Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ButtonRenderer.cs / 1 / ButtonRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class ButtonRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement ButtonElement = VisualStyleElement.Button.PushButton.Normal; private static bool renderMatchingApplicationState = true; //cannot instantiate private ButtonRenderer() { } ////// This is a rendering class for the Button control. It works downlevel too (obviously /// without visual styles applied.) /// ////// /// public static bool RenderMatchingApplicationState { get { return renderMatchingApplicationState; } set { renderMatchingApplicationState = value; } } private static bool RenderWithVisualStyles { get { return (!renderMatchingApplicationState || Application.RenderWithVisualStyles); } } ////// If this property is true, then the renderer will use the setting from Application.RenderWithVisualStyles to /// determine how to render. /// If this property is false, the renderer will always render with visualstyles. /// ////// /// public static bool IsBackgroundPartiallyTransparent(PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); return visualStyleRenderer.IsBackgroundPartiallyTransparent(); } else { return false; //for downlevel, this is false } } ////// Returns true if the background corresponding to the given state is partially transparent, else false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] public static void DrawParentBackground(Graphics g, Rectangle bounds, Control childControl) { if (RenderWithVisualStyles) { InitializeRenderer(0); visualStyleRenderer.DrawParentBackground(g, bounds, childControl); } } ////// This is just a convenience wrapper for VisualStyleRenderer.DrawThemeParentBackground. For downlevel, /// this isn't required and does nothing. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageBounds, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } internal static ButtonState ConvertToButtonState(PushButtonState state) { switch (state) { case PushButtonState.Pressed: return ButtonState.Pushed; case PushButtonState.Disabled: return ButtonState.Inactive; default: return ButtonState.Normal; } } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(ButtonElement.ClassName, ButtonElement.Part, state); } else { visualStyleRenderer.SetParameters(ButtonElement.ClassName, ButtonElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Renders a Button control. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class ButtonRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement ButtonElement = VisualStyleElement.Button.PushButton.Normal; private static bool renderMatchingApplicationState = true; //cannot instantiate private ButtonRenderer() { } ////// This is a rendering class for the Button control. It works downlevel too (obviously /// without visual styles applied.) /// ////// /// public static bool RenderMatchingApplicationState { get { return renderMatchingApplicationState; } set { renderMatchingApplicationState = value; } } private static bool RenderWithVisualStyles { get { return (!renderMatchingApplicationState || Application.RenderWithVisualStyles); } } ////// If this property is true, then the renderer will use the setting from Application.RenderWithVisualStyles to /// determine how to render. /// If this property is false, the renderer will always render with visualstyles. /// ////// /// public static bool IsBackgroundPartiallyTransparent(PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); return visualStyleRenderer.IsBackgroundPartiallyTransparent(); } else { return false; //for downlevel, this is false } } ////// Returns true if the background corresponding to the given state is partially transparent, else false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] public static void DrawParentBackground(Graphics g, Rectangle bounds, Control childControl) { if (RenderWithVisualStyles) { InitializeRenderer(0); visualStyleRenderer.DrawParentBackground(g, bounds, childControl); } } ////// This is just a convenience wrapper for VisualStyleRenderer.DrawThemeParentBackground. For downlevel, /// this isn't required and does nothing. