Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebControls / CustomValidator.cs / 1 / CustomValidator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System.Web; using System.Security.Permissions; using System.Web.Util; ////// [ DefaultEvent("ServerValidate"), ToolboxData("<{0}:CustomValidator runat=\"server\" ErrorMessage=\"CustomValidator\">{0}:CustomValidator>") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CustomValidator : BaseValidator { private static readonly object EventServerValidate= new object(); ///Allows custom code to perform /// validation on the client and/or server. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.CustomValidator_ClientValidationFunction) ] public string ClientValidationFunction { get { object o = ViewState["ClientValidationFunction"]; return((o == null) ? String.Empty : (string)o); } set { ViewState["ClientValidationFunction"] = value; } } [ WebCategory("Behavior"), Themeable(false), DefaultValue(false), WebSysDescription(SR.CustomValidator_ValidateEmptyText), ] public bool ValidateEmptyText { get { object o = ViewState["ValidateEmptyText"]; return((o == null) ? false : (bool)o); } set { ViewState["ValidateEmptyText"] = value; } } ///Gets and sets the custom client Javascript function used /// for validation. ////// [ WebSysDescription(SR.CustomValidator_ServerValidate) ] public event ServerValidateEventHandler ServerValidate { add { Events.AddHandler(EventServerValidate, value); } remove { Events.RemoveHandler(EventServerValidate, value); } } ///Represents the method that will handle the /// ///event of a /// . /// /// protected override void AddAttributesToRender(HtmlTextWriter writer) { base.AddAttributesToRender(writer); if (RenderUplevel) { string id = ClientID; HtmlTextWriter expandoAttributeWriter = (EnableLegacyRendering) ? writer : null; AddExpandoAttribute(expandoAttributeWriter, id, "evaluationfunction", "CustomValidatorEvaluateIsValid", false); if (ClientValidationFunction.Length > 0) { AddExpandoAttribute(expandoAttributeWriter, id, "clientvalidationfunction", ClientValidationFunction); if (ValidateEmptyText) { AddExpandoAttribute(expandoAttributeWriter, id, "validateemptytext", "true", false); } } } } ///Adds the properties of the ///control to the /// output stream for rendering on the client. /// /// protected override bool ControlPropertiesValid() { // Need to override the BaseValidator implementation, because for CustomValidator, it is fine // for the ControlToValidate to be blank. string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { // Check that the property points to a valid control. Will throw and exception if not found CheckControlValidationProperty(controlToValidate, "ControlToValidate"); } return true; } ///Checks the properties of the control for valid values. ////// /// EvaluateIsValid method /// protected override bool EvaluateIsValid() { // If no control is specified, we always fire the event. If they have specified a control, we // only fire the event if the input is non-blank. string controlValue = String.Empty; string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { controlValue = GetControlValidationValue(controlToValidate); Debug.Assert(controlValue != null, "Should have been caught be property check"); // If the text is empty, we return true. Whitespace is ignored for coordination wiht // RequiredFieldValidator. if ((controlValue == null || controlValue.Trim().Length == 0) && !ValidateEmptyText) { return true; } } return OnServerValidate(controlValue); } ////// protected virtual bool OnServerValidate(string value) { ServerValidateEventHandler handler = (ServerValidateEventHandler)Events[EventServerValidate]; ServerValidateEventArgs args = new ServerValidateEventArgs(value, true); if (handler != null) { handler(this, args); return args.IsValid; } else { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Raises the /// ///event for the . // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System.Web; using System.Security.Permissions; using System.Web.Util; ////// [ DefaultEvent("ServerValidate"), ToolboxData("<{0}:CustomValidator runat=\"server\" ErrorMessage=\"CustomValidator\">{0}:CustomValidator>") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CustomValidator : BaseValidator { private static readonly object EventServerValidate= new object(); ///Allows custom code to perform /// validation on the client and/or server. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.CustomValidator_ClientValidationFunction) ] public string ClientValidationFunction { get { object o = ViewState["ClientValidationFunction"]; return((o == null) ? String.Empty : (string)o); } set { ViewState["ClientValidationFunction"] = value; } } [ WebCategory("Behavior"), Themeable(false), DefaultValue(false), WebSysDescription(SR.CustomValidator_ValidateEmptyText), ] public bool ValidateEmptyText { get { object o = ViewState["ValidateEmptyText"]; return((o == null) ? false : (bool)o); } set { ViewState["ValidateEmptyText"] = value; } } ///Gets and sets the custom client Javascript function used /// for validation. ////// [ WebSysDescription(SR.CustomValidator_ServerValidate) ] public event ServerValidateEventHandler ServerValidate { add { Events.AddHandler(EventServerValidate, value); } remove { Events.RemoveHandler(EventServerValidate, value); } } ///Represents the method that will handle the /// ///event of a /// . /// /// protected override void AddAttributesToRender(HtmlTextWriter writer) { base.AddAttributesToRender(writer); if (RenderUplevel) { string id = ClientID; HtmlTextWriter expandoAttributeWriter = (EnableLegacyRendering) ? writer : null; AddExpandoAttribute(expandoAttributeWriter, id, "evaluationfunction", "CustomValidatorEvaluateIsValid", false); if (ClientValidationFunction.Length > 0) { AddExpandoAttribute(expandoAttributeWriter, id, "clientvalidationfunction", ClientValidationFunction); if (ValidateEmptyText) { AddExpandoAttribute(expandoAttributeWriter, id, "validateemptytext", "true", false); } } } } ///Adds the properties of the ///control to the /// output stream for rendering on the client. /// /// protected override bool ControlPropertiesValid() { // Need to override the BaseValidator implementation, because for CustomValidator, it is fine // for the ControlToValidate to be blank. string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { // Check that the property points to a valid control. Will throw and exception if not found CheckControlValidationProperty(controlToValidate, "ControlToValidate"); } return true; } ///Checks the properties of the control for valid values. ////// /// EvaluateIsValid method /// protected override bool EvaluateIsValid() { // If no control is specified, we always fire the event. If they have specified a control, we // only fire the event if the input is non-blank. string controlValue = String.Empty; string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { controlValue = GetControlValidationValue(controlToValidate); Debug.Assert(controlValue != null, "Should have been caught be property check"); // If the text is empty, we return true. Whitespace is ignored for coordination wiht // RequiredFieldValidator. if ((controlValue == null || controlValue.Trim().Length == 0) && !ValidateEmptyText) { return true; } } return OnServerValidate(controlValue); } ////// protected virtual bool OnServerValidate(string value) { ServerValidateEventHandler handler = (ServerValidateEventHandler)Events[EventServerValidate]; ServerValidateEventArgs args = new ServerValidateEventArgs(value, true); if (handler != null) { handler(this, args); return args.IsValid; } else { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Raises the /// ///event for the .
