Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Mapping / StorageFunctionMapping.cs / 2 / StorageFunctionMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....],[....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Data.Common.Utils; namespace System.Data.Mapping { ////// Describes function mappings for an association set. /// internal sealed class StorageAssociationSetFunctionMapping { internal StorageAssociationSetFunctionMapping( AssociationSet associationSet, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping) { this.AssociationSet = EntityUtil.CheckArgumentNull(associationSet, "associationSet"); this.DeleteFunctionMapping = EntityUtil.CheckArgumentNull(deleteFunctionMapping, "deleteFunctionMapping"); this.InsertFunctionMapping = EntityUtil.CheckArgumentNull(insertFunctionMapping, "insertFunctionMapping"); } ////// Association set these functions handles. /// internal readonly AssociationSet AssociationSet; ////// Delete function for this association set. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Insert function for this association set. /// internal readonly StorageFunctionMapping InsertFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "AS{{{0}}}:{3}DFunc={{{1}}},{3}IFunc={{{2}}}", AssociationSet, DeleteFunctionMapping, InsertFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Association Set Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function mappings for an entity type within an entity set. /// internal sealed class StorageEntityTypeFunctionMapping { internal StorageEntityTypeFunctionMapping( EntityType entityType, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping, StorageFunctionMapping updateFunctionMapping) { this.EntityType = EntityUtil.CheckArgumentNull(entityType, "entityType"); this.DeleteFunctionMapping = EntityUtil.CheckArgumentNull(deleteFunctionMapping, "deleteFunctionMapping"); this.InsertFunctionMapping = EntityUtil.CheckArgumentNull(insertFunctionMapping, "insertFunctionMapping"); this.UpdateFunctionMapping = EntityUtil.CheckArgumentNull(updateFunctionMapping, "updateFunctionMapping"); } ////// Gets (specific) entity type these functions handle. /// internal readonly EntityType EntityType; ////// Gets delete function for the current entity type. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Gets insert function for the current entity type. /// internal readonly StorageFunctionMapping InsertFunctionMapping; ////// Gets update function for the current entity type. /// internal readonly StorageFunctionMapping UpdateFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "ET{{{0}}}:{4}DFunc={{{1}}},{4}IFunc={{{2}}},{4}UFunc={{{3}}}", EntityType, DeleteFunctionMapping, InsertFunctionMapping, UpdateFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Entity Type Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function binding for change processing of entities or associations. /// internal sealed class StorageFunctionMapping { internal StorageFunctionMapping(EdmFunction function, IEnumerableparameterBindings, FunctionParameter rowsAffectedParameter, IEnumerable resultBindings) { this.RowsAffectedParameter = rowsAffectedParameter; this.Function = EntityUtil.CheckArgumentNull(function, "function"); this.ParameterBindings = new ReadOnlyCollection ( new List (EntityUtil.CheckArgumentNull(parameterBindings, "parameterBindings"))); if (null != resultBindings) { List bindings = new List (resultBindings); if (0 < bindings.Count) { ResultBindings = new ReadOnlyCollection (bindings); } } } /// /// Gets output parameter producing number of rows affected. May be null. /// internal readonly FunctionParameter RowsAffectedParameter; ////// Gets Metadata of function to which we should bind. /// internal readonly EdmFunction Function; ////// Gets bindings for function parameters. /// internal readonly ReadOnlyCollectionParameterBindings; /// /// Gets bindings for the results of function evaluation. /// internal readonly ReadOnlyCollectionResultBindings; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "Func{{{0}}}: Prm={{{1}}}, Result={{{2}}}", Function, StringUtil.ToCommaSeparatedStringSorted(ParameterBindings), StringUtil.ToCommaSeparatedStringSorted(ResultBindings)); } // requires: entitySet and associationSetEnds must not be null // Walks this function mapping to find any references to association sets, adding them to // the given list. internal void AddReferencedAssociationSetEnds(EntitySet entitySet, List associationSetEnds) { Debug.Assert(null != associationSetEnds); associationSetEnds.AddRange(GetReferencedAssociationSetEnds(entitySet)); } // requires: entitySet must not be null // Yields all referenced association set ends in this mapping. internal IEnumerable GetReferencedAssociationSetEnds(EntitySet entitySet) { Debug.Assert(null != entitySet); foreach (StorageFunctionParameterBinding