Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / DrawListViewColumnHeaderEventArgs.cs / 1 / DrawListViewColumnHeaderEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; ////// /// This class contains the information a user needs to paint ListView column header (Details view only). /// public class DrawListViewColumnHeaderEventArgs : EventArgs { private readonly Graphics graphics; private readonly Rectangle bounds; private readonly int columnIndex; private readonly ColumnHeader header; private readonly ListViewItemStates state; private readonly Color foreColor; private readonly Color backColor; private readonly Font font; private bool drawDefault; ////// /// Creates a new DrawListViewColumnHeaderEventArgs with the given parameters. /// public DrawListViewColumnHeaderEventArgs(Graphics graphics, Rectangle bounds, int columnIndex, ColumnHeader header, ListViewItemStates state, Color foreColor, Color backColor, Font font) { this.graphics = graphics; this.bounds = bounds; this.columnIndex = columnIndex; this.header = header; this.state = state; this.foreColor = foreColor; this.backColor = backColor; this.font = font; } ////// /// Causes the item do be drawn by the system instead of owner drawn. /// public bool DrawDefault { get { return drawDefault; } set { drawDefault = value; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The index of this column. /// public int ColumnIndex { get { return columnIndex; } } ////// /// The header object. /// public ColumnHeader Header { get { return header; } } ////// /// State information pertaining to the header. /// public ListViewItemStates State { get { return state; } } ////// /// Color used to draw the header's text. /// public Color ForeColor { get { return foreColor; } } ////// /// Color used to draw the header's background. /// public Color BackColor { get { return backColor; } } ////// /// Font used to render the header's text. /// public Font Font { get { return font; } } ////// /// Draws the header's background. /// public void DrawBackground() { if (Application.RenderWithVisualStyles) { VisualStyleRenderer vsr = new VisualStyleRenderer(VisualStyleElement.Header.Item.Normal); vsr.DrawBackground(graphics, bounds); } else { using (Brush backBrush = new SolidBrush(backColor)) { graphics.FillRectangle(backBrush, bounds); } // draw the 3d header // Rectangle r = this.bounds; r.Width -= 1; r.Height -= 1; // draw the dark border around the whole thing // graphics.DrawRectangle(SystemPens.ControlDarkDark, r); r.Width -= 1; r.Height -= 1; // draw the light 3D border // graphics.DrawLine(SystemPens.ControlLightLight, r.X, r.Y, r.Right, r.Y); graphics.DrawLine(SystemPens.ControlLightLight, r.X, r.Y, r.X, r.Bottom); // draw the dark 3D Border // graphics.DrawLine(SystemPens.ControlDark, r.X + 1, r.Bottom, r.Right, r.Bottom); graphics.DrawLine(SystemPens.ControlDark, r.Right, r.Y + 1, r.Right, r.Bottom); } } ////// /// Draws the header's text (overloaded) /// public void DrawText() { HorizontalAlignment hAlign = header.TextAlign; TextFormatFlags flags = (hAlign == HorizontalAlignment.Left) ? TextFormatFlags.Left : ((hAlign == HorizontalAlignment.Center) ? TextFormatFlags.HorizontalCenter : TextFormatFlags.Right); flags |= TextFormatFlags.WordEllipsis; DrawText(flags); } ////// /// Draws the header's text (overloaded) - takes a TextFormatFlags argument. /// [ SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters") // We want to measure the size of blank space. // So we don't have to localize it. ] public void DrawText(TextFormatFlags flags) { string text = header.Text; int padding = TextRenderer.MeasureText(" ", font).Width; Rectangle newBounds = Rectangle.Inflate(bounds, -padding, 0); TextRenderer.DrawText(graphics, text, font, newBounds, foreColor, flags); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; ////// /// This class contains the information a user needs to paint ListView column header (Details view only). /// public class DrawListViewColumnHeaderEventArgs : EventArgs { private readonly Graphics graphics; private readonly Rectangle bounds; private readonly int columnIndex; private readonly ColumnHeader header; private readonly ListViewItemStates state; private readonly Color foreColor; private readonly Color backColor; private readonly Font font; private bool drawDefault; ////// /// Creates a new DrawListViewColumnHeaderEventArgs with the given parameters. /// public DrawListViewColumnHeaderEventArgs(Graphics graphics, Rectangle bounds, int columnIndex, ColumnHeader header, ListViewItemStates state, Color foreColor, Color backColor, Font font) { this.graphics = graphics; this.bounds = bounds; this.columnIndex = columnIndex; this.header = header; this.state = state; this.foreColor = foreColor; this.backColor = backColor; this.font = font; } ////// /// Causes the item do be drawn by the system instead of owner drawn. /// public bool DrawDefault { get { return drawDefault; } set { drawDefault = value; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The