Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Text / TypographyProperties.cs / 1 / TypographyProperties.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TypographyProperties.cs // // Description: Typography properties. // // History: // 06/13/2003 : sergeym - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.Text { ////// Typography properties provider. /// internal sealed class TypographyProperties : TextRunTypographyProperties { ////// Used as indexes to bitscale for boolean properties /// private enum PropertyId { ///StandardLigatures property StandardLigatures = 0, ///ContextualLigatures property ContextualLigatures = 1, ///DiscretionaryLigatures property DiscretionaryLigatures = 2, ///HistoricalLigatures property HistoricalLigatures = 3, ///CaseSensitiveForms property CaseSensitiveForms = 4, ///ContextualAlternates property ContextualAlternates = 5, ///HistoricalForms property HistoricalForms = 6, ///Kerning property Kerning = 7, ///CapitalSpacing property CapitalSpacing = 8, ///StylisticSet1 property StylisticSet1 = 9, ///StylisticSet2 property StylisticSet2 = 10, ///StylisticSet3 property StylisticSet3 = 11, ///StylisticSet4 property StylisticSet4 = 12, ///StylisticSet5 property StylisticSet5 = 13, ///StylisticSet6 property StylisticSet6 = 14, ///StylisticSet7 property StylisticSet7 = 15, ///StylisticSet8 property StylisticSet8 = 16, ///StylisticSet9 property StylisticSet9 = 17, ///StylisticSet10 property StylisticSet10 = 18, ///StylisticSet11 property StylisticSet11 = 19, ///StylisticSet12 property StylisticSet12 = 20, ///StylisticSet13 property StylisticSet13 = 21, ///StylisticSet14 property StylisticSet14 = 22, ///StylisticSet15 property StylisticSet15 = 23, ///StylisticSet16 property StylisticSet16 = 24, ///StylisticSet17 property StylisticSet17 = 25, ///StylisticSet18 property StylisticSet18 = 26, ///StylisticSet19 property StylisticSet19 = 27, ///StylisticSet20 property StylisticSet20 = 28, ///SlashedZero property SlashedZero = 29, ///MathematicalGreek property MathematicalGreek = 30, ///EastAsianExpertForms property EastAsianExpertForms = 31, ////// Total number of properties. Should not be >32. /// Otherwise bitmask field _idPropertySetFlags should be changed to ulong /// PropertyCount = 32 } ////// Create new typographyProperties with deefault values /// public TypographyProperties() { // Flags are stored in uint (32 bits). // Any way to check it at compile time? Debug.Assert((uint)PropertyId.PropertyCount <= 32); ResetProperties(); } #region Public typography properties ////// /// public override bool StandardLigatures { get { return IsBooleanPropertySet(PropertyId.StandardLigatures); } } public void SetStandardLigatures(bool value) { SetBooleanProperty(PropertyId.StandardLigatures, value); } ////// /// public override bool ContextualLigatures { get { return IsBooleanPropertySet(PropertyId.ContextualLigatures); } } public void SetContextualLigatures(bool value) { SetBooleanProperty(PropertyId.ContextualLigatures, value); } ////// /// public override bool DiscretionaryLigatures { get { return IsBooleanPropertySet(PropertyId.DiscretionaryLigatures); } } public void SetDiscretionaryLigatures(bool value) { SetBooleanProperty(PropertyId.DiscretionaryLigatures, value); } ////// /// public override bool HistoricalLigatures { get { return IsBooleanPropertySet(PropertyId.HistoricalLigatures); } } public void SetHistoricalLigatures(bool value) { SetBooleanProperty(PropertyId.HistoricalLigatures, value); } ////// /// public override bool CaseSensitiveForms { get { return IsBooleanPropertySet(PropertyId.CaseSensitiveForms); } } public void SetCaseSensitiveForms(bool value) { SetBooleanProperty(PropertyId.CaseSensitiveForms, value); } ////// /// public override bool ContextualAlternates { get { return IsBooleanPropertySet(PropertyId.ContextualAlternates); } } public void SetContextualAlternates(bool value) { SetBooleanProperty(PropertyId.ContextualAlternates, value); } ////// /// public override bool HistoricalForms { get { return IsBooleanPropertySet(PropertyId.HistoricalForms); } } public void SetHistoricalForms(bool