Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / KeyGesture.cs / 1305600 / KeyGesture.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyGesture class is used by the developer to create Keyboard Input Bindings // // See spec at : http://avalon/coreUI/Specs/Commanding%20--%20design.htm // //* KeyGesture class serves the purpose of Input Bindings for Keyboard Device. //* one will be passing the instance of this around with CommandLink to represent Accelerator Keys // // History: // 06/01/2003 : chandras - Created // //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Windows.Input; using System.Windows; using System.Windows.Markup; using System.ComponentModel; using MS.Internal.PresentationCore; namespace System.Windows.Input { ////// KeyGesture - Key and Modifier combination. /// Can be set on properties of KeyBinding and RoutedCommand. /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public class KeyGesture : InputGesture { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// constructor /// /// key public KeyGesture(Key key) : this(key, ModifierKeys.None) { } ////// constructor /// /// modifiers /// key public KeyGesture(Key key, ModifierKeys modifiers) : this(key, modifiers, String.Empty, true) { } ////// constructor /// /// modifiers /// key /// display string public KeyGesture(Key key, ModifierKeys modifiers, string displayString) : this(key, modifiers, displayString, true) { } ////// Internal constructor used by KeyBinding to avoid key and modifier validation /// This allows setting KeyBinding.Key and KeyBinding.Modifiers without regard /// to order. /// /// Key /// Modifiers /// If true, throws an exception if the key and modifier are not valid internal KeyGesture(Key key, ModifierKeys modifiers, bool validateGesture) : this(key, modifiers, String.Empty, validateGesture) { } ////// Private constructor that does the real work. /// /// Key /// Modifiers /// display string /// If true, throws an exception if the key and modifier are not valid private KeyGesture(Key key, ModifierKeys modifiers, string displayString, bool validateGesture) { if(!ModifierKeysConverter.IsDefinedModifierKeys(modifiers)) throw new InvalidEnumArgumentException("modifiers", (int)modifiers, typeof(ModifierKeys)); if(!IsDefinedKey(key)) throw new InvalidEnumArgumentException("key", (int)key, typeof(Key)); if (displayString == null) throw new ArgumentNullException("displayString"); if(validateGesture && !IsValid(key, modifiers)) { throw new NotSupportedException(SR.Get(SRID.KeyGesture_Invalid, modifiers, key)); } _modifiers = modifiers; _key = key; _displayString = displayString; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Modifier /// public ModifierKeys Modifiers { get { return _modifiers; } } ////// Key /// public Key Key { get { return _key; } } ////// DisplayString /// public string DisplayString { get { return _displayString; } } ////// Returns a string that can be used to display the KeyGesture. If the /// DisplayString was set by the constructor, it is returned. Otherwise /// a suitable string is created from the Key and Modifiers, with any /// conversions being governed by the given CultureInfo. /// /// the culture used when creating a string from Key and Modifiers public string GetDisplayStringForCulture(CultureInfo culture) { // return the DisplayString, if it was set by the ctor if (!String.IsNullOrEmpty(_displayString)) { return _displayString; } // otherwise use the type converter return (string)_keyGestureConverter.ConvertTo(null, culture, this, typeof(string)); } ////// Compares InputEventArgs with current Input /// /// the element to receive the command /// inputEventArgs to compare to ///True - KeyGesture matches, false otherwise. /// public override bool Matches(object targetElement, InputEventArgs inputEventArgs) { KeyEventArgs keyEventArgs = inputEventArgs as KeyEventArgs; if(keyEventArgs != null && IsDefinedKey(keyEventArgs.Key)) { return ( ( (int)Key == (int)keyEventArgs.RealKey ) && ( this.Modifiers == Keyboard.Modifiers ) ); } return false; } // Check for Valid enum, as any int can be casted to the enum. internal static bool IsDefinedKey(Key key) { return (key >= Key.None && key <= Key.OemClear); } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Is Valid Keyboard input to process for commands /// internal static bool IsValid(Key key, ModifierKeys modifiers) { // // Don't enforce any rules on the Function keys or on the number pad keys. // if(!