Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / DefaultAssemblyResolver.cs / 1 / DefaultAssemblyResolver.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Reflection; using System.IO; using System.Diagnostics; using System.Collections.Generic; using System.Linq; using System.Collections; namespace System.Data.Metadata.Edm { internal class DefaultAssemblyResolver : MetadataArtifactAssemblyResolver { internal override bool TryResolveAssemblyReference(AssemblyName refernceName, out Assembly assembly) { assembly = ResolveAssembly(refernceName); return assembly != null; } internal override IEnumerableGetWildcardAssemblies() { return GetAllDiscoverableAssemblies(); } internal Assembly ResolveAssembly(AssemblyName referenceName) { Assembly assembly = null; // look in the already loaded assemblies foreach (Assembly current in GetAlreadyLoadedNonSystemAssemblies()) { if (AssemblyName.ReferenceMatchesDefinition(referenceName, current.GetName())) { return current; } } // try to load this one specifically if (assembly == null) { try { assembly = Assembly.ReflectionOnlyLoad(referenceName.FullName); if (assembly != null) { return assembly; } } catch (FileNotFoundException) { // eat this one, just means we couldn't locate it } } // try all the discoverable ones TryFindWildcardAssemblyMatch(referenceName, out assembly); return assembly; } private bool TryFindWildcardAssemblyMatch(AssemblyName referenceName, out Assembly assembly) { Debug.Assert(referenceName != null); foreach (Assembly current in GetAllDiscoverableAssemblies()) { if (AssemblyName.ReferenceMatchesDefinition(referenceName, current.GetName())) { assembly = current; return true; } } assembly = null; return false; } /// /// Return all assemblies loaded in the current AppDomain that are not signed /// with the Microsoft Key. /// ///A list of assemblies private static IEnumerableGetAlreadyLoadedNonSystemAssemblies() { Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies(); return assemblies.Where(a => a != null && !ObjectItemCollection.ShouldFilterAssembly(a.FullName)); } /// /// This method returns a list of assemblies whose contents depend on whether we /// are running in an ASP.NET environment. If we are indeed in a Web/ASP.NET /// scenario, we pick up the assemblies that all page compilations need to /// reference. If not, then we simply get the list of assemblies referenced by /// the entry assembly. /// ///A list of assemblies private static IEnumerableGetAllDiscoverableAssemblies() { Assembly assembly = Assembly.GetEntryAssembly(); HashSet assemblyList = new HashSet ( (IEqualityComparer )new AssemblyComparer ()); foreach (Assembly loadedAssembly in GetAlreadyLoadedNonSystemAssemblies()) { assemblyList.Add(loadedAssembly); } AspProxy aspProxy = new AspProxy(); if (!aspProxy.IsAspNetEnvironment()) { if (assembly == null) { return assemblyList; } AssemblyName[] assemblyNames = assembly.GetReferencedAssemblies(); if (assemblyNames != null && assemblyNames.Length > 0) { foreach (AssemblyName assemblyName in assemblyNames) { // skip system assemblies // if (ObjectItemCollection.ShouldFilterAssembly(assemblyName.FullName)) { continue; } assemblyList.Add(Assembly.ReflectionOnlyLoad(assemblyName.FullName)); } } if (!ObjectItemCollection.ShouldFilterAssembly(assembly.FullName)) { assemblyList.Add(assembly); } return assemblyList; } if (aspProxy.HasBuildManagerType()) { IEnumerable referencedAssemblies = aspProxy.GetBuildManagerReferencedAssemblies(); // filter out system assemblies if (referencedAssemblies != null) { foreach (object objAssembly in referencedAssemblies) { Assembly referencedAssembly = objAssembly as Assembly; if (ObjectItemCollection.ShouldFilterAssembly(referencedAssembly.FullName)) { continue; } assemblyList.Add(referencedAssembly); } } } if (assembly != null && !ObjectItemCollection.ShouldFilterAssembly(assembly.FullName)) { assemblyList.Add(assembly); } return assemblyList.Where(a => a != null); } internal sealed