Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Advanced / ImageAttributes.cs / 2 / ImageAttributes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Drawing.Drawing2D; using System.Drawing.Internal; using System.Globalization; // sdkinc\GDIplusImageAttributes.h // There are 5 possible sets of color adjustments: // ColorAdjustDefault, // ColorAdjustBitmap, // ColorAdjustBrush, // ColorAdjustPen, // ColorAdjustText, // Bitmaps, Brushes, Pens, and Text will all use any color adjustments // that have been set into the default ImageAttributes until their own // color adjustments have been set. So as soon as any "Set" method is // called for Bitmaps, Brushes, Pens, or Text, then they start from // scratch with only the color adjustments that have been set for them. // Calling Reset removes any individual color adjustments for a type // and makes it revert back to using all the default color adjustments // (if any). The SetToIdentity method is a way to force a type to // have no color adjustments at all, regardless of what previous adjustments // have been set for the defaults or for that type. ////// /// Contains information about how image colors /// are manipulated during rendering. /// [StructLayout(LayoutKind.Sequential)] public sealed class ImageAttributes : ICloneable, IDisposable { #if FINALIZATION_WATCH private string allocationSite = Graphics.GetAllocationStack(); #endif /* * Handle to native image attributes object */ internal IntPtr nativeImageAttributes; internal void SetNativeImageAttributes(IntPtr handle) { if (handle == IntPtr.Zero) throw new ArgumentNullException("handle"); nativeImageAttributes = handle; } ////// /// Initializes a new instance of the public ImageAttributes() { IntPtr newImageAttributes = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateImageAttributes(out newImageAttributes); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImageAttributes(newImageAttributes); } internal ImageAttributes(IntPtr newNativeImageAttributes) { SetNativeImageAttributes(newNativeImageAttributes); } ///class. /// /// /// Cleans up Windows resources for this /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { #if FINALIZATION_WATCH if (!disposing && nativeImageAttributes != IntPtr.Zero) Debug.WriteLine("**********************\nDisposed through finalization:\n" + allocationSite); #endif if (nativeImageAttributes != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDisposeImageAttributes(new HandleRef(this, nativeImageAttributes)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeImageAttributes = IntPtr.Zero; } } } ///. /// /// /// Cleans up Windows resources for this /// ~ImageAttributes() { Dispose(false); } ///. /// /// /// public object Clone() { IntPtr clone = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneImageAttributes( new HandleRef(this, nativeImageAttributes), out clone); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new ImageAttributes(clone); } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. void SetToIdentity() { SetToIdentity(ColorAdjustType.Default); } void SetToIdentity(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesToIdentity(new HandleRef(this, nativeImageAttributes), type); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } void Reset() { Reset(ColorAdjustType.Default); } void Reset(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipResetImageAttributes(new HandleRef(this, nativeImageAttributes), type); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } */ ////// Creates an exact copy of this ///. /// /// /// public void SetColorMatrix(ColorMatrix newColorMatrix) { SetColorMatrix(newColorMatrix, ColorMatrixFlag.Default, ColorAdjustType.Default); } ////// Sets the 5 X 5 color adjust matrix to the /// specified ///. /// /// /// public void SetColorMatrix(ColorMatrix newColorMatrix, ColorMatrixFlag flags) { SetColorMatrix(newColorMatrix, flags, ColorAdjustType.Default); } ////// Sets the 5 X 5 color adjust matrix to the specified 'Matrix' with the specified 'ColorMatrixFlags'. /// ////// /// public void SetColorMatrix(ColorMatrix newColorMatrix, ColorMatrixFlag mode, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorMatrix( new HandleRef(this, nativeImageAttributes), type, true, newColorMatrix, null, mode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Sets the 5 X 5 color adjust matrix to the specified 'Matrix' with the /// specified 'ColorMatrixFlags'. /// ////// /// Clears the color adjust matrix to all /// zeroes. /// public void ClearColorMatrix() { ClearColorMatrix(ColorAdjustType.Default); } ////// /// public void ClearColorMatrix(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorMatrix( new HandleRef(this, nativeImageAttributes), type, false, null, null, ColorMatrixFlag.Default); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Clears the color adjust matrix. /// ////// /// public void SetColorMatrices(ColorMatrix newColorMatrix, ColorMatrix grayMatrix) { SetColorMatrices(newColorMatrix, grayMatrix, ColorMatrixFlag.Default, ColorAdjustType.Default); } ////// Sets a color adjust matrix for image