Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Markup / RoutedEventConverter.cs / 1 / RoutedEventConverter.cs
// Copyright (C) Microsoft Corporation. All rights reserved. using System.ComponentModel; using System.Globalization; using System.Windows; namespace System.Windows.Markup { ////// Type converter for RoutedEvent type /// public sealed class RoutedEventConverter: TypeConverter { ////// Whether we can convert from a given type - this class only converts from string /// public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only convert from a string and that too only if we have all the contextual information // Note: Sometimes even the serializer calls CanConvertFrom in order // to determine if it is a valid converter to use for serialization. if (sourceType == typeof(string) && (typeDescriptorContext is TypeConvertContext)) { return true; } return false; } ////// Whether we can convert to a given type - this class only converts to string /// public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { return false; } ////// Convert a string like "Button.Click" into the corresponding RoutedEvent /// public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string routedEventName = source as string; if( routedEventName != null ) { IServiceProvider serviceProvier = typeDescriptorContext as IServiceProvider; if (serviceProvier != null) { TypeConvertContext typeConvertContext = typeDescriptorContext as TypeConvertContext; ParserContext parserContext = typeConvertContext == null ? null : typeConvertContext.ParserContext; if( parserContext != null ) { XamlTypeMapper xamlTypeMapper = parserContext.XamlTypeMapper; if( xamlTypeMapper != null ) { RoutedEvent routedEvent; string nameSpace; nameSpace = ExtractNamespaceString( ref routedEventName, parserContext ); // Verify that there's at least one period. (A simple // but not foolproof check for "[class].[event]") if( routedEventName.IndexOf('.') != -1 ) { // When xamlTypeMapper is told to look for RoutedEventID, // we need "[class].[event]" because at this point we // have no idea what the owner type might be. // Fortunately, given "[class].[event]" xamlTypeMapper.GetRoutedEventID // can figure things out, so at this point we can pass // in 'null' for the ownerType and things will still work. routedEvent = xamlTypeMapper.GetRoutedEvent(null /* ownerType */, nameSpace, routedEventName); return routedEvent; } } } } } // Falling through here means we are unable to perform the conversion. throw GetConvertFromException(source); } ////// Convert a RoutedEventID into a XAML string like "Button.Click" /// public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { throw GetConvertToException(value, destinationType); } // This routine is copied from TemplateBamlRecordReader. This functionality // is planned to be part of the utilities exposed by the parser, eliminating // the need to duplicate code. See task #18279 private string ExtractNamespaceString( ref string nameString, ParserContext parserContext ) { // The colon is what we look for to determine if there's a namespace prefix specifier. int nsIndex = nameString.IndexOf(':'); string nsPrefix = string.Empty; if( nsIndex != -1 ) { // Found a namespace prefix separator, so create replacement propertyName. // String processing - split "foons" from "BarClass.BazProp" nsPrefix = nameString.Substring (0, nsIndex); nameString = nameString.Substring (nsIndex + 1); } // Find the namespace, even if its the default one string namespaceURI = parserContext.XmlnsDictionary[nsPrefix]; if (namespaceURI == null) { throw new ArgumentException(SR.Get(SRID.ParserPrefixNSProperty, nsPrefix, nameString)); } return namespaceURI; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
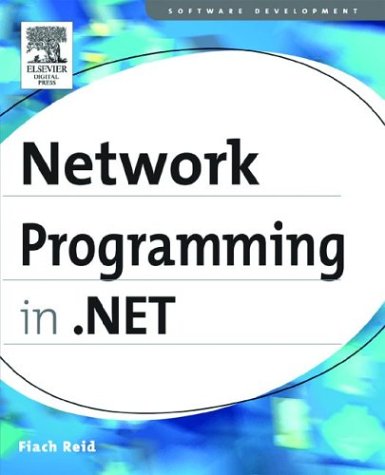
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- dataSvcMapFileLoader.cs
- XmlElementCollection.cs
- ResourceProviderFactory.cs
- IdentityHolder.cs
- PerformanceCounterLib.cs
- APCustomTypeDescriptor.cs
- FlowDecisionDesigner.xaml.cs
- SignalGate.cs
- BinaryObjectWriter.cs
- CharacterHit.cs
- UnsafeNativeMethods.cs
- MarshalByRefObject.cs
- Sequence.cs
- FileAccessException.cs
- DataGridViewRowPostPaintEventArgs.cs
- TextSpanModifier.cs
- ItemsChangedEventArgs.cs
- Point.cs
- ProjectionCamera.cs
- DocumentGrid.cs
- XmlSchemaAttribute.cs
- LightweightCodeGenerator.cs
- SqlBulkCopy.cs
- DataPagerFieldCommandEventArgs.cs
- TargetException.cs
- ListViewInsertedEventArgs.cs
- ReliableMessagingVersionConverter.cs
- EntityRecordInfo.cs
- ResourceProviderFactory.cs
- UrlMapping.cs
- ProvidePropertyAttribute.cs
- PermissionSetTriple.cs
- SynchronizedPool.cs
- TableFieldsEditor.cs
- StringAnimationBase.cs
- DbConnectionStringBuilder.cs
- Rect3D.cs
- ListViewSortEventArgs.cs
- DefaultMergeHelper.cs
- PersonalizationProviderHelper.cs
- IndexedString.cs
- HttpHandlerAction.cs
- SRDisplayNameAttribute.cs
- FormatterConverter.cs
- CompilationUtil.cs
- AsyncCodeActivity.cs
- WebHostedComPlusServiceHost.cs
- SessionIDManager.cs
- FileVersion.cs
- UnaryOperationBinder.cs
- AccessedThroughPropertyAttribute.cs
- ChangeBlockUndoRecord.cs
- SymLanguageType.cs
- Imaging.cs
- PlanCompiler.cs
- QfeChecker.cs
- ConfigXmlCDataSection.cs
- PowerStatus.cs
- SplayTreeNode.cs
- LessThanOrEqual.cs
- ApplicationServiceManager.cs
- SoapObjectWriter.cs
- DataGridViewAutoSizeModeEventArgs.cs
- CodePropertyReferenceExpression.cs
- RecordsAffectedEventArgs.cs
- ServiceDiscoveryElement.cs
- WebBrowserNavigatedEventHandler.cs
- GridViewHeaderRowPresenter.cs
- BitmapEffectInputData.cs
- SqlDataSourceCommandEventArgs.cs
- CodeDelegateCreateExpression.cs
- CodeEventReferenceExpression.cs
- BaseCollection.cs
- SocketException.cs
- StringTraceRecord.cs
- FontFamilyConverter.cs
- DrawListViewColumnHeaderEventArgs.cs
- CustomAttributeBuilder.cs
- GeometryCollection.cs
- UnknownBitmapDecoder.cs
- ContentControl.cs
- SignerInfo.cs
- MaxMessageSizeStream.cs
- fixedPageContentExtractor.cs
- GPPOINTF.cs
- AssociationSetEnd.cs
- ToolStripProgressBar.cs
- BuildManager.cs
- RegionIterator.cs
- DiagnosticsConfigurationHandler.cs
- InheritablePropertyChangeInfo.cs
- TextEffect.cs
- TimeStampChecker.cs
- Contracts.cs
- XmlSchemaObjectTable.cs
- Quack.cs
- ObjectManager.cs
- Permission.cs
- XsdDuration.cs
- ZipArchive.cs