Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Runtime / Serialization / Formatters / SoapFault.cs / 1305376 / SoapFault.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SoapFault ** **Author: Peter de Jong ([....]) ** ** Purpose: Specifies information for a Soap Fault ** ** Date: June 27, 2000 ** ===========================================================*/ #if FEATURE_REMOTING namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Metadata; using System.Globalization; using System.Security.Permissions; //* Class holds soap fault information [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class SoapFault : ISerializable { String faultCode; String faultString; String faultActor; [SoapField(Embedded=true)] Object detail; public SoapFault() { } public SoapFault(String faultCode, String faultString, String faultActor, ServerFault serverFault) { this.faultCode = faultCode; this.faultString = faultString; this.faultActor = faultActor; this.detail = serverFault; } internal SoapFault(SerializationInfo info, StreamingContext context) { SerializationInfoEnumerator siEnum = info.GetEnumerator(); while(siEnum.MoveNext()) { String name = siEnum.Name; Object value = siEnum.Value; SerTrace.Log(this, "SetObjectData enum ",name," value ",value); if (String.Compare(name, "faultCode", true, CultureInfo.InvariantCulture) == 0) { int index = ((String)value).IndexOf(':'); if (index > -1) faultCode = ((String)value).Substring(++index); else faultCode = (String)value; } else if (String.Compare(name, "faultString", true, CultureInfo.InvariantCulture) == 0) faultString = (String)value; else if (String.Compare(name, "faultActor", true, CultureInfo.InvariantCulture) == 0) faultActor = (String)value; else if (String.Compare(name, "detail", true, CultureInfo.InvariantCulture) == 0) detail = value; } } [System.Security.SecurityCritical] // auto-generated_required public void GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("faultcode", "SOAP-ENV:"+faultCode); info.AddValue("faultstring", faultString); if (faultActor != null) info.AddValue("faultactor", faultActor); info.AddValue("detail", detail, typeof(Object)); } public String FaultCode { get {return faultCode;} set { faultCode = value;} } public String FaultString { get {return faultString;} set { faultString = value;} } public String FaultActor { get {return faultActor;} set { faultActor = value;} } public Object Detail { get {return detail;} set {detail = value;} } } [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class ServerFault { String exceptionType; String message; String stackTrace; Exception exception; internal ServerFault(Exception exception) { this.exception = exception; //this.exceptionType = exception.GetType().AssemblyQualifiedName; //this.message = exception.Message; } public ServerFault(String exceptionType, String message, String stackTrace) { this.exceptionType = exceptionType; this.message = message; this.stackTrace = stackTrace; } public String ExceptionType { get {return exceptionType;} set { exceptionType = value;} } public String ExceptionMessage { get {return message;} set { message = value;} } public String StackTrace { get {return stackTrace;} set {stackTrace = value;} } internal Exception Exception { get {return exception;} } } } #endif // FEATURE_REMOTING // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SoapFault ** **Author: Peter de Jong ([....]) ** ** Purpose: Specifies information for a Soap Fault ** ** Date: June 27, 2000 ** ===========================================================*/ #if FEATURE_REMOTING namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Metadata; using System.Globalization; using System.Security.Permissions; //* Class holds soap fault information [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class SoapFault : ISerializable { String faultCode; String faultString; String faultActor; [SoapField(Embedded=true)] Object detail; public SoapFault() { } public SoapFault(String faultCode, String faultString, String faultActor, ServerFault serverFault) { this.faultCode = faultCode; this.faultString = faultString; this.faultActor = faultActor; this.detail = serverFault; } internal SoapFault(SerializationInfo info, StreamingContext context) { SerializationInfoEnumerator siEnum = info.GetEnumerator(); while(siEnum.MoveNext()) { String name = siEnum.Name; Object value = siEnum.Value; SerTrace.Log(this, "SetObjectData enum ",name," value ",value); if (String.Compare(name, "faultCode", true, CultureInfo.InvariantCulture) == 