Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / TypeLoadException.cs / 1 / TypeLoadException.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: TypeLoadException ** ** ** Purpose: The exception class for type loading failures. ** ** =============================================================================*/ namespace System { using System; using System.Globalization; using System.Runtime.Remoting; using System.Runtime.Serialization; using System.Runtime.CompilerServices; using System.Security.Permissions; [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public class TypeLoadException : SystemException, ISerializable { public TypeLoadException() : base(Environment.GetResourceString("Arg_TypeLoadException")) { SetErrorCode(__HResults.COR_E_TYPELOAD); } public TypeLoadException(String message) : base(message) { SetErrorCode(__HResults.COR_E_TYPELOAD); } public TypeLoadException(String message, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_TYPELOAD); } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) { if ((ClassName == null) && (ResourceId == 0)) _message = Environment.GetResourceString("Arg_TypeLoadException"); else { if (AssemblyName == null) AssemblyName = Environment.GetResourceString("IO_UnknownFileName"); if (ClassName == null) ClassName = Environment.GetResourceString("IO_UnknownFileName"); _message = String.Format(CultureInfo.CurrentCulture, GetTypeLoadExceptionMessage(ResourceId), ClassName, AssemblyName, MessageArg); } } } public String TypeName { get { if (ClassName == null) return String.Empty; return ClassName; } } // This is called from inside the EE. private TypeLoadException(String className, String assemblyName, String messageArg, int resourceId) : base(null) { SetErrorCode(__HResults.COR_E_TYPELOAD); ClassName = className; AssemblyName = assemblyName; MessageArg = messageArg; ResourceId = resourceId; // Set the _message field eagerly; debuggers look at this field to // display error info. They don't call the Message property. SetMessageField(); } protected TypeLoadException(SerializationInfo info, StreamingContext context) : base(info, context) { if (info == null) throw new ArgumentNullException("info"); ClassName = info.GetString("TypeLoadClassName"); AssemblyName = info.GetString("TypeLoadAssemblyName"); MessageArg = info.GetString("TypeLoadMessageArg"); ResourceId = info.GetInt32("TypeLoadResourceID"); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetTypeLoadExceptionMessage(int resourceId); //We can rely on the serialization mechanism on Exception to handle most of our needs, but //we need to add a few fields of our own. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue("TypeLoadClassName", ClassName, typeof(String)); info.AddValue("TypeLoadAssemblyName", AssemblyName, typeof(String)); info.AddValue("TypeLoadMessageArg", MessageArg, typeof(String)); info.AddValue("TypeLoadResourceID", ResourceId); } // If ClassName != null, GetMessage will construct on the fly using it // and ResourceId (mscorrc.dll). This allows customization of the // class name format depending on the language environment. private String ClassName; private String AssemblyName; private String MessageArg; internal int ResourceId; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: TypeLoadException ** ** ** Purpose: The exception class for type loading failures. ** ** =============================================================================*/ namespace System { using System; using System.Globalization; using System.Runtime.Remoting; using System.Runtime.Serialization; using System.Runtime.CompilerServices; using System.Security.Permissions; [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public class TypeLoadException : SystemException, ISerializable { public TypeLoadException() : base(Environment.GetResourceString("Arg_TypeLoadException")) { SetErrorCode(__HResults.COR_E_TYPELOAD); } public TypeLoadException(String message) : base(message) { SetErrorCode(__HResults.COR_E_TYPELOAD); } public TypeLoadException(String message, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_TYPELOAD); } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) { if ((ClassName == null) && (ResourceId == 0)) _message = Environment.GetResourceString("Arg_TypeLoadException"); else { if (AssemblyName == null) AssemblyName = Environment.GetResourceString("IO_UnknownFileName"); if (ClassName == null) ClassName = Environment.GetResourceString("IO_UnknownFileName"); _message = String.Format(CultureInfo.CurrentCulture, GetTypeLoadExceptionMessage(ResourceId), ClassName, AssemblyName, MessageArg); } } } public String TypeName { get { if (ClassName == null) return String.Empty; return ClassName; } } // This is called from inside the EE. private TypeLoadException(String className, String assemblyName, String messageArg, int resourceId) : base(null) { SetErrorCode(__HResults.COR_E_TYPELOAD); ClassName = className; AssemblyName = assemblyName; MessageArg = messageArg; ResourceId = resourceId; // Set the _message field eagerly; debuggers look at this field to // display error info. They don't call the Message property. SetMessageField(); } protected TypeLoadException(SerializationInfo info, StreamingContext context) : base(info, context) { if (info == null) throw new ArgumentNullException("info"); ClassName = info.GetString("TypeLoadClassName"); AssemblyName = info.GetString("TypeLoadAssemblyName"); MessageArg = info.GetString("TypeLoadMessageArg"); ResourceId = info.GetInt32("TypeLoadResourceID"); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetTypeLoadExceptionMessage(int resourceId); //We can rely on the serialization mechanism on Exception to handle most of our needs, but //we need to add a few fields of our own. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue("TypeLoadClassName", ClassName, typeof(String)); info.AddValue("TypeLoadAssemblyName", AssemblyName, typeof(String)); info.AddValue("TypeLoadMessageArg", MessageArg, typeof(String)); info.AddValue("TypeLoadResourceID", ResourceId); } // If ClassName != null, GetMessage will construct on the fly using it // and ResourceId (mscorrc.dll). This allows customization of the // class name format depending on the language environment. private String ClassName; private String AssemblyName; private String MessageArg; internal int ResourceId; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
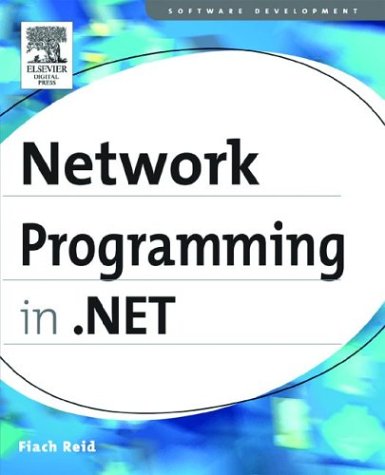
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientRuntimeConfig.cs
- ViewStateChangedEventArgs.cs
- StructuredTypeEmitter.cs
- Vector3dCollection.cs
- BaseTemplateCodeDomTreeGenerator.cs
- ZeroOpNode.cs
- TimeoutConverter.cs
- StaticExtension.cs
- Error.cs
- WebPartConnectionsCloseVerb.cs
- WebPartConnectionsCancelEventArgs.cs
- CodeMethodInvokeExpression.cs
- TextTreeRootTextBlock.cs
- XmlSchemaIdentityConstraint.cs
- MSAANativeProvider.cs
- PropertyGeneratedEventArgs.cs
- AnimatedTypeHelpers.cs
- StorageTypeMapping.cs
- EventListener.cs
- ListBoxItem.cs
- CheckBoxAutomationPeer.cs
- SafeLibraryHandle.cs
- EventHandlersStore.cs
- assertwrapper.cs
- DoubleKeyFrameCollection.cs
- XamlTreeBuilder.cs
- MailDefinition.cs
- DetailsViewDeletedEventArgs.cs
- DbProviderManifest.cs
- XmlNodeChangedEventArgs.cs
- Matrix.cs
- ObjectDataSourceView.cs
- TaskScheduler.cs
- RewritingPass.cs
- WindowsUpDown.cs
- EntityDataSourceChangedEventArgs.cs
- CompareInfo.cs
- WebPartZoneBase.cs
- DeferredReference.cs
- NavigationHelper.cs
- WebHttpSecurityModeHelper.cs
- MessageQueuePermission.cs
- sortedlist.cs
- _NestedSingleAsyncResult.cs
- IconConverter.cs
- Msmq4SubqueuePoisonHandler.cs
- MobileResource.cs
- StoreItemCollection.Loader.cs
- IconBitmapDecoder.cs
- IERequestCache.cs
- MatrixAnimationUsingPath.cs
- TargetException.cs
- _Rfc2616CacheValidators.cs
- HttpMethodConstraint.cs
- NavigationProperty.cs
- DictationGrammar.cs
- BindingContext.cs
- OutputCacheProfileCollection.cs
- TogglePattern.cs
- OTFRasterizer.cs
- DataGridItem.cs
- UpdatePanel.cs
- PenThreadWorker.cs
- LoadWorkflowByKeyAsyncResult.cs
- TypeBuilder.cs
- CheckableControlBaseAdapter.cs
- CodeDomConfigurationHandler.cs
- MediaScriptCommandRoutedEventArgs.cs
- SingleAnimationBase.cs
- SafeThreadHandle.cs
- FormatException.cs
- SqlDependencyListener.cs
- GuidelineCollection.cs
- NavigationProperty.cs
- SystemIPv6InterfaceProperties.cs
- ProfilePropertyMetadata.cs
- Timer.cs
- OpenTypeMethods.cs
- PageBuildProvider.cs
- DynamicValidatorEventArgs.cs
- KeyInfo.cs
- Identity.cs
- EditingMode.cs
- XmlMembersMapping.cs
- HttpCacheVaryByContentEncodings.cs
- SecondaryIndexDefinition.cs
- ActiveXHost.cs
- ManagedFilter.cs
- Debug.cs
- DateTimeOffset.cs
- DataColumn.cs
- CertificateReferenceElement.cs
- SubpageParaClient.cs
- CustomAssemblyResolver.cs
- DataBinder.cs
- CodeAccessPermission.cs
- PeerCollaborationPermission.cs
- CollectionViewSource.cs
- DateTimeAutomationPeer.cs
- DynamicRendererThreadManager.cs