colors /// and a separate gray scale adjust matrix for gray scale values. /// ////// /// public void SetColorMatrices(ColorMatrix newColorMatrix, ColorMatrix grayMatrix, ColorMatrixFlag flags) { SetColorMatrices(newColorMatrix, grayMatrix, flags, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetColorMatrices(ColorMatrix newColorMatrix, ColorMatrix grayMatrix, ColorMatrixFlag mode, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorMatrix( new HandleRef(this, nativeImageAttributes), type, true, newColorMatrix, grayMatrix, mode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetThreshold(float threshold) { SetThreshold(threshold, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetThreshold(float threshold, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesThreshold( new HandleRef(this, nativeImageAttributes), type, true, threshold); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearThreshold() { ClearThreshold(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearThreshold(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesThreshold( new HandleRef(this, nativeImageAttributes), type, false, 0.0f); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetGamma(float gamma) { SetGamma(gamma, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetGamma(float gamma, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesGamma( new HandleRef(this, nativeImageAttributes), type, true, gamma); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearGamma() { ClearGamma(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearGamma(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesGamma( new HandleRef(this, nativeImageAttributes), type, false, 0.0f); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetNoOp() { SetNoOp(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetNoOp(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesNoOp( new HandleRef(this, nativeImageAttributes), type, true); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearNoOp() { ClearNoOp(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearNoOp(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesNoOp( new HandleRef(this, nativeImageAttributes), type, false); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetColorKey(Color colorLow, Color colorHigh) { SetColorKey(colorLow, colorHigh, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetColorKey(Color colorLow, Color colorHigh, ColorAdjustType type) { int lowInt = colorLow.ToArgb(); int highInt = colorHigh.ToArgb(); int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorKeys( new HandleRef(this, nativeImageAttributes), type, true, lowInt, highInt); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearColorKey() { ClearColorKey(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearColorKey(ColorAdjustType type) { int zero = 0; int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorKeys( new HandleRef(this, nativeImageAttributes), type, false, zero, zero); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetOutputChannel(ColorChannelFlag flags) { SetOutputChannel(flags, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetOutputChannel(ColorChannelFlag flags, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannel( new HandleRef(this, nativeImageAttributes), type, true, flags); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearOutputChannel() { ClearOutputChannel(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearOutputChannel(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannel( new HandleRef(this, nativeImageAttributes), type, false, ColorChannelFlag.ColorChannelLast); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetOutputChannelColorProfile(String colorProfileFilename) { SetOutputChannelColorProfile(colorProfileFilename, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetOutputChannelColorProfile(String colorProfileFilename, ColorAdjustType type) { IntSecurity.DemandReadFileIO(colorProfileFilename); int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannelColorProfile( new HandleRef(this, nativeImageAttributes), type, true, colorProfileFilename); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearOutputChannelColorProfile() { ClearOutputChannel(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearOutputChannelColorProfile(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannel( new HandleRef(this, nativeImageAttributes), type, false, ColorChannelFlag.ColorChannelLast); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetRemapTable(ColorMap[] map) { SetRemapTable(map, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetRemapTable(ColorMap[] map, ColorAdjustType type) { int index = 0; int mapSize = map.Length; int size = 4; // Marshal.SizeOf(index.GetType()); IntPtr memory = Marshal.AllocHGlobal(mapSize*size*2); try { for (index=0; index[To be supplied.] ////// /// public void ClearRemapTable() { ClearRemapTable(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearRemapTable(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesRemapTable( new HandleRef(this, nativeImageAttributes), type, false, 