index of this column. /// public int ColumnIndex { get { return columnIndex; } } ////// /// The header object. /// public ColumnHeader Header { get { return header; } } ////// /// State information pertaining to the header. /// public ListViewItemStates State { get { return state; } } ////// /// Color used to draw the header's text. /// public Color ForeColor { get { return foreColor; } } ////// /// Color used to draw the header's background. /// public Color BackColor { get { return backColor; } } ////// /// Font used to render the header's text. /// public Font Font { get { return font; } } ////// /// Draws the header's background. /// public void DrawBackground() { if (Application.RenderWithVisualStyles) { VisualStyleRenderer vsr = new VisualStyleRenderer(VisualStyleElement.Header.Item.Normal); vsr.DrawBackground(graphics, bounds); } else { using (Brush backBrush = new SolidBrush(backColor)) { graphics.FillRectangle(backBrush, bounds); } // draw the 3d header // Rectangle r = this.bounds; r.Width -= 1; r.Height -= 1; // draw the dark border around the whole thing // graphics.DrawRectangle(SystemPens.ControlDarkDark, r); r.Width -= 1; r.Height -= 1; // draw the light 3D border // graphics.DrawLine(SystemPens.ControlLightLight, r.X, r.Y, r.Right, r.Y); graphics.DrawLine(SystemPens.ControlLightLight, r.X, r.Y, r.X, r.Bottom); // draw the dark 3D Border // graphics.DrawLine(SystemPens.ControlDark, r.X + 1, r.Bottom, r.Right, r.Bottom); graphics.DrawLine(SystemPens.ControlDark, r.Right, r.Y + 1, r.Right, r.Bottom); } } ////// /// Draws the header's text (overloaded) /// public void DrawText() { HorizontalAlignment hAlign = header.TextAlign; TextFormatFlags flags = (hAlign == HorizontalAlignment.Left) ? TextFormatFlags.Left : ((hAlign == HorizontalAlignment.Center) ? TextFormatFlags.HorizontalCenter : TextFormatFlags.Right); flags |= TextFormatFlags.WordEllipsis; DrawText(flags); } ////// /// Draws the header's text (overloaded) - takes a TextFormatFlags argument. /// [ SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters") // We want to measure the size of blank space. // So we don't have to localize it. ] public void DrawText(TextFormatFlags flags) { string text = header.Text; int padding = TextRenderer.MeasureText(" ", font).Width; Rectangle newBounds = Rectangle.Inflate(bounds, -padding, 0); TextRenderer.DrawText(graphics, text, font, newBounds, foreColor, flags); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
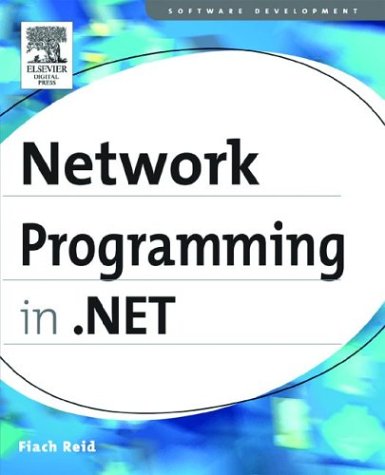
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DashStyle.cs
- RuntimeResourceSet.cs
- HttpApplication.cs
- MessageQueue.cs
- OdbcErrorCollection.cs
- BitmapEffect.cs
- securitycriticaldataClass.cs
- Nodes.cs
- XmlStringTable.cs
- ThemeInfoAttribute.cs
- streamingZipPartStream.cs
- CoTaskMemUnicodeSafeHandle.cs
- TraceSection.cs
- MaskDescriptors.cs
- RegexCharClass.cs
- DataGridTextBoxColumn.cs
- DynamicEntity.cs
- TimeSpan.cs
- ReflectPropertyDescriptor.cs
- PackWebRequestFactory.cs
- InvalidDataException.cs
- DataServiceProcessingPipelineEventArgs.cs
- LiteralLink.cs
- BuildManager.cs
- TableItemPatternIdentifiers.cs
- XmlSchemaSet.cs
- XPathDescendantIterator.cs
- PersistenceTypeAttribute.cs
- PeerCredential.cs
- COM2PropertyDescriptor.cs
- DoubleUtil.cs
- ObjectQueryProvider.cs
- DocumentApplicationJournalEntry.cs
- ResourcePool.cs
- FacetEnabledSchemaElement.cs
- PropertyEmitterBase.cs
- XmlCodeExporter.cs
- SortedList.cs
- DataGridColumnReorderingEventArgs.cs
- TemplateColumn.cs
- AliasedSlot.cs
- GeneralTransform2DTo3D.cs
- WmlCommandAdapter.cs
- QilNode.cs
- TemplatePartAttribute.cs
- _ProxyRegBlob.cs
- TextViewSelectionProcessor.cs
- MarkupCompilePass2.cs
- SpecularMaterial.cs
- DesignTimeVisibleAttribute.cs
- EpmCustomContentSerializer.cs
- ComponentChangingEvent.cs
- SafeThreadHandle.cs
- PagerSettings.cs
- WindowsStatusBar.cs
- ToolStripDropDownButton.cs
- SessionSwitchEventArgs.cs
- ListBindingHelper.cs
- ImageCodecInfo.cs
- StateManagedCollection.cs
- RootBrowserWindowAutomationPeer.cs
- RawKeyboardInputReport.cs
- LocalizationComments.cs
- ConnectionPoint.cs
- XmlChildEnumerator.cs
- WindowsToolbarItemAsMenuItem.cs
- ScrollItemProviderWrapper.cs
- SqlNode.cs
- ElementsClipboardData.cs
- BitHelper.cs
- WinInetCache.cs
- FormViewInsertedEventArgs.cs
- CompilationRelaxations.cs
- DBSchemaTable.cs
- UnsafeNativeMethods.cs
- TypeSchema.cs
- ImageField.cs
- MultiPropertyDescriptorGridEntry.cs
- InkCanvasFeedbackAdorner.cs
- PerfCounters.cs
- EventLogPermissionAttribute.cs
- BindingCollection.cs
- RegexWorker.cs
- TypeListConverter.cs
- NonParentingControl.cs
- ContractListAdapter.cs
- SafeBitVector32.cs
- ByteRangeDownloader.cs
- SqlClientWrapperSmiStream.cs
- StringDictionary.cs
- DynamicMethod.cs
- xmlNames.cs
- RegexCompilationInfo.cs
- AssemblyCollection.cs
- updateconfighost.cs
- clipboard.cs
- UnicodeEncoding.cs
- Pen.cs
- CodeTypeOfExpression.cs
- ColorKeyFrameCollection.cs