Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Mapping / StorageComplexTypeMapping.cs / 1305376 / StorageComplexTypeMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping { ////// Mapping metadata for Complex Types. /// internal class StorageComplexTypeMapping { #region Constructors ////// Construct a new Complex Property mapping object /// /// Whether the property mapping representation is /// totally represented in this table mapping fragment or not. internal StorageComplexTypeMapping(bool isPartial) { m_isPartial = isPartial; } #endregion #region Fields Dictionarym_properties = new Dictionary (StringComparer.Ordinal); //child property mappings that make up this complex property Dictionary m_conditionProperties = new Dictionary (EqualityComparer .Default); //Condition property mappings for this complex type bool m_isPartial; //Whether the property mapping representation is //totally represented in this table mapping fragment or not. private Dictionary m_types = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for. private Dictionary m_isOfTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for // not only the type specified but the sub-types of that type as well. #endregion #region Properties /// /// a list of TypeMetadata that this mapping holds true for. /// internal ReadOnlyCollectionTypes { get { return new List (m_types.Values).AsReadOnly(); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal ReadOnlyCollectionIsOfTypes { get { return new List (m_isOfTypes.Values).AsReadOnly(); } } /// /// List of child properties that make up this complex property /// internal ReadOnlyCollectionProperties { get { return new List (m_properties.Values).AsReadOnly(); } } /// /// Returns all the property mappings defined in the complex type mapping /// including Properties and Condition Properties /// internal ReadOnlyCollectionAllProperties { get { List properties = new List (); properties.AddRange(m_properties.Values); properties.AddRange(m_conditionProperties.Values); return properties.AsReadOnly(); } } ///// ///// Whether the property mapping representation is ///// totally represented in this table mapping fragment or not. ///// //internal bool IsPartial { // get { // return m_isPartial; // } //} #endregion #region Methods ////// Add a Type to the list of types that this mapping is valid for /// internal void AddType(ComplexType type) { m_types.Add(type.FullName, type); } ////// Add a Type to the list of Is-Of types that this mapping is valid for /// internal void AddIsOfType(ComplexType type) { m_isOfTypes.Add(type.FullName, type); } ////// Add a property mapping as a child of this complex property mapping /// /// The mapping that needs to be added internal void AddProperty(StoragePropertyMapping prop) { m_properties.Add(prop.EdmProperty.Name, prop); } ////// Add a condition property mapping as a child of this complex property mapping /// Condition Property Mapping specifies a Condition either on the C side property or S side property. /// /// The Condition Property mapping that needs to be added internal void AddConditionProperty(StorageConditionPropertyMapping conditionPropertyMap) { //Same Member can not have more than one Condition with in the //same Complex Type. EdmProperty conditionMember = (conditionPropertyMap.EdmProperty != null) ? conditionPropertyMap.EdmProperty : conditionPropertyMap.ColumnProperty; Debug.Assert(conditionMember != null); if (m_conditionProperties.ContainsKey(conditionMember)) { throw new MappingException(System.Data.Entity.Strings.Mapping_InvalidContent_Duplicate_Condition_Member_1(conditionMember.Name)); } m_conditionProperties.Add(conditionMember, conditionPropertyMap); } ////// The method finds the type in which the member with the given name exists /// form the list of IsOfTypes and Type. /// /// ///internal ComplexType GetOwnerType(string memberName) { foreach (ComplexType type in m_types.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } foreach (ComplexType type in m_isOfTypes.