( ( key >= Key.F1 && key <= Key.F24 ) || ( key >= Key.NumPad0 && key <= Key.Divide ) )) { // // We check whether Control/Alt/Windows key is down for modifiers. We don't check // for shift at this time as Shift with any combination is already covered in above check. // Shift alone as modifier case, we defer to the next condition to avoid conflicing with // TextInput. if(( modifiers & ( ModifierKeys.Control | ModifierKeys.Alt | ModifierKeys.Windows ) ) != 0) { switch(key) { case Key.LeftCtrl: case Key.RightCtrl: case Key.LeftAlt: case Key.RightAlt: case Key.LWin: case Key.RWin: return false; default: return true; } } else if(( key >= Key.D0 && key <= Key.D9 ) || ( key >= Key.A && key <= Key.Z )) { return false; } } return true; } ////// Decode the strings keyGestures and displayStrings, creating a sequence /// of KeyGestures. Add each KeyGesture to the given InputGestureCollection. /// The two input strings typically come from a resource file. /// internal static void AddGesturesFromResourceStrings(string keyGestures, string displayStrings, InputGestureCollection gestures) { while (!String.IsNullOrEmpty(keyGestures)) { string keyGestureToken; string keyDisplayString; // break apart first gesture from the rest int index = keyGestures.IndexOf(MULTIPLEGESTURE_DELIMITER, StringComparison.Ordinal); if (index >= 0) { // multiple gestures exist keyGestureToken = keyGestures.Substring(0, index); keyGestures = keyGestures.Substring(index + 1); } else { keyGestureToken = keyGestures; keyGestures = String.Empty; } // similarly, break apart first display string from the rest index = displayStrings.IndexOf(MULTIPLEGESTURE_DELIMITER, StringComparison.Ordinal); if (index >= 0) { // multiple display strings exist keyDisplayString = displayStrings.Substring(0, index); displayStrings = displayStrings.Substring(index + 1); } else { keyDisplayString = displayStrings; displayStrings = String.Empty; } KeyGesture keyGesture = CreateFromResourceStrings(keyGestureToken, keyDisplayString); if (keyGesture != null) { gestures.Add(keyGesture); } } } internal static KeyGesture CreateFromResourceStrings(string keyGestureToken, string keyDisplayString) { // combine the gesture and the display string, producing a string // that the type converter will recognize if (!String.IsNullOrEmpty(keyDisplayString)) { keyGestureToken += KeyGestureConverter.DISPLAYSTRING_SEPARATOR + keyDisplayString; } return _keyGestureConverter.ConvertFromInvariantString(keyGestureToken) as KeyGesture; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private ModifierKeys _modifiers = ModifierKeys.None; private Key _key = Key.None; private string _displayString; private const string MULTIPLEGESTURE_DELIMITER = ";"; private static TypeConverter _keyGestureConverter = new KeyGestureConverter(); //private static bool _classRegistered = false; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyGesture class is used by the developer to create Keyboard Input Bindings // // See spec at : http://avalon/coreUI/Specs/Commanding%20--%20design.htm // //* KeyGesture class serves the purpose of Input Bindings for Keyboard Device. //* one will be passing the instance of this around with CommandLink to represent Accelerator Keys // // History: // 06/01/2003 : chandras - Created // //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Windows.Input; using System.Windows; using System.Windows.Markup; using System.ComponentModel; using MS.Internal.PresentationCore; namespace System.Windows.Input { ////// KeyGesture - Key and Modifier combination. /// Can be set on properties of KeyBinding and RoutedCommand. /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public class KeyGesture : InputGesture { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// constructor /// /// key public KeyGesture(Key key) : this(key, ModifierKeys.None) { } ////// constructor /// /// modifiers /// key public KeyGesture(Key key, ModifierKeys modifiers) : this(key, modifiers, String.Empty, true) { } ////// constructor /// /// modifiers /// key /// display string public KeyGesture(Key key, ModifierKeys modifiers, string displayString) : this(key, modifiers, displayString, true) { } ////// Internal constructor used by KeyBinding to avoid key and modifier validation /// This allows setting KeyBinding.Key and KeyBinding.Modifiers without regard /// to order. /// /// Key /// Modifiers /// If true, throws an exception if the key and modifier are not valid internal KeyGesture(Key key, ModifierKeys modifiers, bool validateGesture) : this(key, modifiers, String.Empty, validateGesture) { } ////// Private constructor that does the real work. /// /// Key /// Modifiers /// display string /// If true, throws an exception if the key and modifier are not valid private KeyGesture(Key key, ModifierKeys modifiers, string displayString, bool validateGesture) { if(!ModifierKeysConverter.IsDefinedModifierKeys(modifiers)) throw new InvalidEnumArgumentException("modifiers", (int)modifiers, typeof(ModifierKeys)); if(!IsDefinedKey(key)) throw new InvalidEnumArgumentException("key", (int)key, typeof(Key)); if (displayString == null) throw new ArgumentNullException("displayString"); if(validateGesture && !IsValid(key, modifiers)) { throw new NotSupportedException(SR.Get(SRID.KeyGesture_Invalid, modifiers, key)); } _modifiers = modifiers; _key = key; _displayString = displayString; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Modifier /// public ModifierKeys Modifiers { get { return _modifiers; } } ////// Key /// public Key Key { get { return _key; } } ////// DisplayString /// public string DisplayString { get { return _displayString; } } ////// Returns a string that can be used to display the KeyGesture. If the /// DisplayString was set by the constructor, it is returned. Otherwise /// a suitable string is created from the Key and Modifiers, with any /// conversions being governed by the given CultureInfo. /// /// the culture used when creating a string from Key and Modifiers public string GetDisplayStringForCulture(CultureInfo culture) { // return the DisplayString, if it was set by the ctor if (!String.IsNullOrEmpty(_displayString)) { return _displayString; } // otherwise use the type converter return (string)_keyGestureConverter.ConvertTo(null, culture, this, typeof(string)); } ////// Compares InputEventArgs with current Input /// /// the element to receive the command /// inputEventArgs to compare to ///True - KeyGesture matches, false otherwise. /// public override bool Matches(object targetElement, InputEventArgs inputEventArgs) { KeyEventArgs keyEventArgs = inputEventArgs as KeyEventArgs; if(keyEventArgs != null && IsDefinedKey(keyEventArgs.Key)) { return ( ( (int)Key == (int)keyEventArgs.RealKey ) && ( this.Modifiers == Keyboard.Modifiers ) ); } return false; } // Check for Valid enum, as any int can be casted to the enum. internal static bool IsDefinedKey(Key key) { return (key >= Key.None && key <= Key.OemClear); } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Is Valid Keyboard input to process for commands /// internal static bool IsValid(Key key, ModifierKeys modifiers) { // // Don't enforce any rules on the Function keys or on the number pad keys. // if(!( ( key >= Key.F1 && key <= Key.F24 ) || ( key >= Key.NumPad0 && key <= Key.Divide ) )) { // // We check whether Control/Alt/Windows key is down for modifiers. We don't check // for shift at this time as Shift with any combination is already covered in above check. // Shift alone as modifier case, we defer to the next condition to avoid conflicing with // TextInput. if(( modifiers & ( ModifierKeys.Control | ModifierKeys.Alt | ModifierKeys.Windows ) ) != 0) { switch(key) { case Key.LeftCtrl: case Key.RightCtrl: case Key.LeftAlt: case Key.RightAlt: case Key.LWin: case Key.RWin: return false; default: return true; } } else if(( key >= Key.D0 && key <= Key.D9 ) || ( key >= Key.A && key <= Key.Z )) { return false; } } return true; } ////// Decode the strings keyGestures and displayStrings, creating a sequence /// of KeyGestures. Add each KeyGesture to the given InputGestureCollection. /// The two input strings typically come from a resource file. /// internal static void AddGesturesFromResourceStrings(string keyGestures, string displayStrings, InputGestureCollection