Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / StatusInfoItem.cs / 1 / StatusInfoItem.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Used to control an (RM or DigSig) InfoBar and ToolBar item in MongooseUI // // History: // 06/22/2005 - [....] created // //--------------------------------------------------------------------------- using MS.Internal.Documents; using System; using System.Globalization; using System.Windows; using System.Windows.Controls; using System.Windows.Shapes; using System.Windows.TrustUI; namespace MS.Internal.Documents.Application { ////// The type of StatusInfoItem (DigSig, RM) /// internal enum StatusInfoItemType { Unknown, DigSig, RM, } ////// Class used to control an item of the InfoBar, and the relevant ToolBar Button. /// internal class StatusInfoItem { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Constructs a new StatusInfoItem /// /// Type of Item /// InfoBar button to update /// ToolBar button to update public StatusInfoItem(StatusInfoItemType type, Button infoBarButton, Control toolBarControl) { // Set Type _type = type; // If InfoBar button is not null, set references to the Icon and Text. if (infoBarButton != null) { _infoBarButton = infoBarButton; _infoBarIcon = infoBarButton.Template.FindName("PUIInfoBarIcon", infoBarButton) as Rectangle; _infoBarText = infoBarButton.Template.FindName("PUIInfoBarText", infoBarButton) as TextBlock; } // Set ToolBar button reference. if (toolBarControl != null) { _toolBarControl = toolBarControl; } } //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// Controls the visibility of the InfoBar button. /// public Visibility Visibility { get { return _infoBarButton.Visibility; } set { _infoBarButton.Visibility = value; // When the Visibility is set, notify the DocumentViewer to check the visibility // of the entire InfoBar if (InfoBarVisibilityChanged != null) { InfoBarVisibilityChanged(this, new EventArgs()); } } } //------------------------------------------------------ // // Public Events // //------------------------------------------------------ ////// Fired whenever the visibility has been set on the StatusInfoItem, useful for determining /// if the InfoBar should be hidden. /// public event EventHandler InfoBarVisibilityChanged; //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ ////// Used to be notified of possible DigSig status changes. /// /// /// public void OnStatusChange(object sender, DocumentSignatureManager.SignatureStatusEventArgs args) { if ((args != null) && (_type == StatusInfoItemType.DigSig)) { UpdateUI(args.StatusResources); // If the Document is not signed, collapse the infobar button. if (args.SignatureStatus != SignatureStatus.NotSigned) { this.Visibility = Visibility.Visible; } else { this.Visibility = Visibility.Collapsed; } } } ////// Used to be notified of possible RM status changes. /// /// /// public void OnStatusChange(object sender, DocumentRightsManagementManager.RightsManagementStatusEventArgs args) { if ((args != null) && (_type == StatusInfoItemType.RM)) { UpdateUI(args.StatusResources); // If the Document is not RM protected, collapse the infobar button. if (args.RMStatus == RightsManagementStatus.Protected) { this.Visibility = Visibility.Visible; } else { this.Visibility = Visibility.Collapsed; } } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- ////// Used to change the Icons and Text of the InfoBarItems and ToolBarButton. /// /// ////// This function directly updates the