Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / ColumnTypeConverter.cs / 1 / ColumnTypeConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- /* */ namespace System.Data { using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Data.SqlTypes; ////// internal sealed class ColumnTypeConverter : TypeConverter { private static Type[] types = new Type[] { typeof(Boolean), typeof(Byte), typeof(Byte[]), typeof(Char), typeof(DateTime), typeof(Decimal), typeof(Double), typeof(Guid), typeof(Int16), typeof(Int32), typeof(Int64), typeof(object), typeof(SByte), typeof(Single), typeof(string), typeof(TimeSpan), typeof(UInt16), typeof(UInt32), typeof(UInt64), typeof(SqlInt16), typeof(SqlInt32), typeof(SqlInt64), typeof(SqlDecimal), typeof(SqlSingle), typeof(SqlDouble), typeof(SqlString), typeof(SqlBoolean), typeof(SqlBinary), typeof(SqlByte), typeof(SqlDateTime), typeof(SqlGuid), typeof(SqlMoney), typeof(SqlBytes), typeof(SqlChars), typeof(SqlXml) }; private StandardValuesCollection values; // converter classes should have public ctor public ColumnTypeConverter() { } ///Provides a type /// converter that can be used to populate a list box with available types. ////// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { if (value == null) { return String.Empty; } else { value.ToString(); } } if (value != null && destinationType == typeof(InstanceDescriptor)) { Object newValue = value; if (value is string) { for (int i = 0; i < types.Length; i++) { if (types[i].ToString().Equals(value)) newValue = types[i]; } } if (value is Type || value is string) { System.Reflection.MethodInfo method = typeof(Type).GetMethod("GetType", new Type[] {typeof(string)}); // change done for security review if (method != null) { return new InstanceDescriptor(method, new object[] {((Type)newValue).AssemblyQualifiedName}); } } } return base.ConvertTo(context, culture, value, destinationType); } public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertTo(context, sourceType); } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value != null && value.GetType() == typeof(string)) { for (int i = 0; i < types.Length; i++) { if (types[i].ToString().Equals(value)) return types[i]; } return typeof(string); } return base.ConvertFrom(context, culture, value); } ///Converts the given value object to the specified destination type. ////// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { object[] objTypes; if (types != null) { objTypes = new object[types.Length]; Array.Copy(types, objTypes, types.Length); } else { objTypes = null; } values = new StandardValuesCollection(objTypes); } return values; } ///Gets a collection of standard values for the data type this validator is /// designed for. ////// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return true; } ///Gets a value indicating whether the list of standard values returned from /// ///is an exclusive list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Gets a value indicating whether this object supports a /// standard set of values that can be picked from a list using the specified /// context. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- /* */ namespace System.Data { using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Data.SqlTypes; ////// internal sealed class ColumnTypeConverter : TypeConverter { private static Type[] types = new Type[] { typeof(Boolean), typeof(Byte), typeof(Byte[]), typeof(Char), typeof(DateTime), typeof(Decimal), typeof(Double), typeof(Guid), typeof(Int16), typeof(Int32), typeof(Int64), typeof(object), typeof(SByte), typeof(Single), typeof(string), typeof(TimeSpan), typeof(UInt16), typeof(UInt32), typeof(UInt64), typeof(SqlInt16), typeof(SqlInt32), typeof(SqlInt64), typeof(SqlDecimal), typeof(SqlSingle), typeof(SqlDouble), typeof(SqlString), typeof(SqlBoolean), typeof(SqlBinary), typeof(SqlByte), typeof(SqlDateTime), typeof(SqlGuid), typeof(SqlMoney), typeof(SqlBytes), typeof(SqlChars), typeof(SqlXml) }; private StandardValuesCollection values; // converter classes should have public ctor public ColumnTypeConverter() { } ///Provides a type /// converter that can be used to populate a list box with available types. ////// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { if (value == null) { return String.Empty; } else { value.ToString(); } } if (value != null && destinationType == typeof(InstanceDescriptor)) { Object newValue = value; if (value is string) { for (int i = 0; i < types.Length; i++) { if (types[i].ToString().Equals(value)) newValue = types[i]; } } if (value is Type || value is string) { System.Reflection.MethodInfo method = typeof(Type).GetMethod("GetType", new Type[] {typeof(string)}); // change done for security review if (method != null) { return new InstanceDescriptor(method, new object[] {((Type)newValue).AssemblyQualifiedName}); } } } return base.ConvertTo(context, culture, value, destinationType); } public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertTo(context, sourceType); } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value != null && value.GetType() == typeof(string)) { for (int i = 0; i < types.Length; i++) { if (types[i].ToString().Equals(value)) return types[i]; } return typeof(string); } return base.ConvertFrom(context, culture, value); } ///Converts the given value object to the specified destination type. ////// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { object[] objTypes; if (types != null) { objTypes = new object[types.Length]; Array.Copy(types, objTypes, types.Length); } else { objTypes = null; } values = new StandardValuesCollection(objTypes); } return values; } ///Gets a collection of standard values for the data type this validator is /// designed for. ////// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return true; } ///Gets a value indicating whether the list of standard values returned from /// ///is an exclusive list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Gets a value indicating whether this object supports a /// standard set of values that can be picked from a list using the specified /// context. ///
Link Menu
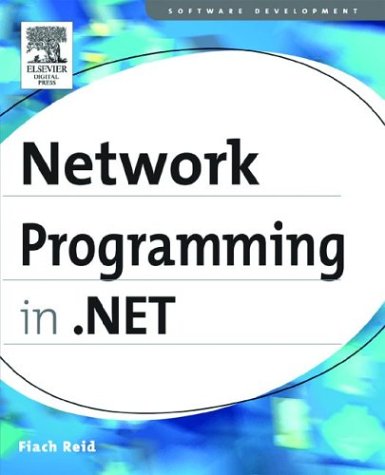
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Compress.cs
- OverlappedAsyncResult.cs
- ReflectTypeDescriptionProvider.cs
- DurableMessageDispatchInspector.cs
- DecodeHelper.cs
- FlowDocumentPaginator.cs
- NullExtension.cs
- ServiceRouteHandler.cs
- SmtpNetworkElement.cs
- SettingsProperty.cs
- FieldNameLookup.cs
- KeyProperty.cs
- ValidationRuleCollection.cs
- UpDownBase.cs
- SqlDataSourceView.cs
- HScrollProperties.cs
- Listbox.cs
- DataGridViewHeaderCell.cs
- XmlStreamNodeWriter.cs
- DesignerAttributeInfo.cs
- ProcessHostServerConfig.cs
- ListViewSortEventArgs.cs
- StateManagedCollection.cs
- FolderNameEditor.cs
- SafeHandle.cs
- WaitForChangedResult.cs
- EdmComplexPropertyAttribute.cs
- FontNamesConverter.cs
- XdrBuilder.cs
- SiteMapNodeItem.cs
- BamlLocalizabilityResolver.cs
- XmlQueryContext.cs
- Pointer.cs
- EdmProviderManifest.cs
- xmlformatgeneratorstatics.cs
- StructuredTypeEmitter.cs
- StrokeIntersection.cs
- FieldToken.cs
- RoutingEndpointTrait.cs
- HttpResponseMessageProperty.cs
- SystemDropShadowChrome.cs
- SiteMapNodeCollection.cs
- BatchParser.cs
- DataGridViewCellMouseEventArgs.cs
- storepermissionattribute.cs
- InvalidPrinterException.cs
- DataGridColumnCollection.cs
- Serializer.cs
- ControllableStoryboardAction.cs
- TypeBuilderInstantiation.cs
- ContractsBCL.cs
- DataObjectFieldAttribute.cs
- GuidelineSet.cs
- SmtpClient.cs
- RequestResponse.cs
- XmlDigitalSignatureProcessor.cs
- AbstractSvcMapFileLoader.cs
- EasingFunctionBase.cs
- CompilerTypeWithParams.cs
- TextPenaltyModule.cs
- PreparingEnlistment.cs
- WindowClosedEventArgs.cs
- SelectiveScrollingGrid.cs
- _SSPISessionCache.cs
- RMEnrollmentPage2.cs
- PerfService.cs
- CodeParameterDeclarationExpression.cs
- RequestResizeEvent.cs
- WindowsEditBox.cs
- iisPickupDirectory.cs
- Property.cs
- Renderer.cs
- SafeProcessHandle.cs
- DefaultDiscoveryService.cs
- FormDesigner.cs
- TemplatedWizardStep.cs
- MouseButton.cs
- ClientRuntimeConfig.cs
- BitVector32.cs
- _KerberosClient.cs
- AmbiguousMatchException.cs
- NativeMethods.cs
- XmlArrayItemAttributes.cs
- HttpCachePolicyElement.cs
- CompiledQuery.cs
- XmlCharCheckingWriter.cs
- ConditionalAttribute.cs
- PropertyGrid.cs
- Pick.cs
- Thread.cs
- PagerSettings.cs
- _NTAuthentication.cs
- GridViewItemAutomationPeer.cs
- RuleSetDialog.cs
- DbMetaDataCollectionNames.cs
- PrintDialog.cs
- securestring.cs
- ReferentialConstraint.cs
- QualifiedCellIdBoolean.cs
- CurrentChangingEventArgs.cs