Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / BoolLiteral.cs / 2 / BoolLiteral.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Linq; namespace System.Data.Mapping.ViewGeneration.Structures { using DomainBoolExpr = BoolExpr>; using DomainNotExpr = NotExpr >; using DomainTermExpr = TermExpr >; // A class that "ties" up all the literals in boolean expressions -- // e.g., OneOfConst and CellIdBoolean derive from it // Conditions represented by BoolLiterals need to be synchronized with DomainConstraints, // which may be modified upon calling ExpensiveSimplify. This is what // the method FixRange is used for. internal abstract class BoolLiteral : InternalBase { #region Fields internal static readonly IEqualityComparer EqualityComparer = new BoolLiteralComparer(); internal static readonly IEqualityComparer EqualityIdentifierComparer = new IdentifierComparer(); #endregion #region methods // effects: Creates a term expression of the form: "literal in range with all possible values being domain" internal static DomainTermExpr MakeTermExpression(BoolLiteral literal, IEnumerable domain, IEnumerable range) { Set domainSet = new Set (domain, CellConstant.EqualityComparer); domainSet.MakeReadOnly(); DomainVariable variable = new DomainVariable (literal, domainSet, EqualityIdentifierComparer); Set rangeSet = new Set (range, CellConstant.EqualityComparer); rangeSet.MakeReadOnly(); DomainConstraint constraint = new DomainConstraint (variable, rangeSet); DomainTermExpr result = new DomainTermExpr(EqualityComparer >.Default, constraint); return result; } // effects: Fixes the range of this using the new values provided in // range and returns a boolean expression corresponding to the new value internal abstract DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap); internal abstract DomainBoolExpr GetDomainBoolExpression(MemberDomainMap domainMap); // effects: See BoolExpression.RemapBool internal abstract BoolLiteral RemapBool(Dictionary remap); // effects: See BoolExpression.GetRequiredSlots internal abstract void GetRequiredSlots(MemberPathMapBase projectedSlotMap, bool[] requiredSlots); // effects: See BoolExpression.AsCql internal abstract StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull); internal abstract StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull); internal abstract StringBuilder AsNegatedUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull); // effects: Checks if the identifier in this is the same as the one in right protected virtual bool IsIdentifierEqualTo(BoolLiteral right) { return IsEqualTo(right); } // effects: Get the hash code based on the identifier protected virtual int GetIdentifierHash() { return GetHash(); } protected abstract bool IsEqualTo(BoolLiteral right); protected abstract int GetHash(); #endregion #region Comparer class // This class compares boolean expressions private class BoolLiteralComparer : IEqualityComparer { public bool Equals(BoolLiteral left, BoolLiteral right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsEqualTo(right); } public int GetHashCode(BoolLiteral literal) { return literal.GetHash(); } } #endregion #region Identifier Comparer class // This class compares just the identifier in boolean expressions private class IdentifierComparer : IEqualityComparer { public bool Equals(BoolLiteral left, BoolLiteral right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsIdentifierEqualTo(right); } public int GetHashCode(BoolLiteral literal) { return literal.GetIdentifierHash(); } } #endregion } internal abstract class TrueFalseLiteral : BoolLiteral { internal override DomainBoolExpr GetDomainBoolExpression(MemberDomainMap domainMap) { // Essentially say that the variable can take values true or false and here its value is only true IEnumerable actualValues = new CellConstant[] { new ScalarConstant(true) }; IEnumerable possibleValues = new CellConstant[] { new ScalarConstant(true), new ScalarConstant(false) }; Set variableDomain = new Set (possibleValues, CellConstant.EqualityComparer).MakeReadOnly(); Set