Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Misc / GDI / WindowsPen.cs / 1305376 / WindowsPen.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if [....]_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Drawing2D; using System.Globalization; using System.Runtime.Versioning; ////// #if [....]_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif sealed partial class WindowsPen : MarshalByRefObject, ICloneable, IDisposable { // // Handle to the native Windows pen object. // private IntPtr nativeHandle; private const int dashStyleMask = 0x0000000F; private const int endCapMask = 0x00000F00; private const int joinMask = 0x0000F000; private DeviceContext dc; // // Fields with default values // private WindowsBrush wndBrush; private WindowsPenStyle style; private Color color; private int width; private const int cosmeticPenWidth = 1; // Cosmetic pen width. #if GDI_FINALIZATION_WATCH private string AllocationSite = DbgUtil.StackTrace; #endif [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, Color.Black ) { } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, Color color ) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, color ) { } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsBrush windowsBrush ) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, windowsBrush ) { } [ResourceExposure(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsPenStyle style, int width, Color color) { this.style = style; this.width = width; this.color = color; this.dc = dc; // CreatePen() created on demand. } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsPenStyle style, int width, WindowsBrush windowsBrush ) { Debug.Assert(windowsBrush != null, "null windowsBrush" ); this.style = style; this.wndBrush = (WindowsBrush) windowsBrush.Clone(); this.width = width; this.color = windowsBrush.Color; this.dc = dc; // CreatePen() created on demand. } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] private void CreatePen() { if (this.width > 1) // Geometric pen. { // From MSDN: if width > 1, the style must be PS_NULL, PS_SOLID, or PS_INSIDEFRAME. this.style |= WindowsPenStyle.Geometric | WindowsPenStyle.Solid; } if (this.wndBrush == null) { this.nativeHandle = IntSafeNativeMethods.CreatePen((int) this.style, this.width, ColorTranslator.ToWin32(this.color) ); } else { IntNativeMethods.LOGBRUSH lb = new IntNativeMethods.LOGBRUSH(); lb.lbColor = ColorTranslator.ToWin32( this.wndBrush.Color ); lb.lbStyle = IntNativeMethods.BS_SOLID; lb.lbHatch = 0; // // Note: We currently don't support custom styles, that's why 0 and null for last two params. this.nativeHandle = IntSafeNativeMethods.ExtCreatePen((int)this.style, this.width, lb, 0, null ); } } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public object Clone() { return (this.wndBrush != null) ? new WindowsPen(this.dc, this.style, this.width, (WindowsBrush) this.wndBrush.Clone()) : new WindowsPen(this.dc, this.style, this.width, this.color); } ~WindowsPen() { Dispose(false); } public void Dispose() { Dispose(true); } void Dispose(bool disposing) { if (this.nativeHandle != IntPtr.Zero && dc != null) { DbgUtil.AssertFinalization(this, disposing); dc.DeleteObject(this.nativeHandle, GdiObjectType.Pen); this.nativeHandle = IntPtr.Zero; } if (this.wndBrush != null) { this.wndBrush.Dispose(); this.wndBrush = null; } if (disposing) { GC.SuppressFinalize(this); } } public IntPtr HPen { get { if( this.nativeHandle == IntPtr.Zero ) { CreatePen(); } return this.nativeHandle; } } public override string ToString() { return String.Format( CultureInfo.InvariantCulture, "{0}: Style={1}, Color={2}, Width={3}, Brush={4}", this.GetType().Name, this.style, this.color, this.width, this.wndBrush != null ? this.wndBrush.ToString() : "null" ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Encapsulates a GDI Pen object. /// ///
Link Menu
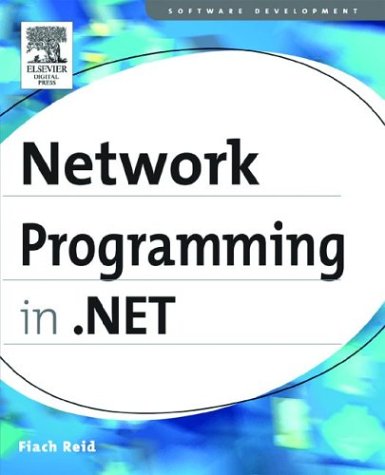
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProtocolsSection.cs
- TextDecoration.cs
- CounterNameConverter.cs
- ZipIOBlockManager.cs
- DbConnectionPoolOptions.cs
- AstTree.cs
- ConnectivityStatus.cs
- ControlAdapter.cs
- BindingValueChangedEventArgs.cs
- RuntimeHelpers.cs
- ProxyHelper.cs
- LocalizeDesigner.cs
- LockCookie.cs
- RegexReplacement.cs
- XmlCountingReader.cs
- wpf-etw.cs
- columnmapkeybuilder.cs
- LingerOption.cs
- SamlSerializer.cs
- SqlBuilder.cs
- PeerName.cs
- XmlSchemaSimpleTypeList.cs
- XNodeNavigator.cs
- Trigger.cs
- AtomPub10ServiceDocumentFormatter.cs
- WebPartConnectVerb.cs
- RenderDataDrawingContext.cs
- BevelBitmapEffect.cs
- Identity.cs
- TextRunTypographyProperties.cs
- ApplicationInfo.cs
- FileUtil.cs
- EncodingInfo.cs
- XslAst.cs
- Vector3DAnimation.cs
- SqlUtil.cs
- TextBoxView.cs
- TextParagraphProperties.cs
- AvTraceDetails.cs
- PtsHelper.cs
- ThreadStateException.cs
- AQNBuilder.cs
- DataGridViewTextBoxCell.cs
- ContainerVisual.cs
- VerificationAttribute.cs
- PageContentCollection.cs
- SoapMessage.cs
- ExceptionWrapper.cs
- QueryReaderSettings.cs
- CodeRemoveEventStatement.cs
- DesignerSerializationOptionsAttribute.cs
- GradientStopCollection.cs
- SafeHGlobalHandleCritical.cs
- Literal.cs
- StylusLogic.cs
- XmlCharCheckingReader.cs
- DataSetSchema.cs
- DesignTimeSiteMapProvider.cs
- ListViewUpdatedEventArgs.cs
- DLinqDataModelProvider.cs
- FrameworkElementFactory.cs
- GetChildSubtree.cs
- TextServicesPropertyRanges.cs
- AnnotationObservableCollection.cs
- MenuCommand.cs
- OutputCacheProfile.cs
- StrongNameKeyPair.cs
- WindowsAltTab.cs
- BooleanConverter.cs
- DrawingContext.cs
- ComIntegrationManifestGenerator.cs
- AccessDataSource.cs
- SoapAttributes.cs
- SchemaTypeEmitter.cs
- FormViewDeleteEventArgs.cs
- Size3D.cs
- TablePattern.cs
- XmlBaseWriter.cs
- SafeCloseHandleCritical.cs
- SoapSchemaMember.cs
- SynchronousSendBindingElement.cs
- SimpleWebHandlerParser.cs
- EditingCommands.cs
- httpserverutility.cs
- OutputCacheSettings.cs
- SlotInfo.cs
- SocketSettings.cs
- TypeSystemHelpers.cs
- AmbientProperties.cs
- CalendarButtonAutomationPeer.cs
- SrgsItemList.cs
- PrinterSettings.cs
- DropShadowEffect.cs
- SqlPersonalizationProvider.cs
- TraceHandler.cs
- XslTransform.cs
- WindowsStatusBar.cs
- MemberHolder.cs
- DataColumnCollection.cs
- TraceLevelStore.cs