Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / BaseDataBoundControl.cs / 1305376 / BaseDataBoundControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.Util; ////// A BaseDataBoundControl is bound to a data source and generates its /// user interface (or child control hierarchy typically), by enumerating /// the items in the data source it is bound to. /// BaseDataBoundControl is an abstract base class that defines the common /// characteristics of all controls that use a list as a data source, such as /// DataGrid, DataBoundTable, ListBox etc. It encapsulates the logic /// of how a data-bound control binds to collections or DataControl instances. /// [ Designer("System.Web.UI.Design.WebControls.BaseDataBoundControlDesigner, " + AssemblyRef.SystemDesign), DefaultProperty("DataSourceID") ] public abstract class BaseDataBoundControl : WebControl { private static readonly object EventDataBound = new object(); private object _dataSource; private bool _requiresDataBinding; private bool _inited; private bool _preRendered; private bool _requiresBindToNull; private bool _throwOnDataPropertyChange; ////// The data source to bind to. This allows a BaseDataBoundControl to bind /// to arbitrary lists of data items. /// [ Bindable(true), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Themeable(false), WebCategory("Data"), WebSysDescription(SR.BaseDataBoundControl_DataSource), ] public virtual object DataSource { get { return _dataSource; } set { if (value != null) { ValidateDataSource(value); } _dataSource = value; OnDataPropertyChanged(); } } ////// The ID of the DataControl that this control should use to retrieve /// its data source. When the control is bound to a DataControl, it /// can retrieve a data source instance on-demand, and thereby attempt /// to work in auto-DataBind mode. /// [ DefaultValue(""), Themeable(false), WebCategory("Data"), WebSysDescription(SR.BaseDataBoundControl_DataSourceID) ] public virtual string DataSourceID { get { object o = ViewState["DataSourceID"]; if (o != null) { return (string)o; } return String.Empty; } set { if (String.IsNullOrEmpty(value) && !String.IsNullOrEmpty(DataSourceID)) { _requiresBindToNull = true; } ViewState["DataSourceID"] = value; OnDataPropertyChanged(); } } protected bool Initialized { get { return _inited; } } protected bool IsBoundUsingDataSourceID { get { return (DataSourceID.Length > 0); } } public override bool SupportsDisabledAttribute { get { return RenderingCompatibility < VersionUtil.Framework40; } } protected bool RequiresDataBinding { get { return _requiresDataBinding; } set { // if we have to play catch-up here because we've already PreRendered, call EnsureDataBound if (value && _preRendered && DataSourceID.Length > 0 && Page != null && !Page.IsCallback) { _requiresDataBinding = true; EnsureDataBound(); } else { _requiresDataBinding = value; } } } [ WebCategory("Data"), WebSysDescription(SR.BaseDataBoundControl_OnDataBound) ] public event EventHandler DataBound { add { Events.AddHandler(EventDataBound, value); } remove { Events.RemoveHandler(EventDataBound, value); } } protected void ConfirmInitState() { _inited = true; // do this in OnLoad in case we were added to the page after Page.OnPreLoad } ////// Overriden by BaseDataBoundControl to use its properties to determine the real /// data source that the control should bind to. It then clears the existing /// control hierarchy, and calls CreateChildControls to create a new control /// hierarchy based on the resolved data source. /// The implementation resolves various data source related properties to /// arrive at the appropriate IEnumerable implementation to use as the real /// data source. /// When resolving data sources, the DataSourceID takes highest precedence. /// If DataSourceID is not set, the value of the DataSource property is used. /// In this second alternative, DataMember is used to extract the appropriate /// list if the control has been handed an IListSource as a data source. /// /// Data bound controls should override PerformDataBinding instead /// of DataBind. If DataBind if overridden, the OnDataBinding and OnDataBound events will /// fire in the wrong order. However, for backwards compat on ListControl and AdRotator, we /// can't seal this method. It is sealed on all new BaseDataBoundControl-derived controls. /// public override void DataBind() { // Don't databind when the control is in the designer but not top-level if (DesignMode) { IDictionary designModeState = GetDesignModeState(); if (((designModeState == null) || (designModeState["EnableDesignTimeDataBinding"] == null)) && (Site == null)) { return; } } PerformSelect(); } protected virtual void EnsureDataBound() { try { _throwOnDataPropertyChange = true; if (RequiresDataBinding && (DataSourceID.Length > 0 || _requiresBindToNull)) { DataBind(); _requiresBindToNull = false; } } finally { _throwOnDataPropertyChange = false; } } protected virtual void OnDataBound(EventArgs e) { EventHandler handler = Events[EventDataBound] as EventHandler; if (handler != null) { handler(this, e); } } ////// This method is called when DataMember, DataSource, or DataSourceID is changed. /// protected virtual void OnDataPropertyChanged() { if (_throwOnDataPropertyChange) { throw new HttpException(SR.GetString(SR.DataBoundControl_InvalidDataPropertyChange, ID)); } if (_inited) { RequiresDataBinding = true; } } protected internal override void OnInit(EventArgs e) { base.OnInit(e); if (Page != null) { Page.PreLoad += new EventHandler(this.OnPagePreLoad); if (!IsViewStateEnabled && Page.IsPostBack) { RequiresDataBinding = true; } } } protected virtual void OnPagePreLoad(object sender, EventArgs e) { _inited = true; if (Page != null) { Page.PreLoad -= new EventHandler(this.OnPagePreLoad); } } protected internal override void OnPreRender(EventArgs e) { _preRendered = true; EnsureDataBound(); base.OnPreRender(e); } ////// Override to control how the data is selected and the control is databound. /// protected abstract void PerformSelect(); protected abstract void ValidateDataSource(object dataSource); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.Util; ////// A BaseDataBoundControl is bound to a data source and generates its /// user interface (or child control hierarchy typically), by enumerating /// the items in the data source it is bound to. /// BaseDataBoundControl is an abstract base class that defines the common /// characteristics of all controls that use a list as a data source, such as /// DataGrid, DataBoundTable, ListBox etc. It encapsulates the logic /// of how a data-bound control binds to collections or DataControl instances. /// [ Designer("System.Web.UI.Design.WebControls.BaseDataBoundControlDesigner, " + AssemblyRef.SystemDesign), DefaultProperty("DataSourceID") ] public abstract class BaseDataBoundControl : WebControl { private static readonly object EventDataBound = new object(); private object _dataSource; private bool _requiresDataBinding; private bool _inited; private bool _preRendered; private bool _requiresBindToNull; private bool _throwOnDataPropertyChange; ////// The data source to bind to. This allows a BaseDataBoundControl to bind /// to arbitrary lists of data items. /// [ Bindable(true), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), Themeable(false), WebCategory("Data"), WebSysDescription(SR.BaseDataBoundControl_DataSource), ] public virtual object DataSource { get { return _dataSource; } set { if (value != null) { ValidateDataSource(value); } _dataSource = value; OnDataPropertyChanged(); } } ////// The ID of the DataControl that this control should use to retrieve /// its data source. When the control is bound to a DataControl, it /// can retrieve a data source instance on-demand, and thereby attempt /// to work in auto-DataBind mode. /// [ DefaultValue(""), Themeable(false), WebCategory("Data"), WebSysDescription(SR.BaseDataBoundControl_DataSourceID) ] public virtual string DataSourceID { get { object o = ViewState["DataSourceID"]; if (o != null) { return (string)o; } return String.Empty; } set { if (String.IsNullOrEmpty(value) && !String.IsNullOrEmpty(DataSourceID)) { _requiresBindToNull = true; } ViewState["DataSourceID"] = value; OnDataPropertyChanged(); } } protected bool Initialized { get { return _inited; } } protected bool IsBoundUsingDataSourceID { get { return (DataSourceID.Length > 0); } } public override bool SupportsDisabledAttribute { get { return RenderingCompatibility < VersionUtil.Framework40; } } protected bool RequiresDataBinding { get { return _requiresDataBinding; } set { // if we have to play catch-up here because we've already PreRendered, call EnsureDataBound if (value && _preRendered && DataSourceID.Length > 0 && Page != null && !Page.IsCallback) { _requiresDataBinding = true; EnsureDataBound(); } else { _requiresDataBinding = value; } } } [ WebCategory("Data"), WebSysDescription(SR.BaseDataBoundControl_OnDataBound) ] public event EventHandler DataBound { add { Events.AddHandler(EventDataBound, value); } remove { Events.RemoveHandler(EventDataBound, value); } } protected void ConfirmInitState() { _inited = true; // do this in OnLoad in case we were added to the page after Page.OnPreLoad } ////// Overriden by BaseDataBoundControl to use its properties to determine the real /// data source that the control should bind to. It then clears the existing /// control hierarchy, and calls CreateChildControls to create a new control /// hierarchy based on the resolved data source. /// The implementation resolves various data source related properties to /// arrive at the appropriate IEnumerable implementation to use as the real /// data source. /// When resolving data sources, the DataSourceID takes highest precedence. /// If DataSourceID is not set, the value of