Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / WebResponse.cs / 1 / WebResponse.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.IO; using System.Runtime.Serialization; using System.Security.Permissions; /*++ WebResponse - The abstract base class for all WebResponse objects. --*/ ////// [Serializable] public abstract class WebResponse : MarshalByRefObject, ISerializable, IDisposable { private bool m_IsCacheFresh; private bool m_IsFromCache; ////// A /// response from a Uniform Resource Indentifier (Uri). This is an abstract class. /// ////// protected WebResponse() { } // // ISerializable constructor // ///Initializes a new /// instance of the ////// class. /// protected WebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext) { } // // ISerializable method // ///[To be supplied.] ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide a way for derived classes to access this method even if they reimplement ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected virtual void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { } /*++ Close - Closes the Response after the use. This causes the read stream to be closed. --*/ public virtual void Close() { throw ExceptionHelper.MethodNotImplementedException; } /// void IDisposable.Dispose() { try { Close(); OnDispose(); } catch { } } internal virtual void OnDispose(){ } public virtual bool IsFromCache { get {return m_IsFromCache;} } internal bool InternalSetFromCache { set { m_IsFromCache = value; } } internal virtual bool IsCacheFresh { get {return m_IsCacheFresh;} } internal bool InternalSetIsCacheFresh { set { m_IsCacheFresh = value; } } public virtual bool IsMutuallyAuthenticated { get {return false;} } /*++ ContentLength - Content length of response. This property returns the content length of the response. --*/ /// /// public virtual long ContentLength { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ContentType - Content type of response. This property returns the content type of the response. --*/ ///When overridden in a derived class, gets or /// sets /// the content length of data being received. ////// public virtual string ContentType { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ResponseStream - Get the response stream for this response. This property returns the response stream for this WebResponse. Input: Nothing. Returns: Response stream for response. read-only --*/ ///When overridden in a derived class, /// gets /// or sets the content type of the data being received. ////// public virtual Stream GetResponseStream() { throw ExceptionHelper.MethodNotImplementedException; } /*++ ResponseUri - Gets the final Response Uri, that includes any changes that may have transpired from the orginal request This property returns Uri for this WebResponse. Input: Nothing. Returns: Response Uri for response. read-only --*/ ///When overridden in a derived class, returns the ///object used /// for reading data from the resource referenced in the /// object. /// public virtual Uri ResponseUri { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ Headers - Gets any request specific headers associated with this request, this is simply a name/value pair collection Input: Nothing. Returns: This property returns WebHeaderCollection. read-only --*/ ///When overridden in a derived class, gets the Uri that /// actually responded to the request. ////// public virtual WebHeaderCollection Headers { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } }; // class WebResponse } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //When overridden in a derived class, gets /// a collection of header name-value pairs associated with this /// request. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.IO; using System.Runtime.Serialization; using System.Security.Permissions; /*++ WebResponse - The abstract base class for all WebResponse objects. --*/ ////// [Serializable] public abstract class WebResponse : MarshalByRefObject, ISerializable, IDisposable { private bool m_IsCacheFresh; private bool m_IsFromCache; ////// A /// response from a Uniform Resource Indentifier (Uri). This is an abstract class. /// ////// protected WebResponse() { } // // ISerializable constructor // ///Initializes a new /// instance of the ////// class. /// protected WebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext) { } // // ISerializable method // ///[To be supplied.] ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide a way for derived classes to access this method even if they reimplement ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected virtual void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { } /*++ Close - Closes the Response after the use. This causes the read stream to be closed. --*/ public virtual void Close() { throw ExceptionHelper.MethodNotImplementedException; } /// void IDisposable.Dispose() { try { Close(); OnDispose(); } catch { } } internal virtual void OnDispose(){ } public virtual bool IsFromCache { get {return m_IsFromCache;} } internal bool InternalSetFromCache { set { m_IsFromCache = value; } } internal virtual bool IsCacheFresh { get {return m_IsCacheFresh;} } internal bool InternalSetIsCacheFresh { set { m_IsCacheFresh = value; } } public virtual bool IsMutuallyAuthenticated { get {return false;} } /*++ ContentLength - Content length of response. This property returns the content length of the response. --*/ /// /// public virtual long ContentLength { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ContentType - Content type of response. This property returns the content type of the response. --*/ ///When overridden in a derived class, gets or /// sets /// the content length of data being received. ////// public virtual string ContentType { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ResponseStream - Get the response stream for this response. This property returns the response stream for this WebResponse. Input: Nothing. Returns: Response stream for response. read-only --*/ ///When overridden in a derived class, /// gets /// or sets the content type of the data being received. ////// public virtual Stream GetResponseStream() { throw ExceptionHelper.MethodNotImplementedException; } /*++ ResponseUri - Gets the final Response Uri, that includes any changes that may have transpired from the orginal request This property returns Uri for this WebResponse. Input: Nothing. Returns: Response Uri for response. read-only --*/ ///When overridden in a derived class, returns the ///object used /// for reading data from the resource referenced in the /// object. /// public virtual Uri ResponseUri { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ Headers - Gets any request specific headers associated with this request, this is simply a name/value pair collection Input: Nothing. Returns: This property returns WebHeaderCollection. read-only --*/ ///When overridden in a derived class, gets the Uri that /// actually responded to the request. ////// public virtual WebHeaderCollection Headers { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } }; // class WebResponse } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007.When overridden in a derived class, gets /// a collection of header name-value pairs associated with this /// request. ///
Link Menu
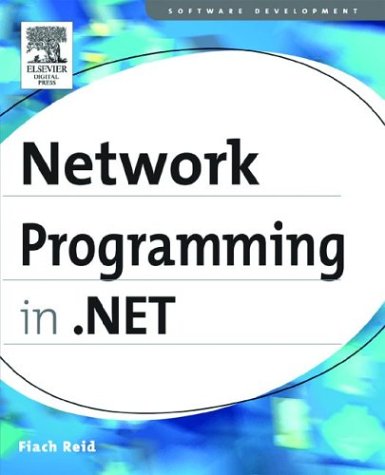
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Catch.cs
- basevalidator.cs
- WindowsAltTab.cs
- XmlSchemaSimpleTypeList.cs
- BamlVersionHeader.cs
- CounterSampleCalculator.cs
- Int64Storage.cs
- OleDbPermission.cs
- TextMetrics.cs
- EditableTreeList.cs
- SizeIndependentAnimationStorage.cs
- ContextQuery.cs
- DesignRelationCollection.cs
- Header.cs
- MarshalByValueComponent.cs
- MetadataException.cs
- ServiceModelConfigurationElementCollection.cs
- StreamGeometry.cs
- PresentationSource.cs
- BitmapInitialize.cs
- RawUIStateInputReport.cs
- Misc.cs
- FormsAuthenticationEventArgs.cs
- EdgeProfileValidation.cs
- CustomLineCap.cs
- MiniParameterInfo.cs
- Axis.cs
- LayoutTableCell.cs
- Decimal.cs
- ClosableStream.cs
- BCLDebug.cs
- CookieHandler.cs
- PerfCounters.cs
- WebPartCloseVerb.cs
- XmlQualifiedName.cs
- CompilationUtil.cs
- IntellisenseTextBox.designer.cs
- PenCursorManager.cs
- ScriptDescriptor.cs
- SamlAuthorizationDecisionClaimResource.cs
- BitmapMetadata.cs
- Slider.cs
- HtmlShimManager.cs
- NavigationFailedEventArgs.cs
- ChannelServices.cs
- ListViewTableCell.cs
- SqlResolver.cs
- DataTableReaderListener.cs
- QueryCursorEventArgs.cs
- JapaneseCalendar.cs
- WindowsButton.cs
- ErrorWebPart.cs
- GetPageNumberCompletedEventArgs.cs
- ContainerTracking.cs
- XmlQueryCardinality.cs
- WindowsAltTab.cs
- BoundPropertyEntry.cs
- AuthorizationRuleCollection.cs
- ButtonField.cs
- BrowserCapabilitiesCompiler.cs
- WizardPanelChangingEventArgs.cs
- RepeaterItemEventArgs.cs
- List.cs
- HtmlInputCheckBox.cs
- ListBase.cs
- HelpKeywordAttribute.cs
- Random.cs
- OdbcParameter.cs
- SerTrace.cs
- ProxyWebPartConnectionCollection.cs
- ActivityExecutorDelegateInfo.cs
- InputMethod.cs
- CodeCompiler.cs
- ClientTargetCollection.cs
- ComboBoxRenderer.cs
- XmlHierarchicalDataSourceView.cs
- MessagePropertyAttribute.cs
- NavigationWindow.cs
- WebBrowserPermission.cs
- RequestQueue.cs
- SmtpTransport.cs
- LogEntrySerializationException.cs
- ColumnResult.cs
- DownloadProgressEventArgs.cs
- HttpConfigurationSystem.cs
- CategoryAttribute.cs
- TableStyle.cs
- DeferrableContentConverter.cs
- ColumnHeaderConverter.cs
- LogReserveAndAppendState.cs
- TraceLevelStore.cs
- PersistenceMetadataNamespace.cs
- NGCSerializer.cs
- NamespaceEmitter.cs
- DtdParser.cs
- DocumentViewer.cs
- PreloadedPackages.cs
- Math.cs
- MLangCodePageEncoding.cs
- HtmlProps.cs