value) { SetBooleanProperty(PropertyId.HistoricalForms, value); } ////// /// public override bool Kerning { get { return IsBooleanPropertySet(PropertyId.Kerning); } } public void SetKerning(bool value) { SetBooleanProperty(PropertyId.Kerning, value); } ////// /// public override bool CapitalSpacing { get { return IsBooleanPropertySet(PropertyId.CapitalSpacing); } } public void SetCapitalSpacing(bool value) { SetBooleanProperty(PropertyId.CapitalSpacing, value); } ////// /// public override bool StylisticSet1 { get { return IsBooleanPropertySet(PropertyId.StylisticSet1); } } public void SetStylisticSet1(bool value) { SetBooleanProperty(PropertyId.StylisticSet1, value); } ////// /// public override bool StylisticSet2 { get { return IsBooleanPropertySet(PropertyId.StylisticSet2); } } public void SetStylisticSet2(bool value) { SetBooleanProperty(PropertyId.StylisticSet2, value); } ////// /// public override bool StylisticSet3 { get { return IsBooleanPropertySet(PropertyId.StylisticSet3); } } public void SetStylisticSet3(bool value) { SetBooleanProperty(PropertyId.StylisticSet3, value); } ////// /// public override bool StylisticSet4 { get { return IsBooleanPropertySet(PropertyId.StylisticSet4); } } public void SetStylisticSet4(bool value) { SetBooleanProperty(PropertyId.StylisticSet4, value); } ////// /// public override bool StylisticSet5 { get { return IsBooleanPropertySet(PropertyId.StylisticSet5); } } public void SetStylisticSet5(bool value) { SetBooleanProperty(PropertyId.StylisticSet5, value); } ////// /// public override bool StylisticSet6 { get { return IsBooleanPropertySet(PropertyId.StylisticSet6); } } public void SetStylisticSet6(bool value) { SetBooleanProperty(PropertyId.StylisticSet6, value); } ////// /// public override bool StylisticSet7 { get { return IsBooleanPropertySet(PropertyId.StylisticSet7); } } public void SetStylisticSet7(bool value) { SetBooleanProperty(PropertyId.StylisticSet7, value); } ////// /// public override bool StylisticSet8 { get { return IsBooleanPropertySet(PropertyId.StylisticSet8); } } public void SetStylisticSet8(bool value) { SetBooleanProperty(PropertyId.StylisticSet8, value); } ////// /// public override bool StylisticSet9 { get { return IsBooleanPropertySet(PropertyId.StylisticSet9); } } public void SetStylisticSet9(bool value) { SetBooleanProperty(PropertyId.StylisticSet9, value); } ////// /// public override bool StylisticSet10 { get { return IsBooleanPropertySet(PropertyId.StylisticSet10); } } public void SetStylisticSet10(bool value) { SetBooleanProperty(PropertyId.StylisticSet10, value); } ////// /// public override bool StylisticSet11 { get { return IsBooleanPropertySet(PropertyId.StylisticSet11); } } public void SetStylisticSet11(bool value) { SetBooleanProperty(PropertyId.StylisticSet11, value); } ////// /// public override bool StylisticSet12 { get { return IsBooleanPropertySet(PropertyId.StylisticSet12); } } public void SetStylisticSet12(bool value) { SetBooleanProperty(PropertyId.StylisticSet12, value); } ////// /// public override bool StylisticSet13 { get { return IsBooleanPropertySet(PropertyId.StylisticSet13); } } public void SetStylisticSet13(bool value) { SetBooleanProperty(PropertyId.StylisticSet13, value); } ////// /// public override bool StylisticSet14 { get { return IsBooleanPropertySet(PropertyId.StylisticSet14); } } public void SetStylisticSet14(bool value) { SetBooleanProperty(PropertyId.StylisticSet14, value); } ////// /// public override bool StylisticSet15 { get { return IsBooleanPropertySet(PropertyId.StylisticSet15); } } public void SetStylisticSet15(bool value) { SetBooleanProperty(PropertyId.StylisticSet15, value); } ////// /// public override bool StylisticSet16 { get { return IsBooleanPropertySet(PropertyId.StylisticSet16); } } public void SetStylisticSet16(bool value) { SetBooleanProperty(PropertyId.StylisticSet16, value); } ////// /// public override bool StylisticSet17 { get { return IsBooleanPropertySet(PropertyId.StylisticSet17); } } public void SetStylisticSet17(bool value) { SetBooleanProperty(PropertyId.StylisticSet17, value); } ////// /// public override bool StylisticSet18 { get { return IsBooleanPropertySet(PropertyId.StylisticSet18); } } public void SetStylisticSet18(bool value) { SetBooleanProperty(PropertyId.StylisticSet18, value); } ////// /// public override bool StylisticSet19 { get { return IsBooleanPropertySet(PropertyId.StylisticSet19); } } public void SetStylisticSet19(bool value) { SetBooleanProperty(PropertyId.StylisticSet19, value); } ////// /// public override bool StylisticSet20 { get { return IsBooleanPropertySet(PropertyId.StylisticSet20); } } public void SetStylisticSet20(bool value) { SetBooleanProperty(PropertyId.StylisticSet20, value); } ////// /// public override FontFraction Fraction { get { return _fraction; } } public void SetFraction(FontFraction value) { _fraction = value; OnPropertiesChanged(); } ////// /// public override bool SlashedZero { get { return IsBooleanPropertySet(PropertyId.SlashedZero); } } public void SetSlashedZero(bool value) { SetBooleanProperty(PropertyId.SlashedZero, value); } ////// /// public override bool MathematicalGreek { get { return IsBooleanPropertySet(PropertyId.MathematicalGreek); } } public void SetMathematicalGreek(bool value) { SetBooleanProperty(PropertyId.MathematicalGreek, value); } ////// /// public override bool EastAsianExpertForms { get { return IsBooleanPropertySet(PropertyId.EastAsianExpertForms); } } public void SetEastAsianExpertForms(bool value) { SetBooleanProperty(PropertyId.EastAsianExpertForms, value); } ////// /// public override FontVariants Variants { get { return _variant; } } public void SetVariants(FontVariants value) { _variant = value; OnPropertiesChanged(); } ////// /// public override FontCapitals ----s { get { return _capitals; } } public void Set----s(FontCapitals value) { _capitals = value; OnPropertiesChanged(); } ////// /// public override FontNumeralStyle NumeralStyle { get { return _numeralStyle; } } public void SetNumeralStyle(FontNumeralStyle value) { _numeralStyle = value; OnPropertiesChanged(); } ////// /// public override FontNumeralAlignment NumeralAlignment { get { return _numeralAlignment; } } public void SetNumeralAlignment(FontNumeralAlignment value) { _numeralAlignment = value; OnPropertiesChanged(); } ////// /// public override FontEastAsianWidths EastAsianWidths { get { return _eastAsianWidths; } } public void SetEastAsianWidths(FontEastAsianWidths value) { _eastAsianWidths = value; OnPropertiesChanged(); } ////// /// public override FontEastAsianLanguage EastAsianLanguage { get { return _eastAsianLanguage; } } public void SetEastAsianLanguage(FontEastAsianLanguage value) { _eastAsianLanguage = value; OnPropertiesChanged(); } ////// /// public override int StandardSwashes { get { return _standardSwashes; } } public void SetStandardSwashes(int value) { _standardSwashes = value; OnPropertiesChanged(); } ////// /// public override int ContextualSwashes { get { return _contextualSwashes; } } public void SetContextualSwashes(int value) { _contextualSwashes = value; OnPropertiesChanged(); } ////// /// public override int StylisticAlternates { get { return _stylisticAlternates; } } public void SetStylisticAlternates(int value) { _stylisticAlternates = value; OnPropertiesChanged(); } ////// /// public override int AnnotationAlternates { get { return _annotationAlternates; } } public void SetAnnotationAlternates(int value) { _annotationAlternates = value; OnPropertiesChanged(); } #endregion Public typography properties ////// Check whether two Property sets are equal /// /// property to compare ///public override bool Equals(object other) { if (other == null) { return false; } if (this.GetType() != other.GetType()) { return false; } TypographyProperties genericOther = (TypographyProperties)other; return //This will cover all boolean properties _idPropertySetFlags == genericOther._idPropertySetFlags && //And this will cover the rest _variant == genericOther._variant && _capitals == genericOther._capitals && _fraction == genericOther._fraction && _numeralStyle == genericOther._numeralStyle && _numeralAlignment == genericOther._numeralAlignment && _eastAsianWidths == genericOther._eastAsianWidths && _eastAsianLanguage == genericOther._eastAsianLanguage && _standardSwashes == genericOther._standardSwashes && _contextualSwashes == genericOther._contextualSwashes && _stylisticAlternates == genericOther._stylisticAlternates && _annotationAlternates == genericOther._annotationAlternates; } public override int GetHashCode() { return (int)(_idPropertySetFlags >> 32) ^ (int)(_idPropertySetFlags & 0xFFFFFFFF) ^ (int)_variant << 28 ^ (int)_capitals << 24 ^ (int)_numeralStyle << 20 ^ (int)_numeralAlignment << 18 ^ (int)_eastAsianWidths << 14 ^ (int)_eastAsianLanguage << 10 ^ (int)_standardSwashes << 6 ^ (int)_contextualSwashes << 2 ^ (int)_stylisticAlternates ^ (int)_fraction << 16 ^ (int)_annotationAlternates << 12; } public static bool operator ==(TypographyProperties first, TypographyProperties second) { //Need to cast to object to do null comparision. if (((object)first) == null) return (((object)second) == null); return first.Equals(second); } public static bool operator !=(TypographyProperties first, TypographyProperties second) { return !(first == second); } #region Private methods /// /// Set all properties to default value /// private void ResetProperties() { //clean flags _idPropertySetFlags = 0; //assign non-trivial(not bolean) values _standardSwashes = 0; _contextualSwashes = 0; _stylisticAlternates = 0; _annotationAlternates = 0; _variant = FontVariants.Normal; _capitals = FontCapitals.Normal; _numeralStyle = FontNumeralStyle.Normal; _numeralAlignment = FontNumeralAlignment.Normal; _eastAsianWidths = FontEastAsianWidths.Normal; _eastAsianLanguage = FontEastAsianLanguage.Normal; _fraction = FontFraction.Normal; OnPropertiesChanged(); } ////// Check whether boolean property is set to non-default value /// /// PropertyId ///private bool IsBooleanPropertySet(PropertyId propertyId) { Debug.Assert((uint)propertyId < (uint)PropertyId.PropertyCount, "Invalid typography property id"); uint flagMask = (uint)(((uint)1) << ((int)propertyId)); return (_idPropertySetFlags & flagMask) != 0; } /// /// Set/clean flag that property value is non-default /// Used only internally to support quick checks while forming FeatureSet /// /// /// Property id /// Value of the flag private void SetBooleanProperty(PropertyId propertyId, bool flagValue) { Debug.Assert((uint)propertyId < (uint)PropertyId.PropertyCount, "Invalid typography property id"); uint flagMask = (uint)(((uint)1) << ((int)propertyId)); if (flagValue) _idPropertySetFlags |= flagMask; else _idPropertySetFlags &= ~flagMask; OnPropertiesChanged(); } private uint _idPropertySetFlags; private int _standardSwashes; private int _contextualSwashes; private int _stylisticAlternates; private int _annotationAlternates; private FontVariants _variant; private FontCapitals _capitals; private FontFraction _fraction; private FontNumeralStyle _numeralStyle; private FontNumeralAlignment _numeralAlignment; private FontEastAsianWidths _eastAsianWidths; private FontEastAsianLanguage _eastAsianLanguage; #endregion Private members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TypographyProperties.cs // // Description: Typography properties. // // History: // 06/13/2003 : sergeym - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.Text { ////// Typography properties provider. /// internal sealed class TypographyProperties : TextRunTypographyProperties { ////// Used as indexes to bitscale for boolean properties /// private enum PropertyId { ///StandardLigatures property StandardLigatures = 0, ///ContextualLigatures property ContextualLigatures = 1, ///DiscretionaryLigatures property DiscretionaryLigatures = 2, ///HistoricalLigatures property HistoricalLigatures = 3, ///CaseSensitiveForms property CaseSensitiveForms = 4, ///ContextualAlternates property ContextualAlternates = 5, ///HistoricalForms property HistoricalForms = 6, ///Kerning property Kerning = 7, ///CapitalSpacing property CapitalSpacing = 8, ///StylisticSet1 property StylisticSet1 = 9, ///StylisticSet2 property StylisticSet2 = 10, ///StylisticSet3 property StylisticSet3 = 11, ///StylisticSet4 property StylisticSet4 = 12, ///StylisticSet5 property StylisticSet5 = 13, ///StylisticSet6 property