Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / Stylus / PenThreadPool.cs / 1 / PenThreadPool.cs
using System; using System.Collections.Generic; using System.Threading; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Win32.Penimc; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// /// internal class PenThreadPool { ////// Critical - Constructor for singleton of our PenThreadPool. /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// Instance (above) /// /// [SecurityCritical] static PenThreadPool() { } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] [ThreadStatic] private static PenThreadPool _penThreadPool; ///////////////////////////////////////////////////////////////////// ////// ////// Critical - Returns a PenThread (creates as needed). /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// PenContext.Dispose /// PenContext.Enable /// PenContext.Disable /// /// [SecurityCritical] internal static PenThread GetPenThreadForPenContext(PenContext penContext) { // Create the threadstatic DynamicRendererThreadManager as needed for calling thread. // It only creates one if (_penThreadPool == null) { _penThreadPool = new PenThreadPool(); } return _penThreadPool.GetPenThreadForPenContextHelper(penContext); // Adds to weak ref list if creating new one. } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] private List_penThreadWeakRefList; ///////////////////////////////////////////////////////////////////// /// /// /// ////// Critical - Initializes critical data: m_PenThreads /// /// [SecurityCritical] internal PenThreadPool() { _penThreadWeakRefList = new List(); } /// /// Critical - Calls SecurityCritical code (PenThread constructor). /// Called by BeginService. /// TreatAsSafe boundry is Stylus.EnableCore, Stylus.RegisterHwndForInput /// and HwndWrapperHook class (via HwndSource.InputFilterMessage). /// [SecurityCritical] private PenThread GetPenThreadForPenContextHelper(PenContext penContext) { bool needCleanup = false; PenThread penThread = null; int i; // Scan existing penthreads to see if we have an available slot for context. for (i=0; i < _penThreadWeakRefList.Count; i++) { PenThread penThreadFound = _penThreadWeakRefList[i].Target as PenThread; if (penThreadFound == null) { needCleanup = true; } else { // See if we can use this one if (penContext == null || penThreadFound.AddPenContext(penContext)) { // We can use this one. penThread = penThreadFound; break; } } } if (needCleanup) { // prune invalid refs for (i=_penThreadWeakRefList.Count - 1; i >= 0; i--) { if (_penThreadWeakRefList[i].Target == null) { _penThreadWeakRefList.RemoveAt(i); } } } if (penThread == null) { penThread = new PenThread(); // Make sure we add this context to the penthread if (penContext != null) { penThread.AddPenContext(penContext); } _penThreadWeakRefList.Add(new WeakReference(penThread)); } return penThread; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Threading; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Win32.Penimc; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// /// internal class PenThreadPool { ////// Critical - Constructor for singleton of our PenThreadPool. /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// Instance (above) /// /// [SecurityCritical] static PenThreadPool() { } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] [ThreadStatic] private static PenThreadPool _penThreadPool; ///////////////////////////////////////////////////////////////////// ////// ////// Critical - Returns a PenThread (creates as needed). /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// PenContext.Dispose /// PenContext.Enable /// PenContext.Disable /// /// [SecurityCritical] internal static PenThread GetPenThreadForPenContext(PenContext penContext) { // Create the threadstatic DynamicRendererThreadManager as needed for calling thread. // It only creates one if (_penThreadPool == null) { _penThreadPool = new PenThreadPool(); } return _penThreadPool.GetPenThreadForPenContextHelper(penContext); // Adds to weak ref list if creating new one. } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] private