0, NativeMethods.NullHandleRef); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetBrushRemapTable(ColorMap[] map) { SetRemapTable(map, ColorAdjustType.Brush); } ///[To be supplied.] ////// /// public void ClearBrushRemapTable() { ClearRemapTable(ColorAdjustType.Brush); } ///[To be supplied.] ////// /// public void SetWrapMode(WrapMode mode) { SetWrapMode(mode, new Color(), false); } ///[To be supplied.] ////// /// public void SetWrapMode(WrapMode mode, Color color) { SetWrapMode(mode, color, false); } ///[To be supplied.] ////// /// public void SetWrapMode(WrapMode mode, Color color, bool clamp) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesWrapMode( new HandleRef(this, nativeImageAttributes), (int)mode, color.ToArgb(), clamp); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void GetAdjustedPalette(ColorPalette palette, ColorAdjustType type) { // does inplace adjustment IntPtr memory = palette.ConvertToMemory(); try { int status = SafeNativeMethods.Gdip.GdipGetImageAttributesAdjustedPalette( new HandleRef(this, nativeImageAttributes), new HandleRef(null, memory), type); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } palette.ConvertFromMemory(memory); } finally { if(memory != IntPtr.Zero) { Marshal.FreeHGlobal(memory); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Drawing.Drawing2D; using System.Drawing.Internal; using System.Globalization; // sdkinc\GDIplusImageAttributes.h // There are 5 possible sets of color adjustments: // ColorAdjustDefault, // ColorAdjustBitmap, // ColorAdjustBrush, // ColorAdjustPen, // ColorAdjustText, // Bitmaps, Brushes, Pens, and Text will all use any color adjustments // that have been set into the default ImageAttributes until their own // color adjustments have been set. So as soon as any "Set" method is // called for Bitmaps, Brushes, Pens, or Text, then they start from // scratch with only the color adjustments that have been set for them. // Calling Reset removes any individual color adjustments for a type // and makes it revert back to using all the default color adjustments // (if any). The SetToIdentity method is a way to force a type to // have no color adjustments at all, regardless of what previous adjustments // have been set for the defaults or for that type. ////// /// Contains information about how image colors /// are manipulated during rendering. /// [StructLayout(LayoutKind.Sequential)] public sealed class ImageAttributes : ICloneable, IDisposable { #if FINALIZATION_WATCH private string allocationSite = Graphics.GetAllocationStack(); #endif /* * Handle to native image attributes object */ internal IntPtr nativeImageAttributes; internal void SetNativeImageAttributes(IntPtr handle) { if (handle == IntPtr.Zero) throw new ArgumentNullException("handle"); nativeImageAttributes = handle; } ////// /// Initializes a new instance of the public ImageAttributes() { IntPtr newImageAttributes = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateImageAttributes(out newImageAttributes); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeImageAttributes(newImageAttributes); } internal ImageAttributes(IntPtr newNativeImageAttributes) { SetNativeImageAttributes(newNativeImageAttributes); } ///class. /// /// /// Cleans up Windows resources for this /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { #if FINALIZATION_WATCH if (!disposing && nativeImageAttributes != IntPtr.Zero) Debug.WriteLine("**********************\nDisposed through finalization:\n" + allocationSite); #endif if (nativeImageAttributes != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDisposeImageAttributes(new HandleRef(this, nativeImageAttributes)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeImageAttributes = IntPtr.Zero; } } } ///. /// /// /// Cleans up Windows resources for this /// ~ImageAttributes() { Dispose(false); } ///. /// /// /// public object Clone() { IntPtr clone = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneImageAttributes( new HandleRef(this, nativeImageAttributes), out clone); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new ImageAttributes(clone); } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. void SetToIdentity() { SetToIdentity(ColorAdjustType.Default); } void SetToIdentity(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesToIdentity(new HandleRef(this, nativeImageAttributes), type); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } void Reset() { Reset(ColorAdjustType.Default); } void Reset(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipResetImageAttributes(new HandleRef(this, nativeImageAttributes), type); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } */ ////// Creates an exact copy of this ///. /// /// /// public void SetColorMatrix(ColorMatrix newColorMatrix) { SetColorMatrix(newColorMatrix, ColorMatrixFlag.Default, ColorAdjustType.Default); } ////// Sets the 5 X 5 color adjust matrix to the /// specified ///. /// /// /// public void SetColorMatrix(ColorMatrix newColorMatrix, ColorMatrixFlag flags) { SetColorMatrix(newColorMatrix, flags, ColorAdjustType.Default); } ////// Sets