Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / RawKeyboardInputReport.cs / 1 / RawKeyboardInputReport.cs
using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32; using System.Windows; namespace System.Windows.Input { ////// The RawKeyboardInputReport class encapsulates the raw input /// provided from a keyboard. /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be /// marshalled across application domains. /// internal class RawKeyboardInputReport : InputReport { ////// Constructs ad instance of the RawKeyboardInputReport class. /// /// /// The input source that provided this input. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The set of actions being reported. /// /// /// The scan code if a key is being reported. /// /// /// The true if a key is an extended key. /// /// /// The true if a key is a system key. /// /// /// The Win32 virtual key code if a key is being reported. /// /// /// Any extra information being provided along with the input. /// ////// Critical:This handles critical data in the form of PresentationSource and /// ExtraInformation /// TreatAsSafe:The data has demands on the property when someone tries to access it. /// [SecurityCritical,SecurityTreatAsSafe] public RawKeyboardInputReport( PresentationSource inputSource, InputMode mode, int timestamp, RawKeyboardActions actions, int scanCode, bool isExtendedKey, bool isSystemKey, int virtualKey, IntPtr extraInformation) : base(inputSource, InputType.Keyboard, mode, timestamp) { if (!IsValidRawKeyboardActions(actions)) throw new System.ComponentModel.InvalidEnumArgumentException("actions", (int)actions, typeof(RawKeyboardActions)); _actions = actions; _scanCode = scanCode; _isExtendedKey = isExtendedKey; _isSystemKey = isSystemKey; _virtualKey = virtualKey; _extraInformation = new SecurityCriticalData(extraInformation); } /// /// Read-only access to the set of actions that were reported. /// public RawKeyboardActions Actions {get {return _actions;}} ////// Read-only access to the scan code that was reported. /// public int ScanCode {get {return _scanCode;}} ////// Read-only access to the flag of an extended key. /// public bool IsExtendedKey {get {return _isExtendedKey;}} ////// Read-only access to the flag of a system key. /// public bool IsSystemKey {get {return _isSystemKey;}} ////// Read-only access to the virtual key that was reported. /// public int VirtualKey {get {return _virtualKey;}} ////// Read-only access to the extra information was provided along /// with the input. /// ////// Critical: This data was got under an elevation and is not safe to expose /// public IntPtr ExtraInformation { [SecurityCritical] get { return _extraInformation.Value; } } // IsValid Method for RawKeyboardActions. Relies on the enum being flags. internal static bool IsValidRawKeyboardActions(RawKeyboardActions actions) { if (((RawKeyboardActions.AttributesChanged | RawKeyboardActions.Activate | RawKeyboardActions.Deactivate | RawKeyboardActions.KeyDown | RawKeyboardActions.KeyUp) & actions) == actions) { if (!((((RawKeyboardActions.KeyUp | RawKeyboardActions.KeyDown) & actions) == (RawKeyboardActions.KeyUp | RawKeyboardActions.KeyDown)) || ((RawKeyboardActions.Deactivate & actions) == actions && RawKeyboardActions.Deactivate != actions))) { return true; } } return false; } private RawKeyboardActions _actions; private int _scanCode; private bool _isExtendedKey; private bool _isSystemKey; private int _virtualKey; ////// Critical: This information is got under an elevation and can latch onto /// any arbitrary data /// private SecurityCriticalData_extraInformation; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32; using System.Windows; namespace System.Windows.Input { /// /// The RawKeyboardInputReport class encapsulates the raw input /// provided from a keyboard. /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be /// marshalled across application domains. /// internal class RawKeyboardInputReport : InputReport { ////// Constructs ad instance of the RawKeyboardInputReport class. /// /// /// The input source that provided this input. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The set of actions being reported. /// /// /// The scan code if a key is being reported. /// /// /// The true if a key is an extended key. /// /// /// The true if a key is a system key. /// /// /// The Win32 virtual key code if a key is being reported. /// /// /// Any extra information being provided along with the input. /// ////// Critical:This handles critical data in the form of PresentationSource and /// ExtraInformation /// TreatAsSafe:The data has demands on the property when someone tries to access it. /// [SecurityCritical,SecurityTreatAsSafe] public RawKeyboardInputReport( PresentationSource inputSource, InputMode mode, int timestamp, RawKeyboardActions actions, int scanCode, bool isExtendedKey, bool isSystemKey, int