class AssemblyComparer : IEqualityComparer where T : Assembly { /// /// if two assemblies have the same full name, we will consider them as the same. /// for example, /// both of x and y have the full name as "{RES, Version=3.5.0.0, Culture=neutral, PublicKeyToken=null}", /// although they are different instances since the ReflectionOnly field in them are different, we sitll /// consider them as the same. /// /// /// ///public bool Equals(T x, T y) { // return *true* when either the reference are the same // *or* the Assembly names are commutative equal return object.ReferenceEquals(x, y) || (AssemblyName.ReferenceMatchesDefinition(x.GetName(), y.GetName()) && AssemblyName.ReferenceMatchesDefinition(y.GetName(), x.GetName())); } public int GetHashCode(T assembly) { return assembly.FullName.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Reflection; using System.IO; using System.Diagnostics; using System.Collections.Generic; using System.Linq; using System.Collections; namespace System.Data.Metadata.Edm { internal class DefaultAssemblyResolver : MetadataArtifactAssemblyResolver { internal override bool TryResolveAssemblyReference(AssemblyName refernceName, out Assembly assembly) { assembly = ResolveAssembly(refernceName); return assembly != null; } internal override IEnumerableGetWildcardAssemblies() { return GetAllDiscoverableAssemblies(); } internal Assembly ResolveAssembly(AssemblyName referenceName) { Assembly assembly = null; // look in the already loaded assemblies foreach (Assembly current in GetAlreadyLoadedNonSystemAssemblies()) { if (AssemblyName.ReferenceMatchesDefinition(referenceName, current.GetName())) { return current; } } // try to load this one specifically if (assembly == null) { try { assembly = Assembly.ReflectionOnlyLoad(referenceName.FullName); if (assembly != null) { return assembly; } } catch (FileNotFoundException) { // eat this one, just means we couldn't locate it } } // try all the discoverable ones TryFindWildcardAssemblyMatch(referenceName, out assembly); return assembly; } private bool TryFindWildcardAssemblyMatch(AssemblyName referenceName, out Assembly assembly) { Debug.Assert(referenceName != null); foreach (Assembly current in GetAllDiscoverableAssemblies()) { if (AssemblyName.ReferenceMatchesDefinition(referenceName, current.GetName())) { assembly = current; return true; } } assembly = null; return false; } /// /// Return all assemblies loaded in the current AppDomain that are not signed /// with the Microsoft Key. /// ///A list of assemblies private static IEnumerableGetAlreadyLoadedNonSystemAssemblies() { Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies(); return assemblies.Where(a => a != null && !ObjectItemCollection.ShouldFilterAssembly(a.FullName)); } /// /// This method returns a list of assemblies whose contents depend on whether we /// are running in an ASP.NET environment. If we are indeed in a Web/ASP.NET /// scenario, we pick up the assemblies that all page compilations need to /// reference. If not, then we simply get the list of assemblies referenced by /// the entry assembly. /// ///A list of assemblies private static IEnumerableGetAllDiscoverableAssemblies() { Assembly assembly = Assembly.GetEntryAssembly(); HashSet assemblyList = new HashSet ( (IEqualityComparer )new AssemblyComparer ()); foreach (Assembly loadedAssembly in GetAlreadyLoadedNonSystemAssemblies()) { assemblyList.Add(loadedAssembly); } AspProxy aspProxy = new AspProxy(); if (!aspProxy.IsAspNetEnvironment()) { if (assembly == null) { return assemblyList; } AssemblyName[] assemblyNames = assembly.GetReferencedAssemblies(); if (assemblyNames != null && assemblyNames.Length > 0) { foreach (AssemblyName assemblyName in assemblyNames) { // skip system assemblies // if (ObjectItemCollection.ShouldFilterAssembly(assemblyName.FullName)) { continue; } assemblyList.Add(Assembly.ReflectionOnlyLoad(assemblyName.FullName)); } } if (!ObjectItemCollection.ShouldFilterAssembly(assembly.FullName)) { assemblyList.Add(assembly); } return assemblyList; } if (aspProxy.HasBuildManagerType()) { IEnumerable referencedAssemblies = aspProxy.GetBuildManagerReferencedAssemblies(); // filter out system assemblies if (referencedAssemblies != null) { foreach (object objAssembly in referencedAssemblies) { Assembly referencedAssembly = objAssembly as Assembly; if (ObjectItemCollection.ShouldFilterAssembly(referencedAssembly.FullName)) { continue; } assemblyList.Add(referencedAssembly); } } } if (assembly != null && !ObjectItemCollection.ShouldFilterAssembly(assembly.FullName)) { assemblyList.Add(assembly); } return assemblyList.Where(a => a != null); } internal sealed class AssemblyComparer : IEqualityComparer where T : Assembly { /// /// if two assemblies have the same full name, we will consider them as the same. /// for example, /// both of x and y have the full name as "{RES, Version=3.5.0.0, Culture=neutral, PublicKeyToken=null}", /// although they are different instances since the ReflectionOnly field in them are different, we sitll /// consider them as the same. /// /// /// ///public bool Equals(T x, T y) { // return *true* when either the reference are the same // *or* the Assembly names are commutative equal return object.ReferenceEquals(x, y) || (AssemblyName.ReferenceMatchesDefinition(x.GetName(), y.GetName()) && AssemblyName.ReferenceMatchesDefinition(y.GetName(), x.GetName())); } public int GetHashCode(T assembly) { return assembly.FullName.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
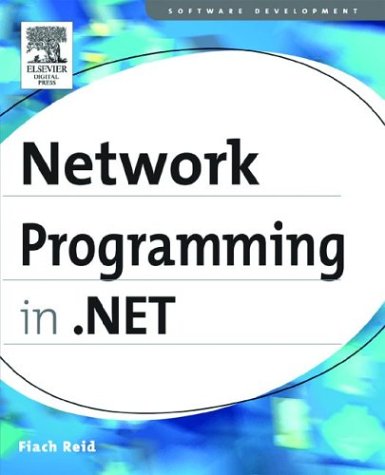
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColorPalette.cs
- TextBlockAutomationPeer.cs
- UnmanagedBitmapWrapper.cs
- DeviceContexts.cs
- HostingPreferredMapPath.cs
- DnsPermission.cs
- ISO2022Encoding.cs
- DataTableTypeConverter.cs
- DocumentPaginator.cs
- CombinedGeometry.cs
- OracleCommand.cs
- FormView.cs
- COAUTHINFO.cs
- ListQueryResults.cs
- MessageRpc.cs
- PackagingUtilities.cs
- HttpException.cs
- ListViewPagedDataSource.cs
- TrustManagerMoreInformation.cs
- XmlSchemaParticle.cs
- Hash.cs
- StrokeRenderer.cs
- TriggerCollection.cs
- LogicalMethodInfo.cs
- X509CertificateClaimSet.cs
- ButtonRenderer.cs
- DataControlFieldCollection.cs
- FrugalMap.cs
- PageRequestManager.cs
- ObjectSecurityT.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ConnectionInterfaceCollection.cs
- RbTree.cs
- pingexception.cs
- returneventsaver.cs
- XslNumber.cs
- NonSerializedAttribute.cs
- DbReferenceCollection.cs
- CodePageUtils.cs
- RangeValidator.cs
- ConditionCollection.cs
- TypeUtils.cs
- StoreAnnotationsMap.cs
- StylusOverProperty.cs
- MetadataConversionError.cs
- ObjectDataSourceEventArgs.cs
- ServiceEndpoint.cs
- MemberInfoSerializationHolder.cs
- FieldCollectionEditor.cs
- InvalidPipelineStoreException.cs
- ObjectStateEntry.cs
- TypeInfo.cs
- EntityException.cs
- XmlQueryRuntime.cs
- UnsafeNativeMethods.cs
- CryptoApi.cs
- ConnectionPointGlyph.cs
- FormatException.cs
- SqlClientWrapperSmiStream.cs
- ProfilePropertyMetadata.cs
- Validator.cs
- Misc.cs
- CodeCommentStatement.cs
- WmlListAdapter.cs
- Stylesheet.cs
- GridEntry.cs
- WeakKeyDictionary.cs
- EndpointAddressProcessor.cs
- ThousandthOfEmRealPoints.cs
- SecurityTokenValidationException.cs
- XmlConvert.cs
- InputLanguageSource.cs
- StoreContentChangedEventArgs.cs
- MailHeaderInfo.cs
- CodeDelegateCreateExpression.cs
- Token.cs
- StylusButtonCollection.cs
- OdbcConnectionHandle.cs
- ObjectResult.cs
- InheritanceAttribute.cs
- NativeMethods.cs
- BoolExpr.cs
- RemotingConfiguration.cs
- QilNode.cs
- TemplateDefinition.cs
- LinkDescriptor.cs
- AtomParser.cs
- CookieProtection.cs
- DataChangedEventManager.cs
- Reference.cs
- ArgumentOutOfRangeException.cs
- CollectionChangedEventManager.cs
- PromptStyle.cs
- ServiceThrottlingElement.cs
- ScrollItemPattern.cs
- ExpressionBuilderCollection.cs
- ImageAttributes.cs
- TextEditorMouse.cs
- DbParameterCollection.cs
- NamespaceTable.cs