thisDomain = new Set (actualValues, CellConstant.EqualityComparer).MakeReadOnly(); DomainTermExpr result = MakeTermExpression(this, variableDomain, thisDomain); return result; } // effects: Fixes the range of this using the new values provided in // range and returns a boolean expression corresponding to the new value internal override DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap) { Debug.Assert(range.Count == 1, "For BoolLiterals, there should be precisely one value - true or false"); ScalarConstant scalar = (ScalarConstant)range.First(); DomainBoolExpr expr = GetDomainBoolExpression(memberDomainMap); if ((bool)scalar.Value == false) { // The range of the variable was "inverted". Return a NOT of // the expression expr = new DomainNotExpr(expr); } return expr; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Linq; namespace System.Data.Mapping.ViewGeneration.Structures { using DomainBoolExpr = BoolExpr>; using DomainNotExpr = NotExpr >; using DomainTermExpr = TermExpr >; // A class that "ties" up all the literals in boolean expressions -- // e.g., OneOfConst and CellIdBoolean derive from it // Conditions represented by BoolLiterals need to be synchronized with DomainConstraints, // which may be modified upon calling ExpensiveSimplify. This is what // the method FixRange is used for. internal abstract class BoolLiteral : InternalBase { #region Fields internal static readonly IEqualityComparer EqualityComparer = new BoolLiteralComparer(); internal static readonly IEqualityComparer EqualityIdentifierComparer = new IdentifierComparer(); #endregion #region methods // effects: Creates a term expression of the form: "literal in range with all possible values being domain" internal static DomainTermExpr MakeTermExpression(BoolLiteral literal, IEnumerable domain, IEnumerable range) { Set domainSet = new Set (domain, CellConstant.EqualityComparer); domainSet.MakeReadOnly(); DomainVariable variable = new DomainVariable (literal, domainSet, EqualityIdentifierComparer); Set rangeSet = new Set (range, CellConstant.EqualityComparer); rangeSet.MakeReadOnly(); DomainConstraint constraint = new DomainConstraint (variable, rangeSet); DomainTermExpr result = new DomainTermExpr(EqualityComparer >.Default, constraint); return result; } // effects: Fixes the range of this using the new values provided in // range and returns a boolean expression corresponding to the new value internal abstract DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap); internal abstract DomainBoolExpr GetDomainBoolExpression(MemberDomainMap domainMap); // effects: See BoolExpression.RemapBool internal abstract BoolLiteral RemapBool(Dictionary remap); // effects: See BoolExpression.GetRequiredSlots internal abstract void GetRequiredSlots(MemberPathMapBase projectedSlotMap, bool[] requiredSlots); // effects: See BoolExpression.AsCql internal abstract StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull); internal abstract StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull); internal abstract StringBuilder AsNegatedUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull); // effects: Checks if the identifier in this is the same as the one in right protected virtual bool IsIdentifierEqualTo(BoolLiteral right) { return IsEqualTo(right); } // effects: Get the hash code based on the identifier protected virtual int GetIdentifierHash() { return GetHash(); } protected abstract bool IsEqualTo(BoolLiteral right); protected abstract int GetHash(); #endregion #region Comparer class // This class compares boolean expressions private class BoolLiteralComparer : IEqualityComparer { public bool Equals(BoolLiteral left, BoolLiteral right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsEqualTo(right); } public int GetHashCode(BoolLiteral literal) { return literal.GetHash(); } } #endregion #region Identifier Comparer class // This class compares just the identifier in boolean expressions private class IdentifierComparer : IEqualityComparer { public bool Equals(BoolLiteral left, BoolLiteral