List_penThreadWeakRefList; ///////////////////////////////////////////////////////////////////// /// /// /// ////// Critical - Initializes critical data: m_PenThreads /// /// [SecurityCritical] internal PenThreadPool() { _penThreadWeakRefList = new List(); } /// /// Critical - Calls SecurityCritical code (PenThread constructor). /// Called by BeginService. /// TreatAsSafe boundry is Stylus.EnableCore, Stylus.RegisterHwndForInput /// and HwndWrapperHook class (via HwndSource.InputFilterMessage). /// [SecurityCritical] private PenThread GetPenThreadForPenContextHelper(PenContext penContext) { bool needCleanup = false; PenThread penThread = null; int i; // Scan existing penthreads to see if we have an available slot for context. for (i=0; i < _penThreadWeakRefList.Count; i++) { PenThread penThreadFound = _penThreadWeakRefList[i].Target as PenThread; if (penThreadFound == null) { needCleanup = true; } else { // See if we can use this one if (penContext == null || penThreadFound.AddPenContext(penContext)) { // We can use this one. penThread = penThreadFound; break; } } } if (needCleanup) { // prune invalid refs for (i=_penThreadWeakRefList.Count - 1; i >= 0; i--) { if (_penThreadWeakRefList[i].Target == null) { _penThreadWeakRefList.RemoveAt(i); } } } if (penThread == null) { penThread = new PenThread(); // Make sure we add this context to the penthread if (penContext != null) { penThread.AddPenContext(penContext); } _penThreadWeakRefList.Add(new WeakReference(penThread)); } return penThread; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
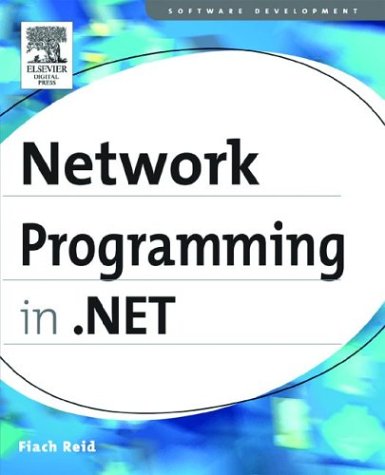
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IEnumerable.cs
- FormsAuthenticationModule.cs
- MobileControlsSectionHelper.cs
- RouteValueDictionary.cs
- InvokeProviderWrapper.cs
- TreeView.cs
- TrackBarRenderer.cs
- DefaultBindingPropertyAttribute.cs
- Stylus.cs
- ISAPIRuntime.cs
- RelationshipConstraintValidator.cs
- InvokeHandlers.cs
- ResourceDefaultValueAttribute.cs
- LineSegment.cs
- Command.cs
- MD5.cs
- ByteStream.cs
- ClientOptions.cs
- TrackingLocationCollection.cs
- URLString.cs
- UniqueIdentifierService.cs
- Int16.cs
- MediaCommands.cs
- WindowsTokenRoleProvider.cs
- ResourcesBuildProvider.cs
- FileSystemInfo.cs
- _DisconnectOverlappedAsyncResult.cs
- SecurityKeyIdentifierClause.cs
- CollectionView.cs
- RefreshEventArgs.cs
- PriorityItem.cs
- HttpModuleCollection.cs
- CopyNamespacesAction.cs
- XmlIterators.cs
- RecordConverter.cs
- WindowPatternIdentifiers.cs
- AssemblyHelper.cs
- KeyboardNavigation.cs
- TransformerTypeCollection.cs
- RootBrowserWindowAutomationPeer.cs
- SettingsPropertyWrongTypeException.cs
- SmtpDigestAuthenticationModule.cs
- ItemCheckedEvent.cs
- EmptyStringExpandableObjectConverter.cs
- GridViewRowPresenterBase.cs
- MdiWindowListItemConverter.cs
- TreeViewDataItemAutomationPeer.cs
- DrawingContext.cs
- PlainXmlDeserializer.cs
- ExceptQueryOperator.cs
- ByeMessageCD1.cs
- TypeDescriptorFilterService.cs
- TextRunCacheImp.cs
- SqlBulkCopyColumnMappingCollection.cs
- AttachmentService.cs
- TextLineBreak.cs
- XmlILOptimizerVisitor.cs
- uribuilder.cs
- Size3DConverter.cs
- ProcessModuleDesigner.cs
- DeleteMemberBinder.cs
- HScrollProperties.cs
- CellRelation.cs
- ReachUIElementCollectionSerializer.cs
- ArrayExtension.cs
- ClientRoleProvider.cs
- DataGridViewCellStyleChangedEventArgs.cs
- EntityViewContainer.cs
- PropertyGridCommands.cs
- AttributeConverter.cs
- XPathDocumentNavigator.cs
- IPAddress.cs
- DefaultAssemblyResolver.cs
- CorrelationTokenInvalidatedHandler.cs
- WebServiceParameterData.cs
- DispatcherHookEventArgs.cs
- TreeViewBindingsEditorForm.cs
- NamedPipeChannelListener.cs
- DocumentReferenceCollection.cs
- EntityContainerEmitter.cs
- XomlDesignerLoader.cs
- StringWriter.cs
- ContractSearchPattern.cs
- Deflater.cs
- MultiSelector.cs
- HostingEnvironmentException.cs
- MenuEventArgs.cs
- SqlClientMetaDataCollectionNames.cs
- _LoggingObject.cs
- PropertyItemInternal.cs
- DefaultSerializationProviderAttribute.cs
- WhitespaceRuleLookup.cs
- MenuItemBindingCollection.cs
- SqlDataSourceView.cs
- BrushMappingModeValidation.cs
- HttpCachePolicy.cs
- IssuedTokenServiceElement.cs
- BitmapEffectGroup.cs
- DesignerDataRelationship.cs
- Page.cs