Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / FacetDescription.cs / 4 / FacetDescription.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; using System.Diagnostics; using System.Threading; namespace System.Data.Metadata.Edm { ////// Class for representing a FacetDescription object /// public sealed class FacetDescription { #region Constructors internal FacetDescription(string facetName, EdmType facetType, int? minValue, int? maxValue, object defaultValue, bool isConstant, string declaringTypeName) { _facetName = facetName; _facetType = facetType; _minValue = minValue; _maxValue = maxValue; // this ctor doesn't allow you to set the defaultValue to null if (defaultValue != null) { _defaultValue = defaultValue; } else { _defaultValue = _notInitializedSentinel; } _isConstant = isConstant; Validate(declaringTypeName); if (_isConstant) { UpdateMinMaxValueForConstant(_facetName, _facetType, ref _minValue, ref _maxValue, _defaultValue); } } ////// The constructor for constructing a facet description object /// /// The name of this facet /// The type of this facet /// The min value for this facet /// The max value for this facet /// The default value for this facet ///Thrown if either facetName, facetType or applicableType arguments are null internal FacetDescription(string facetName, EdmType facetType, int? minValue, int? maxValue, object defaultValue) { EntityUtil.CheckStringArgument(facetName, "facetName"); EntityUtil.GenericCheckArgumentNull(facetType, "facetType"); if (minValue.HasValue || maxValue.HasValue) { Debug.Assert(FacetDescription.IsNumericType(facetType), "Min and Max Values can only be specified for numeric facets"); if (minValue.HasValue && maxValue.HasValue) { Debug.Assert(minValue != maxValue, "minValue should not be equal to maxValue"); } } _facetName = facetName; _facetType = facetType; _minValue = minValue; _maxValue = maxValue; _defaultValue = defaultValue; } #endregion #region Fields private readonly string _facetName; private readonly EdmType _facetType; private readonly int? _minValue; private readonly int? _maxValue; private readonly object _defaultValue; private readonly bool _isConstant; ///A facet with the default value for this description. private Facet _defaultValueFacet; ///A facet with a null value for this description. private Facet _nullValueFacet; ///Type-dependant cache for additional values (possibly null). private Facet[] _valueCache; // we need to differentiate when the default value is null vs when the default value is not initialized private static object _notInitializedSentinel = new object(); #endregion #region Properties ////// Gets the name of this facet /// public string FacetName { get { return _facetName; } } ////// Gets the type of this facet /// public EdmType FacetType { get { return _facetType; } } ////// Gets the lower bound a facet with this facet description can take /// public int? MinValue { get { return _minValue; } } ////// Gets the upper bound a facet with this facet description can take /// public int? MaxValue { get { return _maxValue; } } ////// Gets the default value of a facet with this facet description /// public object DefaultValue { get { if (_defaultValue == _notInitializedSentinel) { return null; } return _defaultValue; } } ////// Gets whether the value of this facet must be constant /// public bool IsConstant { get { return _isConstant; } } ////// Gets whether this facet is a required facet or not /// public bool IsRequired { get { return _defaultValue == _notInitializedSentinel; } } #region Internal properties. ////// Gets a facet with the default value for this description. /// internal Facet DefaultValueFacet { get { if (_defaultValueFacet == null) { Facet defaultValueFacet = Facet.Create(this, this.DefaultValue, true); Interlocked.CompareExchange(ref _defaultValueFacet, defaultValueFacet, null); } return _defaultValueFacet; } } ////// Gets a facet with a null value for this description. /// internal Facet NullValueFacet { get { if (_nullValueFacet == null) { Facet nullValueFacet = Facet.Create(this, null, true); Interlocked.CompareExchange(ref _nullValueFacet, nullValueFacet, null); } return _nullValueFacet; } } #endregion Internal properties. #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.FacetName; } ////// Gets a cached facet instance with the specified boolean value. /// /// Value for the Facet result. ///A cached facet instance with the specified boolean value. internal Facet GetBooleanFacet(bool value) { System.Diagnostics.Debug.Assert(this.FacetType.Identity == "Edm.Boolean"); if (_valueCache == null) { Facet[] valueCache = new Facet[2]; valueCache[0] = Facet.Create(this, true, true); valueCache[1] = Facet.Create(this, false, true); System.Threading.Interlocked.CompareExchange( ref _valueCache, valueCache, null ); } return (value) ? _valueCache[0] : _valueCache[1]; } ////// Returns true if the facet type is of numeric type /// /// Type of the facet ///internal static bool IsNumericType(EdmType facetType) { if (Helper.IsPrimitiveType(facetType)) { PrimitiveType primitiveType = (PrimitiveType)facetType; return primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.Byte || primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.SByte || primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.Int16 || primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.Int32; } return false; } private static void UpdateMinMaxValueForConstant(string facetName, EdmType facetType, ref int? minValue, ref int? maxValue, object defaultValue) { if (FacetDescription.IsNumericType(facetType)) { if (facetName == EdmProviderManifest.PrecisionFacetName || facetName == EdmProviderManifest.ScaleFacetName) { minValue = (int?)((byte?)defaultValue); maxValue = (int?)((byte?)defaultValue); } else { minValue = (int?)defaultValue; maxValue = (int?)defaultValue; } } } private void Validate(string declaringTypeName) { if (_defaultValue == _notInitializedSentinel) { if (_isConstant) { throw EntityUtil.MissingDefaultValueForConstantFacet(_facetName, declaringTypeName); } } else if (FacetDescription.IsNumericType(_facetType)) { if (_isConstant) { // Either both of them are not specified or both of them have the same value if ((_minValue.HasValue != _maxValue.HasValue) || (_minValue.HasValue && _minValue.Value != _maxValue.Value)) { throw EntityUtil.MinAndMaxValueMustBeSameForConstantFacet(_facetName, declaringTypeName); } } // If its not constant, then both of the minValue and maxValue must be specified else if (!_minValue.HasValue || !_maxValue.HasValue) { throw EntityUtil.BothMinAndMaxValueMustBeSpecifiedForNonConstantFacet(_facetName, declaringTypeName); } else if (_minValue.Value == _maxValue) { throw EntityUtil.MinAndMaxValueMustBeDifferentForNonConstantFacet(_facetName, declaringTypeName); } else if (_minValue < 0 || _maxValue < 0) { throw EntityUtil.MinAndMaxMustBePositive(_facetName, declaringTypeName); } else if (_minValue > _maxValue) { throw EntityUtil.MinMustBeLessThanMax(_minValue.ToString(), _facetName, declaringTypeName); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; using System.Diagnostics; using System.Threading; namespace System.Data.Metadata.Edm { ////// Class for representing a FacetDescription object /// public sealed class FacetDescription { #region Constructors internal FacetDescription(string facetName, EdmType facetType, int? minValue, int? maxValue, object defaultValue, bool isConstant, string declaringTypeName) { _facetName = facetName; _facetType = facetType; _minValue = minValue; _maxValue = maxValue; // this ctor doesn't allow you to set the defaultValue to null if (defaultValue != null) { _defaultValue = defaultValue; } else { _defaultValue = _notInitializedSentinel; } _isConstant = isConstant; Validate(declaringTypeName); if (_isConstant) { UpdateMinMaxValueForConstant(_facetName, _facetType, ref _minValue, ref _maxValue, _defaultValue); } } ////// The constructor for constructing a facet description object /// /// The name of this facet /// The type of this facet /// The min value for this facet /// The max value for this facet /// The default value for this facet ///Thrown if either facetName, facetType or applicableType arguments are null internal