Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Data / BindingCollection.cs / 1 / BindingCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines BindingList object, a list of binds. // // Specs: http://avalon/connecteddata/M5%20Specs/UIBind.mht // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Windows; using System.Windows.Markup; using System.Windows.Data; using MS.Utility; using MS.Internal; namespace MS.Internal.Data { ////// A list of bindingss, used by MultiBinding classes. /// internal class BindingCollection : Collection{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- /// Constructor internal BindingCollection(BindingBase owner, BindingCollectionChangedCallback callback) { Invariant.Assert(owner != null && callback != null); _owner = owner; _collectionChangedCallback = callback; } // disable default constructor private BindingCollection() { } //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { _owner.CheckSealed(); base.ClearItems(); OnBindingCollectionChanged(); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { _owner.CheckSealed(); base.RemoveItem(index); OnBindingCollectionChanged(); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, BindingBase item) { if (item == null) throw new ArgumentNullException("item"); ValidateItem(item); _owner.CheckSealed(); base.InsertItem(index, item); OnBindingCollectionChanged(); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, BindingBase item) { if (item == null) throw new ArgumentNullException("item"); ValidateItem(item); _owner.CheckSealed(); base.SetItem(index, item); OnBindingCollectionChanged(); } #endregion Protected Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ void ValidateItem(BindingBase binding) { // for V1, we only allow Binding as an item of BindingCollection. if (!(binding is Binding)) throw new NotSupportedException(SR.Get(SRID.BindingCollectionContainsNonBinding, binding.GetType().Name)); } void OnBindingCollectionChanged() { if (_collectionChangedCallback != null) _collectionChangedCallback(); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ BindingBase _owner; private BindingCollectionChangedCallback _collectionChangedCallback; } // the delegate to use for getting BindingListChanged notifications internal delegate void BindingCollectionChangedCallback(); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines BindingList object, a list of binds. // // Specs: http://avalon/connecteddata/M5%20Specs/UIBind.mht // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Windows; using System.Windows.Markup; using System.Windows.Data; using MS.Utility; using MS.Internal; namespace MS.Internal.Data { ////// A list of bindingss, used by MultiBinding classes. /// internal class BindingCollection : Collection{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- /// Constructor internal BindingCollection(BindingBase owner, BindingCollectionChangedCallback callback) { Invariant.Assert(owner != null && callback != null); _owner = owner; _collectionChangedCallback = callback; } // disable default constructor private BindingCollection() { } //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { _owner.CheckSealed(); base.ClearItems(); OnBindingCollectionChanged(); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { _owner.CheckSealed(); base.RemoveItem(index); OnBindingCollectionChanged(); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, BindingBase item) { if (item == null) throw new ArgumentNullException("item"); ValidateItem(item); _owner.CheckSealed(); base.InsertItem(index, item); OnBindingCollectionChanged(); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, BindingBase item) { if (item == null) throw new ArgumentNullException("item"); ValidateItem(item); _owner.CheckSealed(); base.SetItem(index, item); OnBindingCollectionChanged(); } #endregion Protected Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ void ValidateItem(BindingBase binding) { // for V1, we only allow Binding as an item of BindingCollection. if (!(binding is Binding)) throw new NotSupportedException(SR.Get(SRID.BindingCollectionContainsNonBinding, binding.GetType().Name)); } void OnBindingCollectionChanged() { if (_collectionChangedCallback != null) _collectionChangedCallback(); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ BindingBase _owner; private BindingCollectionChangedCallback _collectionChangedCallback; } // the delegate to use for getting BindingListChanged notifications internal delegate void BindingCollectionChangedCallback(); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
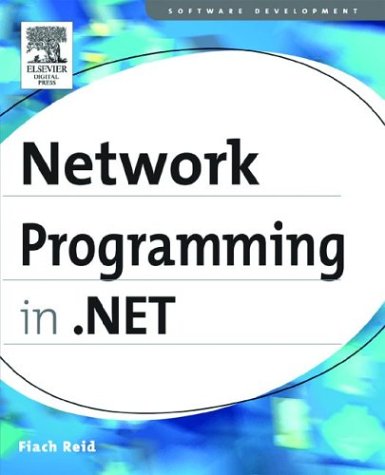
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlCharacterData.cs
- FunctionUpdateCommand.cs
- AppDomainAttributes.cs
- StreamInfo.cs
- HtmlDocument.cs
- SHA1Managed.cs
- WizardForm.cs
- PathGradientBrush.cs
- SBCSCodePageEncoding.cs
- DataServiceResponse.cs
- PngBitmapDecoder.cs
- StateItem.cs
- linebase.cs
- PlaceHolder.cs
- HtmlTable.cs
- _emptywebproxy.cs
- ImagingCache.cs
- ExpressionBuilder.cs
- MethodBody.cs
- RewritingValidator.cs
- BooleanSwitch.cs
- RelationshipFixer.cs
- ContentOnlyMessage.cs
- TypeDependencyAttribute.cs
- IResourceProvider.cs
- RoleService.cs
- Type.cs
- DecoderReplacementFallback.cs
- Utilities.cs
- SqlGatherConsumedAliases.cs
- CanonicalizationDriver.cs
- DataGridDetailsPresenterAutomationPeer.cs
- FixedHighlight.cs
- BooleanKeyFrameCollection.cs
- XmlMembersMapping.cs
- DataTemplateSelector.cs
- GradientBrush.cs
- COAUTHIDENTITY.cs
- SourceFilter.cs
- StringPropertyBuilder.cs
- FileInfo.cs
- ResourcePermissionBase.cs
- SetterBaseCollection.cs
- ToolStripDropDown.cs
- LongTypeConverter.cs
- diagnosticsswitches.cs
- HtmlInputSubmit.cs
- TextDecoration.cs
- messageonlyhwndwrapper.cs
- SqlDataSourceCommandEventArgs.cs
- Errors.cs
- ISFClipboardData.cs
- MarginsConverter.cs
- UnicodeEncoding.cs
- ListDesigner.cs
- ValidatingCollection.cs
- MemoryMappedFile.cs
- OdbcEnvironmentHandle.cs
- _KerberosClient.cs
- DocumentsTrace.cs
- WebPartTransformerAttribute.cs
- UnconditionalPolicy.cs
- SerializableReadOnlyDictionary.cs
- _FtpControlStream.cs
- PlatformNotSupportedException.cs
- ForwardPositionQuery.cs
- StaticExtensionConverter.cs
- StateBag.cs
- Processor.cs
- UITypeEditor.cs
- RestHandlerFactory.cs
- DataGridSortCommandEventArgs.cs
- SizeConverter.cs
- MethodAccessException.cs
- ExtensionWindowHeader.cs
- PolicyLevel.cs
- DbProviderFactories.cs
- EnumerableRowCollection.cs
- sqlstateclientmanager.cs
- SoapHeader.cs
- IdentitySection.cs
- FixedSOMTextRun.cs
- EnumBuilder.cs
- MessageQueueAccessControlEntry.cs
- MarkerProperties.cs
- Invariant.cs
- ComboBoxRenderer.cs
- MiniCustomAttributeInfo.cs
- columnmapfactory.cs
- EntityReference.cs
- RegexRunnerFactory.cs
- HtmlInputReset.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- BitmapEffectGeneralTransform.cs
- FilteredXmlReader.cs
- VisualStateManager.cs
- MD5HashHelper.cs
- ping.cs
- EpmContentDeSerializerBase.cs
- _CookieModule.cs