Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / loginstatus.cs / 2 / loginstatus.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Security.Permissions; using System.Web.Security; using System.Web.UI; ////// Displays a link or button that allows the user to login or logout of the site. /// Shows whether the user is currently logged in. /// [ Bindable(false), DefaultEvent("LoggingOut"), Designer("System.Web.UI.Design.WebControls.LoginStatusDesigner, " + AssemblyRef.SystemDesign), ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class LoginStatus : CompositeControl { private static readonly object EventLoggingOut = new object(); private static readonly object EventLoggedOut = new object(); private LinkButton _logInLinkButton; private ImageButton _logInImageButton; private LinkButton _logOutLinkButton; private ImageButton _logOutImageButton; private bool _loggedIn; ////// Whether a user is currently logged in. /// private bool LoggedIn { get { return _loggedIn; } set { _loggedIn = value; } } /// /// The URL of the image to be shown for the login button. /// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.LoginStatus_LoginImageUrl), Editor("System.Web.UI.Design.ImageUrlEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), UrlProperty() ] public virtual string LoginImageUrl { get { object obj = ViewState["LoginImageUrl"]; return (obj == null) ? String.Empty : (string) obj; } set { ViewState["LoginImageUrl"] = value; } } ////// The text to be shown for the login button. /// [ Localizable(true), WebCategory("Appearance"), WebSysDefaultValue(SR.LoginStatus_DefaultLoginText), WebSysDescription(SR.LoginStatus_LoginText) ] public virtual string LoginText { get { object obj = ViewState["LoginText"]; return (obj == null) ? SR.GetString(SR.LoginStatus_DefaultLoginText) : (string) obj; } set { ViewState["LoginText"] = value; } } ////// The action to perform after logging out. /// [ WebCategory("Behavior"), DefaultValue(LogoutAction.Refresh), Themeable(false), WebSysDescription(SR.LoginStatus_LogoutAction) ] public virtual LogoutAction LogoutAction { get { object obj = ViewState["LogoutAction"]; return (obj == null) ? LogoutAction.Refresh : (LogoutAction) obj; } set { if (value < LogoutAction.Refresh || value > LogoutAction.RedirectToLoginPage) { throw new ArgumentOutOfRangeException("value"); } ViewState["LogoutAction"] = value; } } ////// The URL of the image to be shown for the logout button. /// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.LoginStatus_LogoutImageUrl), Editor("System.Web.UI.Design.ImageUrlEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), UrlProperty() ] public virtual string LogoutImageUrl { get { object obj = ViewState["LogoutImageUrl"]; return (obj == null) ? String.Empty : (string) obj; } set { ViewState["LogoutImageUrl"] = value; } } ////// The URL redirected to after logging out. /// [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.LoginStatus_LogoutPageUrl), Editor("System.Web.UI.Design.UrlEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), Themeable(false), UrlProperty() ] public virtual string LogoutPageUrl { get { object obj = ViewState["LogoutPageUrl"]; return (obj == null) ? String.Empty : (string) obj; } set { ViewState["LogoutPageUrl"] = value; } } ////// The text to be shown for the logout button. /// [ Localizable(true), WebCategory("Appearance"), WebSysDefaultValue(SR.LoginStatus_DefaultLogoutText), WebSysDescription(SR.LoginStatus_LogoutText) ] public virtual string LogoutText { get { object obj = ViewState["LogoutText"]; return (obj == null) ? SR.GetString(SR.LoginStatus_DefaultLogoutText) : (string) obj; } set { ViewState["LogoutText"] = value; } } private string NavigateUrl { get { if (!DesignMode) { return FormsAuthentication.GetLoginPage(null, true); } // For the designer to render a hyperlink return "url"; } } protected override HtmlTextWriterTag TagKey { get { return HtmlTextWriterTag.A; } } ////// Raised after the user is logged out. /// [ WebCategory("Action"), WebSysDescription(SR.LoginStatus_LoggedOut) ] public event EventHandler LoggedOut { add { Events.AddHandler(EventLoggedOut, value); } remove { Events.RemoveHandler(EventLoggedOut, value); } } ////// Raised before the user is logged out. /// [ WebCategory("Action"), WebSysDescription(SR.LoginStatus_LoggingOut) ] public event LoginCancelEventHandler LoggingOut { add { Events.AddHandler(EventLoggingOut, value); } remove { Events.RemoveHandler(EventLoggingOut, value); } } ////// Creates all the child controls that may be rendered. /// protected internal override void CreateChildControls() { Controls.Clear(); _logInLinkButton = new LinkButton(); _logInImageButton = new ImageButton(); _logOutLinkButton = new LinkButton(); _logOutImageButton = new ImageButton(); _logInLinkButton.EnableViewState = false; _logInImageButton.EnableViewState = false; _logOutLinkButton.EnableViewState = false; _logOutImageButton.EnableViewState = false; // Disable theming of child controls ( _logInLinkButton.EnableTheming = false; _logInImageButton.EnableTheming = false; _logInLinkButton.CausesValidation = false; _logInImageButton.CausesValidation = false; _logOutLinkButton.EnableTheming = false; _logOutImageButton.EnableTheming = false; _logOutLinkButton.CausesValidation = false; _logOutImageButton.CausesValidation = false; CommandEventHandler handler = new CommandEventHandler(LogoutClicked); _logOutLinkButton.Command += handler; _logOutImageButton.Command += handler; handler = new CommandEventHandler(LoginClicked); _logInLinkButton.Command += handler; _logInImageButton.Command += handler; Controls.Add(_logOutLinkButton); Controls.Add(_logOutImageButton); Controls.Add(_logInLinkButton); Controls.Add(_logInImageButton); } ////// Logs out and redirects the user when the logout button is clicked. /// private void LogoutClicked(object Source, CommandEventArgs e) { LoginCancelEventArgs cancelEventArgs = new LoginCancelEventArgs(); OnLoggingOut(cancelEventArgs); if (cancelEventArgs.Cancel) { return; } FormsAuthentication.SignOut(); // BugBug: revert to old behavior after SignOut. Page.Response.Clear(); Page.Response.StatusCode = 200; OnLoggedOut(EventArgs.Empty); // Redirect the user as appropriate switch (LogoutAction) { case LogoutAction.RedirectToLoginPage: // We do not want the ReturnUrl in the query string, since this is an information // disclosure. So we must use this instead of FormsAuthentication.RedirectToLoginPage(). // ( Page.Response.Redirect(FormsAuthentication.LoginUrl, false); break; case LogoutAction.Refresh: // If the form method is GET, then we must not include the query string, since // it will cause an infinite redirect loop. If the form method is POST (or there // is no form), then we must include the query string, since the developer could // be using the query string to drive the logic of their page. ( if (Page.Form != null && String.Equals(Page.Form.Method, "get", StringComparison.OrdinalIgnoreCase)) { Page.Response.Redirect(Page.Request.Path, false); } else { Page.Response.Redirect(Page.Request.PathWithQueryString, false); } break; case LogoutAction.Redirect: string url = LogoutPageUrl; if (!String.IsNullOrEmpty(url)) { url = ResolveClientUrl(url); } else { // Use FormsAuthentication.LoginUrl as a fallback url = FormsAuthentication.LoginUrl; } Page.Response.Redirect(url, false); break; } } private void LoginClicked(object Source, CommandEventArgs e) { Page.Response.Redirect(ResolveClientUrl(NavigateUrl), false); } ////// Raises the LoggedOut event. /// protected virtual void OnLoggedOut(EventArgs e) { EventHandler handler = (EventHandler)Events[EventLoggedOut]; if (handler != null) { handler(this, e); } } ////// Raises the LoggingOut event. /// protected virtual void OnLoggingOut(LoginCancelEventArgs e) { LoginCancelEventHandler handler = (LoginCancelEventHandler)Events[EventLoggingOut]; if (handler != null) { handler(this, e); } } ////// Determines whether a user is logged in, and gets the URL of the login page. /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); // Must be set in PreRender instead of Render, because Page.Request.IsAuthenticated is not // valid at design time. LoggedIn = Page.Request.IsAuthenticated; } protected internal override void Render(HtmlTextWriter writer) { RenderContents(writer); } protected internal override void RenderContents(HtmlTextWriter