Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / ColorConverter.cs / 1 / ColorConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001, 2002 // // File: ColorConverter.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ColorConverter Parses a color. /// public sealed class ColorConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFromString /// public static new object ConvertFromString(string value) { if ( null == value) { return null; } return Parsers.ParseColor(value, null); } ////// ConvertFrom - attempt to convert to a Color from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Color. /// public override object ConvertFrom(ITypeDescriptorContext td, System.Globalization.CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } return Parsers.ParseColor(value as string, ci, td); } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a Color, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Color.FromArgb, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Color) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(Color).GetMethod("FromArgb", new Type[]{typeof(byte), typeof(byte), typeof(byte), typeof(byte)}); Color c = (Color)value; return new InstanceDescriptor(mi, new object[]{c.A, c.R, c.G, c.B}); } else if (destinationType == typeof(string)) { Color c = (Color)value; return c.ToString(culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001, 2002 // // File: ColorConverter.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ColorConverter Parses a color. /// public sealed class ColorConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFromString /// public static new object ConvertFromString(string value) { if ( null == value) { return null; } return Parsers.ParseColor(value, null); } ////// ConvertFrom - attempt to convert to a Color from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Color. /// public override object ConvertFrom(ITypeDescriptorContext td, System.Globalization.CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } return Parsers.ParseColor(value as string, ci, td); } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a Color, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Color.FromArgb, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Color) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(Color).GetMethod("FromArgb", new Type[]{typeof(byte), typeof(byte), typeof(byte), typeof(byte)}); Color c = (Color)value; return new InstanceDescriptor(mi, new object[]{c.A, c.R, c.G, c.B}); } else if (destinationType == typeof(string)) { Color c = (Color)value; return c.ToString(culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
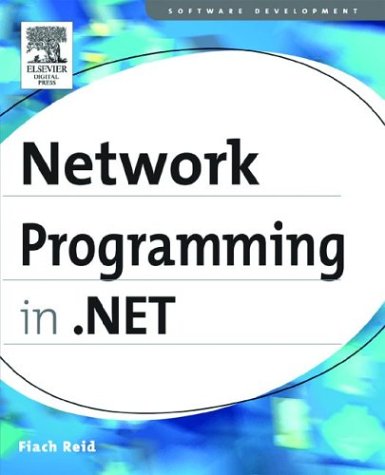
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfileService.cs
- CheckBox.cs
- FullTextBreakpoint.cs
- CheckBoxDesigner.cs
- JavascriptCallbackMessageInspector.cs
- Interlocked.cs
- QueryCacheKey.cs
- CheckoutException.cs
- TextRunCacheImp.cs
- ToolZone.cs
- ProcessRequestArgs.cs
- EntityTypeBase.cs
- Perspective.cs
- StorageBasedPackageProperties.cs
- RadioButtonPopupAdapter.cs
- SchemaImporterExtensionsSection.cs
- QueuePathDialog.cs
- RotateTransform.cs
- TcpProcessProtocolHandler.cs
- IODescriptionAttribute.cs
- RepeatButton.cs
- PathGeometry.cs
- PeerInputChannelListener.cs
- LabelLiteral.cs
- TogglePatternIdentifiers.cs
- PrePostDescendentsWalker.cs
- InputBuffer.cs
- _NtlmClient.cs
- ObjectQuery_EntitySqlExtensions.cs
- PropertyGridCommands.cs
- ExpressionCopier.cs
- DataColumnPropertyDescriptor.cs
- PolyLineSegmentFigureLogic.cs
- ResourceSet.cs
- ApplicationActivator.cs
- LocatorBase.cs
- BasePattern.cs
- SqlWriter.cs
- SqlDataSourceFilteringEventArgs.cs
- NavigationWindowAutomationPeer.cs
- XmlDocument.cs
- JsonWriter.cs
- Variant.cs
- WebBrowserContainer.cs
- SpellerHighlightLayer.cs
- SelectedGridItemChangedEvent.cs
- Command.cs
- NewArray.cs
- FileDataSourceCache.cs
- Tag.cs
- SnapshotChangeTrackingStrategy.cs
- LinkUtilities.cs
- DragDeltaEventArgs.cs
- DashStyles.cs
- RegexReplacement.cs
- XmlSchemaSet.cs
- SoapIgnoreAttribute.cs
- ByteStack.cs
- ThreadPool.cs
- FileVersionInfo.cs
- XmlElement.cs
- Vector3D.cs
- ApplicationServicesHostFactory.cs
- JournalEntry.cs
- PointConverter.cs
- TcpStreams.cs
- PropertyStore.cs
- FormViewDeleteEventArgs.cs
- Types.cs
- WorkflowDesignerMessageFilter.cs
- TailCallAnalyzer.cs
- TreeViewBindingsEditor.cs
- Vector3dCollection.cs
- AppModelKnownContentFactory.cs
- RSAPKCS1SignatureDeformatter.cs
- BitmapFrameEncode.cs
- InternalBufferOverflowException.cs
- TrackingRecord.cs
- HttpResponseBase.cs
- TextBox.cs
- ChannelManagerHelpers.cs
- InvalidComObjectException.cs
- WebServiceData.cs
- ToolStripContentPanelRenderEventArgs.cs
- AuthStoreRoleProvider.cs
- ColorBuilder.cs
- SqlDependencyListener.cs
- ListBoxItemAutomationPeer.cs
- ContentElement.cs
- DataSourceBooleanViewSchemaConverter.cs
- SerializationObjectManager.cs
- SessionSwitchEventArgs.cs
- DrawingCollection.cs
- KeyFrames.cs
- ISO2022Encoding.cs
- GcSettings.cs
- XmlDataSource.cs
- HistoryEventArgs.cs
- XslAstAnalyzer.cs
- Color.cs