UI with information that may be /// used for trust decisions by the user. Since the UI is displayed in /// Avalon controls, however, untrusted callers can directly modify the /// information displayed. As a result making this method critical does /// not add any protection. /// private void UpdateUI(DocumentStatusResources resources) { if (_infoBarIcon != null) { // Set the InfoBar Image _infoBarIcon.Fill = resources.Image; } if (_infoBarText != null) { // Set the InfoBar Text _infoBarText.Text = resources.Text; } if (_infoBarButton != null) { // Set the InfoBar ToolTip _infoBarButton.ToolTip = resources.ToolTip; } if (_toolBarControl != null) { // Set the ToolBarButton Image and ToolTip _toolBarControl.Background = resources.Image; _toolBarControl.ToolTip = resources.ToolTip; } } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private StatusInfoItemType _type; private Rectangle _infoBarIcon; private TextBlock _infoBarText; private Button _infoBarButton; private Control _toolBarControl; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
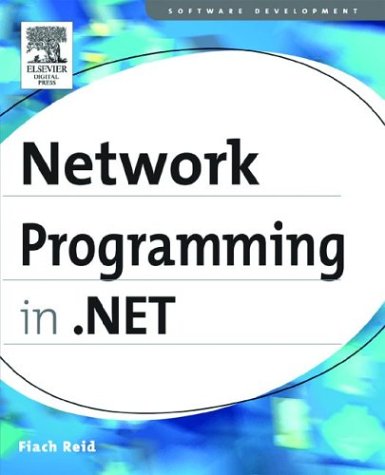
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColorConverter.cs
- DataShape.cs
- TargetParameterCountException.cs
- ColorConvertedBitmap.cs
- COM2Properties.cs
- TriggerCollection.cs
- LineServicesCallbacks.cs
- DataObjectMethodAttribute.cs
- TemplateControlParser.cs
- TimerTable.cs
- TabControl.cs
- DataBindingHandlerAttribute.cs
- SessionEndingEventArgs.cs
- PhonemeEventArgs.cs
- SafeWaitHandle.cs
- TrustSection.cs
- ToolStripDropTargetManager.cs
- NotifyIcon.cs
- SynchronizedDispatch.cs
- NumericUpDown.cs
- CrossContextChannel.cs
- FixedSOMLineCollection.cs
- MissingFieldException.cs
- DockingAttribute.cs
- StickyNote.cs
- BamlResourceContent.cs
- RequestQueryParser.cs
- DeviceContext.cs
- PeerCollaboration.cs
- RegexNode.cs
- XmlILModule.cs
- serverconfig.cs
- KerberosSecurityTokenParameters.cs
- RuntimeHelpers.cs
- PropertyEmitter.cs
- TrackingCondition.cs
- RecipientInfo.cs
- DataGridViewEditingControlShowingEventArgs.cs
- TemplateParser.cs
- ProxyFragment.cs
- Funcletizer.cs
- ScalarConstant.cs
- ImageListDesigner.cs
- ByteStorage.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- EntityClientCacheKey.cs
- ObjectDataSourceMethodEditor.cs
- PathGeometry.cs
- MediaSystem.cs
- DeclarationUpdate.cs
- DataServiceRequest.cs
- KeyNotFoundException.cs
- SoapAttributeAttribute.cs
- GC.cs
- WebHttpSecurityElement.cs
- XmlDataSource.cs
- MethodMessage.cs
- Domain.cs
- DocumentPaginator.cs
- DirectionalLight.cs
- DesignerCommandAdapter.cs
- HMACRIPEMD160.cs
- CultureTable.cs
- XmlSchemaImporter.cs
- ParenthesizePropertyNameAttribute.cs
- CultureTable.cs
- SmtpSection.cs
- ContractCodeDomInfo.cs
- BatchParser.cs
- StateItem.cs
- JumpList.cs
- RawStylusInputCustomData.cs
- FormattedText.cs
- TrackBarRenderer.cs
- InkCanvasInnerCanvas.cs
- TemplateApplicationHelper.cs
- CodeDelegateCreateExpression.cs
- pingexception.cs
- ArcSegment.cs
- BorderGapMaskConverter.cs
- CommonProperties.cs
- IssuanceLicense.cs
- TimeStampChecker.cs
- AssemblyNameProxy.cs
- ResolvedKeyFrameEntry.cs
- BindableAttribute.cs
- ServiceDescription.cs
- StorageMappingItemCollection.cs
- InvalidFilterCriteriaException.cs
- DataGridTextBox.cs
- ListBoxItemAutomationPeer.cs
- FormViewInsertedEventArgs.cs
- FixedMaxHeap.cs
- JsonSerializer.cs
- ExternalFile.cs
- ErrorsHelper.cs
- ChangePassword.cs
- MulticastNotSupportedException.cs
- AdornerLayer.cs
- DataGrid.cs