parameterBinding in this.ParameterBindings) { AssociationSetEnd end = parameterBinding.MemberPath.AssociationSetEnd; if (null != end) { yield return end; } } // If there is a referential constraint, it counts as an implicit mapping of // the association set foreach (AssociationSet assocationSet in MetadataHelper.GetAssociationsForEntitySet(entitySet)) { ReadOnlyMetadataCollection constraints = assocationSet.ElementType.ReferentialConstraints; if (null != constraints) { foreach (ReferentialConstraint constraint in constraints) { if (assocationSet.AssociationSetEnds[constraint.ToRole.Name].EntitySet == entitySet) { yield return assocationSet.AssociationSetEnds[constraint.FromRole.Name]; } } } } } } /// /// Defines a binding from a named result set column to a member taking the value. /// internal sealed class StorageFunctionResultBinding { internal StorageFunctionResultBinding(string columnName, EdmProperty property) { this.ColumnName = EntityUtil.CheckArgumentNull(columnName, "columnName"); this.Property = EntityUtil.CheckArgumentNull(property, "property"); } ////// Gets the name of the column to bind from the function result set. We use a string /// value rather than EdmMember, since there is no metadata for function result sets. /// internal readonly string ColumnName; ////// Gets the property to be set on the entity. /// internal readonly EdmProperty Property; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}->{1}", ColumnName, Property); } } ////// Binds a function parameter to a member of the entity or association being modified. /// internal sealed class StorageFunctionParameterBinding { internal StorageFunctionParameterBinding(FunctionParameter parameter, StorageFunctionMemberPath memberPath, bool isCurrent) { this.Parameter = EntityUtil.CheckArgumentNull(parameter, "parameter"); this.MemberPath = EntityUtil.CheckArgumentNull(memberPath, "memberPath"); this.IsCurrent = isCurrent; } ////// Gets the parameter taking the value. /// internal readonly FunctionParameter Parameter; ////// Gets the path to the entity or association member defining the value. /// internal readonly StorageFunctionMemberPath MemberPath; ////// Gets a value indicating whether the current or original /// member value is being bound. /// internal readonly bool IsCurrent; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "@{0}->{1}{2}", Parameter, IsCurrent ? "+" : "-", MemberPath); } } ////// Describes the location of a member within an entity or association type structure. /// internal sealed class StorageFunctionMemberPath { internal StorageFunctionMemberPath(IEnumerablemembers, AssociationSet associationSetNavigation) { this.Members = new ReadOnlyCollection (new List ( EntityUtil.CheckArgumentNull(members, "members"))); if (null != associationSetNavigation) { Debug.Assert(2 == this.Members.Count, "Association bindings must always consist of the end and the key"); // find the association set end this.AssociationSetEnd = associationSetNavigation.AssociationSetEnds[this.Members[1].Name]; } } /// /// Gets the members in the path from the leaf (the member being bound) /// to the Root of the structure. /// internal readonly ReadOnlyCollectionMembers; /// /// Gets the association set to which we are navigating via this member. If the value /// is null, this is not a navigation member path. /// internal readonly AssociationSetEnd AssociationSetEnd; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}{1}", null == AssociationSetEnd ? String.Empty : "[" + AssociationSetEnd.ParentAssociationSet.ToString() + "]", StringUtil.BuildDelimitedList(Members, null, ".")); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....],[....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Data.Common.Utils; namespace System.Data.Mapping { ////// Describes function mappings for an association set. /// internal sealed class StorageAssociationSetFunctionMapping { internal StorageAssociationSetFunctionMapping( AssociationSet associationSet, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping) { this.AssociationSet = EntityUtil.CheckArgumentNull(associationSet, "associationSet"); this.DeleteFunctionMapping = EntityUtil.CheckArgumentNull(deleteFunctionMapping, "deleteFunctionMapping"); this.InsertFunctionMapping = EntityUtil.CheckArgumentNull(insertFunctionMapping, "insertFunctionMapping"); } ////// Association set these functions handles. /// internal readonly AssociationSet AssociationSet; ////// Delete function for this association set. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Insert function for this association set. /// internal readonly StorageFunctionMapping InsertFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "AS{{{0}}}:{3}DFunc={{{1}}},{3}IFunc={{{2}}}", AssociationSet, DeleteFunctionMapping, InsertFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Association Set Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function mappings for an entity type within an entity set. /// internal sealed class StorageEntityTypeFunctionMapping { internal StorageEntityTypeFunctionMapping( EntityType entityType, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping, StorageFunctionMapping updateFunctionMapping) { this.EntityType = EntityUtil.CheckArgumentNull(entityType, "entityType"); this.DeleteFunctionMapping = EntityUtil.CheckArgumentNull(deleteFunctionMapping, "deleteFunctionMapping"); this.InsertFunctionMapping = EntityUtil.CheckArgumentNull(insertFunctionMapping, "insertFunctionMapping"); this.UpdateFunctionMapping = EntityUtil.CheckArgumentNull(updateFunctionMapping, "updateFunctionMapping"); } ////// Gets (specific) entity type these functions handle. /// internal readonly EntityType EntityType; ////// Gets delete function for the current entity type. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Gets insert function for the current entity type. /// internal readonly StorageFunctionMapping InsertFunctionMapping; ////// Gets update function for the current entity type. /// internal readonly StorageFunctionMapping UpdateFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "ET{{{0}}}:{4}DFunc={{{1}}},{4}IFunc={{{2}}},{4}UFunc={{{3}}}", EntityType, DeleteFunctionMapping, InsertFunctionMapping, UpdateFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Entity Type Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function binding for change processing of entities or associations. /// internal sealed class StorageFunctionMapping { internal StorageFunctionMapping(EdmFunction function, IEnumerableparameterBindings, FunctionParameter rowsAffectedParameter, IEnumerable resultBindings) { this.RowsAffectedParameter = rowsAffectedParameter; this.Function = EntityUtil.CheckArgumentNull(function, "function"); this.ParameterBindings = new ReadOnlyCollection ( new List (EntityUtil.CheckArgumentNull(parameterBindings, "parameterBindings"))); if (null != resultBindings) { List bindings = new List (resultBindings); if (0 < bindings.Count) { ResultBindings = new ReadOnlyCollection (bindings); } } } /// /// Gets output parameter producing number of rows affected. May be null. /// internal readonly FunctionParameter RowsAffectedParameter; ////// Gets Metadata of function to which we should bind. /// internal readonly EdmFunction Function; ////// Gets bindings for function parameters. /// internal readonly ReadOnlyCollectionParameterBindings; /// /// Gets bindings for the results of function evaluation. /// internal readonly ReadOnlyCollectionResultBindings; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "Func{{{0}}}: Prm={{{1}}}, Result={{{2}}}", Function, StringUtil.ToCommaSeparatedStringSorted(ParameterBindings), StringUtil.ToCommaSeparatedStringSorted(ResultBindings)); } // requires: entitySet and associationSetEnds must not be null // Walks this function mapping to find any references to association sets, adding them to // the given list. internal void AddReferencedAssociationSetEnds(EntitySet entitySet, List associationSetEnds) { Debug.Assert(null != associationSetEnds); associationSetEnds.AddRange(GetReferencedAssociationSetEnds(entitySet)); } // requires: entitySet must not be null // Yields all referenced association set ends in this mapping. internal IEnumerable GetReferencedAssociationSetEnds(EntitySet entitySet) { Debug.Assert(null != entitySet); foreach (StorageFunctionParameterBinding parameterBinding in this.ParameterBindings) { AssociationSetEnd end = parameterBinding.MemberPath.AssociationSetEnd; if (null != end) { yield return end; } } // If there is a referential constraint, it counts as an implicit mapping of // the association set foreach (AssociationSet assocationSet in MetadataHelper.GetAssociationsForEntitySet(entitySet)) { ReadOnlyMetadataCollection constraints = assocationSet.ElementType.ReferentialConstraints; if (null != constraints) { foreach (ReferentialConstraint constraint in constraints) { if (assocationSet.AssociationSetEnds[constraint.ToRole.Name].EntitySet == entitySet) { yield return assocationSet.AssociationSetEnds[constraint.FromRole.Name]; } } } } } } /// /// Defines a binding from a named result set column to a member taking the value. /// internal sealed class StorageFunctionResultBinding { internal StorageFunctionResultBinding(string columnName, EdmProperty property) { this.ColumnName = EntityUtil.CheckArgumentNull(columnName, "columnName"); this.Property = EntityUtil.CheckArgumentNull(property, "property"); } ////// Gets the name of the column to bind from the function result set. We use a string /// value rather than EdmMember, since there is no metadata for function result sets. /// internal readonly string ColumnName; ////// Gets the property