writer) { if (Page != null) { Page.VerifyRenderingInServerForm(this); } SetChildProperties(); if ((ID != null) && (ID.Length != 0)) { // writer.AddAttribute(HtmlTextWriterAttribute.Id, ClientID); } base.RenderContents(writer); } ////// Sets the visiblity, style, and other properties of child controls. /// private void SetChildProperties() { EnsureChildControls(); // Set all buttons to nonvisible, then later set the selected button to visible _logInLinkButton.Visible = false; _logInImageButton.Visible = false; _logOutLinkButton.Visible = false; _logOutImageButton.Visible = false; WebControl visibleControl = null; bool loggedIn = LoggedIn; if (loggedIn) { string logoutImageUrl = LogoutImageUrl; if (logoutImageUrl.Length > 0) { _logOutImageButton.AlternateText = LogoutText; _logOutImageButton.ImageUrl = logoutImageUrl; visibleControl = _logOutImageButton; } else { _logOutLinkButton.Text = LogoutText; visibleControl = _logOutLinkButton; } } else { string loginImageUrl = LoginImageUrl; if (loginImageUrl.Length > 0) { _logInImageButton.AlternateText = LoginText; _logInImageButton.ImageUrl = loginImageUrl; visibleControl = _logInImageButton; } else { _logInLinkButton.Text = LoginText; visibleControl = _logInLinkButton; } } visibleControl.CopyBaseAttributes(this); visibleControl.ApplyStyle(ControlStyle); visibleControl.Visible = true; } ////// /// Allows the designer to set the LoggedIn and NavigateUrl properties for proper rendering in the designer. /// [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] protected override void SetDesignModeState(IDictionary data) { if (data != null) { object o = data["LoggedIn"]; if (o != null) { LoggedIn = (bool)o; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
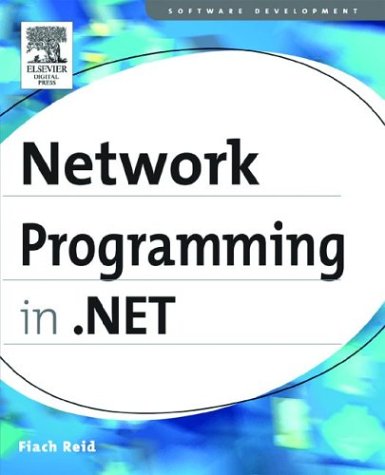
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataErrorValidationRule.cs
- SerializationObjectManager.cs
- PageRouteHandler.cs
- TimeSpanSecondsConverter.cs
- TogglePattern.cs
- OletxVolatileEnlistment.cs
- EdmEntityTypeAttribute.cs
- ColorMatrix.cs
- SqlFormatter.cs
- UnsafeNativeMethods.cs
- DataGridViewCellCancelEventArgs.cs
- FontStyles.cs
- Int16KeyFrameCollection.cs
- SiteMapNodeItemEventArgs.cs
- ListItemCollection.cs
- RemotingConfigParser.cs
- ReferenceList.cs
- StateDesigner.cs
- IntSecurity.cs
- COSERVERINFO.cs
- Event.cs
- LayoutInformation.cs
- HostingEnvironmentSection.cs
- CustomError.cs
- DllNotFoundException.cs
- DataGridViewCellFormattingEventArgs.cs
- DelayedRegex.cs
- ChtmlTextWriter.cs
- TextEffect.cs
- EntityDataSourceContainerNameItem.cs
- BindableTemplateBuilder.cs
- LoginAutoFormat.cs
- initElementDictionary.cs
- XpsInterleavingPolicy.cs
- GridErrorDlg.cs
- WebExceptionStatus.cs
- Transform3DCollection.cs
- SoapClientProtocol.cs
- COAUTHINFO.cs
- ChannelDispatcherBase.cs
- XmlNamedNodeMap.cs
- ResourceWriter.cs
- ColorDialog.cs
- UnsafeCollabNativeMethods.cs
- SourceElementsCollection.cs
- FilterableAttribute.cs
- ProcessInfo.cs
- DataObjectFieldAttribute.cs
- FlowDocumentPaginator.cs
- MenuTracker.cs
- PerspectiveCamera.cs
- XmlSchemaSimpleTypeRestriction.cs
- EnumerableRowCollectionExtensions.cs
- FileUpload.cs
- HttpWrapper.cs
- HMACMD5.cs
- WorkflowMarkupSerializationException.cs
- TraversalRequest.cs
- PopOutPanel.cs
- DataControlPagerLinkButton.cs
- _ProxyRegBlob.cs
- Normalizer.cs
- _SslState.cs
- AtomPub10ServiceDocumentFormatter.cs
- DbDataAdapter.cs
- PreservationFileWriter.cs
- XmlWhitespace.cs
- NamedPipeAppDomainProtocolHandler.cs
- XmlSchemaInferenceException.cs
- DbTransaction.cs
- SafeRightsManagementSessionHandle.cs
- InvalidWMPVersionException.cs
- XNameConverter.cs
- Types.cs
- PropertyValueChangedEvent.cs
- Image.cs
- ControlPaint.cs
- XPathNode.cs
- ModelItemDictionary.cs
- Version.cs
- nulltextnavigator.cs
- SerializationStore.cs
- EntitySetBase.cs
- _Win32.cs
- FormView.cs
- QuaternionAnimationBase.cs
- KeyGesture.cs
- Converter.cs
- CompilerState.cs
- ConfigurationLoader.cs
- FloaterBaseParaClient.cs
- TextRangeBase.cs
- Pkcs9Attribute.cs
- TextTreeRootNode.cs
- DesignerHelpers.cs
- TreeSet.cs
- SkipQueryOptionExpression.cs
- InternalTransaction.cs
- TextElementEnumerator.cs
- ApplicationServicesHostFactory.cs