Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / Diagnostics / TraceSource.cs / 1305376 / TraceSource.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Threading; using System.Configuration; using System.Security; using System.Security.Permissions; namespace System.Diagnostics { public class TraceSource { private static Listtracesources = new List (); private static int s_LastCollectionCount; private SourceSwitch internalSwitch; private TraceListenerCollection listeners; private StringDictionary attributes; private SourceLevels switchLevel; private string sourceName; internal bool _initCalled = false; // Whether we've called Initialize already. public TraceSource(string name) : this(name, SourceLevels.Off) { } public TraceSource(string name, SourceLevels defaultLevel) { if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException("name"); sourceName = name; switchLevel = defaultLevel; // Delay load config to avoid perf (and working set) issues in retail // Add a weakreference to this source and cleanup invalid references lock(tracesources) { _pruneCachedTraceSources(); tracesources.Add(new WeakReference(this)); } } private static void _pruneCachedTraceSources() { lock (tracesources) { if (s_LastCollectionCount != GC.CollectionCount(2)) { List buffer = new List (tracesources.Count); for (int i = 0; i < tracesources.Count; i++) { TraceSource tracesource = ((TraceSource)tracesources[i].Target); if (tracesource != null) { buffer.Add(tracesources[i]); } } if (buffer.Count < tracesources.Count) { tracesources.Clear(); tracesources.AddRange(buffer); tracesources.TrimExcess(); } s_LastCollectionCount = GC.CollectionCount(2); } } } private void Initialize() { if (!_initCalled) { lock(this) { if (_initCalled) return; SourceElementsCollection sourceElements = DiagnosticsConfiguration.Sources; if (sourceElements != null) { SourceElement sourceElement = sourceElements[sourceName]; if (sourceElement != null) { if (!String.IsNullOrEmpty(sourceElement.SwitchName)) { CreateSwitch(sourceElement.SwitchType, sourceElement.SwitchName); } else { CreateSwitch(sourceElement.SwitchType, sourceName); if (!String.IsNullOrEmpty(sourceElement.SwitchValue)) internalSwitch.Level = (SourceLevels) Enum.Parse(typeof(SourceLevels), sourceElement.SwitchValue); } listeners = sourceElement.Listeners.GetRuntimeObject(); attributes = new StringDictionary(); TraceUtils.VerifyAttributes(sourceElement.Attributes, GetSupportedAttributes(), this); attributes.ReplaceHashtable(sourceElement.Attributes); } else NoConfigInit(); } else NoConfigInit(); _initCalled = true; } } } private void NoConfigInit() { internalSwitch = new SourceSwitch(sourceName, switchLevel.ToString()); listeners = new TraceListenerCollection(); listeners.Add(new DefaultTraceListener()); attributes = null; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public void Close() { // No need to call Initialize() if (listeners != null) { // Use global lock lock (TraceInternal.critSec) { foreach (TraceListener listener in listeners) { listener.Close(); } } } } public void Flush() { // No need to call Initialize() if (listeners != null) { if (TraceInternal.UseGlobalLock) { lock (TraceInternal.critSec) { foreach (TraceListener listener in listeners) { listener.Flush(); } } } else { foreach (TraceListener listener in listeners) { if (!listener.IsThreadSafe) { lock (listener) { listener.Flush(); } } else { listener.Flush(); } } } } } virtual protected internal string[] GetSupportedAttributes() { return null; } internal static void RefreshAll() { lock (tracesources) { _pruneCachedTraceSources(); for (int i=0; i // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Threading; using System.Configuration; using System.Security; using System.Security.Permissions; namespace System.Diagnostics { public class TraceSource { private static List tracesources = new List (); private static int s_LastCollectionCount; private SourceSwitch internalSwitch; private TraceListenerCollection listeners; private StringDictionary attributes; private SourceLevels switchLevel; private string sourceName; internal bool _initCalled = false; // Whether we've called Initialize already. public TraceSource(string name) : this(name, SourceLevels.Off) { } public TraceSource(string name, SourceLevels defaultLevel) { if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException("name"); sourceName = name; switchLevel = defaultLevel; // Delay load config to avoid perf (and working set) issues in retail // Add a weakreference to this source and cleanup invalid references lock(tracesources) { _pruneCachedTraceSources(); tracesources.Add(new WeakReference(this)); } } private static void _pruneCachedTraceSources() { lock (tracesources) { if (s_LastCollectionCount != GC.CollectionCount(2)) { List buffer = new List (tracesources.Count); for (int i = 0; i < tracesources.Count; i++) { TraceSource tracesource = ((TraceSource)tracesources[i].Target); if (tracesource != null) { buffer.Add(tracesources[i]); } } if (buffer.Count < tracesources.Count) { tracesources.Clear(); tracesources.AddRange(buffer); tracesources.TrimExcess(); } s_LastCollectionCount = GC.CollectionCount(2); } } } private void Initialize() { if (!