to be set on the entity. /// internal readonly EdmProperty Property; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}->{1}", ColumnName, Property); } } ////// Binds a function parameter to a member of the entity or association being modified. /// internal sealed class StorageFunctionParameterBinding { internal StorageFunctionParameterBinding(FunctionParameter parameter, StorageFunctionMemberPath memberPath, bool isCurrent) { this.Parameter = EntityUtil.CheckArgumentNull(parameter, "parameter"); this.MemberPath = EntityUtil.CheckArgumentNull(memberPath, "memberPath"); this.IsCurrent = isCurrent; } ////// Gets the parameter taking the value. /// internal readonly FunctionParameter Parameter; ////// Gets the path to the entity or association member defining the value. /// internal readonly StorageFunctionMemberPath MemberPath; ////// Gets a value indicating whether the current or original /// member value is being bound. /// internal readonly bool IsCurrent; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "@{0}->{1}{2}", Parameter, IsCurrent ? "+" : "-", MemberPath); } } ////// Describes the location of a member within an entity or association type structure. /// internal sealed class StorageFunctionMemberPath { internal StorageFunctionMemberPath(IEnumerablemembers, AssociationSet associationSetNavigation) { this.Members = new ReadOnlyCollection (new List ( EntityUtil.CheckArgumentNull(members, "members"))); if (null != associationSetNavigation) { Debug.Assert(2 == this.Members.Count, "Association bindings must always consist of the end and the key"); // find the association set end this.AssociationSetEnd = associationSetNavigation.AssociationSetEnds[this.Members[1].Name]; } } /// /// Gets the members in the path from the leaf (the member being bound) /// to the Root of the structure. /// internal readonly ReadOnlyCollectionMembers; /// /// Gets the association set to which we are navigating via this member. If the value /// is null, this is not a navigation member path. /// internal readonly AssociationSetEnd AssociationSetEnd; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}{1}", null == AssociationSetEnd ? String.Empty : "[" + AssociationSetEnd.ParentAssociationSet.ToString() + "]", StringUtil.BuildDelimitedList(Members, null, ".")); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
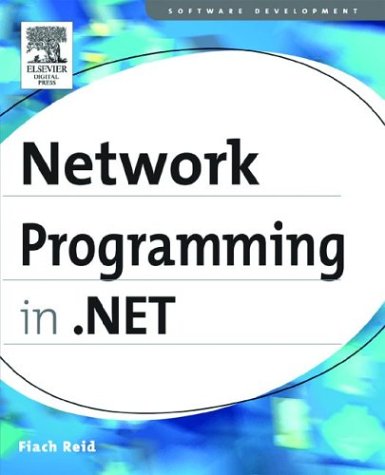
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Config.cs
- InputBuffer.cs
- PropertyGridEditorPart.cs
- TemplatePartAttribute.cs
- InternalConfigEventArgs.cs
- ProgressPage.cs
- NullableBoolConverter.cs
- SmiRecordBuffer.cs
- PtsContext.cs
- WebPartManagerInternals.cs
- WindowsIPAddress.cs
- MergeExecutor.cs
- DocumentViewerAutomationPeer.cs
- MasterPageParser.cs
- DataGridTableStyleMappingNameEditor.cs
- XmlHierarchicalDataSourceView.cs
- ControlBuilderAttribute.cs
- AQNBuilder.cs
- NavigationProperty.cs
- CustomAttributeBuilder.cs
- PathSegmentCollection.cs
- AudienceUriMode.cs
- Native.cs
- DrawListViewColumnHeaderEventArgs.cs
- SocketException.cs
- HwndMouseInputProvider.cs
- ConfigurationValidatorAttribute.cs
- EdmFunctionAttribute.cs
- Soap12ProtocolImporter.cs
- EntityConnectionStringBuilderItem.cs
- QfeChecker.cs
- ReceiveContent.cs
- SqlDeflator.cs
- EventlogProvider.cs
- Delegate.cs
- ContainerActivationHelper.cs
- CodeTypeParameterCollection.cs
- FtpWebResponse.cs
- ScriptResourceInfo.cs
- LinkButton.cs
- TemplateKey.cs
- DecimalStorage.cs
- RootProfilePropertySettingsCollection.cs
- ImageAttributes.cs
- DataControlFieldHeaderCell.cs
- DocumentPageHost.cs
- SerializationHelper.cs
- ContentDisposition.cs
- sapiproxy.cs
- ListDesigner.cs
- IApplicationTrustManager.cs
- BaseContextMenu.cs
- TagPrefixCollection.cs
- ClosureBinding.cs
- SqlCachedBuffer.cs
- CapabilitiesState.cs
- LocalIdKeyIdentifierClause.cs
- SiteMapNodeCollection.cs
- ExpressionList.cs
- DesignerView.cs
- cookie.cs
- VariableExpressionConverter.cs
- GeneralTransform.cs
- Size3DConverter.cs
- ProviderCollection.cs
- KeyInfo.cs
- FlowDocument.cs
- ActivityBuilderHelper.cs
- FontFaceLayoutInfo.cs
- NamespaceExpr.cs
- ColorTransformHelper.cs
- RoleGroupCollection.cs
- _UriSyntax.cs
- StringConverter.cs
- TailPinnedEventArgs.cs
- BindableAttribute.cs
- HttpsHostedTransportConfiguration.cs
- SplineKeyFrames.cs
- PermissionToken.cs
- ObjectViewEntityCollectionData.cs
- SortFieldComparer.cs
- OutputCacheModule.cs
- ScrollProviderWrapper.cs
- SchemaExporter.cs
- WhitespaceRuleLookup.cs
- CatchBlock.cs
- Variant.cs
- ResourceKey.cs
- SelectedCellsCollection.cs
- Validator.cs
- GACIdentityPermission.cs
- ObjectViewEntityCollectionData.cs
- AutomationIdentifierGuids.cs
- UpdateExpressionVisitor.cs
- FormattedText.cs
- PublishLicense.cs
- PointLightBase.cs
- PropertyConverter.cs
- HtmlTableRow.cs
- ReachUIElementCollectionSerializerAsync.cs