Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / IO / FileFormatException.cs / 1 / FileFormatException.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: The FileFormatException class is thrown when an input file or a data stream that is supposed to conform // to a certain file format specification is malformed. // // History: // 10/21/2004 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Windows; using MS.Internal.WindowsBase; namespace System.IO { ////// The FileFormatException class is thrown when an input file or a data stream that is supposed to conform /// to a certain file format specification is malformed. /// [Serializable()] public class FileFormatException : FormatException, ISerializable { ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance to a system-supplied message that describes the error, /// such as "An input file or a data stream does not conform to the expected file format specification." /// This message takes into account the current system culture. /// public FileFormatException() : base(SR.Get(SRID.FileFormatException)) {} ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// /// The message that describes the error. public FileFormatException(string message) : base(message) {} ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// The InnerException property is initialized using the innerException parameter. /// /// The error message that explains the reason for the exception. /// The exception that is the cause of the current exception. public FileFormatException(string message, Exception innerException) : base(message, innerException) {} ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance to a system-supplied message that describes the error and includes the file name, /// such as "The file 'sourceUri' does not conform to the expected file format specification." /// This message takes into account the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// /// The Uri of a file that caused this error. public FileFormatException(Uri sourceUri) : base( sourceUri == null ? SR.Get(SRID.FileFormatException) : SR.Get(SRID.FileFormatExceptionWithFileName, sourceUri)) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// /// The Uri of a file that caused this error. /// The message that describes the error. public FileFormatException(Uri sourceUri, String message) : base(message) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance to a system-supplied message that describes the error and includes the file name, /// such as "The file 'sourceUri' does not conform to the expected file format specification." /// This message takes into account the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The Uri of a file that caused this error. /// The exception that is the cause of the current exception. public FileFormatException(Uri sourceUri, Exception innerException) : base( sourceUri == null ? SR.Get(SRID.FileFormatException) : SR.Get(SRID.FileFormatExceptionWithFileName, sourceUri), innerException) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The Uri of a file that caused this error. /// The message that describes the error. /// The exception that is the cause of the current exception. public FileFormatException(Uri sourceUri, String message, Exception innerException) : base(message, innerException) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class and initializes it with serialized data. /// This constructor is called during deserialization to reconstitute the exception object transmitted over a stream. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected FileFormatException(SerializationInfo info, StreamingContext context) : base(info, context) { string sourceUriString = info.GetString("SourceUri"); if (sourceUriString != null) _sourceUri = new Uri(sourceUriString, UriKind.RelativeOrAbsolute); } ////// Sets the SerializationInfo object with the file name and additional exception information. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. ////// Critical: calls Exception.GetObjectData which LinkDemands /// PublicOK: a demand exists here /// [SecurityCritical] [SecurityPermissionAttribute(SecurityAction.Demand, Flags = SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); Uri sourceUri = SourceUri; info.AddValue( "SourceUri", sourceUri == null ? null : sourceUri.GetComponents(UriComponents.SerializationInfoString, UriFormat.SafeUnescaped), typeof(String) ); } ////// Returns the name of a file that caused this exception. This property may be equal to an empty string /// if obtaining the file path that caused the error was not possible. /// ///The file name. public Uri SourceUri { get { // Security: defense in depth, make sure the caller has path discovery permission for local file case. if (_sourceUri != null && _sourceUri.IsAbsoluteUri && _sourceUri.IsFile) SecurityHelper.DemandPathDiscovery(_sourceUri.LocalPath); return _sourceUri; } } private Uri _sourceUri; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: The FileFormatException class is thrown when an input file or a data stream that is supposed to conform // to a certain file format specification is malformed. // // History: // 10/21/2004 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Windows; using MS.Internal.WindowsBase; namespace System.IO { ////// The FileFormatException class is thrown when an input file or a data stream that is supposed to conform /// to a certain file format specification is malformed. /// [Serializable()] public class FileFormatException : FormatException, ISerializable { ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance to a system-supplied message that describes the error, /// such as "An input file or a data stream does not conform to the expected file format specification." /// This message takes into account the current system culture. /// public FileFormatException() : base(SR.Get(SRID.FileFormatException)) {} ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// /// The message that describes the error. public FileFormatException(string message) : base(message) {} ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// The InnerException property is