Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / KeyEvent.cs / 1305376 / KeyEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Diagnostics.CodeAnalysis; ////// /// [System.Runtime.InteropServices.ComVisible(true)] public class KeyEventArgs : EventArgs { ////// Provides data for the ///or /// event. /// /// /// Contains key data for KeyDown and KeyUp events. This is a combination /// of keycode and modifer flags. /// private readonly Keys keyData; ////// /// Determines if this event has been handled by a handler. If handled, the /// key event will not be sent along to Windows. If not handled, the event /// will be sent to Windows for default processing. /// private bool handled = false; ////// /// private bool suppressKeyPress = false; ////// /// public KeyEventArgs(Keys keyData) { this.keyData = keyData; } ////// Initializes a new /// instance of the ///class. /// /// /// public virtual bool Alt { get { return (keyData & Keys.Alt) == Keys.Alt; } } ////// Gets a value indicating whether the ALT key was pressed. /// ////// /// public bool Control { get { return (keyData & Keys.Control) == Keys.Control; } } ////// Gets a value indicating whether the CTRL key was pressed. /// ////// /// // public bool Handled { get { return handled; } set { handled = value; } } ////// Gets or sets a value /// indicating whether the event was handled. /// ////// /// //subhag : changed the behaviour of the KeyCode as per the new requirements. public Keys KeyCode { [ // Keys is discontiguous so we have to use Enum.IsDefined. SuppressMessage("Microsoft.Performance", "CA1803:AvoidCostlyCallsWherePossible") ] get { Keys keyGenerated = keyData & Keys.KeyCode; // since Keys can be discontiguous, keeping Enum.IsDefined. if (!Enum.IsDefined(typeof(Keys),(int)keyGenerated)) return Keys.None; else return keyGenerated; } } ////// Gets the keyboard code for a ///or /// event. /// /// /// //subhag : added the KeyValue as per the new requirements. public int KeyValue { get { return (int)(keyData & Keys.KeyCode); } } ////// Gets the keyboard value for a ///or /// event. /// /// /// public Keys KeyData { get { return keyData; } } ////// Gets the key data for a ///or /// event. /// /// /// public Keys Modifiers { get { return keyData & Keys.Modifiers; } } ////// Gets the modifier flags for a ///or event. /// This indicates which modifier keys (CTRL, SHIFT, and/or ALT) were pressed. /// /// /// public virtual bool Shift { get { return (keyData & Keys.Shift) == Keys.Shift; } } ////// Gets /// a value indicating whether the SHIFT key was pressed. /// ////// /// // public bool SuppressKeyPress { get { return suppressKeyPress; } set { suppressKeyPress = value; handled = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Diagnostics.CodeAnalysis; ////// /// [System.Runtime.InteropServices.ComVisible(true)] public class KeyEventArgs : EventArgs { ////// Provides data for the ///or /// event. /// /// /// Contains key data for KeyDown and KeyUp events. This is a combination /// of keycode and modifer flags. /// private readonly Keys keyData; ////// /// Determines if this event has been handled by a handler. If handled, the /// key event will not be sent along to Windows. If not handled, the event /// will be sent to Windows for default processing. /// private bool handled = false; ////// /// private bool suppressKeyPress = false; ////// /// public KeyEventArgs(Keys keyData) { this.keyData = keyData; } ////// Initializes a new /// instance of the ///class. /// /// /// public virtual bool Alt { get { return (keyData & Keys.Alt) == Keys.Alt; } } ////// Gets a value indicating whether the ALT key was pressed. /// ////// /// public bool Control { get { return (keyData & Keys.Control) == Keys.Control; } } ////// Gets a value indicating whether the CTRL key was pressed. /// ////// /// // public bool Handled { get { return handled; } set { handled = value; } } ////// Gets or sets a value /// indicating whether the event was handled. /// ////// /// //subhag : changed the behaviour of the KeyCode as per the new requirements. public Keys KeyCode { [ // Keys is discontiguous so we have to use Enum.IsDefined. SuppressMessage("Microsoft.Performance", "CA1803:AvoidCostlyCallsWherePossible") ] get { Keys keyGenerated = keyData & Keys.KeyCode; // since Keys can be discontiguous, keeping Enum.IsDefined. if (!Enum.IsDefined(typeof(Keys),(int)keyGenerated)) return Keys.None; else return keyGenerated; } } ////// Gets the keyboard code for a ///or /// event. /// /// /// //subhag : added the KeyValue as per the new requirements. public int KeyValue { get { return (int)(keyData & Keys.KeyCode); } } ////// Gets the keyboard value for a ///or /// event. /// /// /// public Keys KeyData { get { return keyData; } } ////// Gets the key data for a ///or /// event. /// /// /// public Keys Modifiers { get { return keyData & Keys.Modifiers; } } ////// Gets the modifier flags for a ///or event. /// This indicates which modifier keys (CTRL, SHIFT, and/or ALT) were pressed. /// /// /// public virtual bool Shift { get { return (keyData & Keys.Shift) == Keys.Shift; } } ////// Gets /// a value indicating whether the SHIFT key was pressed. /// ////// /// // public bool SuppressKeyPress { get { return suppressKeyPress; } set { suppressKeyPress = value; handled = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
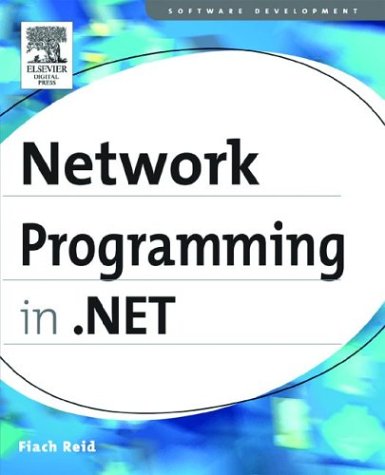
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PerformanceCountersElement.cs
- KeyGestureValueSerializer.cs
- BamlRecords.cs
- DependencyProperty.cs
- ColorIndependentAnimationStorage.cs
- PageParserFilter.cs
- XmlComment.cs
- ContextMenu.cs
- ReadOnlyDataSourceView.cs
- SyntaxCheck.cs
- NavigationPropertyEmitter.cs
- ToolStripGrip.cs
- LayoutDump.cs
- _SslState.cs
- SrgsElementFactory.cs
- ResourceExpressionBuilder.cs
- Switch.cs
- ISAPIApplicationHost.cs
- HtmlContainerControl.cs
- BindStream.cs
- MessageLogTraceRecord.cs
- TdsParserSessionPool.cs
- CacheModeConverter.cs
- ContainerAction.cs
- ListControlStringCollectionEditor.cs
- ResourceReferenceKeyNotFoundException.cs
- RemotingSurrogateSelector.cs
- RequestTimeoutManager.cs
- WindowsListViewGroup.cs
- Perspective.cs
- OleStrCAMarshaler.cs
- DataGridAddNewRow.cs
- PersonalizationDictionary.cs
- ReferentialConstraint.cs
- Translator.cs
- EntityDataSourceSelectingEventArgs.cs
- infer.cs
- PropertyTabChangedEvent.cs
- TimeZone.cs
- DNS.cs
- X509Extension.cs
- PeerInputChannel.cs
- FormViewPagerRow.cs
- EmbeddedMailObjectsCollection.cs
- UshortList2.cs
- XmlSchemaException.cs
- ImpersonateTokenRef.cs
- CredentialSelector.cs
- HtmlWindowCollection.cs
- TypeDescriptionProvider.cs
- AuthenticationService.cs
- FrameworkContextData.cs
- DataGridParentRows.cs
- SafeBitVector32.cs
- OleDbCommand.cs
- FormViewModeEventArgs.cs
- FormsAuthenticationUser.cs
- DataGridAutoFormatDialog.cs
- columnmapkeybuilder.cs
- UxThemeWrapper.cs
- ResourceWriter.cs
- DecimalAnimationUsingKeyFrames.cs
- SessionPageStateSection.cs
- Item.cs
- Axis.cs
- SliderAutomationPeer.cs
- XmlDocumentType.cs
- ExpressionDumper.cs
- PartialTrustVisibleAssembly.cs
- InputScopeConverter.cs
- HttpResponseMessageProperty.cs
- CodePropertyReferenceExpression.cs
- TimestampInformation.cs
- DocumentsTrace.cs
- SingleTagSectionHandler.cs
- X509Logo.cs
- Encoder.cs
- BitmapSource.cs
- BrowserCapabilitiesFactory.cs
- SafeNativeMethods.cs
- ComAdminInterfaces.cs
- CmsInterop.cs
- _SecureChannel.cs
- SpotLight.cs
- UniqueConstraint.cs
- XmlUnspecifiedAttribute.cs
- WebSysDescriptionAttribute.cs
- PagedDataSource.cs
- MutexSecurity.cs
- DelegateHelpers.cs
- QuaternionAnimation.cs
- SizeConverter.cs
- XpsLiterals.cs
- ProcessHostFactoryHelper.cs
- ComplusEndpointConfigContainer.cs
- RangeValuePattern.cs
- InitiatorSessionSymmetricTransportSecurityProtocol.cs
- _Connection.cs
- DelegatingHeader.cs
- SchemaSetCompiler.cs