Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlSortKeyAccumulator.cs / 1305376 / XmlSortKeyAccumulator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Accumulates a list of sort keys and stores them in an array. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct XmlSortKeyAccumulator { private XmlSortKey[] keys; private int pos; #if DEBUG private const int DefaultSortKeyCount = 4; #else private const int DefaultSortKeyCount = 64; #endif ////// Initialize the XmlSortKeyAccumulator. /// public void Create() { if (this.keys == null) this.keys = new XmlSortKey[DefaultSortKeyCount]; this.pos = 0; this.keys[0] = null; } ////// Create a new sort key and append it to the current run of sort keys. /// public void AddStringSortKey(XmlCollation collation, string value) { AppendSortKey(collation.CreateSortKey(value)); } public void AddDecimalSortKey(XmlCollation collation, decimal value) { AppendSortKey(new XmlDecimalSortKey(value, collation)); } public void AddIntegerSortKey(XmlCollation collation, long value) { AppendSortKey(new XmlIntegerSortKey(value, collation)); } public void AddIntSortKey(XmlCollation collation, int value) { AppendSortKey(new XmlIntSortKey(value, collation)); } public void AddDoubleSortKey(XmlCollation collation, double value) { AppendSortKey(new XmlDoubleSortKey(value, collation)); } public void AddDateTimeSortKey(XmlCollation collation, DateTime value) { AppendSortKey(new XmlDateTimeSortKey(value, collation)); } public void AddEmptySortKey(XmlCollation collation) { AppendSortKey(new XmlEmptySortKey(collation)); } ////// Finish creating the current run of sort keys and begin a new run. /// public void FinishSortKeys() { this.pos++; if (this.pos >= this.keys.Length) { XmlSortKey[] keysNew = new XmlSortKey[this.pos * 2]; Array.Copy(this.keys, 0, keysNew, 0, this.keys.Length); this.keys = keysNew; } this.keys[this.pos] = null; } ////// Append new sort key to the current run of sort keys. /// private void AppendSortKey(XmlSortKey key) { // Ensure that sort will be stable by setting index of key key.Priority = this.pos; if (this.keys[this.pos] == null) this.keys[this.pos] = key; else this.keys[this.pos].AddSortKey(key); } ////// Get array of sort keys that was constructed by this internal class. /// public Array Keys { get { return this.keys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Accumulates a list of sort keys and stores them in an array. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct XmlSortKeyAccumulator { private XmlSortKey[] keys; private int pos; #if DEBUG private const int DefaultSortKeyCount = 4; #else private const int DefaultSortKeyCount = 64; #endif ////// Initialize the XmlSortKeyAccumulator. /// public void Create() { if (this.keys == null) this.keys = new XmlSortKey[DefaultSortKeyCount]; this.pos = 0; this.keys[0] = null; } ////// Create a new sort key and append it to the current run of sort keys. /// public void AddStringSortKey(XmlCollation collation, string value) { AppendSortKey(collation.CreateSortKey(value)); } public void AddDecimalSortKey(XmlCollation collation, decimal value) { AppendSortKey(new XmlDecimalSortKey(value, collation)); } public void AddIntegerSortKey(XmlCollation collation, long value) { AppendSortKey(new XmlIntegerSortKey(value, collation)); } public void AddIntSortKey(XmlCollation collation, int value) { AppendSortKey(new XmlIntSortKey(value, collation)); } public void AddDoubleSortKey(XmlCollation collation, double value) { AppendSortKey(new