the 5 X 5 color adjust matrix to the specified 'Matrix' with the specified 'ColorMatrixFlags'. /// ////// /// public void SetColorMatrix(ColorMatrix newColorMatrix, ColorMatrixFlag mode, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorMatrix( new HandleRef(this, nativeImageAttributes), type, true, newColorMatrix, null, mode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Sets the 5 X 5 color adjust matrix to the specified 'Matrix' with the /// specified 'ColorMatrixFlags'. /// ////// /// Clears the color adjust matrix to all /// zeroes. /// public void ClearColorMatrix() { ClearColorMatrix(ColorAdjustType.Default); } ////// /// public void ClearColorMatrix(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorMatrix( new HandleRef(this, nativeImageAttributes), type, false, null, null, ColorMatrixFlag.Default); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Clears the color adjust matrix. /// ////// /// public void SetColorMatrices(ColorMatrix newColorMatrix, ColorMatrix grayMatrix) { SetColorMatrices(newColorMatrix, grayMatrix, ColorMatrixFlag.Default, ColorAdjustType.Default); } ////// Sets a color adjust matrix for image colors /// and a separate gray scale adjust matrix for gray scale values. /// ////// /// public void SetColorMatrices(ColorMatrix newColorMatrix, ColorMatrix grayMatrix, ColorMatrixFlag flags) { SetColorMatrices(newColorMatrix, grayMatrix, flags, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetColorMatrices(ColorMatrix newColorMatrix, ColorMatrix grayMatrix, ColorMatrixFlag mode, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorMatrix( new HandleRef(this, nativeImageAttributes), type, true, newColorMatrix, grayMatrix, mode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetThreshold(float threshold) { SetThreshold(threshold, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetThreshold(float threshold, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesThreshold( new HandleRef(this, nativeImageAttributes), type, true, threshold); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearThreshold() { ClearThreshold(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearThreshold(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesThreshold( new HandleRef(this, nativeImageAttributes), type, false, 0.0f); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetGamma(float gamma) { SetGamma(gamma, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetGamma(float gamma, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesGamma( new HandleRef(this, nativeImageAttributes), type, true, gamma); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearGamma() { ClearGamma(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearGamma(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesGamma( new HandleRef(this, nativeImageAttributes), type, false, 0.0f); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetNoOp() { SetNoOp(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetNoOp(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesNoOp( new HandleRef(this, nativeImageAttributes), type, true); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearNoOp() { ClearNoOp(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearNoOp(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesNoOp( new HandleRef(this, nativeImageAttributes), type, false); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetColorKey(Color colorLow, Color colorHigh) { SetColorKey(colorLow, colorHigh, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetColorKey(Color colorLow, Color colorHigh, ColorAdjustType type) { int lowInt = colorLow.ToArgb(); int highInt = colorHigh.ToArgb(); int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorKeys( new HandleRef(this, nativeImageAttributes), type, true, lowInt, highInt); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearColorKey() { ClearColorKey(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearColorKey(ColorAdjustType type) { int zero = 0; int status = SafeNativeMethods.Gdip.GdipSetImageAttributesColorKeys( new HandleRef(this, nativeImageAttributes), type, false, zero, zero); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetOutputChannel(ColorChannelFlag flags) { SetOutputChannel(flags, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetOutputChannel(ColorChannelFlag flags, ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannel( new HandleRef(this, nativeImageAttributes), type, true, flags); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearOutputChannel() { ClearOutputChannel(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearOutputChannel(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannel( new HandleRef(this, nativeImageAttributes), type, false, ColorChannelFlag.ColorChannelLast); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetOutputChannelColorProfile(String colorProfileFilename) { SetOutputChannelColorProfile(colorProfileFilename, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetOutputChannelColorProfile(String colorProfileFilename, ColorAdjustType