Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / DataControlFieldCollection.cs / 1 / DataControlFieldCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataControlFieldCollection : StateManagedCollection { private static readonly Type[] knownTypes = new Type[] { typeof(BoundField), typeof(ButtonField), typeof(CheckBoxField), typeof(CommandField), typeof(HyperLinkField), typeof(ImageField), typeof(TemplateField) }; public event EventHandler FieldsChanged; ///Represents the collection of fields to be displayed in /// a data bound control that uses fields. ////// [ Browsable(false) ] public DataControlField this[int index] { get { return ((IList)this)[index] as DataControlField; } } ///Gets a ///at the specified index in the /// collection. /// public void Add(DataControlField field) { ((IList)this).Add(field); } ///Appends a ///to the collection. /// public DataControlFieldCollection CloneFields() { DataControlFieldCollection fields = new DataControlFieldCollection(); foreach (DataControlField field in this) { fields.Add(field.CloneField()); } return fields; } ///Provides a deep copy of the collection. Used mainly by design time dialogs to implement "cancel" rollback behavior. ////// public bool Contains(DataControlField field) { return ((IList)this).Contains(field); } ///Returns whether a DataControlField is a member of the collection. ////// public void CopyTo(DataControlField[] array, int index) { ((IList)this).CopyTo(array, index); return; } ///Copies the contents of the entire collection into an ///appending at /// the specified index of the . /// protected override object CreateKnownType(int index) { switch (index) { case 0: return new BoundField(); case 1: return new ButtonField(); case 2: return new CheckBoxField(); case 3: return new CommandField(); case 4: return new HyperLinkField(); case 5: return new ImageField(); case 6: return new TemplateField(); default: throw new ArgumentOutOfRangeException(SR.GetString(SR.DataControlFieldCollection_InvalidTypeIndex)); } } ///Creates a known type of DataControlField. ////// protected override Type[] GetKnownTypes() { return knownTypes; } ///Returns an ArrayList of known DataControlField types. ////// public int IndexOf(DataControlField field) { return ((IList)this).IndexOf(field); } ///Returns the index of the first occurrence of a value in a ///. /// public void Insert(int index, DataControlField field) { ((IList)this).Insert(index, field); } ///Inserts a ///to the collection /// at the specified index. /// Called when the Clear() method is complete. /// protected override void OnClearComplete() { OnFieldsChanged(); } ////// void OnFieldChanged(object sender, EventArgs e) { OnFieldsChanged(); } ////// void OnFieldsChanged() { if (FieldsChanged != null) { FieldsChanged(this, EventArgs.Empty); } } ////// Called when the Insert() method is complete. /// protected override void OnInsertComplete(int index, object value) { DataControlField field = value as DataControlField; if (field != null) { field.FieldChanged += new EventHandler(OnFieldChanged); } OnFieldsChanged(); } ////// Called when the Remove() method is complete. /// protected override void OnRemoveComplete(int index, object value) { DataControlField field = value as DataControlField; if (field != null) { field.FieldChanged -= new EventHandler(OnFieldChanged); } OnFieldsChanged(); } ////// protected override void OnValidate(object o) { base.OnValidate(o); if (!(o is DataControlField)) throw new ArgumentException(SR.GetString(SR.DataControlFieldCollection_InvalidType)); } ///Validates that an object is a HotSpot. ////// public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } ///Removes a ///from the collection at the specified /// index. /// public void Remove(DataControlField field) { ((IList)this).Remove(field); } ///Removes the specified ///from the collection. /// protected override void SetDirtyObject(object o) { ((DataControlField)o).SetDirty(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //Marks a DataControlField as dirty so that it will record its entire state into view state. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataControlFieldCollection : StateManagedCollection { private static readonly Type[] knownTypes = new Type[] { typeof(BoundField), typeof(ButtonField), typeof(CheckBoxField), typeof(CommandField), typeof(HyperLinkField), typeof(ImageField), typeof(TemplateField) }; public event EventHandler FieldsChanged; ///Represents the collection of fields to be displayed in /// a data bound control that uses fields. ////// [ Browsable(false) ] public DataControlField this[int index] { get { return ((IList)this)[index] as DataControlField; } } ///Gets a ///at the specified index in the /// collection. /// public void Add(DataControlField field) { ((IList)this).Add(field); } ///Appends a ///to the collection. /// public DataControlFieldCollection CloneFields() { DataControlFieldCollection fields = new DataControlFieldCollection(); foreach (DataControlField field in this) { fields.Add(field.CloneField()); } return fields; } ///Provides a deep copy of the collection. Used mainly by design time dialogs to implement "cancel" rollback behavior. ////// public bool Contains(DataControlField field) { return ((IList)this).Contains(field); } ///Returns whether a