Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / DragEventArgs.cs / 1305600 / DragEventArgs.cs
//---------------------------------------------------------------------------- // // File: DragEventArgs.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: DragEventArgs for drag-and-drop operation. // // // History: // 08/19/2004 : sangilj Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// The DragEventArgs class represents a type of RoutedEventArgs that /// are relevant to all drag events(DragEnter/DragOver/DragLeave/DragDrop). /// public sealed class DragEventArgs : RoutedEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructs a DragEventArgs instance. /// /// /// The data object used in this drag drop operation. /// /// /// The current state of the mouse button and modifier keys. /// /// /// Allowed effects of a drag-and-drop operation. /// /// /// The target of the event. /// /// /// The current mouse position of the target. /// internal DragEventArgs(IDataObject data, DragDropKeyStates dragDropKeyStates, DragDropEffects allowedEffects, DependencyObject target, Point point) { if (!DragDrop.IsValidDragDropKeyStates(dragDropKeyStates)) { Debug.Assert(false, "Invalid dragDropKeyStates"); } if (!DragDrop.IsValidDragDropEffects(allowedEffects)) { Debug.Assert(false, "Invalid allowedEffects"); } if (target == null) { Debug.Assert(false, "Invalid target"); } this._data = data; this._dragDropKeyStates = dragDropKeyStates; this._allowedEffects = allowedEffects; this._target = target; this._dropPoint = point; this._effects = allowedEffects; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// The point of drop operation that based on relativeTo. /// public Point GetPosition(IInputElement relativeTo) { Point dropPoint; if (relativeTo == null) { throw new ArgumentNullException("relativeTo"); } dropPoint = new Point(0, 0); if (_target != null) { // Translate the drop point from the drop target to the relative element. dropPoint = InputElement.TranslatePoint(_dropPoint, _target, (DependencyObject)relativeTo); } return dropPoint; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// The data object of drop operation /// public IDataObject Data { get { return _data; } } ////// The DragDropKeyStates that indicates the current states for /// physical keyboard keys and mouse buttons. /// public DragDropKeyStates KeyStates { get { return _dragDropKeyStates; } } ////// The allowed effects of drag and drop operation /// public DragDropEffects AllowedEffects { get { return _allowedEffects; } } ////// The effects of drag and drop operation /// public DragDropEffects Effects { get { return _effects; } set { if (!DragDrop.IsValidDragDropEffects(value)) { throw new ArgumentException(SR.Get(SRID.DragDrop_DragDropEffectsInvalid, "value")); } _effects = value; } } #endregion Public Properties #region Protected Methods //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DragEventHandler handler = (DragEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private IDataObject _data; private DragDropKeyStates _dragDropKeyStates; private DragDropEffects _allowedEffects; private DragDropEffects _effects; private DependencyObject _target; private Point _dropPoint; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: DragEventArgs.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: DragEventArgs for drag-and-drop operation. // // // History: // 08/19/2004 : sangilj Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// The DragEventArgs class represents a type of RoutedEventArgs that /// are relevant to all drag events(DragEnter/DragOver/DragLeave/DragDrop). /// public sealed class DragEventArgs : RoutedEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructs a DragEventArgs instance. /// /// /// The data object used in this drag drop operation. /// /// /// The current state of the mouse button and modifier keys. /// /// /// Allowed effects of a drag-and-drop operation. /// /// /// The target of the event. /// /// /// The current mouse position of the target. /// internal DragEventArgs(IDataObject data, DragDropKeyStates dragDropKeyStates, DragDropEffects allowedEffects, DependencyObject target, Point point) { if (!DragDrop.IsValidDragDropKeyStates(dragDropKeyStates)) { Debug.Assert(false, "Invalid dragDropKeyStates"); } if (!DragDrop.IsValidDragDropEffects(allowedEffects)) { Debug.Assert(false, "Invalid allowedEffects"); } if (target == null) { Debug.Assert(false, "Invalid target"); } this._data = data; this._dragDropKeyStates = dragDropKeyStates; this._allowedEffects = allowedEffects; this._target = target; this._dropPoint = point; this._effects = allowedEffects; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// The point of drop operation that based on relativeTo. /// public Point GetPosition(IInputElement relativeTo) { Point dropPoint; if (relativeTo == null) { throw new ArgumentNullException("relativeTo"); } dropPoint = new Point(0, 0); if (_target != null) { // Translate the drop point from the drop target to the relative element. dropPoint = InputElement.TranslatePoint(_dropPoint, _target, (DependencyObject)relativeTo); } return dropPoint; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// The data object of drop operation /// public IDataObject Data { get { return _data; } } ////// The DragDropKeyStates that indicates the current states for /// physical keyboard keys and mouse buttons. /// public DragDropKeyStates KeyStates { get { return _dragDropKeyStates; } } ////// The allowed effects of drag and drop operation /// public DragDropEffects AllowedEffects { get { return _allowedEffects; } } ////// The effects of drag and drop operation /// public DragDropEffects Effects { get { return _effects; } set { if (!DragDrop.IsValidDragDropEffects(value)) { throw new ArgumentException(SR.Get(SRID.DragDrop_DragDropEffectsInvalid, "value")); } _effects = value; } } #endregion Public Properties #region Protected Methods //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DragEventHandler handler = (DragEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private IDataObject _data; private DragDropKeyStates _dragDropKeyStates; private DragDropEffects _allowedEffects; private DragDropEffects _effects; private DependencyObject _target; private Point _dropPoint; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
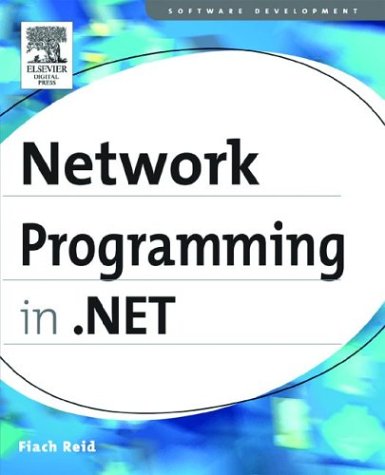
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentGridContextMenu.cs
- ScrollItemProviderWrapper.cs
- MenuItem.cs
- RichTextBoxConstants.cs
- AssemblyName.cs
- ReadOnlyDataSource.cs
- SwitchAttribute.cs
- UnmanagedMemoryStream.cs
- NativeCompoundFileAPIs.cs
- HtmlTableRowCollection.cs
- TemplateControl.cs
- CardSpaceSelector.cs
- DispatcherObject.cs
- UnsafeNativeMethods.cs
- DataGridViewComboBoxCell.cs
- BamlWriter.cs
- AnnotationResourceChangedEventArgs.cs
- CompiledIdentityConstraint.cs
- CodeDirectoryCompiler.cs
- ConfigurationPropertyAttribute.cs
- login.cs
- StorageTypeMapping.cs
- Listbox.cs
- XmlTypeMapping.cs
- TransformPatternIdentifiers.cs
- TransformedBitmap.cs
- BlurBitmapEffect.cs
- ZipPackage.cs
- DeferredSelectedIndexReference.cs
- XmlSchemaComplexContent.cs
- EntityViewGenerator.cs
- FileDialog_Vista_Interop.cs
- Type.cs
- CodeTypeReferenceCollection.cs
- XmlSchemaIdentityConstraint.cs
- StrongNamePublicKeyBlob.cs
- CustomAssemblyResolver.cs
- EntityKeyElement.cs
- WebDisplayNameAttribute.cs
- SQLMoneyStorage.cs
- CodeCatchClause.cs
- ObjectContext.cs
- ListItemViewControl.cs
- DispatchChannelSink.cs
- RightsManagementPermission.cs
- DispatcherHookEventArgs.cs
- SafeFileHandle.cs
- MimeFormatExtensions.cs
- URI.cs
- InternalPermissions.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ServiceDescription.cs
- HashCodeCombiner.cs
- RuntimeHelpers.cs
- TimeoutHelper.cs
- VectorCollectionConverter.cs
- TypeLibConverter.cs
- AssemblyInfo.cs
- InfoCardCryptoHelper.cs
- StrongNameMembershipCondition.cs
- AffineTransform3D.cs
- DaylightTime.cs
- WebServiceData.cs
- XmlMembersMapping.cs
- SQLGuid.cs
- SuppressMessageAttribute.cs
- OSFeature.cs
- ReferencedCollectionType.cs
- FirstQueryOperator.cs
- ThrowHelper.cs
- IntSecurity.cs
- SecurityKeyType.cs
- ProfilePropertySettings.cs
- SoapDocumentServiceAttribute.cs
- Transform.cs
- StringReader.cs
- CodeSnippetExpression.cs
- SqlDataSourceEnumerator.cs
- SQLBinary.cs
- MediaSystem.cs
- GradientStop.cs
- PageCache.cs
- TextUtf8RawTextWriter.cs
- StringResourceManager.cs
- DataPager.cs
- XmlIlVisitor.cs
- _UriSyntax.cs
- FileDialog_Vista_Interop.cs
- GridViewAutomationPeer.cs
- FocusWithinProperty.cs
- EdmItemError.cs
- IfJoinedCondition.cs
- BaseDataList.cs
- RIPEMD160.cs
- PenThreadPool.cs
- JavaScriptString.cs
- GroupBoxRenderer.cs
- ByteRangeDownloader.cs
- BitmapEffectDrawing.cs
- ColumnBinding.cs