0) { int index = ((String)value).IndexOf(':'); if (index > -1) faultCode = ((String)value).Substring(++index); else faultCode = (String)value; } else if (String.Compare(name, "faultString", true, CultureInfo.InvariantCulture) == 0) faultString = (String)value; else if (String.Compare(name, "faultActor", true, CultureInfo.InvariantCulture) == 0) faultActor = (String)value; else if (String.Compare(name, "detail", true, CultureInfo.InvariantCulture) == 0) detail = value; } } [System.Security.SecurityCritical] // auto-generated_required public void GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("faultcode", "SOAP-ENV:"+faultCode); info.AddValue("faultstring", faultString); if (faultActor != null) info.AddValue("faultactor", faultActor); info.AddValue("detail", detail, typeof(Object)); } public String FaultCode { get {return faultCode;} set { faultCode = value;} } public String FaultString { get {return faultString;} set { faultString = value;} } public String FaultActor { get {return faultActor;} set { faultActor = value;} } public Object Detail { get {return detail;} set {detail = value;} } } [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class ServerFault { String exceptionType; String message; String stackTrace; Exception exception; internal ServerFault(Exception exception) { this.exception = exception; //this.exceptionType = exception.GetType().AssemblyQualifiedName; //this.message = exception.Message; } public ServerFault(String exceptionType, String message, String stackTrace) { this.exceptionType = exceptionType; this.message = message; this.stackTrace = stackTrace; } public String ExceptionType { get {return exceptionType;} set { exceptionType = value;} } public String ExceptionMessage { get {return message;} set { message = value;} } public String StackTrace { get {return stackTrace;} set {stackTrace = value;} } internal Exception Exception { get {return exception;} } } } #endif // FEATURE_REMOTING // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
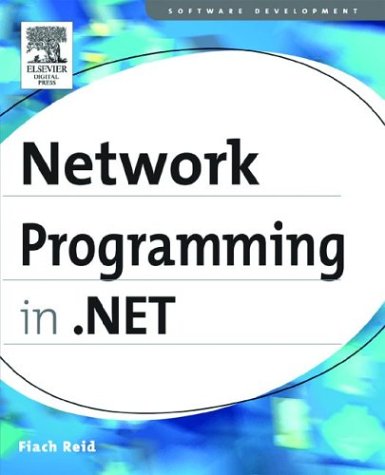
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIPermission.cs
- XmlSchemaValidator.cs
- UniformGrid.cs
- listviewsubitemcollectioneditor.cs
- QueryResponse.cs
- sqlnorm.cs
- CompleteWizardStep.cs
- NameValueCollection.cs
- MLangCodePageEncoding.cs
- LinearGradientBrush.cs
- ResourceDisplayNameAttribute.cs
- IISUnsafeMethods.cs
- MethodImplAttribute.cs
- ToolStripButton.cs
- DataGridTextBoxColumn.cs
- XAMLParseException.cs
- ListBindableAttribute.cs
- SHA1CryptoServiceProvider.cs
- clipboard.cs
- XmlReflectionImporter.cs
- ElementNotEnabledException.cs
- DataPager.cs
- RoutedEventValueSerializer.cs
- InternalCache.cs
- ImageDrawing.cs
- HelloMessage11.cs
- OrthographicCamera.cs
- MethodRental.cs
- TransactionManager.cs
- SqlProviderServices.cs
- WithStatement.cs
- RequestCachePolicy.cs
- CircleHotSpot.cs
- OracleConnectionString.cs
- Stackframe.cs
- InternalResources.cs
- ParsedAttributeCollection.cs
- ConditionalExpression.cs
- BuildResult.cs
- TextServicesLoader.cs
- ImageListDesigner.cs
- IDispatchConstantAttribute.cs
- ItemsControl.cs
- SettingsPropertyValue.cs
- UseLicense.cs
- CodeValidator.cs
- WrapPanel.cs
- EndSelectCardRequest.cs
- SapiAttributeParser.cs
- ProviderConnectionPoint.cs
- ProtectedConfigurationSection.cs
- ArrangedElementCollection.cs
- QilVisitor.cs
- DataContractSerializerOperationBehavior.cs
- EventEntry.cs
- XomlCompilerResults.cs
- SafeFileHandle.cs
- TemplateBaseAction.cs
- CachedRequestParams.cs
- _SslState.cs
- QuaternionKeyFrameCollection.cs
- MutexSecurity.cs
- _NTAuthentication.cs
- EventLogTraceListener.cs
- FormViewInsertedEventArgs.cs
- XPathBuilder.cs
- FontStyleConverter.cs
- XmlMapping.cs
- ICspAsymmetricAlgorithm.cs
- AutoResizedEvent.cs
- IPipelineRuntime.cs
- FastPropertyAccessor.cs
- RefreshInfo.cs
- SqlCommand.cs
- HiddenFieldPageStatePersister.cs
- EditCommandColumn.cs
- PropertyValueChangedEvent.cs
- ApplicationFileParser.cs
- RegexCharClass.cs
- XmlQueryType.cs
- WebSysDisplayNameAttribute.cs
- BevelBitmapEffect.cs
- InvokeMemberBinder.cs
- EdmToObjectNamespaceMap.cs
- ServiceReflector.cs
- GlobalDataBindingHandler.cs
- _Events.cs
- XmlSchemaNotation.cs
- TypeHelpers.cs
- RootBrowserWindowAutomationPeer.cs
- TextSelection.cs
- Predicate.cs
- FixedBufferAttribute.cs
- XmlAttributeCollection.cs
- Win32.cs
- ImmutableAssemblyCacheEntry.cs
- BufferAllocator.cs
- SimpleBitVector32.cs
- validationstate.cs
- XslVisitor.cs