right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsIdentifierEqualTo(right); } public int GetHashCode(BoolLiteral literal) { return literal.GetIdentifierHash(); } } #endregion } internal abstract class TrueFalseLiteral : BoolLiteral { internal override DomainBoolExpr GetDomainBoolExpression(MemberDomainMap domainMap) { // Essentially say that the variable can take values true or false and here its value is only true IEnumerable actualValues = new CellConstant[] { new ScalarConstant(true) }; IEnumerable possibleValues = new CellConstant[] { new ScalarConstant(true), new ScalarConstant(false) }; Set variableDomain = new Set (possibleValues, CellConstant.EqualityComparer).MakeReadOnly(); Set thisDomain = new Set (actualValues, CellConstant.EqualityComparer).MakeReadOnly(); DomainTermExpr result = MakeTermExpression(this, variableDomain, thisDomain); return result; } // effects: Fixes the range of this using the new values provided in // range and returns a boolean expression corresponding to the new value internal override DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap) { Debug.Assert(range.Count == 1, "For BoolLiterals, there should be precisely one value - true or false"); ScalarConstant scalar = (ScalarConstant)range.First(); DomainBoolExpr expr = GetDomainBoolExpression(memberDomainMap); if ((bool)scalar.Value == false) { // The range of the variable was "inverted". Return a NOT of // the expression expr = new DomainNotExpr(expr); } return expr; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
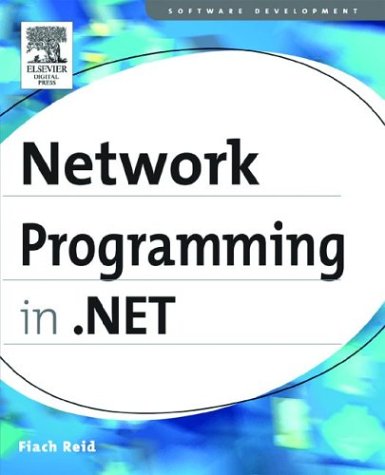
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeDirectoryCompiler.cs
- TypedTableBaseExtensions.cs
- WindowsBrush.cs
- CustomValidator.cs
- OleAutBinder.cs
- EntityKey.cs
- ExpandCollapsePattern.cs
- WebScriptServiceHostFactory.cs
- DataGridViewTextBoxColumn.cs
- FileUtil.cs
- ExpressionBuilder.cs
- WorkerRequest.cs
- VectorCollectionConverter.cs
- HtmlInputRadioButton.cs
- ScaleTransform.cs
- XNodeValidator.cs
- DataGridViewRowPrePaintEventArgs.cs
- ExclusiveCanonicalizationTransform.cs
- Codec.cs
- X509CertificateTrustedIssuerElement.cs
- CapabilitiesSection.cs
- ThumbButtonInfoCollection.cs
- TransformedBitmap.cs
- WebInvokeAttribute.cs
- DefaultMemberAttribute.cs
- DtrList.cs
- HttpCookie.cs
- versioninfo.cs
- DataRowView.cs
- Rect3DValueSerializer.cs
- SerialStream.cs
- CommonDialog.cs
- SystemTcpStatistics.cs
- LocatorManager.cs
- DNS.cs
- HttpClientChannel.cs
- ResourceDisplayNameAttribute.cs
- RecordsAffectedEventArgs.cs
- TabItem.cs
- MergePropertyDescriptor.cs
- ArgumentValidation.cs
- DependencyPropertyChangedEventArgs.cs
- SchemaContext.cs
- PathFigure.cs
- MonthCalendar.cs
- InputProviderSite.cs
- SoapAttributeAttribute.cs
- XmlnsCompatibleWithAttribute.cs
- HttpPostedFileBase.cs
- LocalClientSecuritySettingsElement.cs
- TreeNodeClickEventArgs.cs
- EntityDataSourceContainerNameConverter.cs
- TerminatorSinks.cs
- ScaleTransform.cs
- ObjectConverter.cs
- SessionPageStateSection.cs
- XD.cs
- InvalidOperationException.cs
- AppDomainFactory.cs
- BitmapEffectGroup.cs
- CroppedBitmap.cs
- SamlSecurityToken.cs
- ParserHooks.cs
- TextEditorThreadLocalStore.cs
- FileDetails.cs
- RemoteEndpointMessageProperty.cs
- Matrix3DConverter.cs
- LogicalExpr.cs
- InfocardChannelParameter.cs
- SqlCachedBuffer.cs
- NegotiationTokenAuthenticatorStateCache.cs
- ILGenerator.cs
- SpellerStatusTable.cs
- SerializationFieldInfo.cs
- NonClientArea.cs
- Freezable.cs
- Identity.cs
- HtmlToClrEventProxy.cs
- WhitespaceSignificantCollectionAttribute.cs
- TableRowGroup.cs
- XmlUTF8TextReader.cs
- X509Utils.cs
- MessageUtil.cs
- DateTimeConstantAttribute.cs
- DataContractAttribute.cs
- CountAggregationOperator.cs
- MobileErrorInfo.cs
- ButtonFlatAdapter.cs
- SetState.cs
- PointCollection.cs
- DateTimeParse.cs
- AnimatedTypeHelpers.cs
- EventLogPropertySelector.cs
- DataViewSettingCollection.cs
- DataGridCell.cs
- ExceptionHandlers.cs
- DataGridViewCellStyleConverter.cs
- SettingsSavedEventArgs.cs
- TextTreeRootTextBlock.cs
- SmtpAuthenticationManager.cs