Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / InfoCardBinaryReader.cs / 1 / InfoCardBinaryReader.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Runtime.InteropServices; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Internal BinaryWriter class that will throw InfoCardArgumentExceptions // when stream appears to be corrupted. // internal sealed class InfoCardBinaryReader : BinaryReader { static readonly char[] s_singleChar = { 'a' }; int m_charSize; public InfoCardBinaryReader(Stream stream, Encoding encoding) : base(stream, encoding ) { m_charSize = encoding.GetByteCount( s_singleChar ); } public InfoCardBinaryReader(Stream stream) : this(stream,Encoding.Unicode) { } public override int Read() { EnsureBufferBounds(1); return base.Read(); } public override int Read(byte[] buffer, int index, int count) { // // This method will not read past the end of the buffer, // and are used to read blocks of information. The count // provided may exceed the max, but only what is present // will be returned. // return base.Read(buffer, index, count); } public override int Read(char[] buffer, int index, int count) { // // This method will not read past the end of the buffer, // and are used to read blocks of information. The count // provided may exceed the max, but only what is present // will be returned. // return base.Read(buffer, index, count); } public override bool ReadBoolean() { EnsureBufferBounds(1); return base.ReadBoolean(); } public override byte ReadByte() { EnsureBufferBounds(1); return base.ReadByte(); } public override byte[] ReadBytes(int count) { EnsureBufferBounds(count); return base.ReadBytes(count); } public override char ReadChar() { EnsureBufferBounds(m_charSize); return base.ReadChar(); } public override char[] ReadChars(int count) { EnsureBufferBounds(Convert.ToInt64(m_charSize * count)); return base.ReadChars(count); } public override decimal ReadDecimal() { EnsureBufferBounds( Convert.ToInt64( Marshal.SizeOf( typeof(Decimal) ) ) ); return base.ReadDecimal(); } public override double ReadDouble() { EnsureBufferBounds(sizeof(Double)); return base.ReadDouble(); } public override short ReadInt16() { EnsureBufferBounds(sizeof(Int16)); return base.ReadInt16(); } public override int ReadInt32() { EnsureBufferBounds(sizeof(Int32)); return base.ReadInt32(); } public override long ReadInt64() { EnsureBufferBounds(sizeof(Int64)); return base.ReadInt64(); } public override sbyte ReadSByte() { EnsureBufferBounds(sizeof(SByte)); return base.ReadSByte(); } public override float ReadSingle() { EnsureBufferBounds(sizeof(Single)); return base.ReadSingle(); } public override string ReadString() { EnsureBufferBounds( (m_charSize * PeekChar()) + sizeof(Int32) ); return base.ReadString(); } public override ushort ReadUInt16() { EnsureBufferBounds(sizeof(UInt32)); return base.ReadUInt16(); } public override uint ReadUInt32() { EnsureBufferBounds(sizeof(UInt32)); return base.ReadUInt32(); } public override ulong ReadUInt64() { EnsureBufferBounds(sizeof(UInt64)); return base.ReadUInt64(); } // // Summary: // Ensures that the offset will not overrun the stream. // void EnsureBufferBounds(long offset) { if( (ulong)(BaseStream.Position + offset) > (ulong)BaseStream.Length) { throw IDT.ThrowHelperError( new InfoCardArgumentException( SR.GetString( SR.InvalidOrCorruptArgumentStream ) ) ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
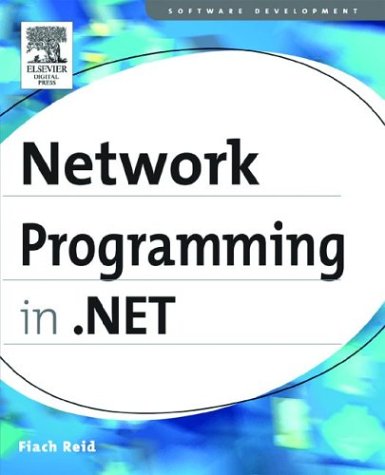
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LiteralDesigner.cs
- GuidelineSet.cs
- TypeConverterValueSerializer.cs
- AssemblyResourceLoader.cs
- TypeElementCollection.cs
- ResolveNameEventArgs.cs
- HotSpotCollection.cs
- ResourceExpressionBuilder.cs
- WebPartZone.cs
- CodeTypeReferenceExpression.cs
- OdbcReferenceCollection.cs
- DataGridCommandEventArgs.cs
- ComAdminInterfaces.cs
- CompositionTarget.cs
- ObjectKeyFrameCollection.cs
- GridViewAutomationPeer.cs
- ZipIOFileItemStream.cs
- RegexCaptureCollection.cs
- ClientConfigPaths.cs
- EncryptedXml.cs
- TimeZone.cs
- FixedSOMPage.cs
- SQLBoolean.cs
- QueryContinueDragEvent.cs
- StringAnimationUsingKeyFrames.cs
- PipeSecurity.cs
- FileLogRecordEnumerator.cs
- TreeNodeStyleCollection.cs
- __Error.cs
- NativeMethods.cs
- Compiler.cs
- Clock.cs
- SemanticBasicElement.cs
- SoundPlayer.cs
- BamlLocalizabilityResolver.cs
- ImageSource.cs
- SubMenuStyleCollection.cs
- DllNotFoundException.cs
- ToolStripButton.cs
- TraversalRequest.cs
- RawUIStateInputReport.cs
- SystemIPInterfaceStatistics.cs
- XmlEntity.cs
- Hash.cs
- StackBuilderSink.cs
- AppDomain.cs
- ExceptionValidationRule.cs
- CommandExpr.cs
- StatusInfoItem.cs
- _TLSstream.cs
- Trace.cs
- OleDbConnectionFactory.cs
- TimersDescriptionAttribute.cs
- PeerFlooder.cs
- DataTableClearEvent.cs
- ProcessHostFactoryHelper.cs
- BitmapEffectDrawing.cs
- SecurityProtocolFactory.cs
- RowUpdatedEventArgs.cs
- LabelLiteral.cs
- ImageSourceConverter.cs
- WebPart.cs
- Setter.cs
- SqlDataSource.cs
- CorrelationRequestContext.cs
- EdmPropertyAttribute.cs
- AnimationClock.cs
- ConstantExpression.cs
- LinqExpressionNormalizer.cs
- ChannelManager.cs
- ResourceContainer.cs
- PrimitiveSchema.cs
- WebBrowserSiteBase.cs
- ClientSideProviderDescription.cs
- SingleStorage.cs
- VirtualPath.cs
- GridViewEditEventArgs.cs
- CodeValidator.cs
- MultiSelector.cs
- RSAOAEPKeyExchangeFormatter.cs
- LinqDataSourceContextData.cs
- ShaperBuffers.cs
- MultiTrigger.cs
- TypeElement.cs
- ScriptRef.cs
- SchemaTableOptionalColumn.cs
- Convert.cs
- GradientStop.cs
- ThrowHelper.cs
- CTreeGenerator.cs
- ParameterRetriever.cs
- TrustManagerMoreInformation.cs
- DataGridCell.cs
- ThaiBuddhistCalendar.cs
- ApplicationManager.cs
- WindowsSolidBrush.cs
- LoginName.cs
- IDQuery.cs
- ImageMetadata.cs
- SpecularMaterial.cs