Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Runtime / Serialization / ObjectIDGenerator.cs / 1 / ObjectIDGenerator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ObjectIDGenerator ** ** ** Purpose: ObjectIDGenerator is a simple id generator that keeps track of ** objects with a hashtable. ** ** ===========================================================*/ namespace System.Runtime.Serialization { using System; using System.Runtime.CompilerServices; using System.Runtime.Remoting; using System.Runtime.Remoting.Proxies; [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public class ObjectIDGenerator { private const int numbins = 4; internal int m_currentCount; internal int m_currentSize; internal long []m_ids; internal Object []m_objs; // Table of prime numbers to use as hash table sizes. Each entry is the // smallest prime number larger than twice the previous entry. private static readonly int[] sizes = { 5, 11, 29, 47, 97, 197, 397, 797, 1597, 3203, 6421, 12853, 25717, 51437, 102877, 205759, 411527, 823117, 1646237, 3292489, 6584983}; // Constructs a new ObjectID generator, initalizing all of the necessary variables. public ObjectIDGenerator() { m_currentCount=1; m_currentSize = sizes[0]; m_ids = new long[m_currentSize*numbins]; m_objs = new Object[m_currentSize*numbins]; } // Determines where element obj lives, or should live, // within the table. It calculates the hashcode and searches all of the // bins where the given object could live. If it's not found within the bin, // we rehash and go look for it in another bin. If we find the object, we // set found to true and return it's position. If we can't find the object, // we set found to false and return the position where the object should be inserted. // private int FindElement(Object obj, out bool found) { //This double-hashing algorithm is recomended by CLR, pg 236. //We're hosed if somebody rehashes the table while we're doing the lookup, but that's //generally true for most of these operations. int hashcode = RuntimeHelpers.GetHashCode(obj); int hashIncrement = (1+((hashcode&0x7FFFFFFF)%(m_currentSize-2))); do { int pos = ((hashcode&0x7FFFFFFF)%m_currentSize)*numbins; for (int i=pos; i(m_currentSize*numbins)/2) { Rehash(); } } else { BCLDebug.Trace("SER", "[ObjectIDGenerator.GetID] Found objectid: ", (m_ids[pos]), " with hashcode: ", RuntimeHelpers.GetHashCode(obj), " Type: ", obj); foundID = m_ids[pos]; } firstTime = !found; return foundID; } // Checks to see if obj has already been assigned an id. If it has, // we return that id, otherwise we return 0. // public virtual long HasId(Object obj, out bool firstTime) { bool found; if (obj==null) { throw new ArgumentNullException("obj", Environment.GetResourceString("ArgumentNull_Obj")); } int pos = FindElement(obj, out found); if (found) { firstTime = false; return m_ids[pos]; } firstTime=true; return 0; } // Rehashes the table by finding the next larger size in the list provided, // allocating two new arrays of that size and rehashing all of the elements in // the old arrays into the new ones. Expensive but necessary. // private void Rehash() { int i,pos; long [] newIds; long [] oldIds; Object[] newObjs; Object[] oldObjs; bool found; int currSize; for (i=0, currSize=m_currentSize; i
Link Menu
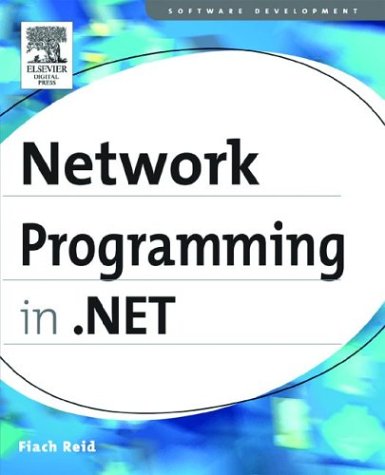
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RectAnimationBase.cs
- Int32CollectionConverter.cs
- WebServiceClientProxyGenerator.cs
- OdbcParameter.cs
- QueryExecutionOption.cs
- XmlMemberMapping.cs
- ThemeableAttribute.cs
- XmlSchemaSearchPattern.cs
- SingleConverter.cs
- QueryableDataSourceHelper.cs
- QilGeneratorEnv.cs
- ExeConfigurationFileMap.cs
- TypeLoadException.cs
- Config.cs
- XmlDataSource.cs
- Style.cs
- ReadWriteSpinLock.cs
- AutoSizeComboBox.cs
- SortedList.cs
- SqlInternalConnection.cs
- CopyCodeAction.cs
- ScriptingSectionGroup.cs
- LinkTarget.cs
- DrawingAttributeSerializer.cs
- FormViewDeletedEventArgs.cs
- BindingEditor.xaml.cs
- FolderNameEditor.cs
- InputMethodStateChangeEventArgs.cs
- TextEditorSelection.cs
- PerformanceCounterPermissionAttribute.cs
- TimeSpanSecondsConverter.cs
- AQNBuilder.cs
- TextSpanModifier.cs
- SafeNativeMethodsOther.cs
- OutgoingWebResponseContext.cs
- ValuePattern.cs
- PickBranch.cs
- RecognizerInfo.cs
- DataKey.cs
- AdornerPresentationContext.cs
- PolicyValidationException.cs
- InnerItemCollectionView.cs
- SQLUtility.cs
- TreeBuilderXamlTranslator.cs
- SpellerHighlightLayer.cs
- StrokeNode.cs
- TimelineGroup.cs
- Pair.cs
- Brush.cs
- TemplateContainer.cs
- COM2PropertyDescriptor.cs
- InstanceCreationEditor.cs
- PriorityQueue.cs
- SamlAssertionKeyIdentifierClause.cs
- XmlnsCache.cs
- SqlUdtInfo.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- PixelFormatConverter.cs
- MulticastIPAddressInformationCollection.cs
- DataSourceCache.cs
- PaintValueEventArgs.cs
- UidPropertyAttribute.cs
- TextTreeTextBlock.cs
- Matrix.cs
- Bits.cs
- ScriptingProfileServiceSection.cs
- OraclePermission.cs
- ItemsChangedEventArgs.cs
- HttpConfigurationSystem.cs
- SocketStream.cs
- StylusDevice.cs
- WebPartMinimizeVerb.cs
- HostedElements.cs
- sqlcontext.cs
- EventDescriptorCollection.cs
- HierarchicalDataTemplate.cs
- RegexGroupCollection.cs
- InvalidComObjectException.cs
- TreeNodeCollectionEditor.cs
- ReadOnlyMetadataCollection.cs
- AutomationTextAttribute.cs
- SearchExpression.cs
- ParseNumbers.cs
- DoubleAnimationUsingPath.cs
- PropertyInformation.cs
- RadioButtonFlatAdapter.cs
- ConfigXmlComment.cs
- CompiledQueryCacheKey.cs
- X509SecurityTokenProvider.cs
- SizeConverter.cs
- EntityDataSourceViewSchema.cs
- NamedPipeWorkerProcess.cs
- GeneralTransform3DGroup.cs
- X509SecurityTokenAuthenticator.cs
- IdnMapping.cs
- DrawingAttributesDefaultValueFactory.cs
- controlskin.cs
- ExternalException.cs
- WrapPanel.cs
- Glyph.cs