StylisticSet6 = 14, ///StylisticSet7 property StylisticSet7 = 15, ///StylisticSet8 property StylisticSet8 = 16, ///StylisticSet9 property StylisticSet9 = 17, ///StylisticSet10 property StylisticSet10 = 18, ///StylisticSet11 property StylisticSet11 = 19, ///StylisticSet12 property StylisticSet12 = 20, ///StylisticSet13 property StylisticSet13 = 21, ///StylisticSet14 property StylisticSet14 = 22, ///StylisticSet15 property StylisticSet15 = 23, ///StylisticSet16 property StylisticSet16 = 24, ///StylisticSet17 property StylisticSet17 = 25, ///StylisticSet18 property StylisticSet18 = 26, ///StylisticSet19 property StylisticSet19 = 27, ///StylisticSet20 property StylisticSet20 = 28, ///SlashedZero property SlashedZero = 29, ///MathematicalGreek property MathematicalGreek = 30, ///EastAsianExpertForms property EastAsianExpertForms = 31, ////// Total number of properties. Should not be >32. /// Otherwise bitmask field _idPropertySetFlags should be changed to ulong /// PropertyCount = 32 } ////// Create new typographyProperties with deefault values /// public TypographyProperties() { // Flags are stored in uint (32 bits). // Any way to check it at compile time? Debug.Assert((uint)PropertyId.PropertyCount <= 32); ResetProperties(); } #region Public typography properties ////// /// public override bool StandardLigatures { get { return IsBooleanPropertySet(PropertyId.StandardLigatures); } } public void SetStandardLigatures(bool value) { SetBooleanProperty(PropertyId.StandardLigatures, value); } ////// /// public override bool ContextualLigatures { get { return IsBooleanPropertySet(PropertyId.ContextualLigatures); } } public void SetContextualLigatures(bool value) { SetBooleanProperty(PropertyId.ContextualLigatures, value); } ////// /// public override bool DiscretionaryLigatures { get { return IsBooleanPropertySet(PropertyId.DiscretionaryLigatures); } } public void SetDiscretionaryLigatures(bool value) { SetBooleanProperty(PropertyId.DiscretionaryLigatures, value); } ////// /// public override bool HistoricalLigatures { get { return IsBooleanPropertySet(PropertyId.HistoricalLigatures); } } public void SetHistoricalLigatures(bool value) { SetBooleanProperty(PropertyId.HistoricalLigatures, value); } ////// /// public override bool CaseSensitiveForms { get { return IsBooleanPropertySet(PropertyId.CaseSensitiveForms); } } public void SetCaseSensitiveForms(bool value) { SetBooleanProperty(PropertyId.CaseSensitiveForms, value); } ////// /// public override bool ContextualAlternates { get { return IsBooleanPropertySet(PropertyId.ContextualAlternates); } } public void SetContextualAlternates(bool value) { SetBooleanProperty(PropertyId.ContextualAlternates, value); } ////// /// public override bool HistoricalForms { get { return IsBooleanPropertySet(PropertyId.HistoricalForms); } } public void SetHistoricalForms(bool value) { SetBooleanProperty(PropertyId.HistoricalForms, value); } ////// /// public override bool Kerning { get { return IsBooleanPropertySet(PropertyId.Kerning); } } public void SetKerning(bool value) { SetBooleanProperty(PropertyId.Kerning, value); } ////// /// public override bool CapitalSpacing { get { return IsBooleanPropertySet(PropertyId.CapitalSpacing); } } public void SetCapitalSpacing(bool value) { SetBooleanProperty(PropertyId.CapitalSpacing, value); } ////// /// public override bool StylisticSet1 { get { return IsBooleanPropertySet(PropertyId.StylisticSet1); } } public void SetStylisticSet1(bool value) { SetBooleanProperty(PropertyId.StylisticSet1, value); } ////// /// public override bool StylisticSet2 { get { return IsBooleanPropertySet(PropertyId.StylisticSet2); } } public void SetStylisticSet2(bool value) { SetBooleanProperty(PropertyId.StylisticSet2, value); } ////// /// public override bool StylisticSet3 { get { return IsBooleanPropertySet(PropertyId.StylisticSet3); } } public void SetStylisticSet3(bool value) { SetBooleanProperty(PropertyId.StylisticSet3, value); } ////// /// public override bool StylisticSet4 { get { return IsBooleanPropertySet(PropertyId.StylisticSet4); } } public void SetStylisticSet4(bool