Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / OutputBuffer.cs / 1305376 / OutputBuffer.cs
namespace System.IO.Compression { using System.Diagnostics; internal class OutputBuffer { private byte[] byteBuffer; // buffer for storing bytes private int pos; // position private uint bitBuf; // store uncomplete bits private int bitCount; // number of bits in bitBuffer // set the output buffer we will be using internal void UpdateBuffer(byte[] output) { byteBuffer = output; pos = 0; } internal int BytesWritten { get { return pos; } } internal int FreeBytes { get { return byteBuffer.Length - pos; } } internal void WriteUInt16(ushort value) { Debug.Assert(FreeBytes >= 2, "No enough space in output buffer!"); byteBuffer[pos++] = (byte)value; byteBuffer[pos++] = (byte)(value >> 8); } internal void WriteBits(int n, uint bits) { Debug.Assert(n <= 16, "length must be larger than 16!"); bitBuf |= bits << bitCount; bitCount += n; if (bitCount >= 16) { Debug.Assert(byteBuffer.Length - pos >= 2, "No enough space in output buffer!"); byteBuffer[pos++] = unchecked((byte)bitBuf); byteBuffer[pos++] = unchecked((byte)(bitBuf >> 8)); bitCount -= 16; bitBuf >>= 16; } } // write the bits left in the output as bytes. internal void FlushBits() { // flush bits from bit buffer to output buffer while (bitCount >= 8) { byteBuffer[pos++] = unchecked((byte)bitBuf); bitCount -= 8; bitBuf >>= 8; } if (bitCount > 0) { byteBuffer[pos++] = unchecked((byte)bitBuf); bitBuf = 0; bitCount = 0; } } internal void WriteBytes(byte[] byteArray, int offset, int count) { Debug.Assert(FreeBytes >= count, "Not enough space in output buffer!"); // faster if (bitCount == 0) { Array.Copy(byteArray, offset, byteBuffer, pos, count); pos += count; } else { WriteBytesUnaligned(byteArray, offset, count); } } private void WriteBytesUnaligned(byte[] byteArray, int offset, int count) { for (int i = 0; i < count; i++) { byte b = byteArray[offset + i]; WriteByteUnaligned(b); } } private void WriteByteUnaligned(byte b) { WriteBits(8, b); } internal int BitsInBuffer { get { return (bitCount / 8) + 1; } } internal OutputBuffer.BufferState DumpState() { OutputBuffer.BufferState savedState; savedState.pos = pos; savedState.bitBuf = bitBuf; savedState.bitCount = bitCount; return savedState; } internal void RestoreState(OutputBuffer.BufferState state) { pos = state.pos; bitBuf = state.bitBuf; bitCount = state.bitCount; } internal struct BufferState { internal int pos; // position internal uint bitBuf; // store uncomplete bits internal int bitCount; // number of bits in bitBuffer } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
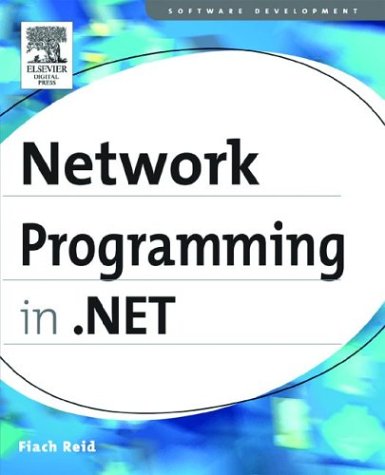
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeNameConverter.cs
- RequestedSignatureDialog.cs
- NativeMethods.cs
- TimeEnumHelper.cs
- StatusBarPanelClickEvent.cs
- MediaTimeline.cs
- PathNode.cs
- XmlSignatureProperties.cs
- XmlDataImplementation.cs
- SqlDelegatedTransaction.cs
- Operators.cs
- BufferedStream.cs
- Compiler.cs
- GridPattern.cs
- PeerResolverBindingElement.cs
- SupportsPreviewControlAttribute.cs
- DbConnectionPoolOptions.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- BamlRecords.cs
- SharedDp.cs
- ExpressionParser.cs
- PasswordDeriveBytes.cs
- XmlWrappingReader.cs
- FloaterBaseParagraph.cs
- PropertyEntry.cs
- XslAst.cs
- PathFigureCollection.cs
- ListSourceHelper.cs
- PenLineJoinValidation.cs
- TraceListener.cs
- ListArgumentProvider.cs
- QueryTreeBuilder.cs
- ServiceHttpHandlerFactory.cs
- EventLogEntryCollection.cs
- ConfigurationSectionGroupCollection.cs
- Semaphore.cs
- DataGridSortCommandEventArgs.cs
- CrossSiteScriptingValidation.cs
- DataGridCell.cs
- IdentifierElement.cs
- WebBrowserPermission.cs
- ReflectEventDescriptor.cs
- ChangeBlockUndoRecord.cs
- PropertyItem.cs
- StringValidatorAttribute.cs
- Pair.cs
- TypeBrowser.xaml.cs
- smtppermission.cs
- SendDesigner.xaml.cs
- QilInvokeLateBound.cs
- RtfControlWordInfo.cs
- AbstractSvcMapFileLoader.cs
- XmlSchemaSimpleTypeRestriction.cs
- RoleBoolean.cs
- SystemInformation.cs
- HtmlTableRow.cs
- precedingsibling.cs
- NetSectionGroup.cs
- NavigatorOutput.cs
- GlobalProxySelection.cs
- LazyTextWriterCreator.cs
- Binding.cs
- RayHitTestParameters.cs
- InitializerFacet.cs
- SimpleType.cs
- ApplicationInterop.cs
- RegionIterator.cs
- SecurityPolicySection.cs
- TextServicesDisplayAttribute.cs
- TdsParserStaticMethods.cs
- MimeWriter.cs
- ImportDesigner.xaml.cs
- BindMarkupExtensionSerializer.cs
- Utilities.cs
- IDispatchConstantAttribute.cs
- GeneralTransform3D.cs
- Pkcs7Signer.cs
- ResXBuildProvider.cs
- CompModSwitches.cs
- GridViewDesigner.cs
- StringUtil.cs
- RtfControlWordInfo.cs
- OdbcParameterCollection.cs
- HyperlinkAutomationPeer.cs
- ClaimTypeRequirement.cs
- ToolStripDropDownClosingEventArgs.cs
- EnumValidator.cs
- FormattedTextSymbols.cs
- HierarchicalDataBoundControl.cs
- WizardPanelChangingEventArgs.cs
- MenuEventArgs.cs
- InputLanguageProfileNotifySink.cs
- InfoCardAsymmetricCrypto.cs
- TrackingRecord.cs
- GenericsInstances.cs
- HttpCookieCollection.cs
- TextEditorThreadLocalStore.cs
- ScrollChrome.cs
- DataGridColumnHeaderCollection.cs
- SystemFonts.cs