Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripGrip.cs / 2 / ToolStripGrip.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.VisualStyles; using System.Windows.Forms.Layout; using System.Diagnostics; ////// internal class ToolStripGrip : ToolStripButton { private Cursor oldCursor; private int gripThickness = 0; Point startLocation = Point.Empty; private bool movingToolStrip = false; private Point lastEndLocation = ToolStrip.InvalidMouseEnter; private static Size DragSize = LayoutUtils.MaxSize; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] internal ToolStripGrip() { // if we're using XP themes we've got to be a bit thicker. gripThickness = ToolStripManager.VisualStylesEnabled ? 5 : 3; SupportsItemClick = false; } /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected internal override Padding DefaultMargin { get { return new Padding(2); } } public override bool CanSelect { get { return false; } } internal int GripThickness { get { return gripThickness; } } internal bool MovingToolStrip { get { return ((ToolStripPanelRow != null) && movingToolStrip); } set { if ((movingToolStrip != value) && ParentInternal != null) { if (value) { // dont let grips move the toolstrip if (ParentInternal.ToolStripPanelRow == null) { return; } } movingToolStrip = value; lastEndLocation = ToolStrip.InvalidMouseEnter; if (movingToolStrip) { ((ISupportToolStripPanel)this.ParentInternal).BeginDrag(); } else { ((ISupportToolStripPanel)this.ParentInternal).EndDrag(); } } } } private ToolStripPanelRow ToolStripPanelRow { get { return (ParentInternal == null) ? null : ((ISupportToolStripPanel)ParentInternal).ToolStripPanelRow; } } protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripGripAccessibleObject(this); } public override Size GetPreferredSize(Size constrainingSize) { Size preferredSize = Size.Empty; if (this.ParentInternal != null) { if (this.ParentInternal.LayoutStyle == ToolStripLayoutStyle.VerticalStackWithOverflow) { preferredSize = new Size(this.ParentInternal.Width, gripThickness); } else { preferredSize = new Size(gripThickness, this.ParentInternal.Height); } } // Constrain ourselves if (preferredSize.Width > constrainingSize.Width) { preferredSize.Width = constrainingSize.Width; } if (preferredSize.Height > constrainingSize.Height) { preferredSize.Height = constrainingSize.Height; } return preferredSize; } private bool LeftMouseButtonIsDown() { return (Control.MouseButtons == MouseButtons.Left) && (Control.ModifierKeys == Keys.None); } protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { // all the grip painting should be on the ToolStrip itself. if (ParentInternal != null) { ParentInternal.OnPaintGrip(e); } } ////// /// /// protected override void OnMouseDown(System.Windows.Forms.MouseEventArgs mea) { startLocation = TranslatePoint(new Point(mea.X, mea.Y), ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); base.OnMouseDown(mea); } protected override void OnMouseMove(System.Windows.Forms.MouseEventArgs mea) { bool leftMouseButtonDown = LeftMouseButtonIsDown(); if (!MovingToolStrip && leftMouseButtonDown) { // determine if we've moved far enough such that the toolstrip // can be considered as moving. Point currentLocation = TranslatePoint(mea.Location, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); int deltaX = currentLocation.X - startLocation.X; deltaX = (deltaX < 0) ? deltaX *-1 : deltaX; if (DragSize == LayoutUtils.MaxSize) { DragSize = SystemInformation.DragSize; } if (deltaX >= DragSize.Width) { MovingToolStrip = true; } else { int deltaY = currentLocation.Y - startLocation.Y; deltaY = (deltaY < 0) ? deltaY *-1 : deltaY; if (deltaY >= DragSize.Height) { MovingToolStrip = true; } } } if (MovingToolStrip) { if (leftMouseButtonDown) { Point