Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / DataBindingCollection.cs / 1 / DataBindingCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Web.Util; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataBindingCollection : ICollection { private EventHandler changedEvent; private Hashtable bindings; private Hashtable removedBindings; ////// public DataBindingCollection() { this.bindings = new Hashtable(StringComparer.OrdinalIgnoreCase); } ////// public int Count { get { return bindings.Count; } } ////// public bool IsReadOnly { get { return false; } } ////// public bool IsSynchronized { get { return false; } } ////// public string[] RemovedBindings { get { int bindingCount = 0; ICollection keys = null; if (removedBindings != null) { keys = removedBindings.Keys; bindingCount = keys.Count; string[] removedNames = new string[bindingCount]; int i = 0; foreach (string s in keys) { removedNames[i++] = s; } removedBindings.Clear(); return removedNames; } else { return new string[0]; } } } ////// private Hashtable RemovedBindingsTable { get { if (removedBindings == null) { removedBindings = new Hashtable(StringComparer.OrdinalIgnoreCase); } return removedBindings; } } ////// public object SyncRoot { get { return this; } } ////// public DataBinding this[string propertyName] { get { object o = bindings[propertyName]; if (o != null) return(DataBinding)o; return null; } } public event EventHandler Changed { add { changedEvent = (EventHandler)Delegate.Combine(changedEvent, value); } remove { changedEvent = (EventHandler)Delegate.Remove(changedEvent, value); } } ////// public void Add(DataBinding binding) { bindings[binding.PropertyName] = binding; RemovedBindingsTable.Remove(binding.PropertyName); OnChanged(); } ////// public bool Contains(string propertyName) { return bindings.Contains(propertyName); } ////// public void Clear() { ICollection keys = bindings.Keys; if ((keys.Count != 0) && (removedBindings == null)) { // ensure the removedBindings hashtable is created Hashtable h = RemovedBindingsTable; } foreach (string s in keys) { removedBindings[s] = String.Empty; } bindings.Clear(); OnChanged(); } ////// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ////// public IEnumerator GetEnumerator() { return bindings.Values.GetEnumerator(); } private void OnChanged() { if (changedEvent != null) { changedEvent(this, EventArgs.Empty); } } ////// public void Remove(string propertyName) { Remove(propertyName, true); } ////// public void Remove(DataBinding binding) { Remove(binding.PropertyName, true); } ////// public void Remove(string propertyName, bool addToRemovedList) { if (Contains(propertyName)) { bindings.Remove(propertyName); if (addToRemovedList) { RemovedBindingsTable[propertyName] = String.Empty; } OnChanged(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Web.Util; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataBindingCollection : ICollection { private EventHandler changedEvent; private Hashtable bindings; private Hashtable removedBindings; ////// public DataBindingCollection() { this.bindings = new Hashtable(StringComparer.OrdinalIgnoreCase); } ////// public int Count { get { return bindings.Count; } } ////// public bool IsReadOnly { get { return false; } } ////// public bool IsSynchronized { get { return false; } } ////// public string[] RemovedBindings { get { int bindingCount = 0; ICollection keys = null; if (removedBindings != null) { keys = removedBindings.Keys; bindingCount = keys.Count; string[] removedNames = new string[bindingCount]; int i = 0; foreach (string s in keys) { removedNames[i++] = s; } removedBindings.Clear(); return removedNames; } else { return new string[0]; } } } ////// private Hashtable RemovedBindingsTable { get { if (removedBindings == null) { removedBindings = new Hashtable(StringComparer.OrdinalIgnoreCase); } return removedBindings; } } ////// public object SyncRoot { get { return this; } } ////// public DataBinding this[string propertyName] { get { object o = bindings[propertyName]; if (o != null) return(DataBinding)o; return null; } } public event EventHandler Changed { add { changedEvent = (EventHandler)Delegate.Combine(changedEvent, value); } remove { changedEvent = (EventHandler)Delegate.Remove(changedEvent, value); } } ////// public void Add(DataBinding binding) { bindings[binding.PropertyName] = binding; RemovedBindingsTable.Remove(binding.PropertyName); OnChanged(); } ////// public bool Contains(string propertyName) { return bindings.Contains(propertyName); } ////// public void Clear() { ICollection keys = bindings.Keys; if ((keys.Count != 0) && (removedBindings == null)) { // ensure the removedBindings hashtable is created Hashtable h = RemovedBindingsTable; } foreach (string s in keys) { removedBindings[s] = String.Empty; } bindings.Clear(); OnChanged(); } ////// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ////// public IEnumerator GetEnumerator() { return bindings.Values.GetEnumerator(); } private void OnChanged() { if (changedEvent != null) { changedEvent(this, EventArgs.Empty); } } ////// public void Remove(string propertyName) { Remove(propertyName, true); } ////// public void Remove(DataBinding binding) { Remove(binding.PropertyName, true); } ////// public void Remove(string propertyName, bool addToRemovedList) { if (Contains(propertyName)) { bindings.Remove(propertyName); if (addToRemovedList) { RemovedBindingsTable[propertyName] = String.Empty; } OnChanged(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
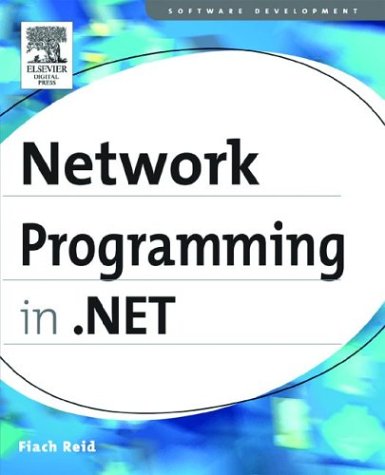
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrtCap_Builder.cs
- TextRangeAdaptor.cs
- TileBrush.cs
- ClientSettingsSection.cs
- PixelFormat.cs
- XmlElementAttributes.cs
- ContractsBCL.cs
- Animatable.cs
- LinearGradientBrush.cs
- WebPartConnectionsConnectVerb.cs
- Int16AnimationBase.cs
- NamedPipeConnectionPool.cs
- KeyInfo.cs
- ClientSideQueueItem.cs
- StatusBarItemAutomationPeer.cs
- QueryUtil.cs
- DesignTimeVisibleAttribute.cs
- BooleanSwitch.cs
- GlobalDataBindingHandler.cs
- GridErrorDlg.cs
- MessageAction.cs
- ConstNode.cs
- ToolStripSplitStackLayout.cs
- EndpointNameMessageFilter.cs
- XmlObjectSerializerWriteContextComplex.cs
- DataObjectPastingEventArgs.cs
- EntityDesignerDataSourceView.cs
- BodyWriter.cs
- CompModSwitches.cs
- ScriptServiceAttribute.cs
- HandoffBehavior.cs
- SQLDouble.cs
- Converter.cs
- Attributes.cs
- SymLanguageType.cs
- ToolStripContentPanel.cs
- SocketException.cs
- __Filters.cs
- LocalizableResourceBuilder.cs
- MappingMetadataHelper.cs
- AbandonedMutexException.cs
- PropertyChangedEventManager.cs
- GPPOINT.cs
- SevenBitStream.cs
- XhtmlBasicObjectListAdapter.cs
- ProcessModelSection.cs
- ProcessHostConfigUtils.cs
- PassportPrincipal.cs
- DbParameterCollectionHelper.cs
- XmlSignatureManifest.cs
- AuthenticatedStream.cs
- WsdlParser.cs
- RenderCapability.cs
- IISUnsafeMethods.cs
- FileAuthorizationModule.cs
- CqlLexer.cs
- MailWriter.cs
- IriParsingElement.cs
- ScriptingJsonSerializationSection.cs
- XmlDictionaryString.cs
- ModifiableIteratorCollection.cs
- JsonObjectDataContract.cs
- ActivityPreviewDesigner.cs
- DataGridTablesFactory.cs
- VisualStyleRenderer.cs
- MemoryMappedViewAccessor.cs
- CopyAttributesAction.cs
- SoapHeaders.cs
- DesignerSerializationVisibilityAttribute.cs
- CroppedBitmap.cs
- NativeWindow.cs
- DataGridViewColumn.cs
- OleDbCommandBuilder.cs
- ActionFrame.cs
- SqlCacheDependencySection.cs
- WebBrowserDocumentCompletedEventHandler.cs
- CompleteWizardStep.cs
- LoadedOrUnloadedOperation.cs
- XmlnsDictionary.cs
- ValidationErrorCollection.cs
- Transform3D.cs
- FlowDocumentView.cs
- ToolTipService.cs
- LambdaCompiler.Statements.cs
- ArgumentException.cs
- HtmlTextBoxAdapter.cs
- FilterQuery.cs
- NumericExpr.cs
- SQLDecimalStorage.cs
- RegexCompiler.cs
- ScriptMethodAttribute.cs
- CqlLexer.cs
- MsmqNonTransactedPoisonHandler.cs
- CharacterMetricsDictionary.cs
- AnnotationStore.cs
- SizeIndependentAnimationStorage.cs
- _DynamicWinsockMethods.cs
- CssTextWriter.cs
- GenericWebPart.cs
- IssuanceTokenProviderState.cs