Code:
/ DotNET / DotNET / 8.0 / untmp / Orcas / RTM / ndp / fx / src / xsp / System / Web / Extensions / ClientServices / Providers / ProxyHelper.cs / 1 / ProxyHelper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ClientServices.Providers { using System; using System.Security; using System.Security.Permissions; using System.Security.AccessControl; using System.Security.Principal; using System.Threading; using System.Runtime.InteropServices; using System.Collections; using System.Globalization; using System.Net; using System.Text; using System.Runtime.Serialization; using System.Collections.Specialized; using System.Collections.Generic; using System.Xml; using System.Collections.ObjectModel; using System.Web.Resources; using System.Web.Script.Serialization; using System.IO; using System.Diagnostics.CodeAnalysis; ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// internal static class ProxyHelper { ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// internal static object CreateWebRequestAndGetResponse(string serverUri, ref CookieContainer cookies, string username, string connectionString, string connectionStringProvider, string [] paramNames, object [] paramValues, Type returnType) { HttpWebRequest request = (HttpWebRequest) WebRequest.Create(serverUri); request.UseDefaultCredentials = true; request.ContentType = "application/json; charset=utf-8"; request.AllowAutoRedirect = true; request.Method = "POST"; if (cookies == null) cookies = ConstructCookieContainer(serverUri, username, connectionString, connectionStringProvider); if (cookies != null) request.CookieContainer = cookies; if (paramNames != null && paramNames.Length > 0) { byte [] postedBody = GetSerializedParameters(paramNames, paramValues); request.ContentLength = postedBody.Length; using(Stream s = request.GetRequestStream()) { s.Write(postedBody, 0, postedBody.Length); } } else { request.ContentLength = 0; } // Get the response try { using(HttpWebResponse response = (HttpWebResponse)request.GetResponse()) { if (response == null) throw new WebException(AtlasWeb.ClientService_BadJsonResponse); GetCookiesFromResponse(response, cookies, serverUri, username, connectionString, connectionStringProvider); if (returnType == null) return null; JavaScriptSerializer js = JavaScriptSerializer.CreateInstance(new SimpleTypeResolver()); string responseJson = GetResponseString(response); DictionarywrapperObject = js.DeserializeObject(responseJson) as Dictionary ; if (wrapperObject == null || !wrapperObject.ContainsKey("d")) { throw new WebException(AtlasWeb.ClientService_BadJsonResponse); } return ObjectConverter.ConvertObjectToType(wrapperObject["d"], returnType, js); } } catch(WebException we) { HttpWebResponse response = (HttpWebResponse) we.Response; if (response == null) throw; throw new WebException(String.Format(CultureInfo.CurrentCulture, AtlasWeb.ProxyHelper_BadStatusCode, response.StatusCode.ToString(), GetResponseString(response)), we); } } ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// private static void GetCookiesFromResponse(HttpWebResponse response, CookieContainer cookies, string serverUri, string username, string connectionString, string connectionStringProvider) { foreach (Cookie c in response.Cookies) cookies.Add(c); int numHeaders = response.Headers.Count; for(int iter=0; iter len) len = (int) s.Length; char[] read = new char[len]; StringBuilder sb = new StringBuilder(len); int count = readStream.Read(read, 0, len); while (count > 0) { sb.Append(new string(read, 0, count)); count = readStream.Read(read, 0, len); } return sb.ToString(); } } } ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// #if ENABLE_WCF_SUPPORT [SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods", Justification="Reviewed and approved by feature crew")] internal static CustomBinding GetBinding() { HttpTransportBindingElement be = new HttpTransportBindingElement(); be.AllowCookies = false; if (Thread.CurrentPrincipal != null && Thread.CurrentPrincipal.Identity is WindowsIdentity) be.AuthenticationScheme = AuthenticationSchemes.Negotiate; TextMessageEncodingBindingElement tmbe = new TextMessageEncodingBindingElement(MessageVersion.Soap11, Encoding.UTF8); CustomBinding binding = new CustomBinding(tmbe, be); return binding; } ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// [SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods", Justification="Reviewed and approved by feature crew")] internal static void GetCookiesFromWCF(CookieContainer authenticationCookies, string serverUri, string username, string connectionString, string connectionStringProvider) { if (username == null) { if (Thread.CurrentPrincipal != null) username = Thread.CurrentPrincipal.Identity.Name; else