Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebParts / Part.cs / 1 / Part.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.ComponentModel; using System.Drawing.Design; using System.Security.Permissions; using System.Web.UI.WebControls; ////// Base class for all Part classes in the WebPart framework. Common to all Part controls are properties that /// allow the control to be rendered in a modular fashion. /// [ Designer("System.Web.UI.Design.WebControls.WebParts.PartDesigner, " + AssemblyRef.SystemDesign), ParseChildren(true), PersistChildren(false), ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class Part : Panel, INamingContainer, ICompositeControlDesignerAccessor { // Prevent class from being subclassed outside of our assembly internal Part() { } ////// The UI state of the part /// [ DefaultValue(PartChromeState.Normal), WebCategory("WebPartAppearance"), WebSysDescription(SR.Part_ChromeState), ] public virtual PartChromeState ChromeState { get { object o = ViewState["ChromeState"]; return (o != null) ? (PartChromeState)o : PartChromeState.Normal; } set { if ((value < PartChromeState.Normal) || (value > PartChromeState.Minimized)) { throw new ArgumentOutOfRangeException("value"); } ViewState["ChromeState"] = value; } } ////// The type of frame/border for the part. /// [ DefaultValue(PartChromeType.Default), WebCategory("WebPartAppearance"), WebSysDescription(SR.Part_ChromeType), ] public virtual PartChromeType ChromeType { get { object o = ViewState["ChromeType"]; return (o != null) ? (PartChromeType)(int)o : PartChromeType.Default; } set { if ((value < PartChromeType.Default) || (value > PartChromeType.BorderOnly)) { throw new ArgumentOutOfRangeException("value"); } ViewState["ChromeType"] = (int)value; } } // Copied from CompositeControl ////// /// public override ControlCollection Controls { get { EnsureChildControls(); return base.Controls; } } ////// Short descriptive text used in tooltips and web part catalog descriptions /// [ DefaultValue(""), Localizable(true), WebCategory("WebPartAppearance"), WebSysDescription(SR.Part_Description), ] public virtual string Description { get { string s = (string)ViewState["Description"]; return (s != null) ? s : String.Empty; } set { ViewState["Description"] = value; } } [ // Must use WebSysDefaultValue instead of DefaultValue, since it is overridden in extending classes Localizable(true), WebSysDefaultValue(""), WebCategory("WebPartAppearance"), WebSysDescription(SR.Part_Title), ] public virtual string Title { get { string s = (string)ViewState["Title"]; return (s != null) ? s : String.Empty; } set { ViewState["Title"] = value; } } // Copied from CompositeControl ////// Perform our own databinding, then perform our child controls' databinding. /// public override void DataBind() { OnDataBinding(EventArgs.Empty); EnsureChildControls(); DataBindChildren(); } // Copied from CompositeControl #region ICompositeControlDesignerAccessor implementation void ICompositeControlDesignerAccessor.RecreateChildControls() { ChildControlsCreated = false; EnsureChildControls(); } #endregion } }
Link Menu
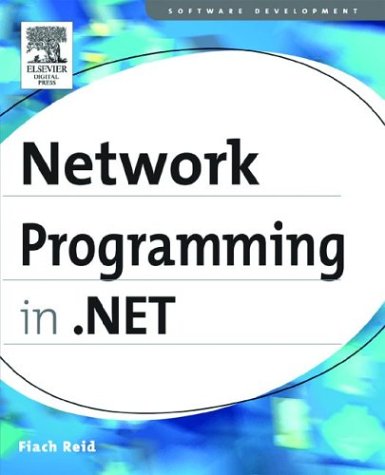
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectIDGenerator.cs
- Rijndael.cs
- SqlClientPermission.cs
- Utility.cs
- CqlWriter.cs
- JournalEntry.cs
- ConcurrentDictionary.cs
- ACE.cs
- QuerySetOp.cs
- DataListItem.cs
- WebPartConnectionsCancelVerb.cs
- SystemDiagnosticsSection.cs
- Int32Collection.cs
- DefaultSection.cs
- PopupRoot.cs
- SRDisplayNameAttribute.cs
- AssertFilter.cs
- DockPatternIdentifiers.cs
- ValidateNames.cs
- MasterPageBuildProvider.cs
- BinaryConverter.cs
- DecimalConstantAttribute.cs
- Mapping.cs
- ComboBoxItem.cs
- StructuredTypeInfo.cs
- WebPartDisplayModeCollection.cs
- Attributes.cs
- XamlPointCollectionSerializer.cs
- Encoder.cs
- Zone.cs
- TextServicesCompartment.cs
- WebPartTransformerCollection.cs
- DataSourceExpression.cs
- ToggleProviderWrapper.cs
- Int16Storage.cs
- TailPinnedEventArgs.cs
- DataContractSerializerOperationFormatter.cs
- PkcsUtils.cs
- MexHttpBindingCollectionElement.cs
- DynamicActionMessageFilter.cs
- FastEncoder.cs
- TextSerializer.cs
- DataGridItemEventArgs.cs
- SqlDataSource.cs
- DataSourceControlBuilder.cs
- PackageController.cs
- CellPartitioner.cs
- Rect3D.cs
- OperatingSystem.cs
- SafeEventLogWriteHandle.cs
- SapiRecognizer.cs
- FrameworkContentElement.cs
- MbpInfo.cs
- SmiEventStream.cs
- MatrixCamera.cs
- Int64Converter.cs
- DashStyle.cs
- ChangePasswordDesigner.cs
- XPathNodeInfoAtom.cs
- InstanceOwner.cs
- ProgressBarAutomationPeer.cs
- TimeoutHelper.cs
- LoginView.cs
- SplineKeyFrames.cs
- SHA512Managed.cs
- ExtractorMetadata.cs
- Registry.cs
- WindowsContainer.cs
- SoapReflectionImporter.cs
- TagPrefixInfo.cs
- Encoder.cs
- xdrvalidator.cs
- RotateTransform3D.cs
- RecognizerInfo.cs
- DeferredTextReference.cs
- PointHitTestResult.cs
- KeySpline.cs
- MLangCodePageEncoding.cs
- XmlDataSourceView.cs
- SqlFacetAttribute.cs
- AnnotationDocumentPaginator.cs
- SecureEnvironment.cs
- ProcessInputEventArgs.cs
- ExpressionReplacer.cs
- SchemaImporter.cs
- EventLogEntryCollection.cs
- InvalidWMPVersionException.cs
- SafeHandle.cs
- BitmapInitialize.cs
- File.cs
- HideDisabledControlAdapter.cs
- XsltArgumentList.cs
- ListViewUpdateEventArgs.cs
- DataGridViewCellStyleChangedEventArgs.cs
- ChameleonKey.cs
- File.cs
- GraphicsPathIterator.cs
- QilExpression.cs
- NameValueFileSectionHandler.cs
- WindowsListViewItemStartMenu.cs