Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / Internal / DescendantOverDescendantQuery.cs / 1305376 / DescendantOverDescendantQuery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; // DescendantOverDescendantQuery: for each input it looks for the topmost descendents that matches to ns:name // This is posible when query which has this query as its input (child query) is descendent as well. // Work of this query doesn't depend on DOD of its input. // It doesn't garate DOD of the output even when input is DOD. internal sealed class DescendantOverDescendantQuery : DescendantBaseQuery { private int level = 0; public DescendantOverDescendantQuery(Query qyParent, bool matchSelf, string name, string prefix, XPathNodeType typeTest, bool abbrAxis) : base(qyParent, name, prefix, typeTest, matchSelf, abbrAxis) {} private DescendantOverDescendantQuery(DescendantOverDescendantQuery other) : base(other) { this.level = other.level; } public override void Reset() { level = 0; base.Reset(); } public override XPathNavigator Advance() { while (true) { if (level == 0) { currentNode = qyInput.Advance(); position = 0; if (currentNode == null) { return null; } if (matchSelf && matches(currentNode)) { position = 1; return currentNode; } currentNode = currentNode.Clone(); if (! MoveToFirstChild()) { continue; } } else { if (!MoveUpUntillNext()) { continue; } } do { if (matches(currentNode)) { position++; return currentNode; } } while (MoveToFirstChild()); } } private bool MoveToFirstChild() { if (currentNode.MoveToFirstChild()) { level++; return true; } return false; } private bool MoveUpUntillNext() { // move up untill we can move next while (! currentNode.MoveToNext()) { -- level; if (level == 0) { return false; } bool result = currentNode.MoveToParent(); Debug.Assert(result, "Algorithm error, We always should be able to move up if level > 0"); } return true; } public override XPathNodeIterator Clone() { return new DescendantOverDescendantQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; // DescendantOverDescendantQuery: for each input it looks for the topmost descendents that matches to ns:name // This is posible when query which has this query as its input (child query) is descendent as well. // Work of this query doesn't depend on DOD of its input. // It doesn't garate DOD of the output even when input is DOD. internal sealed class DescendantOverDescendantQuery : DescendantBaseQuery { private int level = 0; public DescendantOverDescendantQuery(Query qyParent, bool matchSelf, string name, string prefix, XPathNodeType typeTest, bool abbrAxis) : base(qyParent, name, prefix, typeTest, matchSelf, abbrAxis) {} private DescendantOverDescendantQuery(DescendantOverDescendantQuery other) : base(other) { this.level = other.level; } public override void Reset() { level = 0; base.Reset(); } public override XPathNavigator Advance() { while (true) { if (level == 0) { currentNode = qyInput.Advance(); position = 0; if (currentNode == null) { return null; } if (matchSelf && matches(currentNode)) { position = 1; return currentNode; } currentNode = currentNode.Clone(); if (! MoveToFirstChild()) { continue; } } else { if (!MoveUpUntillNext()) { continue; } } do { if (matches(currentNode)) { position++; return currentNode; } } while (MoveToFirstChild()); } } private bool MoveToFirstChild() { if (currentNode.MoveToFirstChild()) { level++; return true; } return false; } private bool MoveUpUntillNext() { // move up untill we can move next while (! currentNode.MoveToNext()) { -- level; if (level == 0) { return false; } bool result = currentNode.MoveToParent(); Debug.Assert(result, "Algorithm error, We always should be able to move up if level > 0"); } return true; } public override XPathNodeIterator Clone() { return new DescendantOverDescendantQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
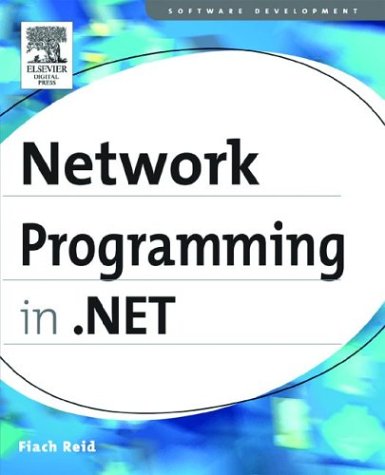
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChangeConflicts.cs
- PriorityItem.cs
- DispatcherExceptionFilterEventArgs.cs
- _OSSOCK.cs
- Debug.cs
- HttpCookie.cs
- MissingMethodException.cs
- ObjectItemConventionAssemblyLoader.cs
- Bidi.cs
- MimeTypeMapper.cs
- IsolatedStorage.cs
- ReceiveActivityDesigner.cs
- ObjectConverter.cs
- StylusPointPropertyInfo.cs
- ServiceOperationWrapper.cs
- WebPartCancelEventArgs.cs
- SystemException.cs
- DesignerActionGlyph.cs
- DeploymentExceptionMapper.cs
- DbMetaDataFactory.cs
- RSAOAEPKeyExchangeFormatter.cs
- RegexCode.cs
- QuadraticBezierSegment.cs
- AsymmetricKeyExchangeFormatter.cs
- ShutDownListener.cs
- HebrewNumber.cs
- IPAddress.cs
- ResourcePermissionBaseEntry.cs
- EntityContainerRelationshipSet.cs
- OleDbConnection.cs
- Ipv6Element.cs
- ExpressionTable.cs
- DrawingAttributeSerializer.cs
- SoapFault.cs
- _PooledStream.cs
- ChannelManagerService.cs
- XPathNavigatorKeyComparer.cs
- DataRowComparer.cs
- RegisteredArrayDeclaration.cs
- DocumentGrid.cs
- DoubleLinkList.cs
- UserControl.cs
- IdentityNotMappedException.cs
- PasswordRecovery.cs
- HostnameComparisonMode.cs
- MetadataExchangeBindings.cs
- MultiAsyncResult.cs
- WebPartDisplayMode.cs
- FontSourceCollection.cs
- PlainXmlSerializer.cs
- WinFormsUtils.cs
- ProjectionPathSegment.cs
- Trace.cs
- WindowCollection.cs
- RemotingException.cs
- FixedSOMElement.cs
- ColorKeyFrameCollection.cs
- StandardOleMarshalObject.cs
- HandoffBehavior.cs
- FileFormatException.cs
- IntSecurity.cs
- QilLiteral.cs
- AnnotationDocumentPaginator.cs
- URLString.cs
- Compiler.cs
- GenerateTemporaryTargetAssembly.cs
- NavigatingCancelEventArgs.cs
- _TimerThread.cs
- CompilationRelaxations.cs
- ILGen.cs
- TraceProvider.cs
- CompilerParameters.cs
- EventManager.cs
- SemanticKeyElement.cs
- MsmqTransportSecurity.cs
- DataGridAutoFormatDialog.cs
- ResXResourceWriter.cs
- IncrementalReadDecoders.cs
- DataGridAutoGeneratingColumnEventArgs.cs
- WebBrowsableAttribute.cs
- UntrustedRecipientException.cs
- AccessViolationException.cs
- CharacterString.cs
- XmlSchemaAppInfo.cs
- ContainerUtilities.cs
- GeneratedContractType.cs
- StringTraceRecord.cs
- FormViewRow.cs
- XmlObjectSerializerReadContextComplexJson.cs
- MobileControlPersister.cs
- PluggableProtocol.cs
- FixedSOMTableRow.cs
- XmlNullResolver.cs
- TableCell.cs
- MimeFormatter.cs
- BitVector32.cs
- DeviceContext.cs
- FixedSOMLineCollection.cs
- PrintDialog.cs
- EmulateRecognizeCompletedEventArgs.cs