username = string.Empty; } HttpResponseMessageProperty httpResponseProperty = (HttpResponseMessageProperty)OperationContext.Current.IncomingMessageProperties[HttpResponseMessageProperty.Name]; if (httpResponseProperty == null || httpResponseProperty.Headers == null || httpResponseProperty.Headers.Count < 1) return; int count = httpResponseProperty.Headers.Count; Uri uri = ((authenticationCookies==null) ? null : new Uri(serverUri)); for(int iter=0; iter 0) return true; return false; } ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// private static void StoreCookie(string serverUri, string cookieHeaders, string username, string connectionString, string connectionStringProvider) { if (string.IsNullOrEmpty(cookieHeaders)) return; string[] cookieHeaderSplits = cookieHeaders.Split(new char[] { ',' }); for(int iter=0; iter 0 && (posSemi < 0 || posSemi > posEq)) break; cookieHeaderStrBuilder.Append(","); cookieHeaderStrBuilder.Append(cookieHeaderSplits[iter++]); } string cookieHeader = cookieHeaderStrBuilder.ToString(); // Split it into name=value //Console.WriteLine("Saving cookie header:: " + cookieHeader); int posEquals = cookieHeader.IndexOf('='); string cookieName = ((posEquals < 0) ? cookieHeader : cookieHeader.Substring(0, posEquals)).Trim(); string cookieValue = ((posEquals < 0) ? string.Empty : cookieHeader.Substring(posEquals + 1)).Trim(); // trim off the HttpOnly and store in our DB if (cookieValue.Length > 0) ChangeCookieAndStoreInDB(ref cookieName, ref cookieValue, username, connectionString, connectionStringProvider); if (UnsafeNativeMethods.InternetSetCookieW(serverUri, null, cookieName + " = " + cookieValue) == 0) { // Marshal.ThrowExceptionForHR(Marshal.GetHRForLastWin32Error()); } } } ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// private static string[] GetCookiesFromIECache(string uri, string username, string connectionString, string connectionStringProvider) { //////////////////////////////////////////////////////////// // Step 1: Get the cookie from IE int size = 0; if (UnsafeNativeMethods.InternetGetCookieW(uri, null, null, ref size) == 0 || size < 1) return null; // Failed to get cookie-size: likely, that no cookie is present StringBuilder cookieValue = new StringBuilder(size); if (UnsafeNativeMethods.InternetGetCookieW(uri, null, cookieValue, ref size) == 0) return null; // fail silently string [] cookies = cookieValue.ToString().Split(new char[] {';'}, StringSplitOptions.RemoveEmptyEntries); if (connectionString != null) { for (int iter = 0; iter < cookies.Length; iter++) { cookies[iter] = GetCookieFromDB(cookies[iter], username, connectionString, connectionStringProvider); } } return cookies; } ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// private static string GetCookieFromDB(string cookieHeader, string username, string connectionString, string connectionStringProvider) { cookieHeader = cookieHeader.Trim(); ///////////////////////////////////////////////////////////////////////////////// // Munged cookie is of the form "[32-digit-guid]=Q" // See if this cookie is of that form if (cookieHeader.Length != 34 || cookieHeader[33] != 'Q' || cookieHeader.IndexOf('=') != 32) return cookieHeader; // not of the correct form return SqlHelper.GetCookieFromDB(cookieHeader.Substring(0, 32), username, connectionString, connectionStringProvider); } ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////////// private static void ChangeCookieAndStoreInDB(ref string cookieName, ref string cookieValue, string username, string connectionString, string connectionStringProvider) { string [] cookieProps = cookieValue.Split(new char[] {';'}); if (cookieProps.Length < 1) return; string actualCookieValue = cookieProps[0]; bool foundHttpOnly = false; StringBuilder sb = new StringBuilder((connectionString==null) ? actualCookieValue : "Q", cookieValue.Length); // Deal with all the properties, e.g. "path=/; expires= NNNN" for(int iter=1; iter GetPropertiesForCurrentUser(string[] properties, bool authenticatedUserOnly); [System.ServiceModel.OperationContractAttribute(Action = "http://tempuri.org/ProfileService/SetPropertiesForCurrentUser", ReplyAction = "http://tempuri.org/ProfileService/SetPropertiesForCurrentUserResponse"), SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods", Justification="Reviewed and approved by feature crew")] //[NetDataContractFormat] Collection SetPropertiesForCurrentUser(System.Collections.Generic.IDictionary values, bool authenticatedUserOnly); [System.ServiceModel.OperationContractAttribute(Action="http://tempuri.org/ProfileService/GetPropertiesMetadata", ReplyAction="http://tempuri.org/ProfileService/GetPropertiesMetadataResponse"), SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods", Justification="Reviewed