Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / GetCryptoTransformRequest.cs / 1 / GetCryptoTransformRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Diagnostics; using System.Security.Principal; using System.Threading; //ManualResetEvent using System.ComponentModel; //Win32Exception using System.IO; //Stream using System.Security.Cryptography; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Wraps a request to get a remoted ICryptoTransform interface based on a SymmetricCryptoSession. // class GetCryptoTransformRequest : ClientRequest { // // The cryptosession id we are attaching to. // int m_cryptoSession; // // The cipher mode being requested. // CipherMode m_mode; // // The padding mode being requested. // PaddingMode m_padding; // // The feedback size being requested // int m_feedbackSize; // // Is Encryption or Decryption being requested. // SymmetricCryptoSession.Direction m_direction; // // The intialization vector. // byte[] m_iv; // // The returned transform session. // TransformCryptoSession m_transformSession; // // Sumamry: // Construct a GetCryptoTransformRequest object // // Arguments: // callingProcess - The process in which the caller originated. // callingIdentity - The WindowsIdentity of the caller // rpcHandle - The handle of the native RPC request // inArgs - The stream to read input data from // outArgs - The stream to write output data to // public GetCryptoTransformRequest( Process callingProcess, WindowsIdentity callingIdentity, IntPtr rpcHandle, Stream inArgs, Stream outArgs ) : base( callingProcess, callingIdentity, rpcHandle, inArgs, outArgs ) { IDT.TraceDebug( "Intiating a GetCryptoTransform request" ); } protected override void OnMarshalInArgs() { IDT.DebugAssert( null != InArgs, "null inargs" ); BinaryReader reader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); m_cryptoSession = reader.ReadInt32(); m_mode = (CipherMode)reader.ReadInt32(); m_padding = (PaddingMode)reader.ReadInt32(); m_feedbackSize = reader.ReadInt32(); m_direction = (SymmetricCryptoSession.Direction)reader.ReadInt32(); m_iv = reader.ReadBytes( reader.ReadInt32() ); IDT.ThrowInvalidArgumentConditional( 0 == m_feedbackSize, "feedbackSize" ); } // // Summary: // Attach to the appropriate cryptosession and get a CryptoTransform // protected override void OnProcess() { SymmetricCryptoSession session = ( SymmetricCryptoSession )CryptoSession.Find( m_cryptoSession, CallerPid, RequestorIdentity.User ); m_transformSession = session.GetCryptoTransform( m_mode, m_padding, m_feedbackSize, m_direction, m_iv ); } // // Summary: // Return the TransformCryptoSession // protected override void OnMarshalOutArgs() { IDT.DebugAssert( null != OutArgs, "Null out args" ); BinaryWriter writer = new BinaryWriter( OutArgs, Encoding.Unicode ); IDT.DebugAssert( null != m_transformSession, "unexpected null outgoing transfromSession" ); m_transformSession.Write( writer ); writer.Flush(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
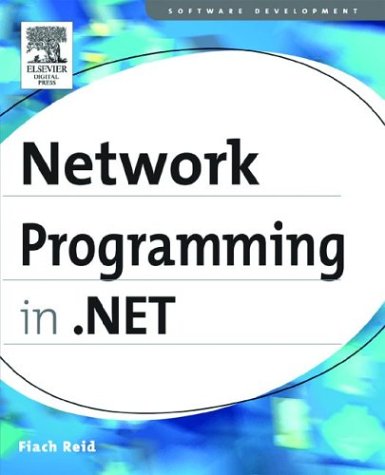
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Panel.cs
- TraversalRequest.cs
- StorageMappingItemCollection.cs
- GeneralTransform2DTo3DTo2D.cs
- DataTransferEventArgs.cs
- Monitor.cs
- MarshalDirectiveException.cs
- GuidTagList.cs
- TextBox.cs
- PieceNameHelper.cs
- ProxyWebPartManager.cs
- XhtmlTextWriter.cs
- regiisutil.cs
- ProxyHwnd.cs
- RequestCache.cs
- SqlTriggerContext.cs
- XmlSchemaProviderAttribute.cs
- _HeaderInfoTable.cs
- CodeAttributeDeclaration.cs
- ProviderCommandInfoUtils.cs
- CheckBoxList.cs
- TextRange.cs
- UIElementIsland.cs
- StrokeNodeOperations2.cs
- TranslateTransform.cs
- DuplicateDetector.cs
- COAUTHINFO.cs
- ParentQuery.cs
- Ray3DHitTestResult.cs
- CommandLibraryHelper.cs
- EncryptedType.cs
- SafePEFileHandle.cs
- KeyInterop.cs
- KerberosSecurityTokenProvider.cs
- Attributes.cs
- ItemsPanelTemplate.cs
- RawMouseInputReport.cs
- PropertyChangingEventArgs.cs
- AnnotationResourceChangedEventArgs.cs
- DragEventArgs.cs
- MatrixCamera.cs
- invalidudtexception.cs
- EntityDataSourceViewSchema.cs
- ToolStripItemImageRenderEventArgs.cs
- TabItemWrapperAutomationPeer.cs
- XmlSchemaComplexContentRestriction.cs
- ServiceRouteHandler.cs
- XdrBuilder.cs
- TrackingParameters.cs
- SharedPersonalizationStateInfo.cs
- figurelengthconverter.cs
- OledbConnectionStringbuilder.cs
- MembershipPasswordException.cs
- ActiveXContainer.cs
- GeometryDrawing.cs
- PageAdapter.cs
- QilTernary.cs
- XamlGridLengthSerializer.cs
- _RequestLifetimeSetter.cs
- WebControlsSection.cs
- RegistrationServices.cs
- StorageFunctionMapping.cs
- QuotedPairReader.cs
- DataServicePagingProviderWrapper.cs
- ClrPerspective.cs
- ScaleTransform.cs
- UnsafeNativeMethods.cs
- SafeTimerHandle.cs
- FixedSOMTextRun.cs
- DoubleCollectionValueSerializer.cs
- StoreAnnotationsMap.cs
- TableAdapterManagerNameHandler.cs
- UIElementCollection.cs
- Animatable.cs
- DataGridViewRowsRemovedEventArgs.cs
- ColorConverter.cs
- CodeGen.cs
- Point3DConverter.cs
- CodeDomSerializerException.cs
- SmiContext.cs
- DropShadowBitmapEffect.cs
- WebBrowserNavigatingEventHandler.cs
- SystemThemeKey.cs
- XamlClipboardData.cs
- SafeLibraryHandle.cs
- Utilities.cs
- SelectedDatesCollection.cs
- BamlRecordReader.cs
- MsmqIntegrationProcessProtocolHandler.cs
- SmiEventSink_Default.cs
- AssociationType.cs
- EmptyImpersonationContext.cs
- FormViewInsertEventArgs.cs
- Permission.cs
- ResourceKey.cs
- ListViewItem.cs
- TreeView.cs
- SingleBodyParameterMessageFormatter.cs
- ProviderUtil.cs
- Debug.cs