FacetDescription(string facetName, EdmType facetType, int? minValue, int? maxValue, object defaultValue) { EntityUtil.CheckStringArgument(facetName, "facetName"); EntityUtil.GenericCheckArgumentNull(facetType, "facetType"); if (minValue.HasValue || maxValue.HasValue) { Debug.Assert(FacetDescription.IsNumericType(facetType), "Min and Max Values can only be specified for numeric facets"); if (minValue.HasValue && maxValue.HasValue) { Debug.Assert(minValue != maxValue, "minValue should not be equal to maxValue"); } } _facetName = facetName; _facetType = facetType; _minValue = minValue; _maxValue = maxValue; _defaultValue = defaultValue; } #endregion #region Fields private readonly string _facetName; private readonly EdmType _facetType; private readonly int? _minValue; private readonly int? _maxValue; private readonly object _defaultValue; private readonly bool _isConstant; ///A facet with the default value for this description. private Facet _defaultValueFacet; ///A facet with a null value for this description. private Facet _nullValueFacet; ///Type-dependant cache for additional values (possibly null). private Facet[] _valueCache; // we need to differentiate when the default value is null vs when the default value is not initialized private static object _notInitializedSentinel = new object(); #endregion #region Properties ////// Gets the name of this facet /// public string FacetName { get { return _facetName; } } ////// Gets the type of this facet /// public EdmType FacetType { get { return _facetType; } } ////// Gets the lower bound a facet with this facet description can take /// public int? MinValue { get { return _minValue; } } ////// Gets the upper bound a facet with this facet description can take /// public int? MaxValue { get { return _maxValue; } } ////// Gets the default value of a facet with this facet description /// public object DefaultValue { get { if (_defaultValue == _notInitializedSentinel) { return null; } return _defaultValue; } } ////// Gets whether the value of this facet must be constant /// public bool IsConstant { get { return _isConstant; } } ////// Gets whether this facet is a required facet or not /// public bool IsRequired { get { return _defaultValue == _notInitializedSentinel; } } #region Internal properties. ////// Gets a facet with the default value for this description. /// internal Facet DefaultValueFacet { get { if (_defaultValueFacet == null) { Facet defaultValueFacet = Facet.Create(this, this.DefaultValue, true); Interlocked.CompareExchange(ref _defaultValueFacet, defaultValueFacet, null); } return _defaultValueFacet; } } ////// Gets a facet with a null value for this description. /// internal Facet NullValueFacet { get { if (_nullValueFacet == null) { Facet nullValueFacet = Facet.Create(this, null, true); Interlocked.CompareExchange(ref _nullValueFacet, nullValueFacet, null); } return _nullValueFacet; } } #endregion Internal properties. #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.FacetName; } ////// Gets a cached facet instance with the specified boolean value. /// /// Value for the Facet result. ///A cached facet instance with the specified boolean value. internal Facet GetBooleanFacet(bool value) { System.Diagnostics.Debug.Assert(this.FacetType.Identity == "Edm.Boolean"); if (_valueCache == null) { Facet[] valueCache = new Facet[2]; valueCache[0] = Facet.Create(this, true, true); valueCache[1] = Facet.Create(this, false, true); System.Threading.Interlocked.CompareExchange( ref _valueCache, valueCache, null ); } return (value) ? _valueCache[0] : _valueCache[1]; } ////// Returns true if the facet type is of numeric type /// /// Type of the facet ///internal static bool IsNumericType(EdmType facetType) { if (Helper.IsPrimitiveType(facetType)) { PrimitiveType primitiveType = (PrimitiveType)facetType; return primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.Byte || primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.SByte || primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.Int16 || primitiveType.PrimitiveTypeKind == PrimitiveTypeKind.Int32; } return false; } private static void UpdateMinMaxValueForConstant(string facetName, EdmType facetType, ref int? minValue, ref int? maxValue, object defaultValue) { if (FacetDescription.IsNumericType(facetType)) { if (facetName == EdmProviderManifest.PrecisionFacetName || facetName == EdmProviderManifest.ScaleFacetName) { minValue = (int?)