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } return null; } /// /// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("ComplexTypeMapping"); sb.Append(" "); if (m_isPartial) { sb.Append("IsPartial:True"); } sb.Append(" "); foreach (ComplexType type in m_types.Values) { sb.Append("Types:"); sb.Append(type.FullName); sb.Append(" "); } foreach (ComplexType type in m_isOfTypes.Values) { sb.Append("Is-Of Types:"); sb.Append(type.FullName); sb.Append(" "); } Console.WriteLine(sb.ToString()); foreach (StorageConditionPropertyMapping conditionMap in m_conditionProperties.Values) (conditionMap).Print(index + 5); foreach (StoragePropertyMapping propertyMapping in Properties) { propertyMapping.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping { ////// Mapping metadata for Complex Types. /// internal class StorageComplexTypeMapping { #region Constructors ////// Construct a new Complex Property mapping object /// /// Whether the property mapping representation is /// totally represented in this table mapping fragment or not. internal StorageComplexTypeMapping(bool isPartial) { m_isPartial = isPartial; } #endregion #region Fields Dictionarym_properties = new Dictionary (StringComparer.Ordinal); //child property mappings that make up this complex property Dictionary m_conditionProperties = new Dictionary (EqualityComparer .Default); //Condition property mappings for this complex type bool m_isPartial; //Whether the property mapping representation is //totally represented in this table mapping fragment or not. private Dictionary m_types = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for. private Dictionary m_isOfTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for // not only the type specified but the sub-types of that type as well. #endregion #region Properties /// /// a list of TypeMetadata that this mapping holds true for. /// internal ReadOnlyCollectionTypes { get { return new List (m_types.Values).AsReadOnly(); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal ReadOnlyCollectionIsOfTypes { get { return new List (m_isOfTypes.Values).AsReadOnly(); } } /// /// List of child properties that make up this complex property /// internal ReadOnlyCollectionProperties { get { return new List (m_properties.Values).AsReadOnly(); } } /// /// Returns all the property mappings defined in the complex type mapping /// including Properties and Condition Properties /// internal ReadOnlyCollectionAllProperties { get { List properties = new List (); properties.AddRange(m_properties.Values); properties.AddRange(m_conditionProperties.Values); return properties.AsReadOnly(); } } ///// ///// Whether the property mapping representation is ///// totally represented in this table mapping fragment or not. ///// //internal bool IsPartial { // get { // return m_isPartial; // } //} #endregion #region Methods ////// Add a Type to the list of types that this mapping is valid for /// internal void AddType(ComplexType type) { m_types.Add(type.FullName, type); } ////// Add a Type to the list of Is-Of types that this mapping is valid for /// internal void AddIsOfType(ComplexType type) { m_isOfTypes.Add(type.FullName, type); } ////// Add a property mapping as a child of this complex property mapping /// /// The mapping that needs to be added internal void AddProperty(StoragePropertyMapping prop) { m_properties.Add(prop.EdmProperty.Name, prop); } ////// Add a condition property mapping as a child of this complex property mapping /// Condition Property Mapping specifies a Condition either on the C side property or S side property. /// /// The Condition Property mapping that needs to be added internal void AddConditionProperty(StorageConditionPropertyMapping conditionPropertyMap) { //Same Member can not have more than one Condition with in the //same Complex Type. EdmProperty conditionMember = (conditionPropertyMap.EdmProperty != null) ? conditionPropertyMap.EdmProperty : conditionPropertyMap.ColumnProperty; Debug.Assert(conditionMember != null); if (m_conditionProperties.ContainsKey(conditionMember)) { throw new MappingException(System.Data.Entity.Strings.Mapping_InvalidContent_Duplicate_Condition_Member_1(conditionMember.Name)); } m_conditionProperties.Add(conditionMember, conditionPropertyMap); } ////// The method finds the type in which the member with the given name exists /// form the list of IsOfTypes and Type. /// /// ///internal ComplexType GetOwnerType(string memberName) { foreach (ComplexType type in m_types.