and approved by feature crew")] System.Web.ApplicationServices.ProfilePropertyMetadata[] GetPropertiesMetadata(); } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// [System.CodeDom.Compiler.GeneratedCodeAttribute("System.ServiceModel", "3.0.0.0")] internal interface ProfileServiceChannel : ProfileService, System.ServiceModel.IClientChannel { } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// [System.Diagnostics.DebuggerStepThroughAttribute()] [System.CodeDom.Compiler.GeneratedCodeAttribute("System.ServiceModel", "3.0.0.0")] internal partial class ProfileServiceClient : System.ServiceModel.ClientBase , ProfileService { public ProfileServiceClient() { } public ProfileServiceClient(string endpointConfigurationName) : base(endpointConfigurationName) { } public ProfileServiceClient(string endpointConfigurationName, string remoteAddress) : base(endpointConfigurationName, remoteAddress) { } public ProfileServiceClient(string endpointConfigurationName, System.ServiceModel.EndpointAddress remoteAddress) : base(endpointConfigurationName, remoteAddress) { } public ProfileServiceClient(System.ServiceModel.Channels.Binding binding, System.ServiceModel.EndpointAddress remoteAddress) : base(binding, remoteAddress) { } [SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods", Justification="Reviewed and approved by feature crew")] public System.Collections.Generic.Dictionary GetPropertiesForCurrentUser(string[] propertyNames, bool authenticatedUserOnly) { return base.Channel.GetPropertiesForCurrentUser(propertyNames, authenticatedUserOnly); } [SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods", Justification="Reviewed and approved by feature crew")] public Collection SetPropertiesForCurrentUser(System.Collections.Generic.IDictionary values, bool authenticatedUserOnly) { return base.Channel.SetPropertiesForCurrentUser(values, authenticatedUserOnly); } [SuppressMessage("Microsoft.Security", "CA2116:AptcaMethodsShouldOnlyCallAptcaMethods", Justification="Reviewed and approved by feature crew")] public System.Web.ApplicationServices.ProfilePropertyMetadata[] GetPropertiesMetadata() { return base.Channel.GetPropertiesMetadata(); } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
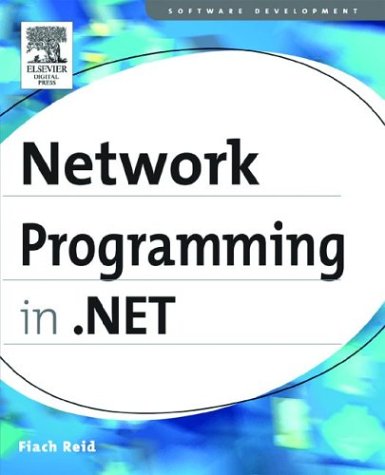
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SpeechUI.cs
- DesignerAdapterUtil.cs
- baseaxisquery.cs
- StructuralObject.cs
- TextFindEngine.cs
- LogReserveAndAppendState.cs
- ImageBrush.cs
- EventRouteFactory.cs
- ContactManager.cs
- TableMethodGenerator.cs
- FunctionQuery.cs
- VirtualDirectoryMapping.cs
- ListViewCancelEventArgs.cs
- SmuggledIUnknown.cs
- ReceiveCompletedEventArgs.cs
- MenuItem.cs
- PtsCache.cs
- BaseHashHelper.cs
- ListCollectionView.cs
- DispatcherTimer.cs
- BitmapEffectGeneralTransform.cs
- ConfigurationStrings.cs
- UnionCqlBlock.cs
- WorkflowDefinitionContext.cs
- DuplexSecurityProtocolFactory.cs
- DockEditor.cs
- EncryptedReference.cs
- SignerInfo.cs
- WebBrowserContainer.cs
- SimpleBitVector32.cs
- OleDbReferenceCollection.cs
- WebErrorHandler.cs
- GPPOINT.cs
- CheckBoxBaseAdapter.cs
- ToolboxItemAttribute.cs
- MaterializeFromAtom.cs
- ContextMenuStrip.cs
- DataBoundControlAdapter.cs
- HttpCookie.cs
- FileDialogPermission.cs
- ToolbarAUtomationPeer.cs
- BuildProviderUtils.cs
- JournalEntryStack.cs
- Canvas.cs
- AppSettingsExpressionBuilder.cs
- OneWayElement.cs
- ReflectPropertyDescriptor.cs
- CancellationTokenSource.cs
- GregorianCalendar.cs
- SqlStatistics.cs
- DataMember.cs
- ProjectionPlanCompiler.cs
- PackWebRequest.cs
- NavigationService.cs
- NumberSubstitution.cs
- EditorAttribute.cs
- ToolStripRenderer.cs
- FullTextLine.cs
- RectangleConverter.cs
- XmlILConstructAnalyzer.cs
- OpenTypeCommon.cs
- designeractionbehavior.cs
- XmlRootAttribute.cs
- DispatchWrapper.cs
- NullableConverter.cs
- SqlUserDefinedAggregateAttribute.cs
- ScriptingProfileServiceSection.cs
- XmlName.cs
- EmptyStringExpandableObjectConverter.cs
- XmlNodeChangedEventArgs.cs
- Misc.cs
- securitycriticaldata.cs
- PackagingUtilities.cs
- AppearanceEditorPart.cs
- MulticastOption.cs
- UniqueCodeIdentifierScope.cs
- ContentControl.cs
- RootBuilder.cs
- Style.cs
- TokenFactoryFactory.cs
- SqlUDTStorage.cs
- MemberPathMap.cs
- WebControlsSection.cs
- HwndKeyboardInputProvider.cs
- NamedPipeAppDomainProtocolHandler.cs
- DecodeHelper.cs
- OutputScopeManager.cs
- EncodingConverter.cs
- PropertyDescriptorGridEntry.cs
- WebPageTraceListener.cs
- HitTestParameters3D.cs
- StylusPoint.cs
- AttributeCollection.cs
- TrustManagerPromptUI.cs
- FileRecordSequenceHelper.cs
- Emitter.cs
- EnumCodeDomSerializer.cs
- ChildTable.cs
- COM2PropertyBuilderUITypeEditor.cs
- CodeDomLoader.cs