value) { SetBooleanProperty(PropertyId.StylisticSet4, value); } ////// /// public override bool StylisticSet5 { get { return IsBooleanPropertySet(PropertyId.StylisticSet5); } } public void SetStylisticSet5(bool value) { SetBooleanProperty(PropertyId.StylisticSet5, value); } ////// /// public override bool StylisticSet6 { get { return IsBooleanPropertySet(PropertyId.StylisticSet6); } } public void SetStylisticSet6(bool value) { SetBooleanProperty(PropertyId.StylisticSet6, value); } ////// /// public override bool StylisticSet7 { get { return IsBooleanPropertySet(PropertyId.StylisticSet7); } } public void SetStylisticSet7(bool value) { SetBooleanProperty(PropertyId.StylisticSet7, value); } ////// /// public override bool StylisticSet8 { get { return IsBooleanPropertySet(PropertyId.StylisticSet8); } } public void SetStylisticSet8(bool value) { SetBooleanProperty(PropertyId.StylisticSet8, value); } ////// /// public override bool StylisticSet9 { get { return IsBooleanPropertySet(PropertyId.StylisticSet9); } } public void SetStylisticSet9(bool value) { SetBooleanProperty(PropertyId.StylisticSet9, value); } ////// /// public override bool StylisticSet10 { get { return IsBooleanPropertySet(PropertyId.StylisticSet10); } } public void SetStylisticSet10(bool value) { SetBooleanProperty(PropertyId.StylisticSet10, value); } ////// /// public override bool StylisticSet11 { get { return IsBooleanPropertySet(PropertyId.StylisticSet11); } } public void SetStylisticSet11(bool value) { SetBooleanProperty(PropertyId.StylisticSet11, value); } ////// /// public override bool StylisticSet12 { get { return IsBooleanPropertySet(PropertyId.StylisticSet12); } } public void SetStylisticSet12(bool value) { SetBooleanProperty(PropertyId.StylisticSet12, value); } ////// /// public override bool StylisticSet13 { get { return IsBooleanPropertySet(PropertyId.StylisticSet13); } } public void SetStylisticSet13(bool value) { SetBooleanProperty(PropertyId.StylisticSet13, value); } ////// /// public override bool StylisticSet14 { get { return IsBooleanPropertySet(PropertyId.StylisticSet14); } } public void SetStylisticSet14(bool value) { SetBooleanProperty(PropertyId.StylisticSet14, value); } ////// /// public override bool StylisticSet15 { get { return IsBooleanPropertySet(PropertyId.StylisticSet15); } } public void SetStylisticSet15(bool value) { SetBooleanProperty(PropertyId.StylisticSet15, value); } ////// /// public override bool StylisticSet16 { get { return IsBooleanPropertySet(PropertyId.StylisticSet16); } } public void SetStylisticSet16(bool value) { SetBooleanProperty(PropertyId.StylisticSet16, value); } ////// /// public override bool StylisticSet17 { get { return IsBooleanPropertySet(PropertyId.StylisticSet17); } } public void SetStylisticSet17(bool value) { SetBooleanProperty(PropertyId.StylisticSet17, value); } ////// /// public override bool StylisticSet18 { get { return IsBooleanPropertySet(PropertyId.StylisticSet18); } } public void SetStylisticSet18(bool value) { SetBooleanProperty(PropertyId.StylisticSet18, value); } ////// /// public override bool StylisticSet19 { get { return IsBooleanPropertySet(PropertyId.StylisticSet19); } } public void SetStylisticSet19(bool value) { SetBooleanProperty(PropertyId.StylisticSet19, value); } ////// /// public override bool StylisticSet20 { get { return IsBooleanPropertySet(PropertyId.StylisticSet20); } } public void SetStylisticSet20(bool value) { SetBooleanProperty(PropertyId.StylisticSet20, value); } ////// /// public override FontFraction Fraction { get { return _fraction; } } public void SetFraction(FontFraction value) { _fraction = value; OnPropertiesChanged(); } ////// /// public override bool SlashedZero { get { return IsBooleanPropertySet(PropertyId.SlashedZero); } } public void SetSlashedZero(bool value) { SetBooleanProperty(PropertyId.SlashedZero, value); } ////// /// public override bool MathematicalGreek { get { return IsBooleanPropertySet(PropertyId.MathematicalGreek); } } public void SetMathematicalGreek(bool value) { SetBooleanProperty(PropertyId.MathematicalGreek, value); } ////// /// public override bool EastAsianExpertForms { get { return