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } foreach (ComplexType type in m_isOfTypes.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } return null; } /// /// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("ComplexTypeMapping"); sb.Append(" "); if (m_isPartial) { sb.Append("IsPartial:True"); } sb.Append(" "); foreach (ComplexType type in m_types.Values) { sb.Append("Types:"); sb.Append(type.FullName); sb.Append(" "); } foreach (ComplexType type in m_isOfTypes.Values) { sb.Append("Is-Of Types:"); sb.Append(type.FullName); sb.Append(" "); } Console.WriteLine(sb.ToString()); foreach (StorageConditionPropertyMapping conditionMap in m_conditionProperties.Values) (conditionMap).Print(index + 5); foreach (StoragePropertyMapping propertyMapping in Properties) { propertyMapping.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
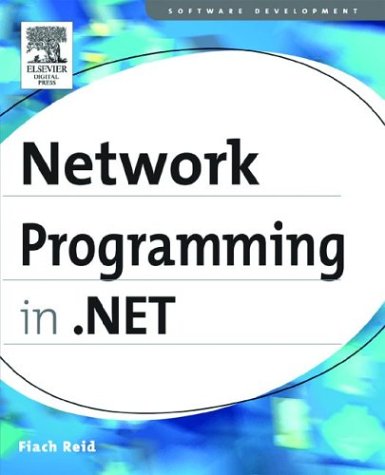
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamespaceInfo.cs
- ObjectDataSourceFilteringEventArgs.cs
- JoinTreeNode.cs
- XslAstAnalyzer.cs
- XamlDesignerSerializationManager.cs
- SafeNativeMethods.cs
- DependencyObjectPropertyDescriptor.cs
- ProcessInfo.cs
- Exception.cs
- Encoder.cs
- QilChoice.cs
- TcpSocketManager.cs
- CacheHelper.cs
- Window.cs
- XmlSchemaSimpleContentExtension.cs
- SrgsNameValueTag.cs
- RequestDescription.cs
- SerializationObjectManager.cs
- ETagAttribute.cs
- PageCatalogPart.cs
- EventlogProvider.cs
- LogArchiveSnapshot.cs
- ClientScriptManagerWrapper.cs
- RSAPKCS1SignatureFormatter.cs
- XmlAutoDetectWriter.cs
- DnsCache.cs
- XPathAncestorQuery.cs
- WSSecurityPolicy11.cs
- DefaultValueTypeConverter.cs
- ToolBarPanel.cs
- DecoderReplacementFallback.cs
- DrawingAttributes.cs
- HtmlTableCellCollection.cs
- Delegate.cs
- QuestionEventArgs.cs
- Marshal.cs
- ConstructorExpr.cs
- Panel.cs
- ResourcesChangeInfo.cs
- ObfuscationAttribute.cs
- SqlInternalConnectionSmi.cs
- _ListenerRequestStream.cs
- ManagementObjectCollection.cs
- TaiwanCalendar.cs
- Win32PrintDialog.cs
- XmlSchemaException.cs
- NavigationHelper.cs
- QilVisitor.cs
- HttpApplication.cs
- SettingsPropertyValue.cs
- ComponentSerializationService.cs
- DocumentPageView.cs
- SafeMILHandleMemoryPressure.cs
- LinqDataSource.cs
- OleDbWrapper.cs
- NetworkInformationPermission.cs
- TextElementEditingBehaviorAttribute.cs
- GridViewRowEventArgs.cs
- VisualStyleTypesAndProperties.cs
- EditingCoordinator.cs
- PerformanceCounter.cs
- XmlDeclaration.cs
- ScaleTransform.cs
- AppDomainUnloadedException.cs
- QuadraticBezierSegment.cs
- DelegateOutArgument.cs
- DesignTimeData.cs
- RecognizedWordUnit.cs
- NetCodeGroup.cs
- CommentEmitter.cs
- Vector3DCollectionConverter.cs
- XhtmlBasicListAdapter.cs
- ResourceReferenceExpressionConverter.cs
- XmlObjectSerializerWriteContextComplex.cs
- PixelFormat.cs
- XmlSchemaParticle.cs
- ConstantProjectedSlot.cs
- ReverseComparer.cs
- InstancePersistenceEvent.cs
- EventHandlersDesigner.cs
- Int64Animation.cs
- WebPartVerb.cs
- MessageRpc.cs
- RawStylusInputReport.cs
- UnionExpr.cs
- MarshalByRefObject.cs
- MouseActionConverter.cs
- FieldDescriptor.cs
- StylusSystemGestureEventArgs.cs
- DiscoveryEndpointElement.cs
- StrokeCollection2.cs
- ThreadNeutralSemaphore.cs
- MasterPageParser.cs
- StaticFileHandler.cs
- Utils.cs
- MergeFailedEvent.cs
- MessageQueueTransaction.cs
- DataBindingCollection.cs
- SvcMapFileSerializer.cs
- LoginUtil.cs