gestures) { while (!String.IsNullOrEmpty(keyGestures)) { string keyGestureToken; string keyDisplayString; // break apart first gesture from the rest int index = keyGestures.IndexOf(MULTIPLEGESTURE_DELIMITER, StringComparison.Ordinal); if (index >= 0) { // multiple gestures exist keyGestureToken = keyGestures.Substring(0, index); keyGestures = keyGestures.Substring(index + 1); } else { keyGestureToken = keyGestures; keyGestures = String.Empty; } // similarly, break apart first display string from the rest index = displayStrings.IndexOf(MULTIPLEGESTURE_DELIMITER, StringComparison.Ordinal); if (index >= 0) { // multiple display strings exist keyDisplayString = displayStrings.Substring(0, index); displayStrings = displayStrings.Substring(index + 1); } else { keyDisplayString = displayStrings; displayStrings = String.Empty; } KeyGesture keyGesture = CreateFromResourceStrings(keyGestureToken, keyDisplayString); if (keyGesture != null) { gestures.Add(keyGesture); } } } internal static KeyGesture CreateFromResourceStrings(string keyGestureToken, string keyDisplayString) { // combine the gesture and the display string, producing a string // that the type converter will recognize if (!String.IsNullOrEmpty(keyDisplayString)) { keyGestureToken += KeyGestureConverter.DISPLAYSTRING_SEPARATOR + keyDisplayString; } return _keyGestureConverter.ConvertFromInvariantString(keyGestureToken) as KeyGesture; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private ModifierKeys _modifiers = ModifierKeys.None; private Key _key = Key.None; private string _displayString; private const string MULTIPLEGESTURE_DELIMITER = ";"; private static TypeConverter _keyGestureConverter = new KeyGestureConverter(); //private static bool _classRegistered = false; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
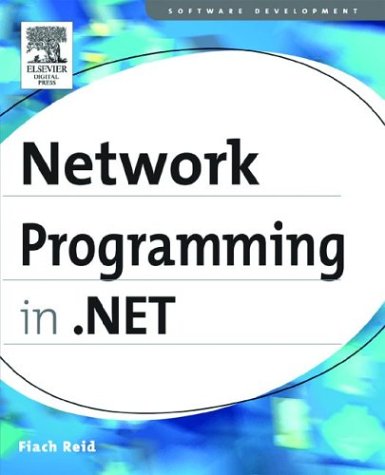
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeObjectFactory.cs
- WorkflowExecutor.cs
- UInt64.cs
- SimpleTableProvider.cs
- WebPartDisplayMode.cs
- objectquery_tresulttype.cs
- MDIControlStrip.cs
- SqlMethodTransformer.cs
- NameValuePermission.cs
- QilStrConcat.cs
- BigInt.cs
- ConsumerConnectionPoint.cs
- IncrementalHitTester.cs
- HotSpot.cs
- ClientSettingsProvider.cs
- SliderAutomationPeer.cs
- CommentAction.cs
- ZipIOFileItemStream.cs
- Properties.cs
- IndentedWriter.cs
- SHA256CryptoServiceProvider.cs
- DataGridViewAutoSizeModeEventArgs.cs
- InkPresenterAutomationPeer.cs
- BorderGapMaskConverter.cs
- XmlDomTextWriter.cs
- LineServicesCallbacks.cs
- Pens.cs
- AddInIpcChannel.cs
- TranslateTransform3D.cs
- Storyboard.cs
- LoopExpression.cs
- ImmutableCollection.cs
- AnnotationResourceChangedEventArgs.cs
- WindowsBrush.cs
- DataGridViewLinkCell.cs
- InteropExecutor.cs
- Typography.cs
- CookieParameter.cs
- EntityAdapter.cs
- ByteStreamGeometryContext.cs
- OneToOneMappingSerializer.cs
- ObjectAnimationUsingKeyFrames.cs
- BigInt.cs
- Binding.cs
- OdbcDataReader.cs
- DataGridViewCellEventArgs.cs
- ListChangedEventArgs.cs
- DataSourceExpressionCollection.cs
- ContextStaticAttribute.cs
- SharedUtils.cs
- PointAnimationBase.cs
- SerializerProvider.cs
- HostedElements.cs
- SafeReversePInvokeHandle.cs
- CmsInterop.cs
- DataTemplate.cs
- ForwardPositionQuery.cs
- HostnameComparisonMode.cs
- ComponentCollection.cs
- ExtentJoinTreeNode.cs
- NonBatchDirectoryCompiler.cs
- SuppressMergeCheckAttribute.cs
- ContentPlaceHolder.cs
- DesignRelation.cs
- TypeDelegator.cs
- X509Certificate2Collection.cs
- ExtensibleClassFactory.cs
- SocketManager.cs
- SchemaInfo.cs
- SqlParameter.cs
- RegexCapture.cs
- KeyedCollection.cs
- RegisteredHiddenField.cs
- ImagingCache.cs
- CompareValidator.cs
- EmissiveMaterial.cs
- BlurBitmapEffect.cs
- DataGridLength.cs
- SmiEventSink_DeferedProcessing.cs
- XsdDateTime.cs
- DesignerLoader.cs
- TextEditorThreadLocalStore.cs
- JoinTreeSlot.cs
- PieceNameHelper.cs
- IInstanceContextProvider.cs
- SafeTimerHandle.cs
- NullPackagingPolicy.cs
- Registry.cs
- SqlProfileProvider.cs
- TargetException.cs
- StoreContentChangedEventArgs.cs
- QueryCursorEventArgs.cs
- ConsumerConnectionPoint.cs
- ProxyWebPart.cs
- ColumnHeaderConverter.cs
- AudienceUriMode.cs
- GridViewRowCollection.cs
- DefaultShape.cs
- documentsequencetextview.cs
- Barrier.cs