_initCalled) { lock(this) { if (_initCalled) return; SourceElementsCollection sourceElements = DiagnosticsConfiguration.Sources; if (sourceElements != null) { SourceElement sourceElement = sourceElements[sourceName]; if (sourceElement != null) { if (!String.IsNullOrEmpty(sourceElement.SwitchName)) { CreateSwitch(sourceElement.SwitchType, sourceElement.SwitchName); } else { CreateSwitch(sourceElement.SwitchType, sourceName); if (!String.IsNullOrEmpty(sourceElement.SwitchValue)) internalSwitch.Level = (SourceLevels) Enum.Parse(typeof(SourceLevels), sourceElement.SwitchValue); } listeners = sourceElement.Listeners.GetRuntimeObject(); attributes = new StringDictionary(); TraceUtils.VerifyAttributes(sourceElement.Attributes, GetSupportedAttributes(), this); attributes.ReplaceHashtable(sourceElement.Attributes); } else NoConfigInit(); } else NoConfigInit(); _initCalled = true; } } } private void NoConfigInit() { internalSwitch = new SourceSwitch(sourceName, switchLevel.ToString()); listeners = new TraceListenerCollection(); listeners.Add(new DefaultTraceListener()); attributes = null; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public void Close() { // No need to call Initialize() if (listeners != null) { // Use global lock lock (TraceInternal.critSec) { foreach (TraceListener listener in listeners) { listener.Close(); } } } } public void Flush() { // No need to call Initialize() if (listeners != null) { if (TraceInternal.UseGlobalLock) { lock (TraceInternal.critSec) { foreach (TraceListener listener in listeners) { listener.Flush(); } } } else { foreach (TraceListener listener in listeners) { if (!listener.IsThreadSafe) { lock (listener) { listener.Flush(); } } else { listener.Flush(); } } } } } virtual protected internal string[] GetSupportedAttributes() { return null; } internal static void RefreshAll() { lock (tracesources) { _pruneCachedTraceSources(); for (int i=0; i
Link Menu
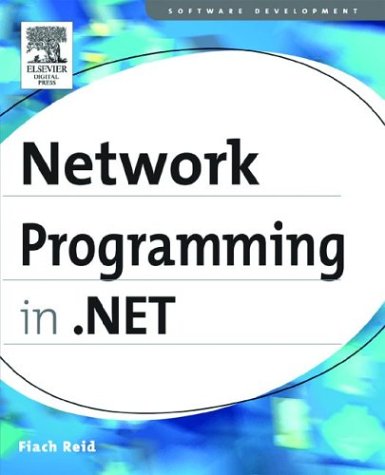
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColorComboBox.cs
- CheckBoxBaseAdapter.cs
- CorrelationScope.cs
- DistinctQueryOperator.cs
- DataGridLinkButton.cs
- ConfigurationElementProperty.cs
- Menu.cs
- RoutedEventValueSerializer.cs
- DbProviderFactory.cs
- SqlDataSourceQueryEditorForm.cs
- CapabilitiesState.cs
- MemberProjectionIndex.cs
- DirectoryGroupQuery.cs
- TreeViewEvent.cs
- ProxySimple.cs
- ResourceContainer.cs
- XmlWhitespace.cs
- SafeWaitHandle.cs
- UpdatePanelTrigger.cs
- ContainerAction.cs
- ExpressionBindingCollection.cs
- LocalizationParserHooks.cs
- StringStorage.cs
- SystemColorTracker.cs
- MobileTextWriter.cs
- ZipIOLocalFileBlock.cs
- SettingsBindableAttribute.cs
- CompositionDesigner.cs
- URLAttribute.cs
- ConfigurationStrings.cs
- TreeNodeMouseHoverEvent.cs
- cache.cs
- DomNameTable.cs
- RegistrySecurity.cs
- PropertyDescriptors.cs
- InkCanvasSelection.cs
- XmlAutoDetectWriter.cs
- SmiXetterAccessMap.cs
- ImmutableAssemblyCacheEntry.cs
- ControlParser.cs
- BamlRecordWriter.cs
- BooleanToVisibilityConverter.cs
- _NestedMultipleAsyncResult.cs
- FunctionDescription.cs
- CurrentTimeZone.cs
- SqlDataRecord.cs
- FileSystemEventArgs.cs
- ColorTranslator.cs
- MetricEntry.cs
- AssemblyUtil.cs
- ImageSource.cs
- AudioFileOut.cs
- ColorPalette.cs
- Win32MouseDevice.cs
- BaseDataBoundControl.cs
- RoleServiceManager.cs
- DataGridClipboardHelper.cs
- SqlUDTStorage.cs
- ToolStripSettings.cs
- EventTask.cs
- HotCommands.cs
- SequentialWorkflowRootDesigner.cs
- UseAttributeSetsAction.cs
- SafeReadContext.cs
- InternalDispatchObject.cs
- MiniConstructorInfo.cs
- HyperLink.cs
- TiffBitmapEncoder.cs
- SrgsSubset.cs
- RegisteredExpandoAttribute.cs
- HandlerBase.cs
- PointHitTestResult.cs
- DataKey.cs
- Bits.cs
- RegistrySecurity.cs
- Polygon.cs
- WindowsRichEdit.cs
- DataGridViewAdvancedBorderStyle.cs
- XmlUtilWriter.cs
- MasterPage.cs
- SqlParameterCollection.cs
- InfoCardBinaryReader.cs
- MetaTableHelper.cs
- WebPartVerbCollection.cs
- CodeTypeParameter.cs
- TextBoxAutoCompleteSourceConverter.cs
- XmlDataSourceView.cs
- OdbcHandle.cs
- TableAdapterManagerMethodGenerator.cs
- StringAnimationBase.cs
- SineEase.cs
- ListenDesigner.cs
- SchemaImporterExtensionsSection.cs
- TemplateControlParser.cs
- WindowsPen.cs
- PreservationFileWriter.cs
- SigningProgress.cs
- HttpCacheVaryByContentEncodings.cs
- SortExpressionBuilder.cs
- DocumentSchemaValidator.cs