XmlDoubleSortKey(value, collation)); } public void AddDateTimeSortKey(XmlCollation collation, DateTime value) { AppendSortKey(new XmlDateTimeSortKey(value, collation)); } public void AddEmptySortKey(XmlCollation collation) { AppendSortKey(new XmlEmptySortKey(collation)); } ////// Finish creating the current run of sort keys and begin a new run. /// public void FinishSortKeys() { this.pos++; if (this.pos >= this.keys.Length) { XmlSortKey[] keysNew = new XmlSortKey[this.pos * 2]; Array.Copy(this.keys, 0, keysNew, 0, this.keys.Length); this.keys = keysNew; } this.keys[this.pos] = null; } ////// Append new sort key to the current run of sort keys. /// private void AppendSortKey(XmlSortKey key) { // Ensure that sort will be stable by setting index of key key.Priority = this.pos; if (this.keys[this.pos] == null) this.keys[this.pos] = key; else this.keys[this.pos].AddSortKey(key); } ////// Get array of sort keys that was constructed by this internal class. /// public Array Keys { get { return this.keys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
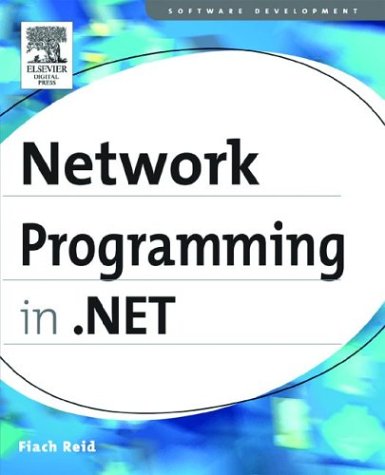
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseTemplateCodeDomTreeGenerator.cs
- FlowDocumentPage.cs
- ResXResourceWriter.cs
- ScriptBehaviorDescriptor.cs
- EdmMember.cs
- ResourceReader.cs
- Setter.cs
- CodeSnippetStatement.cs
- WindowsFormsHostAutomationPeer.cs
- SmtpNetworkElement.cs
- SharedPersonalizationStateInfo.cs
- WebColorConverter.cs
- FontFamilyValueSerializer.cs
- XPathSingletonIterator.cs
- Dictionary.cs
- SQLStringStorage.cs
- DataGridViewAutoSizeModeEventArgs.cs
- MemoryStream.cs
- TargetInvocationException.cs
- StylusPlugInCollection.cs
- AutomationIdentifier.cs
- CallbackValidatorAttribute.cs
- ToolStripOverflow.cs
- AssemblyHelper.cs
- EdmItemCollection.cs
- Matrix.cs
- CipherData.cs
- PreviewPageInfo.cs
- MetadataCache.cs
- ChannelSinkStacks.cs
- ControlCachePolicy.cs
- GlyphRunDrawing.cs
- CodeLinePragma.cs
- GenericsInstances.cs
- PerformanceCounterManager.cs
- ApplicationGesture.cs
- SchemaNames.cs
- BigInt.cs
- CfgParser.cs
- OciLobLocator.cs
- RawUIStateInputReport.cs
- WebPermission.cs
- TouchPoint.cs
- MessageSecurityOverMsmqElement.cs
- Classification.cs
- XmlSchemaAnyAttribute.cs
- SemanticKeyElement.cs
- MaskedTextBox.cs
- RelationshipConstraintValidator.cs
- XamlPathDataSerializer.cs
- FontFamilyIdentifier.cs
- EditingScope.cs
- EncoderReplacementFallback.cs
- WebContext.cs
- MessageSmuggler.cs
- FixedTextView.cs
- IConvertible.cs
- WindowsPen.cs
- StorageFunctionMapping.cs
- NetworkInformationPermission.cs
- CodeDomSerializerException.cs
- ResourceExpressionBuilder.cs
- TabItemAutomationPeer.cs
- DataControlCommands.cs
- DeflateInput.cs
- _SSPISessionCache.cs
- Bind.cs
- Invariant.cs
- ToolBarButton.cs
- BooleanToVisibilityConverter.cs
- RSAPKCS1SignatureDeformatter.cs
- StyleHelper.cs
- AllMembershipCondition.cs
- EntityDataSourceWrapper.cs
- NonVisualControlAttribute.cs
- RewritingPass.cs
- DesignColumn.cs
- SmtpNetworkElement.cs
- PagesSection.cs
- CSharpCodeProvider.cs
- CodeDomSerializer.cs
- GenericsInstances.cs
- TemplateApplicationHelper.cs
- XmlDomTextWriter.cs
- WmlPanelAdapter.cs
- DateTimeStorage.cs
- VarRefManager.cs
- X509ChainElement.cs
- QueryResult.cs
- Types.cs
- DataSourceXmlElementAttribute.cs
- ColorIndependentAnimationStorage.cs
- XmlSignatureProperties.cs
- OpacityConverter.cs
- XmlSchemaAnnotated.cs
- MobileListItemCollection.cs
- SqlNodeTypeOperators.cs
- FormatConvertedBitmap.cs
- ShapeTypeface.cs
- NativeMethods.cs