initialized using the innerException parameter. /// /// The error message that explains the reason for the exception. /// The exception that is the cause of the current exception. public FileFormatException(string message, Exception innerException) : base(message, innerException) {} ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance to a system-supplied message that describes the error and includes the file name, /// such as "The file 'sourceUri' does not conform to the expected file format specification." /// This message takes into account the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// /// The Uri of a file that caused this error. public FileFormatException(Uri sourceUri) : base( sourceUri == null ? SR.Get(SRID.FileFormatException) : SR.Get(SRID.FileFormatExceptionWithFileName, sourceUri)) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// /// The Uri of a file that caused this error. /// The message that describes the error. public FileFormatException(Uri sourceUri, String message) : base(message) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance to a system-supplied message that describes the error and includes the file name, /// such as "The file 'sourceUri' does not conform to the expected file format specification." /// This message takes into account the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The Uri of a file that caused this error. /// The exception that is the cause of the current exception. public FileFormatException(Uri sourceUri, Exception innerException) : base( sourceUri == null ? SR.Get(SRID.FileFormatException) : SR.Get(SRID.FileFormatExceptionWithFileName, sourceUri), innerException) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The SourceUri property is initialized using the sourceUri parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The Uri of a file that caused this error. /// The message that describes the error. /// The exception that is the cause of the current exception. public FileFormatException(Uri sourceUri, String message, Exception innerException) : base(message, innerException) { _sourceUri = sourceUri; } ////// Creates a new instance of FileFormatException class and initializes it with serialized data. /// This constructor is called during deserialization to reconstitute the exception object transmitted over a stream. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected FileFormatException(SerializationInfo info, StreamingContext context) : base(info, context) { string sourceUriString = info.GetString("SourceUri"); if (sourceUriString != null) _sourceUri = new Uri(sourceUriString, UriKind.RelativeOrAbsolute); } ////// Sets the SerializationInfo object with the file name and additional exception information. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. ////// Critical: calls Exception.GetObjectData which LinkDemands /// PublicOK: a demand exists here /// [SecurityCritical] [SecurityPermissionAttribute(SecurityAction.Demand, Flags = SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); Uri sourceUri = SourceUri; info.AddValue( "SourceUri", sourceUri == null ? null : sourceUri.GetComponents(UriComponents.SerializationInfoString, UriFormat.SafeUnescaped), typeof(String) ); } ////// Returns the name of a file that caused this exception. This property may be equal to an empty string /// if obtaining the file path that caused the error was not possible. /// ///The file name. public Uri SourceUri { get { // Security: defense in depth, make sure the caller has path discovery permission for local file case. if (_sourceUri != null && _sourceUri.IsAbsoluteUri && _sourceUri.IsFile) SecurityHelper.DemandPathDiscovery(_sourceUri.LocalPath); return _sourceUri; } } private Uri _sourceUri; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
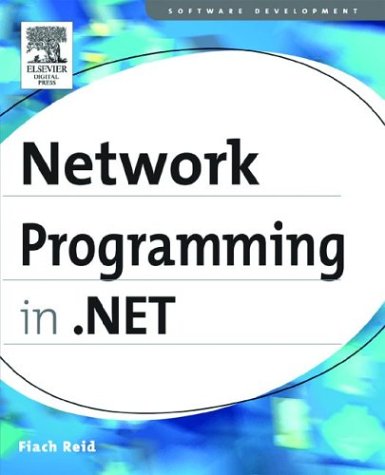
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VisualStyleTypesAndProperties.cs
- ArglessEventHandlerProxy.cs
- WebPartVerbCollection.cs
- CodeMemberProperty.cs
- SystemUdpStatistics.cs
- IsolationInterop.cs
- ArglessEventHandlerProxy.cs
- RelatedPropertyManager.cs
- HttpHandlerActionCollection.cs
- TransformationRules.cs
- LinkedResourceCollection.cs
- ZipIOExtraField.cs
- TextEffect.cs
- PathTooLongException.cs
- exports.cs
- CompressionTransform.cs
- ListComponentEditorPage.cs
- CodePageUtils.cs
- DbConnectionPoolOptions.cs
- ParameterElementCollection.cs
- WindowsRichEditRange.cs
- AssemblyCache.cs
- WindowsListViewItem.cs
- MessageQueueInstaller.cs
- FtpWebResponse.cs
- PathStreamGeometryContext.cs
- ChunkedMemoryStream.cs
- Variable.cs
- Listbox.cs
- CodeSnippetStatement.cs
- SnapLine.cs
- SecurityKeyIdentifier.cs
- CmsInterop.cs
- IteratorFilter.cs
- SqlBulkCopy.cs
- DataBindingCollectionConverter.cs
- DependencyPropertyDescriptor.cs
- CodeSnippetCompileUnit.cs
- BaseTypeViewSchema.cs
- IteratorDescriptor.cs
- JsonReaderDelegator.cs
- ParameterToken.cs
- TypefaceCollection.cs
- HttpCacheParams.cs
- GradientStop.cs
- RuleInfoComparer.cs
- LinqDataSourceView.cs
- NegotiateStream.cs
- XmlParserContext.cs
- WebBrowserSiteBase.cs
- FlowPanelDesigner.cs
- ProcessDesigner.cs
- EventLogInformation.cs
- Roles.cs
- Camera.cs
- _NegotiateClient.cs
- ComboBoxItem.cs
- SectionVisual.cs
- UniqueConstraint.cs
- Char.cs
- SAPICategories.cs
- XmlValidatingReaderImpl.cs
- SqlParameter.cs
- AssemblyHelper.cs
- ManagementEventWatcher.cs
- DataGridCellItemAutomationPeer.cs
- SqlMethodAttribute.cs
- ToolStripPanel.cs
- baseaxisquery.cs
- Trace.cs
- EmptyControlCollection.cs
- XmlSchemaElement.cs
- WindowsTab.cs
- Point3DConverter.cs
- Vector3dCollection.cs
- CompilerWrapper.cs
- WebBrowserUriTypeConverter.cs
- SafeRegistryHandle.cs
- GridViewRow.cs
- DiscardableAttribute.cs
- ListView.cs
- XmlUrlResolver.cs
- StackSpiller.Generated.cs
- CTreeGenerator.cs
- DataServiceExpressionVisitor.cs
- SpellerInterop.cs
- RequestCacheEntry.cs
- Margins.cs
- Solver.cs
- XmlBindingWorker.cs
- MailSettingsSection.cs
- DockingAttribute.cs
- DrawingCollection.cs
- WindowsFormsSynchronizationContext.cs
- HWStack.cs
- ScaleTransform3D.cs
- TreeViewImageGenerator.cs
- CryptoSession.cs
- loginstatus.cs
- GenericUI.cs