Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Stylus / PenThreadPool.cs / 1305600 / PenThreadPool.cs
using System; using System.Collections.Generic; using System.Threading; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using MS.Win32.Penimc; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// /// internal class PenThreadPool { ////// Critical - Constructor for singleton of our PenThreadPool. /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// Instance (above) /// /// [SecurityCritical] static PenThreadPool() { } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] [ThreadStatic] private static PenThreadPool _penThreadPool; ///////////////////////////////////////////////////////////////////// ////// ////// Critical - Returns a PenThread (creates as needed). /// marking this critical to prevent inadvertant access by transparent code /// /// Called by critical methods: /// PenContext.Dispose /// PenContext.Enable /// PenContext.Disable /// /// [SecurityCritical] internal static PenThread GetPenThreadForPenContext(PenContext penContext) { // Create the threadstatic DynamicRendererThreadManager as needed for calling thread. // It only creates one if (_penThreadPool == null) { _penThreadPool = new PenThreadPool(); } return _penThreadPool.GetPenThreadForPenContextHelper(penContext); // Adds to weak ref list if creating new one. } ///////////////////////////////////////////////////////////////////// ////// /// ////// Critical - marking this critical to prevent inadvertant /// access by transparent code /// /// [SecurityCritical] private List_penThreadWeakRefList; ///////////////////////////////////////////////////////////////////// /// /// /// ////// Critical - Initializes critical data: m_PenThreads /// /// [SecurityCritical] internal PenThreadPool() { _penThreadWeakRefList = new List(); } /// /// Critical - Calls SecurityCritical code (PenThread constructor). /// Called by BeginService. /// TreatAsSafe boundry is Stylus.EnableCore, Stylus.RegisterHwndForInput /// and HwndWrapperHook class (via HwndSource.InputFilterMessage). /// [SecurityCritical] private PenThread GetPenThreadForPenContextHelper(PenContext penContext) { bool needCleanup = false; PenThread penThread = null; int i; // Scan existing penthreads to see if we have an available slot for context. for (i=0; i < _penThreadWeakRefList.Count; i++) { PenThread penThreadFound = _penThreadWeakRefList[i].Target as PenThread; if (penThreadFound == null) { needCleanup = true; } else { // See if we can use this one if (penContext == null || penThreadFound.AddPenContext(penContext)) { // We can use this one. penThread = penThreadFound; break; } } } if (needCleanup) { // prune invalid refs for (i=_penThreadWeakRefList.Count - 1; i >= 0; i--) { if (_penThreadWeakRefList[i].Target == null) { _penThreadWeakRefList.RemoveAt(i); } } } if (penThread == null) { penThread = new PenThread(); // Make sure we add this context to the penthread if (penContext != null) { penThread.AddPenContext(penContext); } _penThreadWeakRefList.Add(new WeakReference(penThread)); } return penThread; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
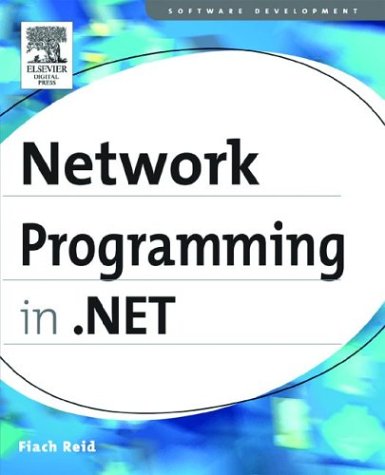
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SignerInfo.cs
- EmptyQuery.cs
- XmlCustomFormatter.cs
- Context.cs
- ValidatingReaderNodeData.cs
- DataSpaceManager.cs
- MultipartContentParser.cs
- X509CertificateCollection.cs
- DataBindingHandlerAttribute.cs
- ValueTypeFieldReference.cs
- WindowsSlider.cs
- DelegatingTypeDescriptionProvider.cs
- MatrixConverter.cs
- XmlCharCheckingReader.cs
- DiscriminatorMap.cs
- JsonEnumDataContract.cs
- SqlServer2KCompatibilityAnnotation.cs
- RowsCopiedEventArgs.cs
- QilTernary.cs
- PassportPrincipal.cs
- NeutralResourcesLanguageAttribute.cs
- WorkflowRuntime.cs
- RectangleConverter.cs
- EntityDataSourceConfigureObjectContext.cs
- EntityTypeEmitter.cs
- ResourcePart.cs
- SoapEnumAttribute.cs
- StyleCollectionEditor.cs
- CacheMemory.cs
- KeyNotFoundException.cs
- SafeSecurityHandles.cs
- HttpProfileBase.cs
- CollectionEditVerbManager.cs
- PlaceHolder.cs
- DataTemplate.cs
- OletxResourceManager.cs
- MultiTrigger.cs
- SchemaCompiler.cs
- NestedContainer.cs
- EndOfStreamException.cs
- TypeConverterAttribute.cs
- TableLayoutPanelDesigner.cs
- TokenizerHelper.cs
- PlanCompiler.cs
- GifBitmapDecoder.cs
- EntityException.cs
- CollectionContainer.cs
- ProxyWebPart.cs
- NavigatingCancelEventArgs.cs
- LineSegment.cs
- ToolStripContextMenu.cs
- DataSourceCache.cs
- TraceUtility.cs
- GridEntryCollection.cs
- KeyboardEventArgs.cs
- RoleService.cs
- querybuilder.cs
- FragmentQueryProcessor.cs
- ModelPerspective.cs
- HMACSHA1.cs
- SelectionPattern.cs
- IntegerValidatorAttribute.cs
- XmlQualifiedName.cs
- ObjectStateEntryDbDataRecord.cs
- Internal.cs
- PromptBuilder.cs
- ClockController.cs
- SystemIPInterfaceProperties.cs
- EventProvider.cs
- WorkflowOperationErrorHandler.cs
- ActiveXContainer.cs
- FeatureAttribute.cs
- ListManagerBindingsCollection.cs
- ParallelQuery.cs
- Visual3D.cs
- PeerNearMe.cs
- RepeatButton.cs
- ItemCheckEvent.cs
- RawKeyboardInputReport.cs
- SystemIPv6InterfaceProperties.cs
- LassoHelper.cs
- ModuleConfigurationInfo.cs
- NetworkStream.cs
- _Events.cs
- DBConnection.cs
- prompt.cs
- DesignerSerializerAttribute.cs
- BatchParser.cs
- TypeCollectionPropertyEditor.cs
- InvalidEnumArgumentException.cs
- StackOverflowException.cs
- OracleConnectionString.cs
- ToolStripTemplateNode.cs
- VectorConverter.cs
- SafeSecurityHelper.cs
- WebPartEditorApplyVerb.cs
- IsolatedStorageFile.cs
- LicenseProviderAttribute.cs
- XsltException.cs
- DSGeneratorProblem.cs