Link Menu
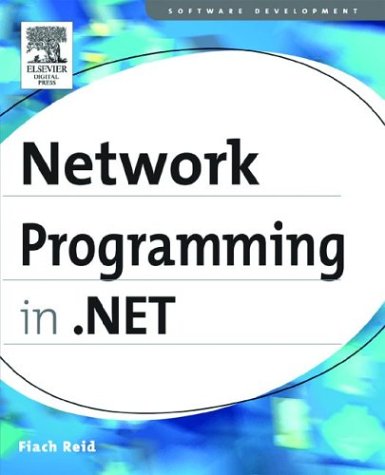
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MethodToken.cs
- EnvelopedPkcs7.cs
- ApplicationFileParser.cs
- OperationCanceledException.cs
- SoapAttributeOverrides.cs
- ChannelPool.cs
- OracleTimeSpan.cs
- WmlValidatorAdapter.cs
- FontSourceCollection.cs
- VersionUtil.cs
- ObsoleteAttribute.cs
- designeractionlistschangedeventargs.cs
- CompilerCollection.cs
- XmlSchemaValidator.cs
- ListViewItemSelectionChangedEvent.cs
- Attributes.cs
- PageRanges.cs
- ButtonFieldBase.cs
- DataTableMappingCollection.cs
- WebRequestModuleElementCollection.cs
- Exceptions.cs
- CharEntityEncoderFallback.cs
- PerfCounters.cs
- ObjectHandle.cs
- AddInControllerImpl.cs
- SchemaReference.cs
- ConnectionManagementSection.cs
- InputProcessorProfiles.cs
- WebPartTransformerAttribute.cs
- sqlpipe.cs
- SqlServer2KCompatibilityCheck.cs
- BindingList.cs
- XmlTextAttribute.cs
- HttpCacheVaryByContentEncodings.cs
- CaseInsensitiveOrdinalStringComparer.cs
- BrowserCapabilitiesFactoryBase.cs
- __Error.cs
- SharedPerformanceCounter.cs
- FactoryId.cs
- CompiledRegexRunner.cs
- NativeMethodsCLR.cs
- StringResourceManager.cs
- ElementHost.cs
- DPTypeDescriptorContext.cs
- FtpWebRequest.cs
- ProcessInfo.cs
- PageCatalogPart.cs
- StateMachineSubscriptionManager.cs
- RequiredFieldValidator.cs
- DoubleLink.cs
- CatalogZone.cs
- StyleCollection.cs
- OutputCacheModule.cs
- __ConsoleStream.cs
- COM2Properties.cs
- GlyphRunDrawing.cs
- CircleHotSpot.cs
- RightNameExpirationInfoPair.cs
- SqlParameterCollection.cs
- MULTI_QI.cs
- StylusCollection.cs
- GregorianCalendar.cs
- WebEventTraceProvider.cs
- GridPattern.cs
- ExpressionList.cs
- ADConnectionHelper.cs
- DataGridViewSelectedRowCollection.cs
- NavigationExpr.cs
- BasicExpressionVisitor.cs
- ValueQuery.cs
- FacetDescription.cs
- RadioButton.cs
- TextPointer.cs
- SqlCacheDependencyDatabaseCollection.cs
- IndexOutOfRangeException.cs
- ListBoxAutomationPeer.cs
- AuthStoreRoleProvider.cs
- HtmlDocument.cs
- MouseGestureValueSerializer.cs
- TheQuery.cs
- XamlTreeBuilderBamlRecordWriter.cs
- Rfc2898DeriveBytes.cs
- WinCategoryAttribute.cs
- SimpleBitVector32.cs
- DbException.cs
- DesignerForm.cs
- CodeTypeMemberCollection.cs
- Misc.cs
- NumericUpDown.cs
- NavigationService.cs
- NamedPermissionSet.cs
- LogRestartAreaEnumerator.cs
- AssemblyUtil.cs
- CaseKeyBox.ViewModel.cs
- TargetException.cs
- SmtpClient.cs
- MaterialGroup.cs
- ClassGenerator.cs
- ProcessModelInfo.cs
- ToolStripGripRenderEventArgs.cs