Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / FixedSOMLineCollection.cs / 1 / FixedSOMLineCollection.cs
/*++ File: FixedSOMLineCollection.cs Copyright (C) 2005 Microsoft Corporation. All rights reserved. Description: Internal helper class that can store a set of sorted horizontal and vertical FixedSOMLineRanges. These ranges are used in construction of FixedBlocks and Tables History: 05/17/2005: [....] - Created --*/ namespace System.Windows.Documents { using System.Collections.Generic; using System.Windows.Shapes; using System.Windows.Media; using System.Diagnostics; using System.Windows; //Stores a collection of horizontal and vertical lines sorted by y and x axis respectively // internal sealed class FixedSOMLineCollection { //-------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors public FixedSOMLineCollection() { _verticals = new List(); _horizontals = new List (); } #endregion Constructors //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Public Methods public bool IsVerticallySeparated(double left, double top, double right, double bottom) { return _IsSeparated(_verticals, left, top, right, bottom); } public bool IsHorizontallySeparated(double left, double top, double right, double bottom) { return _IsSeparated(_horizontals, top, left, bottom, right); } public void AddVertical(Point point1, Point point2) { Debug.Assert(point1.X == point2.X); _AddLineToRanges(_verticals, point1.X, point1.Y, point2.Y); } public void AddHorizontal(Point point1, Point point2) { Debug.Assert(point1.Y == point2.Y); _AddLineToRanges(_horizontals, point1.Y, point1.X, point2.X); } #endregion Public Methods #region Private Methods //Merge line 2 into line 1 private void _AddLineToRanges(List ranges, double line, double start, double end) { if (start > end) { double temp = start; start = end; end = temp; } FixedSOMLineRanges range; double maxSeparation = .5 * FixedSOMLineRanges.MinLineSeparation; for (int i=0; i < ranges.Count; i++) { if (line < ranges[i].Line - maxSeparation) { range = new FixedSOMLineRanges(); range.Line = line; range.AddRange(start, end); ranges.Insert(i, range); return; } else if (line < ranges[i].Line + maxSeparation) { ranges[i].AddRange(start, end); return; } } // add to end range = new FixedSOMLineRanges(); range.Line = line; range.AddRange(start, end); ranges.Add(range); return; } //Generic function that decides whether or not a rectangle as spefied by the points is //divided by any of the lines in the line ranges in the passed in list private bool _IsSeparated(List lines, double parallelLowEnd, double perpLowEnd, double parallelHighEnd, double perpHighEnd) { int startIndex = 0; int endIndex = lines.Count; if (endIndex == 0) { return false; } int i = 0; while (endIndex > startIndex) { i = (startIndex + endIndex) >> 1; if (lines[i].Line < parallelLowEnd) { startIndex = i + 1; } else { if (lines[i].Line <= parallelHighEnd) { break; } endIndex = i; } } if (lines[i].Line >= parallelLowEnd && lines[i].Line <= parallelHighEnd) { do { i--; } while (i>=0 && lines[i].Line >= parallelLowEnd); i++; while (i =0) { double end = lines[i].End[rangeIndex]; if (end >= perpHighEnd - allowedMargin) { return true; } } i++; }; } return false; } #endregion Private Methods #region Public Properties public List HorizontalLines { get { return _horizontals; } } public List VerticalLines { get { return _verticals; } } #endregion Public Properties //-------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields private List _horizontals; private List _verticals; private const double _fudgeFactor = 0.1; // We allow 10% margin at each end #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
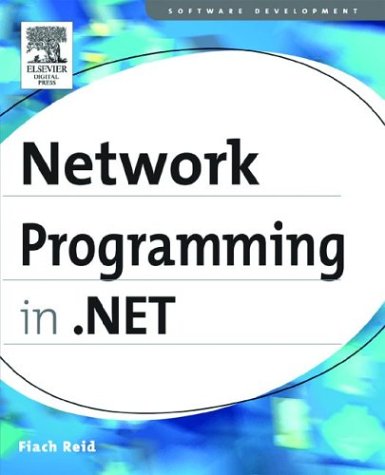
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrinterResolution.cs
- InitializationEventAttribute.cs
- ElementMarkupObject.cs
- DataColumnChangeEvent.cs
- DirectoryNotFoundException.cs
- OrderedEnumerableRowCollection.cs
- HtmlTitle.cs
- DetailsViewRowCollection.cs
- PagerSettings.cs
- CreateUserWizardStep.cs
- EntityReference.cs
- Win32KeyboardDevice.cs
- LineProperties.cs
- AdornerLayer.cs
- _emptywebproxy.cs
- SQLUtility.cs
- ModuleElement.cs
- ControlValuePropertyAttribute.cs
- ScriptMethodAttribute.cs
- XmlDataDocument.cs
- RbTree.cs
- BoundsDrawingContextWalker.cs
- Padding.cs
- GridViewColumn.cs
- MasterPageBuildProvider.cs
- PropertyEmitter.cs
- NotCondition.cs
- EnumMember.cs
- CheckBoxRenderer.cs
- SplitContainer.cs
- ServiceParser.cs
- ResourcesChangeInfo.cs
- PolicyException.cs
- TcpTransportManager.cs
- PersonalizationProviderCollection.cs
- CheckedPointers.cs
- SqlWebEventProvider.cs
- GeneratedContractType.cs
- DataGridViewTextBoxColumn.cs
- AuditLevel.cs
- PolicyLevel.cs
- ProgressBarRenderer.cs
- WebConfigManager.cs
- MessageAction.cs
- RewritingSimplifier.cs
- XamlTreeBuilder.cs
- UnsafeNativeMethods.cs
- OracleDataReader.cs
- TraceListeners.cs
- MenuItem.cs
- _NetworkingPerfCounters.cs
- CustomAttributeSerializer.cs
- SqlNotificationEventArgs.cs
- GlyphInfoList.cs
- UDPClient.cs
- TextView.cs
- SqlEnums.cs
- MappingMetadataHelper.cs
- SqlBuilder.cs
- TreeViewEvent.cs
- _NetRes.cs
- FileAuthorizationModule.cs
- CreateUserWizard.cs
- Comparer.cs
- WebPartDisplayModeCancelEventArgs.cs
- ListViewCommandEventArgs.cs
- MouseEventArgs.cs
- PenThreadPool.cs
- BaseDataList.cs
- WeakReferenceEnumerator.cs
- TypefaceCollection.cs
- HandlerWithFactory.cs
- UIElementPropertyUndoUnit.cs
- JoinTreeNode.cs
- StrokeNodeEnumerator.cs
- MailBnfHelper.cs
- FixedDSBuilder.cs
- ProcessHostFactoryHelper.cs
- DesignTableCollection.cs
- ExternalFile.cs
- SchemaCollectionCompiler.cs
- HttpRequestCacheValidator.cs
- XamlDesignerSerializationManager.cs
- VarRefManager.cs
- log.cs
- TranslateTransform.cs
- MergeFailedEvent.cs
- GeometryCollection.cs
- AnimationClockResource.cs
- XmlSchemaInferenceException.cs
- XamlStream.cs
- AutomationPatternInfo.cs
- columnmapkeybuilder.cs
- TemplateLookupAction.cs
- AuthenticationException.cs
- ApplicationContext.cs
- XsdBuilder.cs
- COM2IDispatchConverter.cs
- FragmentNavigationEventArgs.cs
- PeerContact.cs