endLocation = TranslatePoint(new Point(mea.X, mea.Y), ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); // protect against calling when the mouse hasnt really moved. moving the toolstrip/creating the feedback rect // can cause extra mousemove events, we want to make sure we're not doing all this work // for nothing. if (endLocation != lastEndLocation) { ToolStripPanelRow.ToolStripPanel.MoveControl(ParentInternal, /*startLocation,*/endLocation ); lastEndLocation = endLocation; } startLocation = endLocation; } else { // sometimes we dont get mouseup in DT. Release now. MovingToolStrip = false; } } base.OnMouseMove(mea); } protected override void OnMouseEnter(System.EventArgs e) { // only switch the cursor if we've got a rafting row. if ((ParentInternal != null) && (ToolStripPanelRow != null) && (!ParentInternal.IsInDesignMode)) { oldCursor = ParentInternal.Cursor; SetCursor(ParentInternal, Cursors.SizeAll); } else { oldCursor = null; } base.OnMouseEnter(e); } ////// /// /// protected override void OnMouseLeave(System.EventArgs e) { if (oldCursor != null && !ParentInternal.IsInDesignMode) { SetCursor(ParentInternal,oldCursor); } if (!MovingToolStrip && LeftMouseButtonIsDown()) { MovingToolStrip = true; } base.OnMouseLeave(e); } protected override void OnMouseUp(System.Windows.Forms.MouseEventArgs mea) { if (MovingToolStrip) { Point endLocation = TranslatePoint(new Point(mea.X, mea.Y), ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); ToolStripPanelRow.ToolStripPanel.MoveControl(ParentInternal, /*startLocation,*/endLocation ); } if (!ParentInternal.IsInDesignMode) { SetCursor(ParentInternal, oldCursor); } ToolStripPanel.ClearDragFeedback(); MovingToolStrip = false; base.OnMouseUp(mea); } private static void SetCursor(Control control, Cursor cursor) { IntSecurity.ModifyCursor.Assert(); control.Cursor = cursor; // CodeAccessPermission.RevertAssert automatically called } internal class ToolStripGripAccessibleObject : ToolStripButtonAccessibleObject { private string stockName; public ToolStripGripAccessibleObject(ToolStripGrip owner) : base(owner){ } public override string Name { get { string name = Owner.AccessibleName; if (name != null) { return name; } if (string.IsNullOrEmpty(stockName)) { stockName = SR.GetString(SR.ToolStripGripAccessibleName); } return stockName; } set { base.Name = value; } } public override AccessibleRole Role { get { AccessibleRole role = Owner.AccessibleRole; if (role != AccessibleRole.Default) { return role; } return AccessibleRole.Grip; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.VisualStyles; using System.Windows.Forms.Layout; using System.Diagnostics; ////// internal class ToolStripGrip : ToolStripButton { private Cursor oldCursor; private int gripThickness = 0; Point startLocation = Point.Empty; private bool movingToolStrip = false; private Point lastEndLocation = ToolStrip.InvalidMouseEnter; private static Size DragSize = LayoutUtils.MaxSize; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] internal ToolStripGrip() { // if we're using XP themes we've got to be a bit thicker. gripThickness = ToolStripManager.VisualStylesEnabled ? 5 : 3; SupportsItemClick = false; } /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected internal override Padding DefaultMargin { get { return new Padding(2); } } public override bool CanSelect { get { return false; } } internal int GripThickness { get { return gripThickness; } } internal bool MovingToolStrip { get { return ((ToolStripPanelRow != null) && movingToolStrip); } set { if ((movingToolStrip != value) && ParentInternal != null) { if (value) { // dont let grips move the toolstrip if (ParentInternal.ToolStripPanelRow == null) { return; } } movingToolStrip = value; lastEndLocation = ToolStrip.InvalidMouseEnter; if (movingToolStrip) { ((ISupportToolStripPanel)this.ParentInternal).BeginDrag(); } else { ((ISupportToolStripPanel)this.ParentInternal).EndDrag(); } } } } private ToolStripPanelRow ToolStripPanelRow { get { return (ParentInternal == null) ? null : ((ISupportToolStripPanel)ParentInternal).ToolStripPanelRow; } } protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripGripAccessibleObject(this); } public override Size GetPreferredSize(Size constrainingSize) { Size preferredSize = Size.Empty; if (this.ParentInternal != null) { if (this.ParentInternal.LayoutStyle == ToolStripLayoutStyle.VerticalStackWithOverflow) { preferredSize = new Size(this.ParentInternal.Width, gripThickness); } else { preferredSize = new Size(gripThickness, this.ParentInternal.Height); } } // Constrain ourselves if (preferredSize.Width > constrainingSize.Width) { preferredSize.Width = constrainingSize.Width; } if (preferredSize.Height > constrainingSize.Height) { preferredSize.Height = constrainingSize.Height; } return preferredSize; } private bool LeftMouseButtonIsDown() { return (Control.MouseButtons == MouseButtons.Left) && (Control.ModifierKeys == Keys.None); } protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { // all the grip painting should be on the ToolStrip itself. if (ParentInternal != null) { ParentInternal.OnPaintGrip(e); } } ////// /// /// protected override void OnMouseDown(System.Windows.Forms.MouseEventArgs mea) { startLocation = TranslatePoint(new Point(mea.X, mea.Y), ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); base.OnMouseDown(mea); } protected override void OnMouseMove(System.Windows.Forms.MouseEventArgs mea) { bool leftMouseButtonDown = LeftMouseButtonIsDown(); if (!MovingToolStrip && leftMouseButtonDown) { // determine if we've moved far enough such that the toolstrip // can be considered as moving. Point currentLocation = TranslatePoint(mea.Location, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); int deltaX = currentLocation.X - startLocation.X; deltaX = (deltaX < 0) ? deltaX *-1 : deltaX; if (DragSize == LayoutUtils.MaxSize) { DragSize = SystemInformation.DragSize; } if (deltaX >= DragSize.Width) { MovingToolStrip = true; } else { int deltaY = currentLocation.Y - startLocation.Y; deltaY = (deltaY < 0) ? deltaY *-1 : deltaY; if (deltaY >= DragSize.Height) { MovingToolStrip = true; } } } if (MovingToolStrip) { if (leftMouseButtonDown) { Point endLocation = TranslatePoint(new Point(mea.X, mea.Y), ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); // protect against calling when the mouse hasnt really moved. moving the toolstrip/creating the feedback rect // can cause extra mousemove events, we want to make sure we're not doing all this work // for nothing. if (endLocation != lastEndLocation) { ToolStripPanelRow.ToolStripPanel.MoveControl(ParentInternal, /*startLocation,*/endLocation ); lastEndLocation = endLocation; } startLocation = endLocation; } else { // sometimes we dont get mouseup in DT. Release now. MovingToolStrip = false; } } base.OnMouseMove(mea); } protected override void OnMouseEnter(System.EventArgs e) { // only switch the cursor if we've got a rafting row. if ((ParentInternal != null) && (ToolStripPanelRow != null) && (!ParentInternal.IsInDesignMode)) { oldCursor = ParentInternal.Cursor; SetCursor(ParentInternal, Cursors.SizeAll); } else { oldCursor = null; } base.OnMouseEnter(e); } ////// /// /// protected override void OnMouseLeave(System.EventArgs e) { if (oldCursor != null && !ParentInternal.IsInDesignMode) { SetCursor(ParentInternal,oldCursor); } if (!MovingToolStrip && LeftMouseButtonIsDown()) { MovingToolStrip = true; } base.OnMouseLeave(e); } protected override void OnMouseUp(System.Windows.Forms.MouseEventArgs