IsBooleanPropertySet(PropertyId.EastAsianExpertForms); } } public void SetEastAsianExpertForms(bool value) { SetBooleanProperty(PropertyId.EastAsianExpertForms, value); } ////// /// public override FontVariants Variants { get { return _variant; } } public void SetVariants(FontVariants value) { _variant = value; OnPropertiesChanged(); } ////// /// public override FontCapitals ----s { get { return _capitals; } } public void Set----s(FontCapitals value) { _capitals = value; OnPropertiesChanged(); } ////// /// public override FontNumeralStyle NumeralStyle { get { return _numeralStyle; } } public void SetNumeralStyle(FontNumeralStyle value) { _numeralStyle = value; OnPropertiesChanged(); } ////// /// public override FontNumeralAlignment NumeralAlignment { get { return _numeralAlignment; } } public void SetNumeralAlignment(FontNumeralAlignment value) { _numeralAlignment = value; OnPropertiesChanged(); } ////// /// public override FontEastAsianWidths EastAsianWidths { get { return _eastAsianWidths; } } public void SetEastAsianWidths(FontEastAsianWidths value) { _eastAsianWidths = value; OnPropertiesChanged(); } ////// /// public override FontEastAsianLanguage EastAsianLanguage { get { return _eastAsianLanguage; } } public void SetEastAsianLanguage(FontEastAsianLanguage value) { _eastAsianLanguage = value; OnPropertiesChanged(); } ////// /// public override int StandardSwashes { get { return _standardSwashes; } } public void SetStandardSwashes(int value) { _standardSwashes = value; OnPropertiesChanged(); } ////// /// public override int ContextualSwashes { get { return _contextualSwashes; } } public void SetContextualSwashes(int value) { _contextualSwashes = value; OnPropertiesChanged(); } ////// /// public override int StylisticAlternates { get { return _stylisticAlternates; } } public void SetStylisticAlternates(int value) { _stylisticAlternates = value; OnPropertiesChanged(); } ////// /// public override int AnnotationAlternates { get { return _annotationAlternates; } } public void SetAnnotationAlternates(int value) { _annotationAlternates = value; OnPropertiesChanged(); } #endregion Public typography properties ////// Check whether two Property sets are equal /// /// property to compare ///public override bool Equals(object other) { if (other == null) { return false; } if (this.GetType() != other.GetType()) { return false; } TypographyProperties genericOther = (TypographyProperties)other; return //This will cover all boolean properties _idPropertySetFlags == genericOther._idPropertySetFlags && //And this will cover the rest _variant == genericOther._variant && _capitals == genericOther._capitals && _fraction == genericOther._fraction && _numeralStyle == genericOther._numeralStyle && _numeralAlignment == genericOther._numeralAlignment && _eastAsianWidths == genericOther._eastAsianWidths && _eastAsianLanguage == genericOther._eastAsianLanguage && _standardSwashes == genericOther._standardSwashes && _contextualSwashes == genericOther._contextualSwashes && _stylisticAlternates == genericOther._stylisticAlternates && _annotationAlternates == genericOther._annotationAlternates; } public override int GetHashCode() { return (int)(_idPropertySetFlags >> 32) ^ (int)(_idPropertySetFlags & 0xFFFFFFFF) ^ (int)_variant << 28 ^ (int)_capitals << 24 ^ (int)_numeralStyle << 20 ^ (int)_numeralAlignment << 18 ^ (int)_eastAsianWidths << 14 ^ (int)_eastAsianLanguage << 10 ^ (int)_standardSwashes << 6 ^ (int)_contextualSwashes << 2 ^ (int)_stylisticAlternates ^ (int)_fraction << 16 ^ (int)_annotationAlternates << 12; } public static bool operator ==(TypographyProperties first, TypographyProperties second) { //Need to cast to object to do null comparision. if (((object)first) == null) return (((object)second) == null); return first.Equals(second); } public static bool operator !=(TypographyProperties first, TypographyProperties second) { return !