Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Generated / Point.cs / 1305600 / Point.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows { [Serializable] [TypeConverter(typeof(PointConverter))] [ValueSerializer(typeof(PointValueSerializer))] // Used by MarkupWriter partial struct Point : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Point instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Point instances are exactly equal, false otherwise /// /// The first Point to compare /// The second Point to compare public static bool operator == (Point point1, Point point2) { return point1.X == point2.X && point1.Y == point2.Y; } ////// Compares two Point instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Point instances are exactly unequal, false otherwise /// /// The first Point to compare /// The second Point to compare public static bool operator != (Point point1, Point point2) { return !(point1 == point2); } ////// Compares two Point instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Point instances are exactly equal, false otherwise /// /// The first Point to compare /// The second Point to compare public static bool Equals (Point point1, Point point2) { return point1.X.Equals(point2.X) && point1.Y.Equals(point2.Y); } ////// Equals - compares this Point with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Point and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Point)) { return false; } Point value = (Point)o; return Point.Equals(this,value); } ////// Equals - compares this Point with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Point to compare to "this" public bool Equals(Point value) { return Point.Equals(this, value); } ////// Returns the HashCode for this Point /// ////// int - the HashCode for this Point /// public override int GetHashCode() { // Perform field-by-field XOR of HashCodes return X.GetHashCode() ^ Y.GetHashCode(); } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Point data /// public static Point Parse(string source) { IFormatProvider formatProvider = System.Windows.Markup.TypeConverterHelper.InvariantEnglishUS; TokenizerHelper th = new TokenizerHelper(source, formatProvider); Point value; String firstToken = th.NextTokenRequired(); value = new Point( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// X - double. Default value is 0. /// public double X { get { return _x; } set { _x = value; } } ////// Y - double. Default value is 0. /// public double Y { get { return _y; } set { _y = value; } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}", separator, _x, _y); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal double _x; internal double _y; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
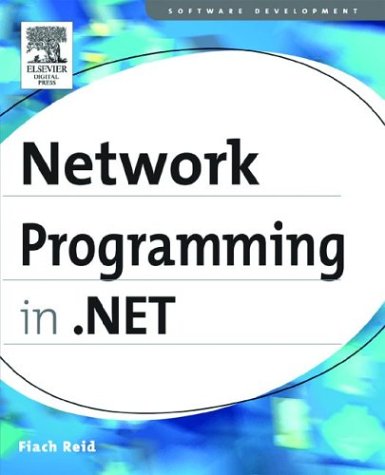
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GB18030Encoding.cs
- XmlConvert.cs
- Decoder.cs
- PasswordBox.cs
- DataGridViewRowStateChangedEventArgs.cs
- OrthographicCamera.cs
- ReversePositionQuery.cs
- GridView.cs
- XsdCachingReader.cs
- ResourceReader.cs
- SubpageParaClient.cs
- FromReply.cs
- SudsCommon.cs
- TextRange.cs
- XmlSchemaCollection.cs
- FontStyle.cs
- FixedSOMLineCollection.cs
- DrawTreeNodeEventArgs.cs
- DataGridViewCellStyleBuilderDialog.cs
- RuntimeIdentifierPropertyAttribute.cs
- StrokeCollection.cs
- SpeechUI.cs
- ClipboardProcessor.cs
- List.cs
- DataListItemCollection.cs
- SmiContext.cs
- StandardTransformFactory.cs
- HideDisabledControlAdapter.cs
- CookieProtection.cs
- FacetEnabledSchemaElement.cs
- PackWebRequest.cs
- UserMapPath.cs
- AttributeQuery.cs
- TimersDescriptionAttribute.cs
- SafeWaitHandle.cs
- CodeDefaultValueExpression.cs
- XmlILConstructAnalyzer.cs
- XmlILStorageConverter.cs
- ColumnReorderedEventArgs.cs
- Decimal.cs
- AssemblyInfo.cs
- BulletedListEventArgs.cs
- PersonalizationEntry.cs
- ThicknessAnimation.cs
- RichTextBox.cs
- ToolStripPanel.cs
- CustomActivityDesigner.cs
- MessageEncoder.cs
- BoundColumn.cs
- XmlObjectSerializerReadContextComplex.cs
- XmlnsPrefixAttribute.cs
- OpenFileDialog.cs
- CheckableControlBaseAdapter.cs
- DescriptionAttribute.cs
- PageContent.cs
- PenThread.cs
- Decoder.cs
- SystemBrushes.cs
- ProvideValueServiceProvider.cs
- ComPlusSynchronizationContext.cs
- IUnknownConstantAttribute.cs
- WebConfigurationHost.cs
- RectIndependentAnimationStorage.cs
- RegistryDataKey.cs
- WorkflowMarkupSerializationException.cs
- ParseChildrenAsPropertiesAttribute.cs
- LockedActivityGlyph.cs
- BindingGraph.cs
- UpdateManifestForBrowserApplication.cs
- TabItemAutomationPeer.cs
- ContentIterators.cs
- WorkflowApplicationAbortedException.cs
- KnowledgeBase.cs
- Reference.cs
- CodeGeneratorOptions.cs
- SerializerProvider.cs
- CroppedBitmap.cs
- BrushValueSerializer.cs
- SchemaMerger.cs
- DataBoundLiteralControl.cs
- VectorAnimationBase.cs
- Assign.cs
- EventPrivateKey.cs
- DetailsViewUpdateEventArgs.cs
- AttributeSetAction.cs
- XamlFxTrace.cs
- ServiceCredentialsElement.cs
- DupHandleConnectionReader.cs
- SurrogateDataContract.cs
- CollectionChange.cs
- CalendarDateRangeChangingEventArgs.cs
- AttachInfo.cs
- mda.cs
- ClientConfigurationSystem.cs
- PropertyMapper.cs
- WebServiceReceiveDesigner.cs
- ApplicationInfo.cs
- HelloOperationAsyncResult.cs
- DoubleAverageAggregationOperator.cs
- Helpers.cs