type) { IntSecurity.DemandReadFileIO(colorProfileFilename); int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannelColorProfile( new HandleRef(this, nativeImageAttributes), type, true, colorProfileFilename); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void ClearOutputChannelColorProfile() { ClearOutputChannel(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearOutputChannelColorProfile(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesOutputChannel( new HandleRef(this, nativeImageAttributes), type, false, ColorChannelFlag.ColorChannelLast); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetRemapTable(ColorMap[] map) { SetRemapTable(map, ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void SetRemapTable(ColorMap[] map, ColorAdjustType type) { int index = 0; int mapSize = map.Length; int size = 4; // Marshal.SizeOf(index.GetType()); IntPtr memory = Marshal.AllocHGlobal(mapSize*size*2); try { for (index=0; index[To be supplied.] ////// /// public void ClearRemapTable() { ClearRemapTable(ColorAdjustType.Default); } ///[To be supplied.] ////// /// public void ClearRemapTable(ColorAdjustType type) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesRemapTable( new HandleRef(this, nativeImageAttributes), type, false, 0, NativeMethods.NullHandleRef); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void SetBrushRemapTable(ColorMap[] map) { SetRemapTable(map, ColorAdjustType.Brush); } ///[To be supplied.] ////// /// public void ClearBrushRemapTable() { ClearRemapTable(ColorAdjustType.Brush); } ///[To be supplied.] ////// /// public void SetWrapMode(WrapMode mode) { SetWrapMode(mode, new Color(), false); } ///[To be supplied.] ////// /// public void SetWrapMode(WrapMode mode, Color color) { SetWrapMode(mode, color, false); } ///[To be supplied.] ////// /// public void SetWrapMode(WrapMode mode, Color color, bool clamp) { int status = SafeNativeMethods.Gdip.GdipSetImageAttributesWrapMode( new HandleRef(this, nativeImageAttributes), (int)mode, color.ToArgb(), clamp); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public void GetAdjustedPalette(ColorPalette palette, ColorAdjustType type) { // does inplace adjustment IntPtr memory = palette.ConvertToMemory(); try { int status = SafeNativeMethods.Gdip.GdipGetImageAttributesAdjustedPalette( new HandleRef(this, nativeImageAttributes), new HandleRef(null, memory), type); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } palette.ConvertFromMemory(memory); } finally { if(memory != IntPtr.Zero) { Marshal.FreeHGlobal(memory); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
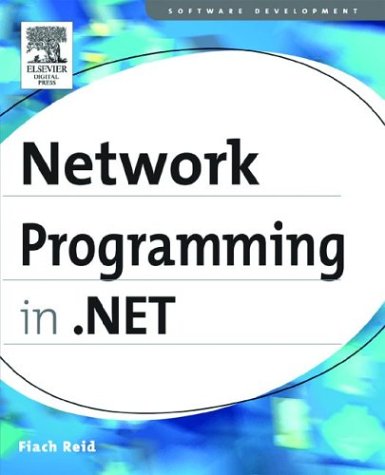
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeometryDrawing.cs
- XappLauncher.cs
- Int32CollectionConverter.cs
- VersionValidator.cs
- InsufficientMemoryException.cs
- TableColumnCollectionInternal.cs
- ValidationEventArgs.cs
- JsonStringDataContract.cs
- SizeF.cs
- LicenseProviderAttribute.cs
- NullableLongAverageAggregationOperator.cs
- _NegoStream.cs
- IdentityValidationException.cs
- TemplateXamlParser.cs
- SineEase.cs
- RankException.cs
- ExternalException.cs
- Popup.cs
- ElementUtil.cs
- PenCursorManager.cs
- EqualityComparer.cs
- RectAnimationClockResource.cs
- ObjectParameter.cs
- ActiveXHost.cs
- StrongTypingException.cs
- ListControl.cs
- PropertyDescriptor.cs
- EntityContainerEntitySet.cs
- CodeExporter.cs
- assemblycache.cs
- ContourSegment.cs
- BitVector32.cs
- SimpleRecyclingCache.cs
- EntityContainerEntitySet.cs
- SmtpNetworkElement.cs
- XmlAttributeCache.cs
- CompilerResults.cs
- ContainerUtilities.cs
- ResourcePermissionBase.cs
- FrameDimension.cs
- CustomWebEventKey.cs
- ChooseAction.cs
- XmlObjectSerializer.cs
- HierarchicalDataSourceControl.cs
- SelectedDatesCollection.cs
- UnknownWrapper.cs
- DataSourceControlBuilder.cs
- StagingAreaInputItem.cs
- StringStorage.cs
- FreezableDefaultValueFactory.cs
- VirtualPathExtension.cs
- BuildManagerHost.cs
- DesignerOptionService.cs
- TextSelectionProcessor.cs
- ProxyElement.cs
- XPathConvert.cs
- BitmapEffect.cs
- PageCatalogPart.cs
- TextEditorThreadLocalStore.cs
- WizardStepBase.cs
- AppDomainShutdownMonitor.cs
- ReflectionUtil.cs
- PasswordRecoveryDesigner.cs
- TextEditorTables.cs
- LongPath.cs
- ToolTipService.cs
- ArrayTypeMismatchException.cs
- LinearGradientBrush.cs
- SqlOuterApplyReducer.cs
- ActivityTypeDesigner.xaml.cs
- DefaultHttpHandler.cs
- DataGridTextBoxColumn.cs
- Select.cs
- ConnectionStringSettings.cs
- ToolStripSeparatorRenderEventArgs.cs
- Font.cs
- ToolStripMenuItem.cs
- HTMLTagNameToTypeMapper.cs
- SpeechRecognizer.cs
- ValuePattern.cs
- StringSource.cs
- OdbcInfoMessageEvent.cs
- XmlSchemaInfo.cs
- IndexingContentUnit.cs
- InfiniteTimeSpanConverter.cs
- AppDomainShutdownMonitor.cs
- TemplateBindingExtension.cs
- SynchronizedDispatch.cs
- FindProgressChangedEventArgs.cs
- GlobalizationAssembly.cs
- ReadonlyMessageFilter.cs
- XamlFilter.cs
- ToolboxItemSnapLineBehavior.cs
- UpdateCommandGenerator.cs
- DebugView.cs
- EntityTypeEmitter.cs
- COM2ComponentEditor.cs
- MonitorWrapper.cs
- FileVersion.cs
- DomainUpDown.cs