(first == second); } #region Private methods /// /// Set all properties to default value /// private void ResetProperties() { //clean flags _idPropertySetFlags = 0; //assign non-trivial(not bolean) values _standardSwashes = 0; _contextualSwashes = 0; _stylisticAlternates = 0; _annotationAlternates = 0; _variant = FontVariants.Normal; _capitals = FontCapitals.Normal; _numeralStyle = FontNumeralStyle.Normal; _numeralAlignment = FontNumeralAlignment.Normal; _eastAsianWidths = FontEastAsianWidths.Normal; _eastAsianLanguage = FontEastAsianLanguage.Normal; _fraction = FontFraction.Normal; OnPropertiesChanged(); } ////// Check whether boolean property is set to non-default value /// /// PropertyId ///private bool IsBooleanPropertySet(PropertyId propertyId) { Debug.Assert((uint)propertyId < (uint)PropertyId.PropertyCount, "Invalid typography property id"); uint flagMask = (uint)(((uint)1) << ((int)propertyId)); return (_idPropertySetFlags & flagMask) != 0; } /// /// Set/clean flag that property value is non-default /// Used only internally to support quick checks while forming FeatureSet /// /// /// Property id /// Value of the flag private void SetBooleanProperty(PropertyId propertyId, bool flagValue) { Debug.Assert((uint)propertyId < (uint)PropertyId.PropertyCount, "Invalid typography property id"); uint flagMask = (uint)(((uint)1) << ((int)propertyId)); if (flagValue) _idPropertySetFlags |= flagMask; else _idPropertySetFlags &= ~flagMask; OnPropertiesChanged(); } private uint _idPropertySetFlags; private int _standardSwashes; private int _contextualSwashes; private int _stylisticAlternates; private int _annotationAlternates; private FontVariants _variant; private FontCapitals _capitals; private FontFraction _fraction; private FontNumeralStyle _numeralStyle; private FontNumeralAlignment _numeralAlignment; private FontEastAsianWidths _eastAsianWidths; private FontEastAsianLanguage _eastAsianLanguage; #endregion Private members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
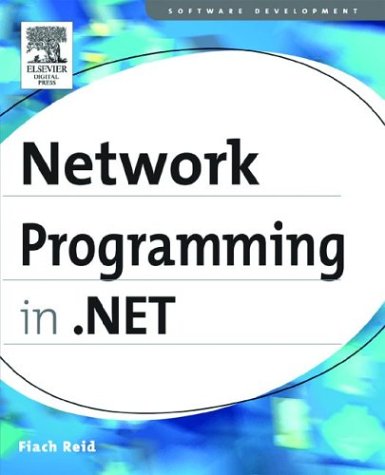
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompilerGlobalScopeAttribute.cs
- OdbcReferenceCollection.cs
- _NegotiateClient.cs
- XmlTextReaderImplHelpers.cs
- SystemIcmpV4Statistics.cs
- DBSchemaRow.cs
- ObjectTag.cs
- IRCollection.cs
- _MultipleConnectAsync.cs
- FrameworkContextData.cs
- UserControlCodeDomTreeGenerator.cs
- CqlIdentifiers.cs
- DoubleAnimation.cs
- AssemblyBuilder.cs
- DataServiceExpressionVisitor.cs
- Compiler.cs
- MaskedTextBox.cs
- CopyAction.cs
- SHA512Managed.cs
- TextDpi.cs
- MethodBody.cs
- ButtonField.cs
- Converter.cs
- OleDbFactory.cs
- NativeMethods.cs
- ButtonField.cs
- KeyBinding.cs
- XmlSchema.cs
- DurableRuntimeValidator.cs
- DetailsViewDeleteEventArgs.cs
- WeakEventManager.cs
- CredentialCache.cs
- AssertHelper.cs
- Semaphore.cs
- XmlSchemaObjectCollection.cs
- SelectionEditingBehavior.cs
- VariableQuery.cs
- WizardPanel.cs
- BindUriHelper.cs
- PropertyMap.cs
- DataBindingCollectionConverter.cs
- FolderNameEditor.cs
- XPathAxisIterator.cs
- CatalogPartChrome.cs
- WebPartDisplayModeCollection.cs
- HtmlInputButton.cs
- SmiContext.cs
- X509Certificate2Collection.cs
- PartialCachingControl.cs
- BinaryConverter.cs
- Animatable.cs
- XmlWellformedWriter.cs
- ConstrainedDataObject.cs
- EntityClassGenerator.cs
- BulletDecorator.cs
- ParameterToken.cs
- WindowsUpDown.cs
- PropertyExpression.cs
- ExtractedStateEntry.cs
- WinFormsComponentEditor.cs
- Internal.cs
- FilteredXmlReader.cs
- CacheSection.cs
- UiaCoreApi.cs
- DataService.cs
- ImageSourceConverter.cs
- EventProvider.cs
- Math.cs
- DelimitedListTraceListener.cs
- SQLRoleProvider.cs
- UriTemplate.cs
- PopOutPanel.cs
- StreamingContext.cs
- RegularExpressionValidator.cs
- ExpressionList.cs
- GridItem.cs
- OleDbFactory.cs
- StaticSiteMapProvider.cs
- GenericUI.cs
- DataGridViewCheckBoxColumn.cs
- VectorCollectionValueSerializer.cs
- DriveNotFoundException.cs
- VersionedStreamOwner.cs
- Internal.cs
- RotateTransform3D.cs
- Exceptions.cs
- SortDescriptionCollection.cs
- ILGenerator.cs
- PropertyGridDesigner.cs
- CacheHelper.cs
- DataGridViewBindingCompleteEventArgs.cs
- SingleAnimation.cs
- PatternMatcher.cs
- ToolboxBitmapAttribute.cs
- EntityRecordInfo.cs
- XsltInput.cs
- IdentifierCreationService.cs
- metadatamappinghashervisitor.cs
- ToolstripProfessionalRenderer.cs
- PersonalizationProviderHelper.cs