virtualKey, IntPtr extraInformation) : base(inputSource, InputType.Keyboard, mode, timestamp) { if (!IsValidRawKeyboardActions(actions)) throw new System.ComponentModel.InvalidEnumArgumentException("actions", (int)actions, typeof(RawKeyboardActions)); _actions = actions; _scanCode = scanCode; _isExtendedKey = isExtendedKey; _isSystemKey = isSystemKey; _virtualKey = virtualKey; _extraInformation = new SecurityCriticalData(extraInformation); } /// /// Read-only access to the set of actions that were reported. /// public RawKeyboardActions Actions {get {return _actions;}} ////// Read-only access to the scan code that was reported. /// public int ScanCode {get {return _scanCode;}} ////// Read-only access to the flag of an extended key. /// public bool IsExtendedKey {get {return _isExtendedKey;}} ////// Read-only access to the flag of a system key. /// public bool IsSystemKey {get {return _isSystemKey;}} ////// Read-only access to the virtual key that was reported. /// public int VirtualKey {get {return _virtualKey;}} ////// Read-only access to the extra information was provided along /// with the input. /// ////// Critical: This data was got under an elevation and is not safe to expose /// public IntPtr ExtraInformation { [SecurityCritical] get { return _extraInformation.Value; } } // IsValid Method for RawKeyboardActions. Relies on the enum being flags. internal static bool IsValidRawKeyboardActions(RawKeyboardActions actions) { if (((RawKeyboardActions.AttributesChanged | RawKeyboardActions.Activate | RawKeyboardActions.Deactivate | RawKeyboardActions.KeyDown | RawKeyboardActions.KeyUp) & actions) == actions) { if (!((((RawKeyboardActions.KeyUp | RawKeyboardActions.KeyDown) & actions) == (RawKeyboardActions.KeyUp | RawKeyboardActions.KeyDown)) || ((RawKeyboardActions.Deactivate & actions) == actions && RawKeyboardActions.Deactivate != actions))) { return true; } } return false; } private RawKeyboardActions _actions; private int _scanCode; private bool _isExtendedKey; private bool _isSystemKey; private int _virtualKey; ////// Critical: This information is got under an elevation and can latch onto /// any arbitrary data /// private SecurityCriticalData_extraInformation; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
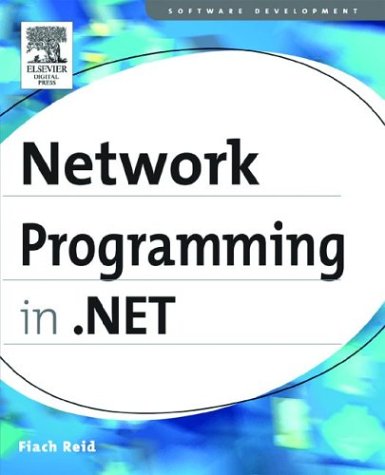
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- oledbconnectionstring.cs
- CurrencyManager.cs
- ExceptionRoutedEventArgs.cs
- PersonalizationState.cs
- EpmContentSerializerBase.cs
- AttributeUsageAttribute.cs
- WorkflowWebHostingModule.cs
- SessionIDManager.cs
- BlurBitmapEffect.cs
- IndexedEnumerable.cs
- ComponentCollection.cs
- Win32MouseDevice.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- DES.cs
- ServiceOperationInfoTypeConverter.cs
- ChannelTraceRecord.cs
- SqlCacheDependencyDatabase.cs
- UpWmlPageAdapter.cs
- LabelDesigner.cs
- EntityDataSourceDesigner.cs
- PointAnimationUsingPath.cs
- MD5.cs
- SafeNativeMethodsCLR.cs
- DummyDataSource.cs
- PrimarySelectionGlyph.cs
- SiteMapPathDesigner.cs
- BindToObject.cs
- Pool.cs
- DataViewListener.cs
- RemotingConfigParser.cs
- SystemIcons.cs
- PointAnimationClockResource.cs
- FixedStringLookup.cs
- NamespaceExpr.cs
- EntityContainerEntitySetDefiningQuery.cs
- PersonalizationProvider.cs
- XmlElementAttributes.cs
- TextSimpleMarkerProperties.cs
- DateTimeUtil.cs
- SqlAggregateChecker.cs
- CacheAxisQuery.cs
- HttpResponse.cs
- SQLUtility.cs
- RayHitTestParameters.cs
- ReadOnlyAttribute.cs
- EncodingNLS.cs
- CompositeDuplexBindingElement.cs
- DataGridColumn.cs
- ComponentCollection.cs
- ActivationArguments.cs
- DataControlLinkButton.cs
- ParameterCollection.cs
- PerformanceCounterPermissionEntry.cs
- NTAccount.cs
- ObjectDataSourceWizardForm.cs
- ZoneLinkButton.cs
- HttpProfileBase.cs
- TextTreeTextBlock.cs
- DbProviderFactory.cs
- WindowsStatusBar.cs
- NetworkInterface.cs
- TextSegment.cs
- SHA256Managed.cs
- BindingNavigator.cs
- EntityDataSourceWrapperCollection.cs
- ValidationPropertyAttribute.cs
- DbProviderSpecificTypePropertyAttribute.cs
- MenuItemStyleCollectionEditor.cs
- ZoneMembershipCondition.cs
- ClassicBorderDecorator.cs
- FindProgressChangedEventArgs.cs
- MetadataPropertyAttribute.cs
- InputReferenceExpression.cs
- PointAnimationUsingPath.cs
- PerformanceCounter.cs
- BitVector32.cs
- ResourceContainerWrapper.cs
- SafeProcessHandle.cs
- BaseWebProxyFinder.cs
- Constants.cs
- TraceSwitch.cs
- FormsAuthenticationCredentials.cs
- CatalogPartDesigner.cs
- TableProviderWrapper.cs
- MenuBindingsEditorForm.cs
- RSAOAEPKeyExchangeFormatter.cs
- TypeDependencyAttribute.cs
- XmlUtil.cs
- Clause.cs
- PngBitmapEncoder.cs
- ParallelEnumerable.cs
- ProcessHost.cs
- IsolatedStorageFilePermission.cs
- VSWCFServiceContractGenerator.cs
- RoutedEventConverter.cs
- QuaternionValueSerializer.cs
- QueryLifecycle.cs
- EditBehavior.cs
- FixedPageProcessor.cs
- SqlUserDefinedTypeAttribute.cs