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageBounds, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } internal static ButtonState ConvertToButtonState(PushButtonState state) { switch (state) { case PushButtonState.Pressed: return ButtonState.Pushed; case PushButtonState.Disabled: return ButtonState.Inactive; default: return ButtonState.Normal; } } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(ButtonElement.ClassName, ButtonElement.Part, state); } else { visualStyleRenderer.SetParameters(ButtonElement.ClassName, ButtonElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Renders a Button control. /// ///
Link Menu
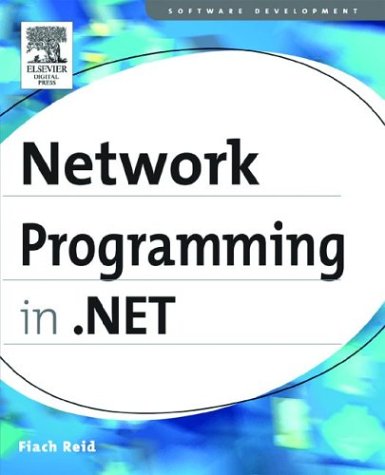
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DrawItemEvent.cs
- DataGridViewTextBoxCell.cs
- NamedObjectList.cs
- StructuredTypeInfo.cs
- RegularExpressionValidator.cs
- CodeArgumentReferenceExpression.cs
- DbDataReader.cs
- TdsParameterSetter.cs
- infer.cs
- VisualStyleElement.cs
- TranslateTransform.cs
- HostedAspNetEnvironment.cs
- OperationValidationEventArgs.cs
- DataGridViewColumnCollectionDialog.cs
- WindowsRegion.cs
- CancellableEnumerable.cs
- TableItemProviderWrapper.cs
- InheritablePropertyChangeInfo.cs
- XLinq.cs
- CodeMethodMap.cs
- StyleSelector.cs
- TemplateInstanceAttribute.cs
- MappingMetadataHelper.cs
- GeometryDrawing.cs
- SafeBuffer.cs
- Formatter.cs
- Shape.cs
- CompensationHandlingFilter.cs
- TypeLoadException.cs
- TransactionState.cs
- ZipPackage.cs
- ProtocolInformationReader.cs
- ChangePassword.cs
- RectAnimationUsingKeyFrames.cs
- ListItemParagraph.cs
- DataFormats.cs
- PointKeyFrameCollection.cs
- ResourceProviderFactory.cs
- PositiveTimeSpanValidator.cs
- Material.cs
- XmlToDatasetMap.cs
- SqlRewriteScalarSubqueries.cs
- StackBuilderSink.cs
- WebPartConnectionsCancelEventArgs.cs
- dbenumerator.cs
- TextPointer.cs
- KnownBoxes.cs
- ExpanderAutomationPeer.cs
- CollectionTypeElement.cs
- MimeMapping.cs
- HatchBrush.cs
- BitmapEffectrendercontext.cs
- WindowsUpDown.cs
- CategoryNameCollection.cs
- EncryptedData.cs
- ControlTemplate.cs
- SrgsRuleRef.cs
- RowVisual.cs
- ContactManager.cs
- Vertex.cs
- EncryptedKey.cs
- DrawingBrush.cs
- CatalogZone.cs
- IdentifierService.cs
- CrossContextChannel.cs
- LowerCaseStringConverter.cs
- ContractReference.cs
- ZoneMembershipCondition.cs
- CheckBoxBaseAdapter.cs
- GridViewRowEventArgs.cs
- SqlUtils.cs
- NavigationFailedEventArgs.cs
- SettingsProperty.cs
- GridViewSelectEventArgs.cs
- TCEAdapterGenerator.cs
- AsynchronousChannelMergeEnumerator.cs
- ReadContentAsBinaryHelper.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- SectionUpdates.cs
- InvalidFilterCriteriaException.cs
- SamlAudienceRestrictionCondition.cs
- RadioButtonAutomationPeer.cs
- SQLResource.cs
- Drawing.cs
- HitTestWithGeometryDrawingContextWalker.cs
- SafeProcessHandle.cs
- FamilyCollection.cs
- xsdvalidator.cs
- Int32AnimationUsingKeyFrames.cs
- XPathItem.cs
- WpfWebRequestHelper.cs
- TableItemPatternIdentifiers.cs
- DataGridToolTip.cs
- PaintEvent.cs
- TextViewBase.cs
- MultiPageTextView.cs
- MdbDataFileEditor.cs
- PackageRelationshipCollection.cs
- MessageContractMemberAttribute.cs
- ObjectViewFactory.cs