM2TypeInfoProcessor.cs
- ObjectListCommandsPage.cs
- ResourcesBuildProvider.cs
- NonVisualControlAttribute.cs
- ResourceBinder.cs
- XmlCDATASection.cs
- PreviewKeyDownEventArgs.cs
- HttpRuntimeSection.cs
- DirectoryObjectSecurity.cs
- DataSourceListEditor.cs
- ADMembershipUser.cs
- AlignmentYValidation.cs
- ToolStripGripRenderEventArgs.cs
- BuildManager.cs
- SiteIdentityPermission.cs
- SystemFonts.cs
- RemotingException.cs
- SqlRowUpdatedEvent.cs
- SemaphoreSecurity.cs
- TemplateControl.cs
- DynamicQueryableWrapper.cs
- PresentationAppDomainManager.cs
- DbCommandDefinition.cs
- CommonProperties.cs
- ObjectComplexPropertyMapping.cs
- SqlVisitor.cs
- ContentPlaceHolderDesigner.cs
- ListSortDescriptionCollection.cs
- WeakReferenceEnumerator.cs
- GenericPrincipal.cs
- XmlNodeComparer.cs
- X509SecurityTokenAuthenticator.cs
- SqlTypeConverter.cs
- PerfCounters.cs
- TextEditorThreadLocalStore.cs
- KnownAssembliesSet.cs
- CellParagraph.cs
- WindowsFont.cs
- KeyFrames.cs
- MissingSatelliteAssemblyException.cs
- BitmapInitialize.cs
- RequestResizeEvent.cs
- Range.cs
- IDataContractSurrogate.cs
- UserInitiatedNavigationPermission.cs
- WindowsRegion.cs
- MobileListItem.cs
- UnmanagedMemoryStream.cs
- LinqDataView.cs
- AccessKeyManager.cs
- ExpressionBuilder.cs
- DetailsViewCommandEventArgs.cs
- StructuredType.cs
- WebPartExportVerb.cs
- FormViewAutoFormat.cs
- SignatureDescription.cs
- DefaultWorkflowLoaderService.cs
- WebBrowserSiteBase.cs
- ASCIIEncoding.cs
- TypeLoadException.cs
- XmlSchemaAnnotated.cs
- BitmapFrameDecode.cs
- HtmlElementEventArgs.cs
- RelationalExpressions.cs
- Empty.cs
- ConfigurationStrings.cs
- ActiveXContainer.cs
- SqlDataSourceConfigureFilterForm.cs
- cookie.cs
- GZipStream.cs
- configsystem.cs
- storepermission.cs
- _AutoWebProxyScriptEngine.cs
- EventHandlerList.cs
- ExcludePathInfo.cs
- CodeDomDesignerLoader.cs
- ClientOptions.cs
- PhysicalFontFamily.cs
- DataIdProcessor.cs
- DictionaryManager.cs
- SchemaTableOptionalColumn.cs
- MatrixCamera.cs
- ChameleonKey.cs
- MatrixTransform3D.cs
- DateTimeFormat.cs
- IssuedSecurityTokenParameters.cs
- CompilerErrorCollection.cs
- CompletedAsyncResult.cs
- ComAdminInterfaces.cs
- DataGridViewColumn.cs
- WebControlsSection.cs
- XmlSchemaInfo.cs
- MatrixTransform3D.cs
- ClientUtils.cs
- ImageConverter.cs
- PixelFormat.cs
- BindingWorker.cs
- CqlIdentifiers.cs