the DataSource property is used. /// In this second alternative, DataMember is used to extract the appropriate /// list if the control has been handed an IListSource as a data source. /// /// Data bound controls should override PerformDataBinding instead /// of DataBind. If DataBind if overridden, the OnDataBinding and OnDataBound events will /// fire in the wrong order. However, for backwards compat on ListControl and AdRotator, we /// can't seal this method. It is sealed on all new BaseDataBoundControl-derived controls. /// public override void DataBind() { // Don't databind when the control is in the designer but not top-level if (DesignMode) { IDictionary designModeState = GetDesignModeState(); if (((designModeState == null) || (designModeState["EnableDesignTimeDataBinding"] == null)) && (Site == null)) { return; } } PerformSelect(); } protected virtual void EnsureDataBound() { try { _throwOnDataPropertyChange = true; if (RequiresDataBinding && (DataSourceID.Length > 0 || _requiresBindToNull)) { DataBind(); _requiresBindToNull = false; } } finally { _throwOnDataPropertyChange = false; } } protected virtual void OnDataBound(EventArgs e) { EventHandler handler = Events[EventDataBound] as EventHandler; if (handler != null) { handler(this, e); } } ////// This method is called when DataMember, DataSource, or DataSourceID is changed. /// protected virtual void OnDataPropertyChanged() { if (_throwOnDataPropertyChange) { throw new HttpException(SR.GetString(SR.DataBoundControl_InvalidDataPropertyChange, ID)); } if (_inited) { RequiresDataBinding = true; } } protected internal override void OnInit(EventArgs e) { base.OnInit(e); if (Page != null) { Page.PreLoad += new EventHandler(this.OnPagePreLoad); if (!IsViewStateEnabled && Page.IsPostBack) { RequiresDataBinding = true; } } } protected virtual void OnPagePreLoad(object sender, EventArgs e) { _inited = true; if (Page != null) { Page.PreLoad -= new EventHandler(this.OnPagePreLoad); } } protected internal override void OnPreRender(EventArgs e) { _preRendered = true; EnsureDataBound(); base.OnPreRender(e); } ////// Override to control how the data is selected and the control is databound. /// protected abstract void PerformSelect(); protected abstract void ValidateDataSource(object dataSource); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
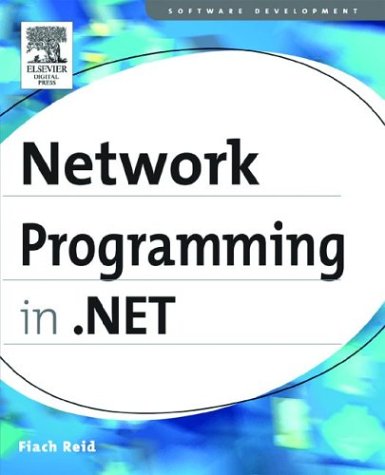
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NameValueConfigurationCollection.cs
- TextFragmentEngine.cs
- sqlinternaltransaction.cs
- MonthCalendar.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- DataGridViewLayoutData.cs
- SHA384Managed.cs
- Int16Converter.cs
- Point3DAnimationUsingKeyFrames.cs
- ViewStateException.cs
- SizeLimitedCache.cs
- DataObjectEventArgs.cs
- DataGridRowEventArgs.cs
- VectorCollectionConverter.cs
- TemplateBindingExpression.cs
- ProfileInfo.cs
- RecommendedAsConfigurableAttribute.cs
- mongolianshape.cs
- AppendHelper.cs
- CalendarSelectionChangedEventArgs.cs
- EntityTemplateFactory.cs
- _NtlmClient.cs
- CodeStatement.cs
- TraceProvider.cs
- Symbol.cs
- TextFormatter.cs
- COAUTHIDENTITY.cs
- DependencyPropertyValueSerializer.cs
- RoleService.cs
- GridEntryCollection.cs
- BackgroundWorker.cs
- BitmapSource.cs
- Sorting.cs
- SlipBehavior.cs
- _ConnectionGroup.cs
- ApplicationDirectory.cs
- SafeSystemMetrics.cs
- StringAnimationBase.cs
- DictionarySectionHandler.cs
- CompilerLocalReference.cs
- HandleRef.cs
- HttpVersion.cs
- CodeGotoStatement.cs
- CompositeDataBoundControl.cs
- CodeTypeReferenceCollection.cs
- MultiBinding.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- RowType.cs
- FillRuleValidation.cs
- StreamGeometry.cs
- OrderByBuilder.cs
- WebPartConnectVerb.cs
- BindingNavigator.cs
- TreeNodeStyle.cs
- GenericTextProperties.cs
- SimpleWorkerRequest.cs
- SoapCodeExporter.cs
- WebPartConnectionsConnectVerb.cs
- ContextTokenTypeConverter.cs
- TypeSemantics.cs
- HtmlElementCollection.cs
- WasAdminWrapper.cs
- ArgumentOutOfRangeException.cs
- CompletedAsyncResult.cs
- RightNameExpirationInfoPair.cs
- TreeChangeInfo.cs
- OdbcDataReader.cs
- BeginStoryboard.cs
- HostSecurityManager.cs
- ProtectedProviderSettings.cs
- GenericTextProperties.cs
- DefaultParameterValueAttribute.cs
- ResponseBodyWriter.cs
- Win32PrintDialog.cs
- XPathBuilder.cs
- GlyphInfoList.cs
- SiteMapPath.cs
- UnaryNode.cs
- XPathSelectionIterator.cs
- NativeMethods.cs
- AccessibleObject.cs
- Compiler.cs
- FastEncoder.cs
- DataGridViewAutoSizeModeEventArgs.cs
- PipelineModuleStepContainer.cs
- DaylightTime.cs
- hresults.cs
- FrameworkReadOnlyPropertyMetadata.cs
- WebPartMovingEventArgs.cs
- formatter.cs
- ConfigurationManagerHelper.cs
- InheritanceContextHelper.cs
- ProfileInfo.cs
- ClipboardProcessor.cs
- BamlLocalizationDictionary.cs
- IntranetCredentialPolicy.cs
- FilterElement.cs
- XmlSchemaParticle.cs
- ToggleProviderWrapper.cs
- HashMembershipCondition.cs