Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripOverflow.cs / 1305376 / ToolStripOverflow.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Layout; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; ////// /// ToolStripOverflow /// [ComVisible(true)] [ClassInterface(ClassInterfaceType.AutoDispatch)] public class ToolStripOverflow : ToolStripDropDown, IArrangedElement { #if DEBUG internal static readonly TraceSwitch PopupLayoutDebug = new TraceSwitch("PopupLayoutDebug", "Debug ToolStripPopup Layout code"); #else internal static readonly TraceSwitch PopupLayoutDebug; #endif private ToolStripOverflowButton ownerItem; ///public ToolStripOverflow (ToolStripItem parentItem) : base(parentItem) { if (parentItem == null) { throw new ArgumentNullException("parentItem"); } ownerItem = parentItem as ToolStripOverflowButton; } /// protected internal override ToolStripItemCollection DisplayedItems { get { if (ParentToolStrip != null) { ToolStripItemCollection items = ParentToolStrip.OverflowItems; return items; } return new ToolStripItemCollection(null, false); } } public override ToolStripItemCollection Items { get { return new ToolStripItemCollection(null, /*ownedCollection=*/false, /*readonly=*/true); } } private ToolStrip ParentToolStrip { get { if (ownerItem != null) { return ownerItem.ParentToolStrip; } return null; } } /// /// ArrangedElementCollection IArrangedElement.Children { get { return DisplayedItems; } } /// /// IArrangedElement IArrangedElement.Container { get { return ParentInternal; } } /// /// bool IArrangedElement.ParticipatesInLayout { get { return GetState(STATE_VISIBLE); } } /// /// PropertyStore IArrangedElement.Properties { get { return Properties; } } /// /// void IArrangedElement.SetBounds(Rectangle bounds, BoundsSpecified specified) { SetBoundsCore(bounds.X, bounds.Y, bounds.Width, bounds.Height, specified); } /// /// /// Summary of CreateLayoutEngine. /// /// public override LayoutEngine LayoutEngine { get { return FlowLayout.Instance; } } protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripOverflowAccessibleObject(this); } [SuppressMessage("Microsoft.Security", "CA2119:SealMethodsThatSatisfyPrivateInterfaces")] public override Size GetPreferredSize(Size constrainingSize) { constrainingSize.Width = 200; return base.GetPreferredSize(constrainingSize); } protected override void OnLayout(LayoutEventArgs e) { if (ParentToolStrip != null && ParentToolStrip.IsInDesignMode) { if (FlowLayout.GetFlowDirection(this) != FlowDirection.TopDown) { FlowLayout.SetFlowDirection(this, FlowDirection.TopDown); } if (FlowLayout.GetWrapContents(this)) { FlowLayout.SetWrapContents(this, false); } } else { if (FlowLayout.GetFlowDirection(this) != FlowDirection.LeftToRight) { FlowLayout.SetFlowDirection(this, FlowDirection.LeftToRight); } if (!FlowLayout.GetWrapContents(this)) { FlowLayout.SetWrapContents(this, true); } } base.OnLayout(e); } ///protected override void SetDisplayedItems() { // do nothing here.... this is really for the setting the overflow/displayed items on the // main winbar. Our working item collection is our displayed item collection... calling // base would clear it out. Size biggestItemSize = Size.Empty; for (int j = 0; j < DisplayedItems.Count; j++) { ToolStripItem item = DisplayedItems[j]; if (((IArrangedElement)item).ParticipatesInLayout) { HasVisibleItems = true; biggestItemSize = LayoutUtils.UnionSizes(biggestItemSize, item.Bounds.Size); } } SetLargestItemSize(biggestItemSize); } private class ToolStripOverflowAccessibleObject : ToolStripAccessibleObject { public ToolStripOverflowAccessibleObject(ToolStripOverflow owner) : base(owner) { } public override AccessibleObject GetChild(int index) { return ((ToolStripOverflow)Owner).DisplayedItems[index].AccessibilityObject; } public override int GetChildCount() { return ((ToolStripOverflow)Owner).DisplayedItems.Count; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Layout; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; ////// /// ToolStripOverflow /// [ComVisible(true)] [ClassInterface(ClassInterfaceType.AutoDispatch)] public class ToolStripOverflow : ToolStripDropDown, IArrangedElement { #if DEBUG internal static readonly TraceSwitch PopupLayoutDebug = new TraceSwitch("PopupLayoutDebug", "Debug ToolStripPopup Layout code"); #else internal static readonly TraceSwitch PopupLayoutDebug; #endif private ToolStripOverflowButton ownerItem; ///public ToolStripOverflow (ToolStripItem parentItem) : base(parentItem) { if (parentItem == null) { throw new ArgumentNullException("parentItem"); } ownerItem = parentItem as ToolStripOverflowButton; } /// protected internal override ToolStripItemCollection DisplayedItems { get { if (ParentToolStrip != null) { ToolStripItemCollection items = ParentToolStrip.OverflowItems; return items; } return new ToolStripItemCollection(null, false); } } public override ToolStripItemCollection Items { get { return new ToolStripItemCollection(null, /*ownedCollection=*/false, /*readonly=*/true); } } private ToolStrip ParentToolStrip { get { if (ownerItem != null) { return ownerItem.ParentToolStrip; } return null; } } /// /// ArrangedElementCollection IArrangedElement.Children { get { return DisplayedItems; } } /// /// IArrangedElement IArrangedElement.Container { get { return ParentInternal; } } /// /// bool IArrangedElement.ParticipatesInLayout { get { return GetState(STATE_VISIBLE); } } /// /// PropertyStore IArrangedElement.Properties { get { return Properties; } } /// /// void IArrangedElement.SetBounds(Rectangle bounds, BoundsSpecified specified) { SetBoundsCore(bounds.X, bounds.Y, bounds.Width, bounds.Height, specified); } /// /// /// Summary of CreateLayoutEngine. /// /// public override LayoutEngine LayoutEngine { get { return FlowLayout.Instance; } } protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripOverflowAccessibleObject(this); } [SuppressMessage("Microsoft.Security", "CA2119:SealMethodsThatSatisfyPrivateInterfaces")] public override Size GetPreferredSize(Size constrainingSize) { constrainingSize.Width = 200; return base.GetPreferredSize(constrainingSize); } protected override void OnLayout(LayoutEventArgs e) { if (ParentToolStrip != null && ParentToolStrip.IsInDesignMode) { if (FlowLayout.GetFlowDirection(this) != FlowDirection.TopDown) { FlowLayout.SetFlowDirection(this, FlowDirection.TopDown); } if (FlowLayout.GetWrapContents(this)) { FlowLayout.SetWrapContents(this, false); } } else { if (FlowLayout.GetFlowDirection(this) != FlowDirection.LeftToRight) { FlowLayout.SetFlowDirection(this, FlowDirection.LeftToRight); } if (!FlowLayout.GetWrapContents(this)) { FlowLayout.SetWrapContents(this, true); } } base.OnLayout(e); } ///protected override void SetDisplayedItems() { // do nothing here.... this is really for the setting the