((byte?)defaultValue); maxValue = (int?)((byte?)defaultValue); } else { minValue = (int?)defaultValue; maxValue = (int?)defaultValue; } } } private void Validate(string declaringTypeName) { if (_defaultValue == _notInitializedSentinel) { if (_isConstant) { throw EntityUtil.MissingDefaultValueForConstantFacet(_facetName, declaringTypeName); } } else if (FacetDescription.IsNumericType(_facetType)) { if (_isConstant) { // Either both of them are not specified or both of them have the same value if ((_minValue.HasValue != _maxValue.HasValue) || (_minValue.HasValue && _minValue.Value != _maxValue.Value)) { throw EntityUtil.MinAndMaxValueMustBeSameForConstantFacet(_facetName, declaringTypeName); } } // If its not constant, then both of the minValue and maxValue must be specified else if (!_minValue.HasValue || !_maxValue.HasValue) { throw EntityUtil.BothMinAndMaxValueMustBeSpecifiedForNonConstantFacet(_facetName, declaringTypeName); } else if (_minValue.Value == _maxValue) { throw EntityUtil.MinAndMaxValueMustBeDifferentForNonConstantFacet(_facetName, declaringTypeName); } else if (_minValue < 0 || _maxValue < 0) { throw EntityUtil.MinAndMaxMustBePositive(_facetName, declaringTypeName); } else if (_minValue > _maxValue) { throw EntityUtil.MinMustBeLessThanMax(_minValue.ToString(), _facetName, declaringTypeName); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
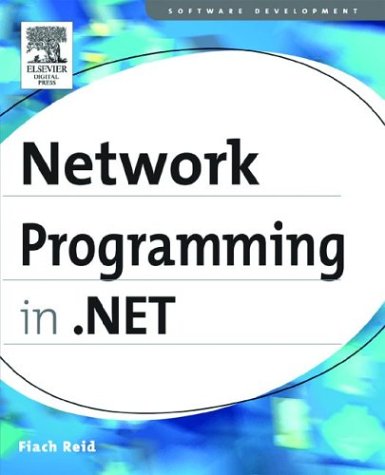
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyConverter.cs
- TreeNodeMouseHoverEvent.cs
- RuntimeConfigLKG.cs
- UnmanagedMemoryAccessor.cs
- Quad.cs
- Variable.cs
- TypeDependencyAttribute.cs
- Base64Stream.cs
- SymmetricAlgorithm.cs
- glyphs.cs
- ReadContentAsBinaryHelper.cs
- ToolConsole.cs
- DynamicActionMessageFilter.cs
- AutomationPropertyInfo.cs
- FontNameEditor.cs
- UIElementPropertyUndoUnit.cs
- XPathEmptyIterator.cs
- SystemIPv6InterfaceProperties.cs
- OpenFileDialog.cs
- EventSinkActivity.cs
- SchemaElement.cs
- MembershipUser.cs
- RoleManagerSection.cs
- PerformanceCounterNameAttribute.cs
- TailCallAnalyzer.cs
- RuleSettings.cs
- CatalogPartChrome.cs
- ValidateNames.cs
- ListComponentEditor.cs
- UserUseLicenseDictionaryLoader.cs
- Bezier.cs
- SourceSwitch.cs
- PrefixHandle.cs
- PtsPage.cs
- CodeDOMUtility.cs
- TimeSpanConverter.cs
- FixedSchema.cs
- ScalarOps.cs
- InternalMappingException.cs
- DataStorage.cs
- Scheduler.cs
- DictionarySectionHandler.cs
- UnsignedPublishLicense.cs
- MarkupCompiler.cs
- TaskScheduler.cs
- LinkArea.cs
- FileSystemInfo.cs
- WorkerRequest.cs
- QueryCursorEventArgs.cs
- ArrayHelper.cs
- MailWebEventProvider.cs
- Wrapper.cs
- ClosableStream.cs
- FrameworkPropertyMetadata.cs
- DockPanel.cs
- SQLMoney.cs
- ControlValuePropertyAttribute.cs
- DocumentCollection.cs
- TreeViewItem.cs
- SQLChars.cs
- QueryOutputWriter.cs
- PrtCap_Builder.cs
- XmlElementAttribute.cs
- DbConnectionClosed.cs
- InvalidMessageContractException.cs
- TimeSpanParse.cs
- ExpressionSelection.cs
- KoreanCalendar.cs
- CapacityStreamGeometryContext.cs
- UIAgentMonitorHandle.cs
- XmlSerializerVersionAttribute.cs
- complextypematerializer.cs
- TrackingMemoryStreamFactory.cs
- JoinGraph.cs
- FontInfo.cs
- ResourceAssociationType.cs
- CallContext.cs
- DataRowExtensions.cs
- Padding.cs
- PerformanceCounterCategory.cs
- SystemIPGlobalProperties.cs
- QilFactory.cs
- CqlLexer.cs
- StringFreezingAttribute.cs
- UrlMapping.cs
- PreservationFileReader.cs
- _SslStream.cs
- HttpRuntime.cs
- ConsumerConnectionPointCollection.cs
- CodeNamespaceImportCollection.cs
- PointCollectionConverter.cs
- cryptoapiTransform.cs
- SmtpReplyReader.cs
- ExceptionRoutedEventArgs.cs
- EditorBrowsableAttribute.cs
- AlignmentYValidation.cs
- ObjectSpanRewriter.cs
- ObfuscateAssemblyAttribute.cs
- MetaTableHelper.cs
- NetDataContractSerializer.cs