mea) { if (MovingToolStrip) { Point endLocation = TranslatePoint(new Point(mea.X, mea.Y), ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords); ToolStripPanelRow.ToolStripPanel.MoveControl(ParentInternal, /*startLocation,*/endLocation ); } if (!ParentInternal.IsInDesignMode) { SetCursor(ParentInternal, oldCursor); } ToolStripPanel.ClearDragFeedback(); MovingToolStrip = false; base.OnMouseUp(mea); } private static void SetCursor(Control control, Cursor cursor) { IntSecurity.ModifyCursor.Assert(); control.Cursor = cursor; // CodeAccessPermission.RevertAssert automatically called } internal class ToolStripGripAccessibleObject : ToolStripButtonAccessibleObject { private string stockName; public ToolStripGripAccessibleObject(ToolStripGrip owner) : base(owner){ } public override string Name { get { string name = Owner.AccessibleName; if (name != null) { return name; } if (string.IsNullOrEmpty(stockName)) { stockName = SR.GetString(SR.ToolStripGripAccessibleName); } return stockName; } set { base.Name = value; } } public override AccessibleRole Role { get { AccessibleRole role = Owner.AccessibleRole; if (role != AccessibleRole.Default) { return role; } return AccessibleRole.Grip; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
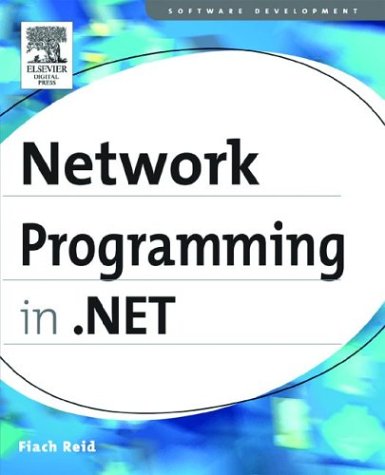
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataRowComparer.cs
- MulticastIPAddressInformationCollection.cs
- Point3DCollection.cs
- RecognizerStateChangedEventArgs.cs
- TypeForwardedFromAttribute.cs
- X509CertificateChain.cs
- ToolStripButton.cs
- DeclarativeCatalogPartDesigner.cs
- SqlDataSourceParameterParser.cs
- ImageClickEventArgs.cs
- CodeTypeConstructor.cs
- Schedule.cs
- _MultipleConnectAsync.cs
- RenamedEventArgs.cs
- ControlAdapter.cs
- ResourceDictionaryCollection.cs
- ECDiffieHellmanCng.cs
- DataObjectPastingEventArgs.cs
- InputScopeManager.cs
- DataError.cs
- MaterialCollection.cs
- CallTemplateAction.cs
- diagnosticsswitches.cs
- HtmlSelect.cs
- ClassHandlersStore.cs
- CodeAssignStatement.cs
- COAUTHIDENTITY.cs
- X509Certificate2Collection.cs
- TextParaClient.cs
- AssociationEndMember.cs
- TextElementAutomationPeer.cs
- OuterGlowBitmapEffect.cs
- Flowchart.cs
- WorkflowOwnerAsyncResult.cs
- ValueQuery.cs
- DesignerInterfaces.cs
- WindowsTooltip.cs
- Transform.cs
- QueryOperationResponseOfT.cs
- CodeCompiler.cs
- ConfigXmlAttribute.cs
- Focus.cs
- Environment.cs
- Literal.cs
- FormViewPagerRow.cs
- EventLogPermission.cs
- TemplatingOptionsDialog.cs
- ServiceTimeoutsBehavior.cs
- Track.cs
- XmlElementAttributes.cs
- EntityFunctions.cs
- PopupEventArgs.cs
- HttpAsyncResult.cs
- DataGridCellInfo.cs
- NavigationProperty.cs
- XmlSortKey.cs
- DataGridCellsPresenter.cs
- DiagnosticsConfigurationHandler.cs
- counter.cs
- WebPartConnectionsCancelVerb.cs
- DataGridViewColumnHeaderCell.cs
- columnmapfactory.cs
- MarkupExtensionSerializer.cs
- MethodCallTranslator.cs
- HostingEnvironment.cs
- ContainerUIElement3D.cs
- EditorZoneBase.cs
- DBParameter.cs
- LoadMessageLogger.cs
- WebBrowser.cs
- MdiWindowListStrip.cs
- TopClause.cs
- lengthconverter.cs
- Visual3D.cs
- PassportIdentity.cs
- SecurityCriticalDataForSet.cs
- SHA512Cng.cs
- Function.cs
- TreeNodeStyle.cs
- Transform.cs
- SocketElement.cs
- RequestCachePolicy.cs
- TransactionScopeDesigner.cs
- LoginCancelEventArgs.cs
- PersonalizationStateQuery.cs
- Html32TextWriter.cs
- RelatedView.cs
- ConnectionProviderAttribute.cs
- COMException.cs
- DataGridViewCellParsingEventArgs.cs
- ServiceNotStartedException.cs
- DataSysAttribute.cs
- TypeCodeDomSerializer.cs
- SafeMemoryMappedViewHandle.cs
- PolyLineSegment.cs
- UInt16Storage.cs
- DbModificationCommandTree.cs
- TextAutomationPeer.cs
- SmtpDigestAuthenticationModule.cs
- ZoneLinkButton.cs