overflow/displayed items on the // main winbar. Our working item collection is our displayed item collection... calling // base would clear it out. Size biggestItemSize = Size.Empty; for (int j = 0; j < DisplayedItems.Count; j++) { ToolStripItem item = DisplayedItems[j]; if (((IArrangedElement)item).ParticipatesInLayout) { HasVisibleItems = true; biggestItemSize = LayoutUtils.UnionSizes(biggestItemSize, item.Bounds.Size); } } SetLargestItemSize(biggestItemSize); } private class ToolStripOverflowAccessibleObject : ToolStripAccessibleObject { public ToolStripOverflowAccessibleObject(ToolStripOverflow owner) : base(owner) { } public override AccessibleObject GetChild(int index) { return ((ToolStripOverflow)Owner).DisplayedItems[index].AccessibilityObject; } public override int GetChildCount() { return ((ToolStripOverflow)Owner).DisplayedItems.Count; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
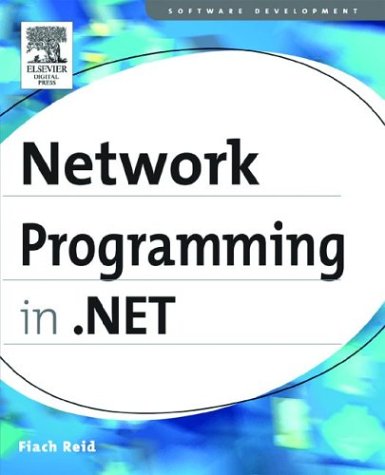
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProcessHostConfigUtils.cs
- RewritingPass.cs
- RowToParametersTransformer.cs
- OracleDateTime.cs
- SimpleWorkerRequest.cs
- LogExtent.cs
- LoginName.cs
- XPathNodeHelper.cs
- dataprotectionpermissionattribute.cs
- WebPartCollection.cs
- Underline.cs
- VisualStyleElement.cs
- BezierSegment.cs
- XmlWellformedWriterHelpers.cs
- QuaternionConverter.cs
- StrokeDescriptor.cs
- XPathException.cs
- JsonQNameDataContract.cs
- StringValidatorAttribute.cs
- FormViewRow.cs
- DataGridColumnFloatingHeader.cs
- VirtualPath.cs
- DataSourceControl.cs
- ControlType.cs
- XamlGridLengthSerializer.cs
- Models.cs
- FixedFindEngine.cs
- RegionInfo.cs
- OracleTransaction.cs
- ParseHttpDate.cs
- FontFamily.cs
- XmlEncodedRawTextWriter.cs
- SafeIUnknown.cs
- JavaScriptSerializer.cs
- BitSet.cs
- ValueUnavailableException.cs
- SemanticBasicElement.cs
- SqlBuilder.cs
- DoubleAnimation.cs
- XmlLangPropertyAttribute.cs
- ResourceAssociationType.cs
- SplitterCancelEvent.cs
- GlobalizationSection.cs
- XmlSchemaInferenceException.cs
- LedgerEntry.cs
- SqlProfileProvider.cs
- BinaryObjectInfo.cs
- LinqDataSourceDisposeEventArgs.cs
- EnumerationRangeValidationUtil.cs
- OptimalTextSource.cs
- followingquery.cs
- RadioButtonStandardAdapter.cs
- BuildDependencySet.cs
- TraceSection.cs
- HashHelpers.cs
- TargetFrameworkAttribute.cs
- ComplusTypeValidator.cs
- ReferencedAssembly.cs
- ExceptionUtil.cs
- AssociationTypeEmitter.cs
- LineProperties.cs
- LinqDataSourceInsertEventArgs.cs
- ThreadNeutralSemaphore.cs
- SoapUnknownHeader.cs
- CatalogPartCollection.cs
- DataControlButton.cs
- SendMailErrorEventArgs.cs
- MonthChangedEventArgs.cs
- AggregateNode.cs
- XmlSchemaAttributeGroupRef.cs
- ServiceBusyException.cs
- SchemaElementLookUpTableEnumerator.cs
- InputProviderSite.cs
- SignatureHelper.cs
- SqlException.cs
- BinaryConverter.cs
- LinqDataSourceEditData.cs
- CodeTypeReferenceCollection.cs
- TextTreeTextElementNode.cs
- DataGridColumn.cs
- SubMenuStyleCollection.cs
- BrushValueSerializer.cs
- Pool.cs
- XmlDictionary.cs
- BindingContext.cs
- ChangesetResponse.cs
- FormViewInsertEventArgs.cs
- EventLogHandle.cs
- HMACRIPEMD160.cs
- EraserBehavior.cs
- HandlerFactoryCache.cs
- RectangleGeometry.cs
- ValidationErrorCollection.cs
- ErrorProvider.cs
- MouseButtonEventArgs.cs
- EventSinkHelperWriter.cs
- IPAddressCollection.cs
- SafeNativeMethods.cs
- ModelPerspective.cs
- StackSpiller.Generated.cs