DataControlField is a member of the collection. ////// public void CopyTo(DataControlField[] array, int index) { ((IList)this).CopyTo(array, index); return; } ///Copies the contents of the entire collection into an ///appending at /// the specified index of the . /// protected override object CreateKnownType(int index) { switch (index) { case 0: return new BoundField(); case 1: return new ButtonField(); case 2: return new CheckBoxField(); case 3: return new CommandField(); case 4: return new HyperLinkField(); case 5: return new ImageField(); case 6: return new TemplateField(); default: throw new ArgumentOutOfRangeException(SR.GetString(SR.DataControlFieldCollection_InvalidTypeIndex)); } } ///Creates a known type of DataControlField. ////// protected override Type[] GetKnownTypes() { return knownTypes; } ///Returns an ArrayList of known DataControlField types. ////// public int IndexOf(DataControlField field) { return ((IList)this).IndexOf(field); } ///Returns the index of the first occurrence of a value in a ///. /// public void Insert(int index, DataControlField field) { ((IList)this).Insert(index, field); } ///Inserts a ///to the collection /// at the specified index. /// Called when the Clear() method is complete. /// protected override void OnClearComplete() { OnFieldsChanged(); } ////// void OnFieldChanged(object sender, EventArgs e) { OnFieldsChanged(); } ////// void OnFieldsChanged() { if (FieldsChanged != null) { FieldsChanged(this, EventArgs.Empty); } } ////// Called when the Insert() method is complete. /// protected override void OnInsertComplete(int index, object value) { DataControlField field = value as DataControlField; if (field != null) { field.FieldChanged += new EventHandler(OnFieldChanged); } OnFieldsChanged(); } ////// Called when the Remove() method is complete. /// protected override void OnRemoveComplete(int index, object value) { DataControlField field = value as DataControlField; if (field != null) { field.FieldChanged -= new EventHandler(OnFieldChanged); } OnFieldsChanged(); } ////// protected override void OnValidate(object o) { base.OnValidate(o); if (!(o is DataControlField)) throw new ArgumentException(SR.GetString(SR.DataControlFieldCollection_InvalidType)); } ///Validates that an object is a HotSpot. ////// public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } ///Removes a ///from the collection at the specified /// index. /// public void Remove(DataControlField field) { ((IList)this).Remove(field); } ///Removes the specified ///from the collection. /// protected override void SetDirtyObject(object o) { ((DataControlField)o).SetDirty(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Marks a DataControlField as dirty so that it will record its entire state into view state. ///
Link Menu
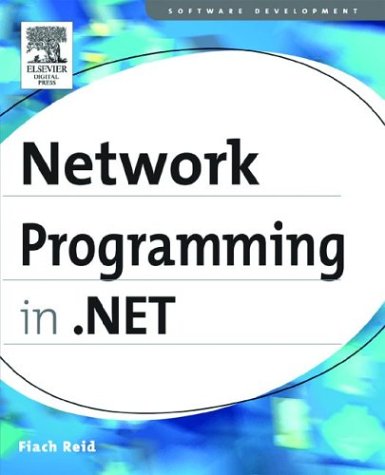
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathNodeInfoAtom.cs
- Encoding.cs
- ExecutionProperties.cs
- EditorResources.cs
- WebPartPersonalization.cs
- DataServiceQueryOfT.cs
- TraceListener.cs
- CookieProtection.cs
- SwitchLevelAttribute.cs
- SchemaImporterExtensionElementCollection.cs
- HandleCollector.cs
- InstalledFontCollection.cs
- HMACSHA1.cs
- HttpApplicationFactory.cs
- ScrollPattern.cs
- Material.cs
- FormViewDesigner.cs
- Int16Animation.cs
- NavigationPropertyEmitter.cs
- PrincipalPermission.cs
- DocumentViewerBase.cs
- ControlValuePropertyAttribute.cs
- TableStyle.cs
- DoWorkEventArgs.cs
- SHA1.cs
- DatatypeImplementation.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- ComponentSerializationService.cs
- Variant.cs
- PieceNameHelper.cs
- EUCJPEncoding.cs
- FusionWrap.cs
- NamespaceMapping.cs
- TreeChangeInfo.cs
- PowerModeChangedEventArgs.cs
- TrustManagerPromptUI.cs
- SiteMapNode.cs
- Material.cs
- TextParaClient.cs
- WindowsListViewGroup.cs
- TagPrefixCollection.cs
- InputChannelBinder.cs
- ContextBase.cs
- SqlInfoMessageEvent.cs
- PropertyChangingEventArgs.cs
- EnumValAlphaComparer.cs
- RetrieveVirtualItemEventArgs.cs
- CodeObject.cs
- BitmapImage.cs
- DataTableMappingCollection.cs
- DataGridViewSortCompareEventArgs.cs
- ContainerControl.cs
- CellNormalizer.cs
- COM2FontConverter.cs
- Enum.cs
- AppDomainProtocolHandler.cs
- PrinterUnitConvert.cs
- TableLayoutRowStyleCollection.cs
- TextBox.cs
- SqlTypesSchemaImporter.cs
- FixUpCollection.cs
- CellConstantDomain.cs
- DeclaredTypeValidatorAttribute.cs
- BrowserDefinition.cs
- ExpandSegmentCollection.cs
- ExtendedPropertiesHandler.cs
- Vector3dCollection.cs
- DeviceContexts.cs
- HandlerElementCollection.cs
- UnhandledExceptionEventArgs.cs
- HttpApplication.cs
- Environment.cs
- NoneExcludedImageIndexConverter.cs
- AuthorizationRule.cs
- BCryptSafeHandles.cs
- CustomCredentialPolicy.cs
- HelpExampleGenerator.cs
- EntitySetDataBindingList.cs
- ExceptionUtil.cs
- CompilerGeneratedAttribute.cs
- InputProviderSite.cs
- TrackingRecord.cs
- DataGridViewSelectedCellCollection.cs
- LineVisual.cs
- XmlSchemaInferenceException.cs
- LinkDescriptor.cs
- DelegateSerializationHolder.cs
- sqlinternaltransaction.cs
- LinkButton.cs
- ContainerCodeDomSerializer.cs
- FixedLineResult.cs
- Aes.cs
- ActivityDesignerHighlighter.cs
- CodeArrayCreateExpression.cs
- MenuBase.cs
- InputScope.cs
- DataGridViewRowCollection.cs
- Psha1DerivedKeyGenerator.cs
- SerialPort.cs
- Substitution.cs